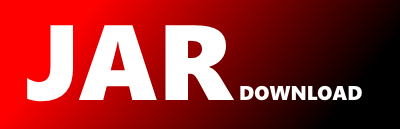
com.okta.sdk.resource.model.ApplicationCredentialsOAuthClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.OAuthEndpointAuthenticationMethod;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* ApplicationCredentialsOAuthClient
*/
@JsonPropertyOrder({ ApplicationCredentialsOAuthClient.JSON_PROPERTY_AUTO_KEY_ROTATION,
ApplicationCredentialsOAuthClient.JSON_PROPERTY_CLIENT_ID,
ApplicationCredentialsOAuthClient.JSON_PROPERTY_CLIENT_SECRET,
ApplicationCredentialsOAuthClient.JSON_PROPERTY_PKCE_REQUIRED,
ApplicationCredentialsOAuthClient.JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHOD })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ApplicationCredentialsOAuthClient implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AUTO_KEY_ROTATION = "autoKeyRotation";
private Boolean autoKeyRotation = true;
public static final String JSON_PROPERTY_CLIENT_ID = "client_id";
private String clientId;
public static final String JSON_PROPERTY_CLIENT_SECRET = "client_secret";
private String clientSecret;
public static final String JSON_PROPERTY_PKCE_REQUIRED = "pkce_required";
private Boolean pkceRequired = true;
public static final String JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHOD = "token_endpoint_auth_method";
private OAuthEndpointAuthenticationMethod tokenEndpointAuthMethod = OAuthEndpointAuthenticationMethod.CLIENT_SECRET_BASIC;
public ApplicationCredentialsOAuthClient() {
}
public ApplicationCredentialsOAuthClient autoKeyRotation(Boolean autoKeyRotation) {
this.autoKeyRotation = autoKeyRotation;
return this;
}
/**
* Requested key rotation mode
*
* @return autoKeyRotation
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Requested key rotation mode")
@JsonProperty(JSON_PROPERTY_AUTO_KEY_ROTATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAutoKeyRotation() {
return autoKeyRotation;
}
@JsonProperty(JSON_PROPERTY_AUTO_KEY_ROTATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAutoKeyRotation(Boolean autoKeyRotation) {
this.autoKeyRotation = autoKeyRotation;
}
public ApplicationCredentialsOAuthClient clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Unique identifier for the OAuth 2.0 client app > **Notes:** > * If you don't specify the
* `client_id`, this immutable property is populated with the [Application instance
* ID](/openapi/okta-management/management/tag/Application/#tag/Application/operation/getApplication!c=200&path=4/id&t=response).
* > * The `client_id` must consist of alphanumeric characters or the following special characters:
* `$-_.+!*'(),`. > * You can't use the reserved word `ALL_CLIENTS`.
*
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the OAuth 2.0 client app > **Notes:** > * If you don't specify the `client_id`, this immutable property is populated with the [Application instance ID](/openapi/okta-management/management/tag/Application/#tag/Application/operation/getApplication!c=200&path=4/id&t=response). > * The `client_id` must consist of alphanumeric characters or the following special characters: `$-_.+!*'(),`. > * You can't use the reserved word `ALL_CLIENTS`.")
@JsonProperty(JSON_PROPERTY_CLIENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getClientId() {
return clientId;
}
@JsonProperty(JSON_PROPERTY_CLIENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setClientId(String clientId) {
this.clientId = clientId;
}
public ApplicationCredentialsOAuthClient clientSecret(String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
/**
* OAuth 2.0 client secret string (used for confidential clients) > **Notes:** If a `client_secret`
* isn't provided on creation, and the `token_endpoint_auth_method` requires one, Okta generates a
* random `client_secret` for the client app. > The `client_secret` is only shown when an
* OAuth 2.0 client app is created or updated (and only if the `token_endpoint_auth_method` requires a
* client secret).
*
* @return clientSecret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "OAuth 2.0 client secret string (used for confidential clients) > **Notes:** If a `client_secret` isn't provided on creation, and the `token_endpoint_auth_method` requires one, Okta generates a random `client_secret` for the client app. > The `client_secret` is only shown when an OAuth 2.0 client app is created or updated (and only if the `token_endpoint_auth_method` requires a client secret).")
@JsonProperty(JSON_PROPERTY_CLIENT_SECRET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getClientSecret() {
return clientSecret;
}
@JsonProperty(JSON_PROPERTY_CLIENT_SECRET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setClientSecret(String clientSecret) {
this.clientSecret = clientSecret;
}
public ApplicationCredentialsOAuthClient pkceRequired(Boolean pkceRequired) {
this.pkceRequired = pkceRequired;
return this;
}
/**
* Requires Proof Key for Code Exchange (PKCE) for additional verification. If
* `token_endpoint_auth_method` is `none`, then `pkce_required` must be
* `true`. The default is `true` for browser and native app types.
*
* @return pkceRequired
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Requires Proof Key for Code Exchange (PKCE) for additional verification. If `token_endpoint_auth_method` is `none`, then `pkce_required` must be `true`. The default is `true` for browser and native app types.")
@JsonProperty(JSON_PROPERTY_PKCE_REQUIRED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getPkceRequired() {
return pkceRequired;
}
@JsonProperty(JSON_PROPERTY_PKCE_REQUIRED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPkceRequired(Boolean pkceRequired) {
this.pkceRequired = pkceRequired;
}
public ApplicationCredentialsOAuthClient tokenEndpointAuthMethod(
OAuthEndpointAuthenticationMethod tokenEndpointAuthMethod) {
this.tokenEndpointAuthMethod = tokenEndpointAuthMethod;
return this;
}
/**
* Get tokenEndpointAuthMethod
*
* @return tokenEndpointAuthMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHOD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OAuthEndpointAuthenticationMethod getTokenEndpointAuthMethod() {
return tokenEndpointAuthMethod;
}
@JsonProperty(JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHOD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTokenEndpointAuthMethod(OAuthEndpointAuthenticationMethod tokenEndpointAuthMethod) {
this.tokenEndpointAuthMethod = tokenEndpointAuthMethod;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ApplicationCredentialsOAuthClient applicationCredentialsOAuthClient = (ApplicationCredentialsOAuthClient) o;
return Objects.equals(this.autoKeyRotation, applicationCredentialsOAuthClient.autoKeyRotation)
&& Objects.equals(this.clientId, applicationCredentialsOAuthClient.clientId)
&& Objects.equals(this.clientSecret, applicationCredentialsOAuthClient.clientSecret)
&& Objects.equals(this.pkceRequired, applicationCredentialsOAuthClient.pkceRequired) && Objects.equals(
this.tokenEndpointAuthMethod, applicationCredentialsOAuthClient.tokenEndpointAuthMethod);
// ;
}
@Override
public int hashCode() {
return Objects.hash(autoKeyRotation, clientId, clientSecret, pkceRequired, tokenEndpointAuthMethod);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ApplicationCredentialsOAuthClient {\n");
sb.append(" autoKeyRotation: ").append(toIndentedString(autoKeyRotation)).append("\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" clientSecret: ").append(toIndentedString(clientSecret)).append("\n");
sb.append(" pkceRequired: ").append(toIndentedString(pkceRequired)).append("\n");
sb.append(" tokenEndpointAuthMethod: ").append(toIndentedString(tokenEndpointAuthMethod)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy