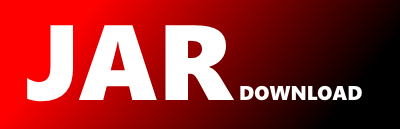
com.okta.sdk.resource.model.ApplicationLinks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.AccessPolicyLink;
import com.okta.sdk.resource.model.GroupsLink;
import com.okta.sdk.resource.model.HelpLink;
import com.okta.sdk.resource.model.HrefObject;
import com.okta.sdk.resource.model.HrefObjectActivateLink;
import com.okta.sdk.resource.model.HrefObjectDeactivateLink;
import com.okta.sdk.resource.model.HrefObjectSelfLink;
import com.okta.sdk.resource.model.MetadataLink;
import com.okta.sdk.resource.model.UsersLink;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Discoverable resources related to the app
*/
@ApiModel(description = "Discoverable resources related to the app")
@JsonPropertyOrder({ ApplicationLinks.JSON_PROPERTY_ACCESS_POLICY, ApplicationLinks.JSON_PROPERTY_ACTIVATE,
ApplicationLinks.JSON_PROPERTY_APP_LINKS, ApplicationLinks.JSON_PROPERTY_DEACTIVATE,
ApplicationLinks.JSON_PROPERTY_GROUPS, ApplicationLinks.JSON_PROPERTY_HELP, ApplicationLinks.JSON_PROPERTY_LOGO,
ApplicationLinks.JSON_PROPERTY_METADATA, ApplicationLinks.JSON_PROPERTY_SELF,
ApplicationLinks.JSON_PROPERTY_USERS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ApplicationLinks implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACCESS_POLICY = "accessPolicy";
private AccessPolicyLink accessPolicy;
public static final String JSON_PROPERTY_ACTIVATE = "activate";
private HrefObjectActivateLink activate;
public static final String JSON_PROPERTY_APP_LINKS = "appLinks";
private List appLinks = null;
public static final String JSON_PROPERTY_DEACTIVATE = "deactivate";
private HrefObjectDeactivateLink deactivate;
public static final String JSON_PROPERTY_GROUPS = "groups";
private GroupsLink groups;
public static final String JSON_PROPERTY_HELP = "help";
private HelpLink help;
public static final String JSON_PROPERTY_LOGO = "logo";
private List logo = null;
public static final String JSON_PROPERTY_METADATA = "metadata";
private MetadataLink metadata;
public static final String JSON_PROPERTY_SELF = "self";
private HrefObjectSelfLink self;
public static final String JSON_PROPERTY_USERS = "users";
private UsersLink users;
public ApplicationLinks() {
}
public ApplicationLinks accessPolicy(AccessPolicyLink accessPolicy) {
this.accessPolicy = accessPolicy;
return this;
}
/**
* Get accessPolicy
*
* @return accessPolicy
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACCESS_POLICY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AccessPolicyLink getAccessPolicy() {
return accessPolicy;
}
@JsonProperty(JSON_PROPERTY_ACCESS_POLICY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccessPolicy(AccessPolicyLink accessPolicy) {
this.accessPolicy = accessPolicy;
}
public ApplicationLinks activate(HrefObjectActivateLink activate) {
this.activate = activate;
return this;
}
/**
* Get activate
*
* @return activate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObjectActivateLink getActivate() {
return activate;
}
@JsonProperty(JSON_PROPERTY_ACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActivate(HrefObjectActivateLink activate) {
this.activate = activate;
}
public ApplicationLinks appLinks(List appLinks) {
this.appLinks = appLinks;
return this;
}
public ApplicationLinks addappLinksItem(HrefObject appLinksItem) {
if (this.appLinks == null) {
this.appLinks = new ArrayList<>();
}
this.appLinks.add(appLinksItem);
return this;
}
/**
* List of app link resources
*
* @return appLinks
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of app link resources")
@JsonProperty(JSON_PROPERTY_APP_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAppLinks() {
return appLinks;
}
@JsonProperty(JSON_PROPERTY_APP_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAppLinks(List appLinks) {
this.appLinks = appLinks;
}
public ApplicationLinks deactivate(HrefObjectDeactivateLink deactivate) {
this.deactivate = deactivate;
return this;
}
/**
* Get deactivate
*
* @return deactivate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DEACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObjectDeactivateLink getDeactivate() {
return deactivate;
}
@JsonProperty(JSON_PROPERTY_DEACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeactivate(HrefObjectDeactivateLink deactivate) {
this.deactivate = deactivate;
}
public ApplicationLinks groups(GroupsLink groups) {
this.groups = groups;
return this;
}
/**
* Get groups
*
* @return groups
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_GROUPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public GroupsLink getGroups() {
return groups;
}
@JsonProperty(JSON_PROPERTY_GROUPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGroups(GroupsLink groups) {
this.groups = groups;
}
public ApplicationLinks help(HelpLink help) {
this.help = help;
return this;
}
/**
* Get help
*
* @return help
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HELP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HelpLink getHelp() {
return help;
}
@JsonProperty(JSON_PROPERTY_HELP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHelp(HelpLink help) {
this.help = help;
}
public ApplicationLinks logo(List logo) {
this.logo = logo;
return this;
}
public ApplicationLinks addlogoItem(HrefObject logoItem) {
if (this.logo == null) {
this.logo = new ArrayList<>();
}
this.logo.add(logoItem);
return this;
}
/**
* List of app logo resources
*
* @return logo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of app logo resources")
@JsonProperty(JSON_PROPERTY_LOGO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getLogo() {
return logo;
}
@JsonProperty(JSON_PROPERTY_LOGO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLogo(List logo) {
this.logo = logo;
}
public ApplicationLinks metadata(MetadataLink metadata) {
this.metadata = metadata;
return this;
}
/**
* Get metadata
*
* @return metadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MetadataLink getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(MetadataLink metadata) {
this.metadata = metadata;
}
public ApplicationLinks self(HrefObjectSelfLink self) {
this.self = self;
return this;
}
/**
* Get self
*
* @return self
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObjectSelfLink getSelf() {
return self;
}
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSelf(HrefObjectSelfLink self) {
this.self = self;
}
public ApplicationLinks users(UsersLink users) {
this.users = users;
return this;
}
/**
* Get users
*
* @return users
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_USERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UsersLink getUsers() {
return users;
}
@JsonProperty(JSON_PROPERTY_USERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUsers(UsersLink users) {
this.users = users;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ApplicationLinks applicationLinks = (ApplicationLinks) o;
return Objects.equals(this.accessPolicy, applicationLinks.accessPolicy)
&& Objects.equals(this.activate, applicationLinks.activate)
&& Objects.equals(this.appLinks, applicationLinks.appLinks)
&& Objects.equals(this.deactivate, applicationLinks.deactivate)
&& Objects.equals(this.groups, applicationLinks.groups)
&& Objects.equals(this.help, applicationLinks.help) && Objects.equals(this.logo, applicationLinks.logo)
&& Objects.equals(this.metadata, applicationLinks.metadata)
&& Objects.equals(this.self, applicationLinks.self)
&& Objects.equals(this.users, applicationLinks.users);
// ;
}
@Override
public int hashCode() {
return Objects.hash(accessPolicy, activate, appLinks, deactivate, groups, help, logo, metadata, self, users);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ApplicationLinks {\n");
sb.append(" accessPolicy: ").append(toIndentedString(accessPolicy)).append("\n");
sb.append(" activate: ").append(toIndentedString(activate)).append("\n");
sb.append(" appLinks: ").append(toIndentedString(appLinks)).append("\n");
sb.append(" deactivate: ").append(toIndentedString(deactivate)).append("\n");
sb.append(" groups: ").append(toIndentedString(groups)).append("\n");
sb.append(" help: ").append(toIndentedString(help)).append("\n");
sb.append(" logo: ").append(toIndentedString(logo)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" self: ").append(toIndentedString(self)).append("\n");
sb.append(" users: ").append(toIndentedString(users)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy