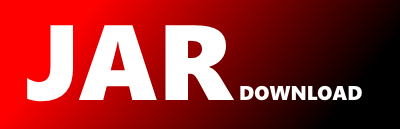
com.okta.sdk.resource.model.AuthenticatorMethodOtp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.AuthenticatorMethodProperty;
import com.okta.sdk.resource.model.AuthenticatorMethodType;
import com.okta.sdk.resource.model.AuthenticatorMethodWithVerifiableProperties;
import com.okta.sdk.resource.model.LifecycleStatus;
import com.okta.sdk.resource.model.LinksSelfAndLifecycle;
import com.okta.sdk.resource.model.OtpProtocol;
import com.okta.sdk.resource.model.OtpTotpAlgorithm;
import com.okta.sdk.resource.model.OtpTotpEncoding;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* AuthenticatorMethodOtp
*/
@JsonPropertyOrder({ AuthenticatorMethodOtp.JSON_PROPERTY_ACCEPTABLE_ADJACENT_INTERVALS,
AuthenticatorMethodOtp.JSON_PROPERTY_ALGORITHM, AuthenticatorMethodOtp.JSON_PROPERTY_ENCODING,
AuthenticatorMethodOtp.JSON_PROPERTY_FACTOR_PROFILE_ID, AuthenticatorMethodOtp.JSON_PROPERTY_PASS_CODE_LENGTH,
AuthenticatorMethodOtp.JSON_PROPERTY_PROTOCOL, AuthenticatorMethodOtp.JSON_PROPERTY_TIME_INTERVAL_IN_SECONDS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.EXISTING_PROPERTY, property = "type", visible = true, defaultImpl = AuthenticatorMethodOtp.class)
public class AuthenticatorMethodOtp extends AuthenticatorMethodWithVerifiableProperties implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACCEPTABLE_ADJACENT_INTERVALS = "acceptableAdjacentIntervals";
private Integer acceptableAdjacentIntervals;
public static final String JSON_PROPERTY_ALGORITHM = "algorithm";
private OtpTotpAlgorithm algorithm;
public static final String JSON_PROPERTY_ENCODING = "encoding";
private OtpTotpEncoding encoding;
public static final String JSON_PROPERTY_FACTOR_PROFILE_ID = "factorProfileId";
private String factorProfileId;
public static final String JSON_PROPERTY_PASS_CODE_LENGTH = "passCodeLength";
private Integer passCodeLength;
public static final String JSON_PROPERTY_PROTOCOL = "protocol";
private OtpProtocol protocol;
public static final String JSON_PROPERTY_TIME_INTERVAL_IN_SECONDS = "timeIntervalInSeconds";
private Integer timeIntervalInSeconds;
public AuthenticatorMethodOtp() {
}
public AuthenticatorMethodOtp acceptableAdjacentIntervals(Integer acceptableAdjacentIntervals) {
this.acceptableAdjacentIntervals = acceptableAdjacentIntervals;
return this;
}
/**
* The number of acceptable adjacent intervals, also known as the clock drift interval. This setting allows you to
* build in tolerance for any time difference between the token and the server. For example, with a
* `timeIntervalInSeconds` of 60 seconds and an `acceptableAdjacentIntervals` value of 5, Okta
* accepts passcodes within 300 seconds (60 * 5) before or after the end user enters their code. minimum: 0 maximum:
* 10
*
* @return acceptableAdjacentIntervals
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of acceptable adjacent intervals, also known as the clock drift interval. This setting allows you to build in tolerance for any time difference between the token and the server. For example, with a `timeIntervalInSeconds` of 60 seconds and an `acceptableAdjacentIntervals` value of 5, Okta accepts passcodes within 300 seconds (60 * 5) before or after the end user enters their code.")
@JsonProperty(JSON_PROPERTY_ACCEPTABLE_ADJACENT_INTERVALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getAcceptableAdjacentIntervals() {
return acceptableAdjacentIntervals;
}
@JsonProperty(JSON_PROPERTY_ACCEPTABLE_ADJACENT_INTERVALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAcceptableAdjacentIntervals(Integer acceptableAdjacentIntervals) {
this.acceptableAdjacentIntervals = acceptableAdjacentIntervals;
}
public AuthenticatorMethodOtp algorithm(OtpTotpAlgorithm algorithm) {
this.algorithm = algorithm;
return this;
}
/**
* Get algorithm
*
* @return algorithm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OtpTotpAlgorithm getAlgorithm() {
return algorithm;
}
@JsonProperty(JSON_PROPERTY_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAlgorithm(OtpTotpAlgorithm algorithm) {
this.algorithm = algorithm;
}
public AuthenticatorMethodOtp encoding(OtpTotpEncoding encoding) {
this.encoding = encoding;
return this;
}
/**
* Get encoding
*
* @return encoding
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENCODING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OtpTotpEncoding getEncoding() {
return encoding;
}
@JsonProperty(JSON_PROPERTY_ENCODING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEncoding(OtpTotpEncoding encoding) {
this.encoding = encoding;
}
public AuthenticatorMethodOtp factorProfileId(String factorProfileId) {
this.factorProfileId = factorProfileId;
return this;
}
/**
* The `id` value of the factor profile
*
* @return factorProfileId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "aut1nd8PQhGcQtSxB0g4", value = "The `id` value of the factor profile")
@JsonProperty(JSON_PROPERTY_FACTOR_PROFILE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFactorProfileId() {
return factorProfileId;
}
@JsonProperty(JSON_PROPERTY_FACTOR_PROFILE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFactorProfileId(String factorProfileId) {
this.factorProfileId = factorProfileId;
}
public AuthenticatorMethodOtp passCodeLength(Integer passCodeLength) {
this.passCodeLength = passCodeLength;
return this;
}
/**
* Number of digits in an OTP value minimum: 6 maximum: 10
*
* @return passCodeLength
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Number of digits in an OTP value")
@JsonProperty(JSON_PROPERTY_PASS_CODE_LENGTH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPassCodeLength() {
return passCodeLength;
}
@JsonProperty(JSON_PROPERTY_PASS_CODE_LENGTH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPassCodeLength(Integer passCodeLength) {
this.passCodeLength = passCodeLength;
}
public AuthenticatorMethodOtp protocol(OtpProtocol protocol) {
this.protocol = protocol;
return this;
}
/**
* Get protocol
*
* @return protocol
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PROTOCOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OtpProtocol getProtocol() {
return protocol;
}
@JsonProperty(JSON_PROPERTY_PROTOCOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProtocol(OtpProtocol protocol) {
this.protocol = protocol;
}
public AuthenticatorMethodOtp timeIntervalInSeconds(Integer timeIntervalInSeconds) {
this.timeIntervalInSeconds = timeIntervalInSeconds;
return this;
}
/**
* Time interval for TOTP in seconds
*
* @return timeIntervalInSeconds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Time interval for TOTP in seconds")
@JsonProperty(JSON_PROPERTY_TIME_INTERVAL_IN_SECONDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getTimeIntervalInSeconds() {
return timeIntervalInSeconds;
}
@JsonProperty(JSON_PROPERTY_TIME_INTERVAL_IN_SECONDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTimeIntervalInSeconds(Integer timeIntervalInSeconds) {
this.timeIntervalInSeconds = timeIntervalInSeconds;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AuthenticatorMethodOtp authenticatorMethodOtp = (AuthenticatorMethodOtp) o;
return Objects.equals(this.acceptableAdjacentIntervals, authenticatorMethodOtp.acceptableAdjacentIntervals)
&& Objects.equals(this.algorithm, authenticatorMethodOtp.algorithm)
&& Objects.equals(this.encoding, authenticatorMethodOtp.encoding)
&& Objects.equals(this.factorProfileId, authenticatorMethodOtp.factorProfileId)
&& Objects.equals(this.passCodeLength, authenticatorMethodOtp.passCodeLength)
&& Objects.equals(this.protocol, authenticatorMethodOtp.protocol)
&& Objects.equals(this.timeIntervalInSeconds, authenticatorMethodOtp.timeIntervalInSeconds);
// && super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(acceptableAdjacentIntervals, algorithm, encoding, factorProfileId, passCodeLength, protocol,
timeIntervalInSeconds, super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AuthenticatorMethodOtp {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" acceptableAdjacentIntervals: ").append(toIndentedString(acceptableAdjacentIntervals))
.append("\n");
sb.append(" algorithm: ").append(toIndentedString(algorithm)).append("\n");
sb.append(" encoding: ").append(toIndentedString(encoding)).append("\n");
sb.append(" factorProfileId: ").append(toIndentedString(factorProfileId)).append("\n");
sb.append(" passCodeLength: ").append(toIndentedString(passCodeLength)).append("\n");
sb.append(" protocol: ").append(toIndentedString(protocol)).append("\n");
sb.append(" timeIntervalInSeconds: ").append(toIndentedString(timeIntervalInSeconds)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy