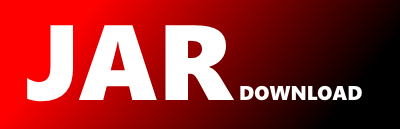
com.okta.sdk.resource.model.AuthorizationServerPolicyRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.AuthorizationServerPolicyRuleActions;
import com.okta.sdk.resource.model.AuthorizationServerPolicyRuleConditions;
import com.okta.sdk.resource.model.LinksSelfAndLifecycle;
import java.time.OffsetDateTime;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* AuthorizationServerPolicyRule
*/
@JsonPropertyOrder({ AuthorizationServerPolicyRule.JSON_PROPERTY_ACTIONS,
AuthorizationServerPolicyRule.JSON_PROPERTY_CONDITIONS, AuthorizationServerPolicyRule.JSON_PROPERTY_CREATED,
AuthorizationServerPolicyRule.JSON_PROPERTY_ID, AuthorizationServerPolicyRule.JSON_PROPERTY_LAST_UPDATED,
AuthorizationServerPolicyRule.JSON_PROPERTY_NAME, AuthorizationServerPolicyRule.JSON_PROPERTY_PRIORITY,
AuthorizationServerPolicyRule.JSON_PROPERTY_STATUS, AuthorizationServerPolicyRule.JSON_PROPERTY_SYSTEM,
AuthorizationServerPolicyRule.JSON_PROPERTY_TYPE, AuthorizationServerPolicyRule.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class AuthorizationServerPolicyRule implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACTIONS = "actions";
private AuthorizationServerPolicyRuleActions actions;
public static final String JSON_PROPERTY_CONDITIONS = "conditions";
private AuthorizationServerPolicyRuleConditions conditions;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_PRIORITY = "priority";
private Integer priority;
/**
* Status of the rule
*/
public enum StatusEnum {
ACTIVE("ACTIVE"),
INACTIVE("INACTIVE"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_STATUS = "status";
private StatusEnum status;
public static final String JSON_PROPERTY_SYSTEM = "system";
private Boolean system;
/**
* Rule type
*/
public enum TypeEnum {
RESOURCE_ACCESS("RESOURCE_ACCESS"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_TYPE = "type";
private TypeEnum type;
public static final String JSON_PROPERTY_LINKS = "_links";
private LinksSelfAndLifecycle links;
public AuthorizationServerPolicyRule() {
}
/*
* @JsonCreator public AuthorizationServerPolicyRule(
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated ) { this(); this.created = created; this.id
* = id; this.lastUpdated = lastUpdated; }
*/
public AuthorizationServerPolicyRule actions(AuthorizationServerPolicyRuleActions actions) {
this.actions = actions;
return this;
}
/**
* Get actions
*
* @return actions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuthorizationServerPolicyRuleActions getActions() {
return actions;
}
@JsonProperty(JSON_PROPERTY_ACTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActions(AuthorizationServerPolicyRuleActions actions) {
this.actions = actions;
}
public AuthorizationServerPolicyRule conditions(AuthorizationServerPolicyRuleConditions conditions) {
this.conditions = conditions;
return this;
}
/**
* Get conditions
*
* @return conditions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CONDITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuthorizationServerPolicyRuleConditions getConditions() {
return conditions;
}
@JsonProperty(JSON_PROPERTY_CONDITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setConditions(AuthorizationServerPolicyRuleConditions conditions) {
this.conditions = conditions;
}
/**
* Timestamp when the rule was created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Timestamp when the rule was created")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
/**
* Identifier of the rule
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the rule")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
/**
* Timestamp when the rule was last modified
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Timestamp when the rule was last modified")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
public AuthorizationServerPolicyRule name(String name) {
this.name = name;
return this;
}
/**
* Name of the rule
*
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the rule")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public AuthorizationServerPolicyRule priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* Priority of the rule
*
* @return priority
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Priority of the rule")
@JsonProperty(JSON_PROPERTY_PRIORITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPriority() {
return priority;
}
@JsonProperty(JSON_PROPERTY_PRIORITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPriority(Integer priority) {
this.priority = priority;
}
public AuthorizationServerPolicyRule status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Status of the rule
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Status of the rule")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public StatusEnum getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatus(StatusEnum status) {
this.status = status;
}
public AuthorizationServerPolicyRule system(Boolean system) {
this.system = system;
return this;
}
/**
* Set to `true` for system rules. You can't delete system rules.
*
* @return system
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set to `true` for system rules. You can't delete system rules.")
@JsonProperty(JSON_PROPERTY_SYSTEM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getSystem() {
return system;
}
@JsonProperty(JSON_PROPERTY_SYSTEM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSystem(Boolean system) {
this.system = system;
}
public AuthorizationServerPolicyRule type(TypeEnum type) {
this.type = type;
return this;
}
/**
* Rule type
*
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Rule type")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TypeEnum getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(TypeEnum type) {
this.type = type;
}
public AuthorizationServerPolicyRule links(LinksSelfAndLifecycle links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksSelfAndLifecycle getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(LinksSelfAndLifecycle links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AuthorizationServerPolicyRule authorizationServerPolicyRule = (AuthorizationServerPolicyRule) o;
return Objects.equals(this.actions, authorizationServerPolicyRule.actions)
&& Objects.equals(this.conditions, authorizationServerPolicyRule.conditions)
&& Objects.equals(this.created, authorizationServerPolicyRule.created)
&& Objects.equals(this.id, authorizationServerPolicyRule.id)
&& Objects.equals(this.lastUpdated, authorizationServerPolicyRule.lastUpdated)
&& Objects.equals(this.name, authorizationServerPolicyRule.name)
&& Objects.equals(this.priority, authorizationServerPolicyRule.priority)
&& Objects.equals(this.status, authorizationServerPolicyRule.status)
&& Objects.equals(this.system, authorizationServerPolicyRule.system)
&& Objects.equals(this.type, authorizationServerPolicyRule.type)
&& Objects.equals(this.links, authorizationServerPolicyRule.links);
// ;
}
@Override
public int hashCode() {
return Objects.hash(actions, conditions, created, id, lastUpdated, name, priority, status, system, type, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AuthorizationServerPolicyRule {\n");
sb.append(" actions: ").append(toIndentedString(actions)).append("\n");
sb.append(" conditions: ").append(toIndentedString(conditions)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" system: ").append(toIndentedString(system)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy