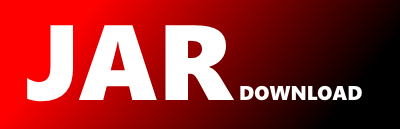
com.okta.sdk.resource.model.BrandWithEmbedded Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.DefaultApp;
import com.okta.sdk.resource.model.DomainResponse;
import com.okta.sdk.resource.model.EmailDomainResponse;
import com.okta.sdk.resource.model.LinksSelf;
import com.okta.sdk.resource.model.ThemeResponse;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* BrandWithEmbedded
*/
@JsonPropertyOrder({ BrandWithEmbedded.JSON_PROPERTY_EMBEDDED, BrandWithEmbedded.JSON_PROPERTY_LINKS,
BrandWithEmbedded.JSON_PROPERTY_AGREE_TO_CUSTOM_PRIVACY_POLICY,
BrandWithEmbedded.JSON_PROPERTY_CUSTOM_PRIVACY_POLICY_URL, BrandWithEmbedded.JSON_PROPERTY_DEFAULT_APP,
BrandWithEmbedded.JSON_PROPERTY_EMAIL_DOMAIN_ID, BrandWithEmbedded.JSON_PROPERTY_ID,
BrandWithEmbedded.JSON_PROPERTY_IS_DEFAULT, BrandWithEmbedded.JSON_PROPERTY_LOCALE,
BrandWithEmbedded.JSON_PROPERTY_NAME, BrandWithEmbedded.JSON_PROPERTY_REMOVE_POWERED_BY_OKTA })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class BrandWithEmbedded implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_EMBEDDED = "_embedded";
private Object embedded;
public static final String JSON_PROPERTY_LINKS = "_links";
private LinksSelf links;
public static final String JSON_PROPERTY_AGREE_TO_CUSTOM_PRIVACY_POLICY = "agreeToCustomPrivacyPolicy";
private Boolean agreeToCustomPrivacyPolicy;
public static final String JSON_PROPERTY_CUSTOM_PRIVACY_POLICY_URL = "customPrivacyPolicyUrl";
private String customPrivacyPolicyUrl;
public static final String JSON_PROPERTY_DEFAULT_APP = "defaultApp";
private DefaultApp defaultApp;
public static final String JSON_PROPERTY_EMAIL_DOMAIN_ID = "emailDomainId";
private String emailDomainId;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IS_DEFAULT = "isDefault";
private Boolean isDefault;
public static final String JSON_PROPERTY_LOCALE = "locale";
private String locale;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_REMOVE_POWERED_BY_OKTA = "removePoweredByOkta";
private Boolean removePoweredByOkta = false;
public BrandWithEmbedded() {
}
/*
* @JsonCreator public BrandWithEmbedded(
*
* @JsonProperty(JSON_PROPERTY_EMBEDDED) Object embedded,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_IS_DEFAULT) Boolean isDefault ) { this(); this.embedded = embedded; this.id = id;
* this.isDefault = isDefault; }
*/
/**
* Get embedded
*
* @return embedded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Object getEmbedded() {
return embedded;
}
public BrandWithEmbedded links(LinksSelf links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksSelf getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(LinksSelf links) {
this.links = links;
}
public BrandWithEmbedded agreeToCustomPrivacyPolicy(Boolean agreeToCustomPrivacyPolicy) {
this.agreeToCustomPrivacyPolicy = agreeToCustomPrivacyPolicy;
return this;
}
/**
* Consent for updating the custom privacy URL. Not required when resetting the URL.
*
* @return agreeToCustomPrivacyPolicy
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Consent for updating the custom privacy URL. Not required when resetting the URL.")
@JsonProperty(JSON_PROPERTY_AGREE_TO_CUSTOM_PRIVACY_POLICY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAgreeToCustomPrivacyPolicy() {
return agreeToCustomPrivacyPolicy;
}
@JsonProperty(JSON_PROPERTY_AGREE_TO_CUSTOM_PRIVACY_POLICY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAgreeToCustomPrivacyPolicy(Boolean agreeToCustomPrivacyPolicy) {
this.agreeToCustomPrivacyPolicy = agreeToCustomPrivacyPolicy;
}
public BrandWithEmbedded customPrivacyPolicyUrl(String customPrivacyPolicyUrl) {
this.customPrivacyPolicyUrl = customPrivacyPolicyUrl;
return this;
}
/**
* Custom privacy policy URL
*
* @return customPrivacyPolicyUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Custom privacy policy URL")
@JsonProperty(JSON_PROPERTY_CUSTOM_PRIVACY_POLICY_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCustomPrivacyPolicyUrl() {
return customPrivacyPolicyUrl;
}
@JsonProperty(JSON_PROPERTY_CUSTOM_PRIVACY_POLICY_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCustomPrivacyPolicyUrl(String customPrivacyPolicyUrl) {
this.customPrivacyPolicyUrl = customPrivacyPolicyUrl;
}
public BrandWithEmbedded defaultApp(DefaultApp defaultApp) {
this.defaultApp = defaultApp;
return this;
}
/**
* Get defaultApp
*
* @return defaultApp
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DEFAULT_APP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DefaultApp getDefaultApp() {
return defaultApp;
}
@JsonProperty(JSON_PROPERTY_DEFAULT_APP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDefaultApp(DefaultApp defaultApp) {
this.defaultApp = defaultApp;
}
public BrandWithEmbedded emailDomainId(String emailDomainId) {
this.emailDomainId = emailDomainId;
return this;
}
/**
* The ID of the email domain
*
* @return emailDomainId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The ID of the email domain")
@JsonProperty(JSON_PROPERTY_EMAIL_DOMAIN_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEmailDomainId() {
return emailDomainId;
}
@JsonProperty(JSON_PROPERTY_EMAIL_DOMAIN_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEmailDomainId(String emailDomainId) {
this.emailDomainId = emailDomainId;
}
/**
* The Brand ID
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Brand ID")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
/**
* If `true`, the Brand is used for the Okta subdomain
*
* @return isDefault
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If `true`, the Brand is used for the Okta subdomain")
@JsonProperty(JSON_PROPERTY_IS_DEFAULT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsDefault() {
return isDefault;
}
public BrandWithEmbedded locale(String locale) {
this.locale = locale;
return this;
}
/**
* The language specified as an [IETF BCP 47 language tag](https://datatracker.ietf.org/doc/html/rfc5646)
*
* @return locale
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The language specified as an [IETF BCP 47 language tag](https://datatracker.ietf.org/doc/html/rfc5646)")
@JsonProperty(JSON_PROPERTY_LOCALE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLocale() {
return locale;
}
@JsonProperty(JSON_PROPERTY_LOCALE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLocale(String locale) {
this.locale = locale;
}
public BrandWithEmbedded name(String name) {
this.name = name;
return this;
}
/**
* The name of the Brand
*
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the Brand")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public BrandWithEmbedded removePoweredByOkta(Boolean removePoweredByOkta) {
this.removePoweredByOkta = removePoweredByOkta;
return this;
}
/**
* Removes \"Powered by Okta\" from the sign-in page in redirect authentication deployments, and \"©
* [current year] Okta, Inc.\" from the Okta End-User Dashboard
*
* @return removePoweredByOkta
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Removes \"Powered by Okta\" from the sign-in page in redirect authentication deployments, and \"© [current year] Okta, Inc.\" from the Okta End-User Dashboard")
@JsonProperty(JSON_PROPERTY_REMOVE_POWERED_BY_OKTA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getRemovePoweredByOkta() {
return removePoweredByOkta;
}
@JsonProperty(JSON_PROPERTY_REMOVE_POWERED_BY_OKTA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRemovePoweredByOkta(Boolean removePoweredByOkta) {
this.removePoweredByOkta = removePoweredByOkta;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BrandWithEmbedded brandWithEmbedded = (BrandWithEmbedded) o;
return Objects.equals(this.embedded, brandWithEmbedded.embedded)
&& Objects.equals(this.links, brandWithEmbedded.links)
&& Objects.equals(this.agreeToCustomPrivacyPolicy, brandWithEmbedded.agreeToCustomPrivacyPolicy)
&& Objects.equals(this.customPrivacyPolicyUrl, brandWithEmbedded.customPrivacyPolicyUrl)
&& Objects.equals(this.defaultApp, brandWithEmbedded.defaultApp)
&& Objects.equals(this.emailDomainId, brandWithEmbedded.emailDomainId)
&& Objects.equals(this.id, brandWithEmbedded.id)
&& Objects.equals(this.isDefault, brandWithEmbedded.isDefault)
&& Objects.equals(this.locale, brandWithEmbedded.locale)
&& Objects.equals(this.name, brandWithEmbedded.name)
&& Objects.equals(this.removePoweredByOkta, brandWithEmbedded.removePoweredByOkta);
// ;
}
@Override
public int hashCode() {
return Objects.hash(embedded, links, agreeToCustomPrivacyPolicy, customPrivacyPolicyUrl, defaultApp,
emailDomainId, id, isDefault, locale, name, removePoweredByOkta);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BrandWithEmbedded {\n");
sb.append(" embedded: ").append(toIndentedString(embedded)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" agreeToCustomPrivacyPolicy: ").append(toIndentedString(agreeToCustomPrivacyPolicy)).append("\n");
sb.append(" customPrivacyPolicyUrl: ").append(toIndentedString(customPrivacyPolicyUrl)).append("\n");
sb.append(" defaultApp: ").append(toIndentedString(defaultApp)).append("\n");
sb.append(" emailDomainId: ").append(toIndentedString(emailDomainId)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isDefault: ").append(toIndentedString(isDefault)).append("\n");
sb.append(" locale: ").append(toIndentedString(locale)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" removePoweredByOkta: ").append(toIndentedString(removePoweredByOkta)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy