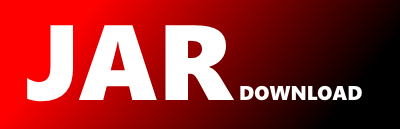
com.okta.sdk.resource.model.ChildOrg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.OrgCreationAdmin;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* ChildOrg
*/
@JsonPropertyOrder({ ChildOrg.JSON_PROPERTY_ADMIN, ChildOrg.JSON_PROPERTY_CREATED, ChildOrg.JSON_PROPERTY_EDITION,
ChildOrg.JSON_PROPERTY_ID, ChildOrg.JSON_PROPERTY_LAST_UPDATED, ChildOrg.JSON_PROPERTY_NAME,
ChildOrg.JSON_PROPERTY_SETTINGS, ChildOrg.JSON_PROPERTY_STATUS, ChildOrg.JSON_PROPERTY_SUBDOMAIN,
ChildOrg.JSON_PROPERTY_TOKEN, ChildOrg.JSON_PROPERTY_TOKEN_TYPE, ChildOrg.JSON_PROPERTY_WEBSITE,
ChildOrg.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ChildOrg implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ADMIN = "admin";
private OrgCreationAdmin admin;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
/**
* Edition for the Org. `SKU` is the only supported value.
*/
public enum EditionEnum {
SKU("SKU"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
EditionEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static EditionEnum fromValue(String value) {
for (EditionEnum b : EditionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_EDITION = "edition";
private EditionEnum edition;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_SETTINGS = "settings";
private Map settings = null;
/**
* Status of the Org. `ACTIVE` is returned after the Org is created.
*/
public enum StatusEnum {
ACTIVE("ACTIVE"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_STATUS = "status";
private StatusEnum status;
public static final String JSON_PROPERTY_SUBDOMAIN = "subdomain";
private String subdomain;
public static final String JSON_PROPERTY_TOKEN = "token";
private String token;
/**
* Type of returned `token`. See [Okta API
* tokens](https://developer.okta.com/docs/guides/create-an-api-token/main/#okta-api-tokens).
*/
public enum TokenTypeEnum {
SSWS("SSWS"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TokenTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TokenTypeEnum fromValue(String value) {
for (TokenTypeEnum b : TokenTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_TOKEN_TYPE = "tokenType";
private TokenTypeEnum tokenType;
public static final String JSON_PROPERTY_WEBSITE = "website";
private String website;
public static final String JSON_PROPERTY_LINKS = "_links";
private Map links = null;
public ChildOrg() {
}
/*
* @JsonCreator public ChildOrg(
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated,
*
* @JsonProperty(JSON_PROPERTY_SETTINGS) Map settings,
*
* @JsonProperty(JSON_PROPERTY_STATUS) StatusEnum status,
*
* @JsonProperty(JSON_PROPERTY_TOKEN) String token,
*
* @JsonProperty(JSON_PROPERTY_TOKEN_TYPE) TokenTypeEnum tokenType,
*
* @JsonProperty(JSON_PROPERTY_LINKS) Map links ) { this(); this.created = created; this.id = id;
* this.lastUpdated = lastUpdated; this.settings = settings; this.status = status; this.token = token;
* this.tokenType = tokenType; this.links = links; }
*/
public ChildOrg admin(OrgCreationAdmin admin) {
this.admin = admin;
return this;
}
/**
* Get admin
*
* @return admin
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_ADMIN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OrgCreationAdmin getAdmin() {
return admin;
}
@JsonProperty(JSON_PROPERTY_ADMIN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAdmin(OrgCreationAdmin admin) {
this.admin = admin;
}
/**
* Timestamp when the Org was created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2022-08-25T00:05Z", value = "Timestamp when the Org was created")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
public ChildOrg edition(EditionEnum edition) {
this.edition = edition;
return this;
}
/**
* Edition for the Org. `SKU` is the only supported value.
*
* @return edition
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "SKU", required = true, value = "Edition for the Org. `SKU` is the only supported value.")
@JsonProperty(JSON_PROPERTY_EDITION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public EditionEnum getEdition() {
return edition;
}
@JsonProperty(JSON_PROPERTY_EDITION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEdition(EditionEnum edition) {
this.edition = edition;
}
/**
* Org ID
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "00o1n8sbwArJ7OQRw406", value = "Org ID")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
/**
* Timestamp when the Org was last updated
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2022-08-25T00:05Z", value = "Timestamp when the Org was last updated")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
public ChildOrg name(String name) {
this.name = name;
return this;
}
/**
* Unique name of the Org. This name appears in the HTML `<title>` tag of the new Org sign-in page.
* Only less than 4-width UTF-8 encoded characters are allowed.
*
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "My Child Org 1", required = true, value = "Unique name of the Org. This name appears in the HTML `` tag of the new Org sign-in page. Only less than 4-width UTF-8 encoded characters are allowed.")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
/**
* Settings associated with the created Org
*
* @return settings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Settings associated with the created Org")
@JsonProperty(JSON_PROPERTY_SETTINGS)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getSettings() {
return settings;
}
/**
* Status of the Org. `ACTIVE` is returned after the Org is created.
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Status of the Org. `ACTIVE` is returned after the Org is created.")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public StatusEnum getStatus() {
return status;
}
public ChildOrg subdomain(String subdomain) {
this.subdomain = subdomain;
return this;
}
/**
* Subdomain of the Org. Must be unique and include no spaces.
*
* @return subdomain
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "my-child-org-1", required = true, value = "Subdomain of the Org. Must be unique and include no spaces.")
@JsonProperty(JSON_PROPERTY_SUBDOMAIN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSubdomain() {
return subdomain;
}
@JsonProperty(JSON_PROPERTY_SUBDOMAIN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSubdomain(String subdomain) {
this.subdomain = subdomain;
}
/**
* API token associated with the child Org super admin account. Use this API token to provision resources (such as
* policies, apps, and groups) on the newly created child Org. This token is revoked if the super admin account is
* deactivated. > **Note:** If this API token expires, sign in to the Admin Console as the super admin user and
* create a new API token. See [Create an API token](https://developer.okta.com/docs/guides/create-an-api-token/).
*
* @return token
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "API token associated with the child Org super admin account. Use this API token to provision resources (such as policies, apps, and groups) on the newly created child Org. This token is revoked if the super admin account is deactivated. > **Note:** If this API token expires, sign in to the Admin Console as the super admin user and create a new API token. See [Create an API token](https://developer.okta.com/docs/guides/create-an-api-token/).")
@JsonProperty(JSON_PROPERTY_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getToken() {
return token;
}
/**
* Type of returned `token`. See [Okta API
* tokens](https://developer.okta.com/docs/guides/create-an-api-token/main/#okta-api-tokens).
*
* @return tokenType
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "SSWS", value = "Type of returned `token`. See [Okta API tokens](https://developer.okta.com/docs/guides/create-an-api-token/main/#okta-api-tokens).")
@JsonProperty(JSON_PROPERTY_TOKEN_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TokenTypeEnum getTokenType() {
return tokenType;
}
public ChildOrg website(String website) {
this.website = website;
return this;
}
/**
* Default website for the Org
*
* @return website
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "https://www.okta.com", value = "Default website for the Org")
@JsonProperty(JSON_PROPERTY_WEBSITE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getWebsite() {
return website;
}
@JsonProperty(JSON_PROPERTY_WEBSITE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWebsite(String website) {
this.website = website;
}
/**
* Specifies available link relations (see [Web Linking](https://www.rfc-editor.org/rfc/rfc8288)) using the [JSON
* Hypertext Application Language](https://datatracker.ietf.org/doc/html/draft-kelly-json-hal-06) specification
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies available link relations (see [Web Linking](https://www.rfc-editor.org/rfc/rfc8288)) using the [JSON Hypertext Application Language](https://datatracker.ietf.org/doc/html/draft-kelly-json-hal-06) specification")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getLinks() {
return links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ChildOrg childOrg = (ChildOrg) o;
return Objects.equals(this.admin, childOrg.admin) && Objects.equals(this.created, childOrg.created)
&& Objects.equals(this.edition, childOrg.edition) && Objects.equals(this.id, childOrg.id)
&& Objects.equals(this.lastUpdated, childOrg.lastUpdated) && Objects.equals(this.name, childOrg.name)
&& Objects.equals(this.settings, childOrg.settings) && Objects.equals(this.status, childOrg.status)
&& Objects.equals(this.subdomain, childOrg.subdomain) && Objects.equals(this.token, childOrg.token)
&& Objects.equals(this.tokenType, childOrg.tokenType) && Objects.equals(this.website, childOrg.website)
&& Objects.equals(this.links, childOrg.links);
// ;
}
@Override
public int hashCode() {
return Objects.hash(admin, created, edition, id, lastUpdated, name, settings, status, subdomain, token,
tokenType, website, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ChildOrg {\n");
sb.append(" admin: ").append(toIndentedString(admin)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" edition: ").append(toIndentedString(edition)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" settings: ").append(toIndentedString(settings)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" subdomain: ").append(toIndentedString(subdomain)).append("\n");
sb.append(" token: ").append(toIndentedString(token)).append("\n");
sb.append(" tokenType: ").append(toIndentedString(tokenType)).append("\n");
sb.append(" website: ").append(toIndentedString(website)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy