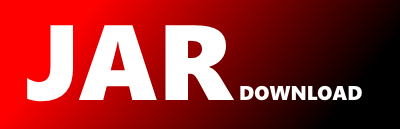
com.okta.sdk.resource.model.DeviceAssuranceWindowsPlatform Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.DeviceAssurance;
import com.okta.sdk.resource.model.DeviceAssuranceAndroidPlatformAllOfScreenLockType;
import com.okta.sdk.resource.model.DeviceAssuranceMacOSPlatformAllOfDiskEncryptionType;
import com.okta.sdk.resource.model.DeviceAssuranceWindowsPlatformAllOfThirdPartySignalProviders;
import com.okta.sdk.resource.model.LinksSelf;
import com.okta.sdk.resource.model.OSVersionConstraint;
import com.okta.sdk.resource.model.OSVersionFourComponents;
import com.okta.sdk.resource.model.Platform;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* DeviceAssuranceWindowsPlatform
*/
@JsonPropertyOrder({ DeviceAssuranceWindowsPlatform.JSON_PROPERTY_DISK_ENCRYPTION_TYPE,
DeviceAssuranceWindowsPlatform.JSON_PROPERTY_OS_VERSION,
DeviceAssuranceWindowsPlatform.JSON_PROPERTY_OS_VERSION_CONSTRAINTS,
DeviceAssuranceWindowsPlatform.JSON_PROPERTY_SCREEN_LOCK_TYPE,
DeviceAssuranceWindowsPlatform.JSON_PROPERTY_SECURE_HARDWARE_PRESENT,
DeviceAssuranceWindowsPlatform.JSON_PROPERTY_THIRD_PARTY_SIGNAL_PROVIDERS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.EXISTING_PROPERTY, property = "platform", visible = true, defaultImpl = DeviceAssuranceWindowsPlatform.class)
public class DeviceAssuranceWindowsPlatform extends DeviceAssurance implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_DISK_ENCRYPTION_TYPE = "diskEncryptionType";
private DeviceAssuranceMacOSPlatformAllOfDiskEncryptionType diskEncryptionType;
public static final String JSON_PROPERTY_OS_VERSION = "osVersion";
private OSVersionFourComponents osVersion;
public static final String JSON_PROPERTY_OS_VERSION_CONSTRAINTS = "osVersionConstraints";
private List osVersionConstraints = null;
public static final String JSON_PROPERTY_SCREEN_LOCK_TYPE = "screenLockType";
private DeviceAssuranceAndroidPlatformAllOfScreenLockType screenLockType;
public static final String JSON_PROPERTY_SECURE_HARDWARE_PRESENT = "secureHardwarePresent";
private Boolean secureHardwarePresent;
public static final String JSON_PROPERTY_THIRD_PARTY_SIGNAL_PROVIDERS = "thirdPartySignalProviders";
private DeviceAssuranceWindowsPlatformAllOfThirdPartySignalProviders thirdPartySignalProviders;
public DeviceAssuranceWindowsPlatform() {
}
/*
* @JsonCreator public DeviceAssuranceWindowsPlatform(
*
* @JsonProperty(JSON_PROPERTY_CREATED_BY) String createdBy,
*
* @JsonProperty(JSON_PROPERTY_CREATED_DATE) String createdDate,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATE) String lastUpdate,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED_BY) String lastUpdatedBy ) { this(); this.createdBy = createdBy;
* this.createdDate = createdDate; this.id = id; this.lastUpdate = lastUpdate; this.lastUpdatedBy = lastUpdatedBy; }
*/
public DeviceAssuranceWindowsPlatform diskEncryptionType(
DeviceAssuranceMacOSPlatformAllOfDiskEncryptionType diskEncryptionType) {
this.diskEncryptionType = diskEncryptionType;
return this;
}
/**
* Get diskEncryptionType
*
* @return diskEncryptionType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DISK_ENCRYPTION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DeviceAssuranceMacOSPlatformAllOfDiskEncryptionType getDiskEncryptionType() {
return diskEncryptionType;
}
@JsonProperty(JSON_PROPERTY_DISK_ENCRYPTION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDiskEncryptionType(DeviceAssuranceMacOSPlatformAllOfDiskEncryptionType diskEncryptionType) {
this.diskEncryptionType = diskEncryptionType;
}
public DeviceAssuranceWindowsPlatform osVersion(OSVersionFourComponents osVersion) {
this.osVersion = osVersion;
return this;
}
/**
* Get osVersion
*
* @return osVersion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_OS_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OSVersionFourComponents getOsVersion() {
return osVersion;
}
@JsonProperty(JSON_PROPERTY_OS_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOsVersion(OSVersionFourComponents osVersion) {
this.osVersion = osVersion;
}
public DeviceAssuranceWindowsPlatform osVersionConstraints(List osVersionConstraints) {
this.osVersionConstraints = osVersionConstraints;
return this;
}
public DeviceAssuranceWindowsPlatform addosVersionConstraintsItem(OSVersionConstraint osVersionConstraintsItem) {
if (this.osVersionConstraints == null) {
this.osVersionConstraints = new ArrayList<>();
}
this.osVersionConstraints.add(osVersionConstraintsItem);
return this;
}
/**
* <div class=\"x-lifecycle-container\"><x-lifecycle
* class=\"ea\"></x-lifecycle></div>Specifies the Windows version requirements for the
* assurance policy. Each requirement must correspond to a different major version (Windows 11 or Windows 10). If a
* requirement isn't specified for a major version, then devices on that major version satisfy the condition.
* There are two types of OS requirements: * **Static**: A specific Windows version requirement that doesn't
* change until you update the policy. A static OS Windows requirement is specified with
* `majorVersionConstraint` and `minimum`. * **Dynamic**: A Windows version requirement that is
* relative to the latest major release and security patch. A dynamic OS Windows requirement is specified with
* `majorVersionConstraint` and `dynamicVersionRequirement`. > **Note:** Dynamic OS
* requirements are available only if the **Dynamic OS version compliance** [self-service
* EA](/openapi/okta-management/guides/release-lifecycle/#early-access-ea) feature is enabled. The
* `osVersionConstraints` property is only supported for the Windows platform. You can't specify both
* `osVersion.minimum` and `osVersionConstraints` properties at the same time.
*
* @return osVersionConstraints
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " Specifies the Windows version requirements for the assurance policy. Each requirement must correspond to a different major version (Windows 11 or Windows 10). If a requirement isn't specified for a major version, then devices on that major version satisfy the condition. There are two types of OS requirements: * **Static**: A specific Windows version requirement that doesn't change until you update the policy. A static OS Windows requirement is specified with `majorVersionConstraint` and `minimum`. * **Dynamic**: A Windows version requirement that is relative to the latest major release and security patch. A dynamic OS Windows requirement is specified with `majorVersionConstraint` and `dynamicVersionRequirement`. > **Note:** Dynamic OS requirements are available only if the **Dynamic OS version compliance** [self-service EA](/openapi/okta-management/guides/release-lifecycle/#early-access-ea) feature is enabled. The `osVersionConstraints` property is only supported for the Windows platform. You can't specify both `osVersion.minimum` and `osVersionConstraints` properties at the same time. ")
@JsonProperty(JSON_PROPERTY_OS_VERSION_CONSTRAINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getOsVersionConstraints() {
return osVersionConstraints;
}
@JsonProperty(JSON_PROPERTY_OS_VERSION_CONSTRAINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOsVersionConstraints(List osVersionConstraints) {
this.osVersionConstraints = osVersionConstraints;
}
public DeviceAssuranceWindowsPlatform screenLockType(
DeviceAssuranceAndroidPlatformAllOfScreenLockType screenLockType) {
this.screenLockType = screenLockType;
return this;
}
/**
* Get screenLockType
*
* @return screenLockType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SCREEN_LOCK_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DeviceAssuranceAndroidPlatformAllOfScreenLockType getScreenLockType() {
return screenLockType;
}
@JsonProperty(JSON_PROPERTY_SCREEN_LOCK_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setScreenLockType(DeviceAssuranceAndroidPlatformAllOfScreenLockType screenLockType) {
this.screenLockType = screenLockType;
}
public DeviceAssuranceWindowsPlatform secureHardwarePresent(Boolean secureHardwarePresent) {
this.secureHardwarePresent = secureHardwarePresent;
return this;
}
/**
* Get secureHardwarePresent
*
* @return secureHardwarePresent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SECURE_HARDWARE_PRESENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getSecureHardwarePresent() {
return secureHardwarePresent;
}
@JsonProperty(JSON_PROPERTY_SECURE_HARDWARE_PRESENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSecureHardwarePresent(Boolean secureHardwarePresent) {
this.secureHardwarePresent = secureHardwarePresent;
}
public DeviceAssuranceWindowsPlatform thirdPartySignalProviders(
DeviceAssuranceWindowsPlatformAllOfThirdPartySignalProviders thirdPartySignalProviders) {
this.thirdPartySignalProviders = thirdPartySignalProviders;
return this;
}
/**
* Get thirdPartySignalProviders
*
* @return thirdPartySignalProviders
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_THIRD_PARTY_SIGNAL_PROVIDERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DeviceAssuranceWindowsPlatformAllOfThirdPartySignalProviders getThirdPartySignalProviders() {
return thirdPartySignalProviders;
}
@JsonProperty(JSON_PROPERTY_THIRD_PARTY_SIGNAL_PROVIDERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setThirdPartySignalProviders(
DeviceAssuranceWindowsPlatformAllOfThirdPartySignalProviders thirdPartySignalProviders) {
this.thirdPartySignalProviders = thirdPartySignalProviders;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DeviceAssuranceWindowsPlatform deviceAssuranceWindowsPlatform = (DeviceAssuranceWindowsPlatform) o;
return Objects.equals(this.diskEncryptionType, deviceAssuranceWindowsPlatform.diskEncryptionType)
&& Objects.equals(this.osVersion, deviceAssuranceWindowsPlatform.osVersion)
&& Objects.equals(this.osVersionConstraints, deviceAssuranceWindowsPlatform.osVersionConstraints)
&& Objects.equals(this.screenLockType, deviceAssuranceWindowsPlatform.screenLockType)
&& Objects.equals(this.secureHardwarePresent, deviceAssuranceWindowsPlatform.secureHardwarePresent)
&& Objects.equals(this.thirdPartySignalProviders,
deviceAssuranceWindowsPlatform.thirdPartySignalProviders);
// && super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(diskEncryptionType, osVersion, osVersionConstraints, screenLockType, secureHardwarePresent,
thirdPartySignalProviders, super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DeviceAssuranceWindowsPlatform {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" diskEncryptionType: ").append(toIndentedString(diskEncryptionType)).append("\n");
sb.append(" osVersion: ").append(toIndentedString(osVersion)).append("\n");
sb.append(" osVersionConstraints: ").append(toIndentedString(osVersionConstraints)).append("\n");
sb.append(" screenLockType: ").append(toIndentedString(screenLockType)).append("\n");
sb.append(" secureHardwarePresent: ").append(toIndentedString(secureHardwarePresent)).append("\n");
sb.append(" thirdPartySignalProviders: ").append(toIndentedString(thirdPartySignalProviders)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy