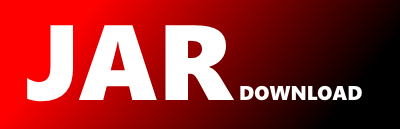
com.okta.sdk.resource.model.DeviceProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.DevicePlatform;
import com.okta.sdk.resource.model.DiskEncryptionTypeDef;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* DeviceProfile
*/
@JsonPropertyOrder({ DeviceProfile.JSON_PROPERTY_DISK_ENCRYPTION_TYPE, DeviceProfile.JSON_PROPERTY_DISPLAY_NAME,
DeviceProfile.JSON_PROPERTY_IMEI, DeviceProfile.JSON_PROPERTY_INTEGRITY_JAILBREAK,
DeviceProfile.JSON_PROPERTY_MANUFACTURER, DeviceProfile.JSON_PROPERTY_MEID, DeviceProfile.JSON_PROPERTY_MODEL,
DeviceProfile.JSON_PROPERTY_OS_VERSION, DeviceProfile.JSON_PROPERTY_PLATFORM,
DeviceProfile.JSON_PROPERTY_REGISTERED, DeviceProfile.JSON_PROPERTY_SECURE_HARDWARE_PRESENT,
DeviceProfile.JSON_PROPERTY_SERIAL_NUMBER, DeviceProfile.JSON_PROPERTY_SID,
DeviceProfile.JSON_PROPERTY_TPM_PUBLIC_KEY_HASH, DeviceProfile.JSON_PROPERTY_UDID })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class DeviceProfile implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_DISK_ENCRYPTION_TYPE = "diskEncryptionType";
private DiskEncryptionTypeDef diskEncryptionType;
public static final String JSON_PROPERTY_DISPLAY_NAME = "displayName";
private String displayName;
public static final String JSON_PROPERTY_IMEI = "imei";
private String imei;
public static final String JSON_PROPERTY_INTEGRITY_JAILBREAK = "integrityJailbreak";
private Boolean integrityJailbreak;
public static final String JSON_PROPERTY_MANUFACTURER = "manufacturer";
private String manufacturer;
public static final String JSON_PROPERTY_MEID = "meid";
private String meid;
public static final String JSON_PROPERTY_MODEL = "model";
private String model;
public static final String JSON_PROPERTY_OS_VERSION = "osVersion";
private String osVersion;
public static final String JSON_PROPERTY_PLATFORM = "platform";
private DevicePlatform platform;
public static final String JSON_PROPERTY_REGISTERED = "registered";
private Boolean registered;
public static final String JSON_PROPERTY_SECURE_HARDWARE_PRESENT = "secureHardwarePresent";
private Boolean secureHardwarePresent;
public static final String JSON_PROPERTY_SERIAL_NUMBER = "serialNumber";
private String serialNumber;
public static final String JSON_PROPERTY_SID = "sid";
private String sid;
public static final String JSON_PROPERTY_TPM_PUBLIC_KEY_HASH = "tpmPublicKeyHash";
private String tpmPublicKeyHash;
public static final String JSON_PROPERTY_UDID = "udid";
private String udid;
public DeviceProfile() {
}
public DeviceProfile diskEncryptionType(DiskEncryptionTypeDef diskEncryptionType) {
this.diskEncryptionType = diskEncryptionType;
return this;
}
/**
* Get diskEncryptionType
*
* @return diskEncryptionType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DISK_ENCRYPTION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DiskEncryptionTypeDef getDiskEncryptionType() {
return diskEncryptionType;
}
@JsonProperty(JSON_PROPERTY_DISK_ENCRYPTION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDiskEncryptionType(DiskEncryptionTypeDef diskEncryptionType) {
this.diskEncryptionType = diskEncryptionType;
}
public DeviceProfile displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Display name of the device
*
* @return displayName
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Display name of the device")
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDisplayName() {
return displayName;
}
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public DeviceProfile imei(String imei) {
this.imei = imei;
return this;
}
/**
* International Mobile Equipment Identity (IMEI) of the device
*
* @return imei
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "International Mobile Equipment Identity (IMEI) of the device")
@JsonProperty(JSON_PROPERTY_IMEI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getImei() {
return imei;
}
@JsonProperty(JSON_PROPERTY_IMEI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setImei(String imei) {
this.imei = imei;
}
public DeviceProfile integrityJailbreak(Boolean integrityJailbreak) {
this.integrityJailbreak = integrityJailbreak;
return this;
}
/**
* Indicates if the device is jailbroken or rooted. Only applicable to `IOS` and `ANDROID`
* platforms
*
* @return integrityJailbreak
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if the device is jailbroken or rooted. Only applicable to `IOS` and `ANDROID` platforms")
@JsonProperty(JSON_PROPERTY_INTEGRITY_JAILBREAK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIntegrityJailbreak() {
return integrityJailbreak;
}
@JsonProperty(JSON_PROPERTY_INTEGRITY_JAILBREAK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIntegrityJailbreak(Boolean integrityJailbreak) {
this.integrityJailbreak = integrityJailbreak;
}
public DeviceProfile manufacturer(String manufacturer) {
this.manufacturer = manufacturer;
return this;
}
/**
* Name of the manufacturer of the device
*
* @return manufacturer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the manufacturer of the device")
@JsonProperty(JSON_PROPERTY_MANUFACTURER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getManufacturer() {
return manufacturer;
}
@JsonProperty(JSON_PROPERTY_MANUFACTURER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setManufacturer(String manufacturer) {
this.manufacturer = manufacturer;
}
public DeviceProfile meid(String meid) {
this.meid = meid;
return this;
}
/**
* Mobile equipment identifier of the device
*
* @return meid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Mobile equipment identifier of the device")
@JsonProperty(JSON_PROPERTY_MEID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMeid() {
return meid;
}
@JsonProperty(JSON_PROPERTY_MEID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMeid(String meid) {
this.meid = meid;
}
public DeviceProfile model(String model) {
this.model = model;
return this;
}
/**
* Model of the device
*
* @return model
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Model of the device")
@JsonProperty(JSON_PROPERTY_MODEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getModel() {
return model;
}
@JsonProperty(JSON_PROPERTY_MODEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setModel(String model) {
this.model = model;
}
public DeviceProfile osVersion(String osVersion) {
this.osVersion = osVersion;
return this;
}
/**
* Version of the device OS
*
* @return osVersion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Version of the device OS")
@JsonProperty(JSON_PROPERTY_OS_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOsVersion() {
return osVersion;
}
@JsonProperty(JSON_PROPERTY_OS_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOsVersion(String osVersion) {
this.osVersion = osVersion;
}
public DeviceProfile platform(DevicePlatform platform) {
this.platform = platform;
return this;
}
/**
* Get platform
*
* @return platform
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_PLATFORM)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DevicePlatform getPlatform() {
return platform;
}
@JsonProperty(JSON_PROPERTY_PLATFORM)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPlatform(DevicePlatform platform) {
this.platform = platform;
}
public DeviceProfile registered(Boolean registered) {
this.registered = registered;
return this;
}
/**
* Indicates if the device is registered at Okta
*
* @return registered
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Indicates if the device is registered at Okta")
@JsonProperty(JSON_PROPERTY_REGISTERED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getRegistered() {
return registered;
}
@JsonProperty(JSON_PROPERTY_REGISTERED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRegistered(Boolean registered) {
this.registered = registered;
}
public DeviceProfile secureHardwarePresent(Boolean secureHardwarePresent) {
this.secureHardwarePresent = secureHardwarePresent;
return this;
}
/**
* Indicates if the device contains a secure hardware functionality
*
* @return secureHardwarePresent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if the device contains a secure hardware functionality")
@JsonProperty(JSON_PROPERTY_SECURE_HARDWARE_PRESENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getSecureHardwarePresent() {
return secureHardwarePresent;
}
@JsonProperty(JSON_PROPERTY_SECURE_HARDWARE_PRESENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSecureHardwarePresent(Boolean secureHardwarePresent) {
this.secureHardwarePresent = secureHardwarePresent;
}
public DeviceProfile serialNumber(String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
/**
* Serial number of the device
*
* @return serialNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Serial number of the device")
@JsonProperty(JSON_PROPERTY_SERIAL_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSerialNumber() {
return serialNumber;
}
@JsonProperty(JSON_PROPERTY_SERIAL_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSerialNumber(String serialNumber) {
this.serialNumber = serialNumber;
}
public DeviceProfile sid(String sid) {
this.sid = sid;
return this;
}
/**
* Windows Security identifier of the device
*
* @return sid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Windows Security identifier of the device")
@JsonProperty(JSON_PROPERTY_SID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSid() {
return sid;
}
@JsonProperty(JSON_PROPERTY_SID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSid(String sid) {
this.sid = sid;
}
public DeviceProfile tpmPublicKeyHash(String tpmPublicKeyHash) {
this.tpmPublicKeyHash = tpmPublicKeyHash;
return this;
}
/**
* Windows Trusted Platform Module hash value
*
* @return tpmPublicKeyHash
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Windows Trusted Platform Module hash value")
@JsonProperty(JSON_PROPERTY_TPM_PUBLIC_KEY_HASH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTpmPublicKeyHash() {
return tpmPublicKeyHash;
}
@JsonProperty(JSON_PROPERTY_TPM_PUBLIC_KEY_HASH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTpmPublicKeyHash(String tpmPublicKeyHash) {
this.tpmPublicKeyHash = tpmPublicKeyHash;
}
public DeviceProfile udid(String udid) {
this.udid = udid;
return this;
}
/**
* macOS Unique Device identifier of the device
*
* @return udid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "macOS Unique Device identifier of the device")
@JsonProperty(JSON_PROPERTY_UDID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUdid() {
return udid;
}
@JsonProperty(JSON_PROPERTY_UDID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUdid(String udid) {
this.udid = udid;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DeviceProfile deviceProfile = (DeviceProfile) o;
return Objects.equals(this.diskEncryptionType, deviceProfile.diskEncryptionType)
&& Objects.equals(this.displayName, deviceProfile.displayName)
&& Objects.equals(this.imei, deviceProfile.imei)
&& Objects.equals(this.integrityJailbreak, deviceProfile.integrityJailbreak)
&& Objects.equals(this.manufacturer, deviceProfile.manufacturer)
&& Objects.equals(this.meid, deviceProfile.meid) && Objects.equals(this.model, deviceProfile.model)
&& Objects.equals(this.osVersion, deviceProfile.osVersion)
&& Objects.equals(this.platform, deviceProfile.platform)
&& Objects.equals(this.registered, deviceProfile.registered)
&& Objects.equals(this.secureHardwarePresent, deviceProfile.secureHardwarePresent)
&& Objects.equals(this.serialNumber, deviceProfile.serialNumber)
&& Objects.equals(this.sid, deviceProfile.sid)
&& Objects.equals(this.tpmPublicKeyHash, deviceProfile.tpmPublicKeyHash)
&& Objects.equals(this.udid, deviceProfile.udid);
// ;
}
@Override
public int hashCode() {
return Objects.hash(diskEncryptionType, displayName, imei, integrityJailbreak, manufacturer, meid, model,
osVersion, platform, registered, secureHardwarePresent, serialNumber, sid, tpmPublicKeyHash, udid);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DeviceProfile {\n");
sb.append(" diskEncryptionType: ").append(toIndentedString(diskEncryptionType)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" imei: ").append(toIndentedString(imei)).append("\n");
sb.append(" integrityJailbreak: ").append(toIndentedString(integrityJailbreak)).append("\n");
sb.append(" manufacturer: ").append(toIndentedString(manufacturer)).append("\n");
sb.append(" meid: ").append(toIndentedString(meid)).append("\n");
sb.append(" model: ").append(toIndentedString(model)).append("\n");
sb.append(" osVersion: ").append(toIndentedString(osVersion)).append("\n");
sb.append(" platform: ").append(toIndentedString(platform)).append("\n");
sb.append(" registered: ").append(toIndentedString(registered)).append("\n");
sb.append(" secureHardwarePresent: ").append(toIndentedString(secureHardwarePresent)).append("\n");
sb.append(" serialNumber: ").append(toIndentedString(serialNumber)).append("\n");
sb.append(" sid: ").append(toIndentedString(sid)).append("\n");
sb.append(" tpmPublicKeyHash: ").append(toIndentedString(tpmPublicKeyHash)).append("\n");
sb.append(" udid: ").append(toIndentedString(udid)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy