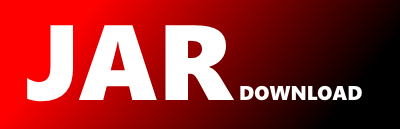
com.okta.sdk.resource.model.ECKeyJWK Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Elliptic Curve Key in JWK format, currently used during enrollment to encrypt fulfillment requests to Yubico, or
* during activation to verify Yubico's JWS objects in fulfillment responses. The currently agreed protocol uses
* P-384.
*/
@ApiModel(description = "Elliptic Curve Key in JWK format, currently used during enrollment to encrypt fulfillment requests to Yubico, or during activation to verify Yubico's JWS objects in fulfillment responses. The currently agreed protocol uses P-384.")
@JsonPropertyOrder({ ECKeyJWK.JSON_PROPERTY_CRV, ECKeyJWK.JSON_PROPERTY_KID, ECKeyJWK.JSON_PROPERTY_KTY,
ECKeyJWK.JSON_PROPERTY_USE, ECKeyJWK.JSON_PROPERTY_X, ECKeyJWK.JSON_PROPERTY_Y })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ECKeyJWK implements Serializable {
private static final long serialVersionUID = 1L;
/**
* Gets or Sets crv
*/
public enum CrvEnum {
P_384("P-384"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
CrvEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static CrvEnum fromValue(String value) {
for (CrvEnum b : CrvEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_CRV = "crv";
private CrvEnum crv;
public static final String JSON_PROPERTY_KID = "kid";
private String kid;
/**
* The type of public key
*/
public enum KtyEnum {
EC("EC"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
KtyEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static KtyEnum fromValue(String value) {
for (KtyEnum b : KtyEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_KTY = "kty";
private KtyEnum kty;
/**
* The intended use for the key. The ECKeyJWK is always `enc` because Okta uses it to encrypt requests to
* Yubico.
*/
public enum UseEnum {
ENC("enc"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
UseEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static UseEnum fromValue(String value) {
for (UseEnum b : UseEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_USE = "use";
private UseEnum use;
public static final String JSON_PROPERTY_X = "x";
private String x;
public static final String JSON_PROPERTY_Y = "y";
private String y;
public ECKeyJWK() {
}
public ECKeyJWK crv(CrvEnum crv) {
this.crv = crv;
return this;
}
/**
* Get crv
*
* @return crv
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_CRV)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public CrvEnum getCrv() {
return crv;
}
@JsonProperty(JSON_PROPERTY_CRV)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCrv(CrvEnum crv) {
this.crv = crv;
}
public ECKeyJWK kid(String kid) {
this.kid = kid;
return this;
}
/**
* The unique identifier of the key
*
* @return kid
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The unique identifier of the key")
@JsonProperty(JSON_PROPERTY_KID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getKid() {
return kid;
}
@JsonProperty(JSON_PROPERTY_KID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setKid(String kid) {
this.kid = kid;
}
public ECKeyJWK kty(KtyEnum kty) {
this.kty = kty;
return this;
}
/**
* The type of public key
*
* @return kty
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The type of public key")
@JsonProperty(JSON_PROPERTY_KTY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public KtyEnum getKty() {
return kty;
}
@JsonProperty(JSON_PROPERTY_KTY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setKty(KtyEnum kty) {
this.kty = kty;
}
public ECKeyJWK use(UseEnum use) {
this.use = use;
return this;
}
/**
* The intended use for the key. The ECKeyJWK is always `enc` because Okta uses it to encrypt requests to
* Yubico.
*
* @return use
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The intended use for the key. The ECKeyJWK is always `enc` because Okta uses it to encrypt requests to Yubico.")
@JsonProperty(JSON_PROPERTY_USE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UseEnum getUse() {
return use;
}
@JsonProperty(JSON_PROPERTY_USE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUse(UseEnum use) {
this.use = use;
}
public ECKeyJWK x(String x) {
this.x = x;
return this;
}
/**
* The public x coordinate for the elliptic curve point
*
* @return x
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The public x coordinate for the elliptic curve point")
@JsonProperty(JSON_PROPERTY_X)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getX() {
return x;
}
@JsonProperty(JSON_PROPERTY_X)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setX(String x) {
this.x = x;
}
public ECKeyJWK y(String y) {
this.y = y;
return this;
}
/**
* The public y coordinate for the elliptic curve point
*
* @return y
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The public y coordinate for the elliptic curve point")
@JsonProperty(JSON_PROPERTY_Y)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getY() {
return y;
}
@JsonProperty(JSON_PROPERTY_Y)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setY(String y) {
this.y = y;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ECKeyJWK ecKeyJWK = (ECKeyJWK) o;
return Objects.equals(this.crv, ecKeyJWK.crv) && Objects.equals(this.kid, ecKeyJWK.kid)
&& Objects.equals(this.kty, ecKeyJWK.kty) && Objects.equals(this.use, ecKeyJWK.use)
&& Objects.equals(this.x, ecKeyJWK.x) && Objects.equals(this.y, ecKeyJWK.y);
// ;
}
@Override
public int hashCode() {
return Objects.hash(crv, kid, kty, use, x, y);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ECKeyJWK {\n");
sb.append(" crv: ").append(toIndentedString(crv)).append("\n");
sb.append(" kid: ").append(toIndentedString(kid)).append("\n");
sb.append(" kty: ").append(toIndentedString(kty)).append("\n");
sb.append(" use: ").append(toIndentedString(use)).append("\n");
sb.append(" x: ").append(toIndentedString(x)).append("\n");
sb.append(" y: ").append(toIndentedString(y)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy