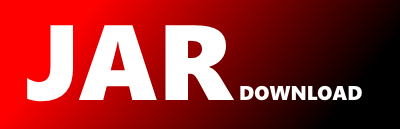
com.okta.sdk.resource.model.EmailCustomization Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.EmailCustomizationAllOfLinks;
import java.time.OffsetDateTime;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* EmailCustomization
*/
@JsonPropertyOrder({ EmailCustomization.JSON_PROPERTY_BODY, EmailCustomization.JSON_PROPERTY_SUBJECT,
EmailCustomization.JSON_PROPERTY_CREATED, EmailCustomization.JSON_PROPERTY_ID,
EmailCustomization.JSON_PROPERTY_IS_DEFAULT, EmailCustomization.JSON_PROPERTY_LANGUAGE,
EmailCustomization.JSON_PROPERTY_LAST_UPDATED, EmailCustomization.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class EmailCustomization implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_BODY = "body";
private String body;
public static final String JSON_PROPERTY_SUBJECT = "subject";
private String subject;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IS_DEFAULT = "isDefault";
private Boolean isDefault;
public static final String JSON_PROPERTY_LANGUAGE = "language";
private String language;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_LINKS = "_links";
private EmailCustomizationAllOfLinks links;
public EmailCustomization() {
}
/*
* @JsonCreator public EmailCustomization(
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated ) { this(); this.created = created; this.id
* = id; this.lastUpdated = lastUpdated; }
*/
public EmailCustomization body(String body) {
this.body = body;
return this;
}
/**
* The HTML body of the email. May contain [variable
* references](https://velocity.apache.org/engine/1.7/user-guide.html#references). <x-lifecycle
* class=\"ea\"></x-lifecycle> Not required if Custom languages for Okta Email Templates is
* enabled. A `null` body is replaced with a default value from one of the following in priority order: 1.
* An existing default email customization, if one exists 2. Okta-provided translated content for the specified
* language, if one exists 3. Okta-provided translated content for the brand locale, if it's set 4.
* Okta-provided content in English
*
* @return body
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The HTML body of the email. May contain [variable references](https://velocity.apache.org/engine/1.7/user-guide.html#references). Not required if Custom languages for Okta Email Templates is enabled. A `null` body is replaced with a default value from one of the following in priority order: 1. An existing default email customization, if one exists 2. Okta-provided translated content for the specified language, if one exists 3. Okta-provided translated content for the brand locale, if it's set 4. Okta-provided content in English ")
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getBody() {
return body;
}
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setBody(String body) {
this.body = body;
}
public EmailCustomization subject(String subject) {
this.subject = subject;
return this;
}
/**
* The email subject. May contain [variable
* references](https://velocity.apache.org/engine/1.7/user-guide.html#references). <x-lifecycle
* class=\"ea\"></x-lifecycle> Not required if Custom languages for Okta Email Templates is
* enabled. A `null` subject is replaced with a default value from one of the following in priority order:
* 1. An existing default email customization, if one exists 2. Okta-provided translated content for the specified
* language, if one exists 3. Okta-provided translated content for the brand locale, if it's set 4.
* Okta-provided content in English
*
* @return subject
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The email subject. May contain [variable references](https://velocity.apache.org/engine/1.7/user-guide.html#references). Not required if Custom languages for Okta Email Templates is enabled. A `null` subject is replaced with a default value from one of the following in priority order: 1. An existing default email customization, if one exists 2. Okta-provided translated content for the specified language, if one exists 3. Okta-provided translated content for the brand locale, if it's set 4. Okta-provided content in English ")
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSubject() {
return subject;
}
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSubject(String subject) {
this.subject = subject;
}
/**
* The UTC time at which this email customization was created.
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The UTC time at which this email customization was created.")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
/**
* A unique identifier for this email customization
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A unique identifier for this email customization")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
public EmailCustomization isDefault(Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
/**
* Whether this is the default customization for the email template. Each customized email template must have
* exactly one default customization. Defaults to `true` for the first customization and `false`
* thereafter.
*
* @return isDefault
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether this is the default customization for the email template. Each customized email template must have exactly one default customization. Defaults to `true` for the first customization and `false` thereafter.")
@JsonProperty(JSON_PROPERTY_IS_DEFAULT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsDefault() {
return isDefault;
}
@JsonProperty(JSON_PROPERTY_IS_DEFAULT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsDefault(Boolean isDefault) {
this.isDefault = isDefault;
}
public EmailCustomization language(String language) {
this.language = language;
return this;
}
/**
* The language specified as an [IETF BCP 47 language tag](https://datatracker.ietf.org/doc/html/rfc5646)
*
* @return language
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The language specified as an [IETF BCP 47 language tag](https://datatracker.ietf.org/doc/html/rfc5646)")
@JsonProperty(JSON_PROPERTY_LANGUAGE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getLanguage() {
return language;
}
@JsonProperty(JSON_PROPERTY_LANGUAGE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLanguage(String language) {
this.language = language;
}
/**
* The UTC time at which this email customization was last updated.
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The UTC time at which this email customization was last updated.")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
public EmailCustomization links(EmailCustomizationAllOfLinks links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EmailCustomizationAllOfLinks getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(EmailCustomizationAllOfLinks links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailCustomization emailCustomization = (EmailCustomization) o;
return Objects.equals(this.body, emailCustomization.body)
&& Objects.equals(this.subject, emailCustomization.subject)
&& Objects.equals(this.created, emailCustomization.created)
&& Objects.equals(this.id, emailCustomization.id)
&& Objects.equals(this.isDefault, emailCustomization.isDefault)
&& Objects.equals(this.language, emailCustomization.language)
&& Objects.equals(this.lastUpdated, emailCustomization.lastUpdated)
&& Objects.equals(this.links, emailCustomization.links);
// ;
}
@Override
public int hashCode() {
return Objects.hash(body, subject, created, id, isDefault, language, lastUpdated, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailCustomization {\n");
sb.append(" body: ").append(toIndentedString(body)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isDefault: ").append(toIndentedString(isDefault)).append("\n");
sb.append(" language: ").append(toIndentedString(language)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy