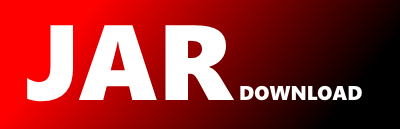
com.okta.sdk.resource.model.EmailDomainResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.EmailDomainDNSRecord;
import com.okta.sdk.resource.model.EmailDomainStatus;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* EmailDomainResponse
*/
@JsonPropertyOrder({ EmailDomainResponse.JSON_PROPERTY_DNS_VALIDATION_RECORDS, EmailDomainResponse.JSON_PROPERTY_DOMAIN,
EmailDomainResponse.JSON_PROPERTY_ID, EmailDomainResponse.JSON_PROPERTY_VALIDATION_STATUS,
EmailDomainResponse.JSON_PROPERTY_VALIDATION_SUBDOMAIN, EmailDomainResponse.JSON_PROPERTY_DISPLAY_NAME,
EmailDomainResponse.JSON_PROPERTY_USER_NAME })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class EmailDomainResponse implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_DNS_VALIDATION_RECORDS = "dnsValidationRecords";
private List dnsValidationRecords = null;
public static final String JSON_PROPERTY_DOMAIN = "domain";
private String domain;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_VALIDATION_STATUS = "validationStatus";
private EmailDomainStatus validationStatus;
public static final String JSON_PROPERTY_VALIDATION_SUBDOMAIN = "validationSubdomain";
private String validationSubdomain = "mail";
public static final String JSON_PROPERTY_DISPLAY_NAME = "displayName";
private String displayName;
public static final String JSON_PROPERTY_USER_NAME = "userName";
private String userName;
public EmailDomainResponse() {
}
public EmailDomainResponse dnsValidationRecords(List dnsValidationRecords) {
this.dnsValidationRecords = dnsValidationRecords;
return this;
}
public EmailDomainResponse adddnsValidationRecordsItem(EmailDomainDNSRecord dnsValidationRecordsItem) {
if (this.dnsValidationRecords == null) {
this.dnsValidationRecords = new ArrayList<>();
}
this.dnsValidationRecords.add(dnsValidationRecordsItem);
return this;
}
/**
* Get dnsValidationRecords
*
* @return dnsValidationRecords
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DNS_VALIDATION_RECORDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getDnsValidationRecords() {
return dnsValidationRecords;
}
@JsonProperty(JSON_PROPERTY_DNS_VALIDATION_RECORDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDnsValidationRecords(List dnsValidationRecords) {
this.dnsValidationRecords = dnsValidationRecords;
}
public EmailDomainResponse domain(String domain) {
this.domain = domain;
return this;
}
/**
* Get domain
*
* @return domain
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DOMAIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDomain() {
return domain;
}
@JsonProperty(JSON_PROPERTY_DOMAIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDomain(String domain) {
this.domain = domain;
}
public EmailDomainResponse id(String id) {
this.id = id;
return this;
}
/**
* Get id
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setId(String id) {
this.id = id;
}
public EmailDomainResponse validationStatus(EmailDomainStatus validationStatus) {
this.validationStatus = validationStatus;
return this;
}
/**
* Get validationStatus
*
* @return validationStatus
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_VALIDATION_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EmailDomainStatus getValidationStatus() {
return validationStatus;
}
@JsonProperty(JSON_PROPERTY_VALIDATION_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setValidationStatus(EmailDomainStatus validationStatus) {
this.validationStatus = validationStatus;
}
public EmailDomainResponse validationSubdomain(String validationSubdomain) {
this.validationSubdomain = validationSubdomain;
return this;
}
/**
* The subdomain for the email sender's custom mail domain
*
* @return validationSubdomain
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The subdomain for the email sender's custom mail domain")
@JsonProperty(JSON_PROPERTY_VALIDATION_SUBDOMAIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getValidationSubdomain() {
return validationSubdomain;
}
@JsonProperty(JSON_PROPERTY_VALIDATION_SUBDOMAIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setValidationSubdomain(String validationSubdomain) {
this.validationSubdomain = validationSubdomain;
}
public EmailDomainResponse displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get displayName
*
* @return displayName
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDisplayName() {
return displayName;
}
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public EmailDomainResponse userName(String userName) {
this.userName = userName;
return this;
}
/**
* Get userName
*
* @return userName
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_USER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getUserName() {
return userName;
}
@JsonProperty(JSON_PROPERTY_USER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUserName(String userName) {
this.userName = userName;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailDomainResponse emailDomainResponse = (EmailDomainResponse) o;
return Objects.equals(this.dnsValidationRecords, emailDomainResponse.dnsValidationRecords)
&& Objects.equals(this.domain, emailDomainResponse.domain)
&& Objects.equals(this.id, emailDomainResponse.id)
&& Objects.equals(this.validationStatus, emailDomainResponse.validationStatus)
&& Objects.equals(this.validationSubdomain, emailDomainResponse.validationSubdomain)
&& Objects.equals(this.displayName, emailDomainResponse.displayName)
&& Objects.equals(this.userName, emailDomainResponse.userName);
// ;
}
@Override
public int hashCode() {
return Objects.hash(dnsValidationRecords, domain, id, validationStatus, validationSubdomain, displayName,
userName);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailDomainResponse {\n");
sb.append(" dnsValidationRecords: ").append(toIndentedString(dnsValidationRecords)).append("\n");
sb.append(" domain: ").append(toIndentedString(domain)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" validationStatus: ").append(toIndentedString(validationStatus)).append("\n");
sb.append(" validationSubdomain: ").append(toIndentedString(validationSubdomain)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" userName: ").append(toIndentedString(userName)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy