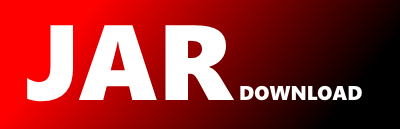
com.okta.sdk.resource.model.EmailPreviewLinks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.HrefObject;
import com.okta.sdk.resource.model.HrefObjectSelfLink;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* EmailPreviewLinks
*/
@JsonPropertyOrder({ EmailPreviewLinks.JSON_PROPERTY_SELF, EmailPreviewLinks.JSON_PROPERTY_CONTENT_SOURCE,
EmailPreviewLinks.JSON_PROPERTY_TEMPLATE, EmailPreviewLinks.JSON_PROPERTY_TEST,
EmailPreviewLinks.JSON_PROPERTY_DEFAULT_CONTENT })
@JsonTypeName("EmailPreview__links")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class EmailPreviewLinks implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_SELF = "self";
private HrefObjectSelfLink self;
public static final String JSON_PROPERTY_CONTENT_SOURCE = "contentSource";
private HrefObject contentSource;
public static final String JSON_PROPERTY_TEMPLATE = "template";
private HrefObject template;
public static final String JSON_PROPERTY_TEST = "test";
private HrefObject test;
public static final String JSON_PROPERTY_DEFAULT_CONTENT = "defaultContent";
private HrefObject defaultContent;
public EmailPreviewLinks() {
}
public EmailPreviewLinks self(HrefObjectSelfLink self) {
this.self = self;
return this;
}
/**
* Get self
*
* @return self
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObjectSelfLink getSelf() {
return self;
}
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSelf(HrefObjectSelfLink self) {
this.self = self;
}
public EmailPreviewLinks contentSource(HrefObject contentSource) {
this.contentSource = contentSource;
return this;
}
/**
* Get contentSource
*
* @return contentSource
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CONTENT_SOURCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getContentSource() {
return contentSource;
}
@JsonProperty(JSON_PROPERTY_CONTENT_SOURCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContentSource(HrefObject contentSource) {
this.contentSource = contentSource;
}
public EmailPreviewLinks template(HrefObject template) {
this.template = template;
return this;
}
/**
* Get template
*
* @return template
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TEMPLATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getTemplate() {
return template;
}
@JsonProperty(JSON_PROPERTY_TEMPLATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTemplate(HrefObject template) {
this.template = template;
}
public EmailPreviewLinks test(HrefObject test) {
this.test = test;
return this;
}
/**
* Get test
*
* @return test
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TEST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getTest() {
return test;
}
@JsonProperty(JSON_PROPERTY_TEST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTest(HrefObject test) {
this.test = test;
}
public EmailPreviewLinks defaultContent(HrefObject defaultContent) {
this.defaultContent = defaultContent;
return this;
}
/**
* Get defaultContent
*
* @return defaultContent
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DEFAULT_CONTENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getDefaultContent() {
return defaultContent;
}
@JsonProperty(JSON_PROPERTY_DEFAULT_CONTENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDefaultContent(HrefObject defaultContent) {
this.defaultContent = defaultContent;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailPreviewLinks emailPreviewLinks = (EmailPreviewLinks) o;
return Objects.equals(this.self, emailPreviewLinks.self)
&& Objects.equals(this.contentSource, emailPreviewLinks.contentSource)
&& Objects.equals(this.template, emailPreviewLinks.template)
&& Objects.equals(this.test, emailPreviewLinks.test)
&& Objects.equals(this.defaultContent, emailPreviewLinks.defaultContent);
// ;
}
@Override
public int hashCode() {
return Objects.hash(self, contentSource, template, test, defaultContent);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailPreviewLinks {\n");
sb.append(" self: ").append(toIndentedString(self)).append("\n");
sb.append(" contentSource: ").append(toIndentedString(contentSource)).append("\n");
sb.append(" template: ").append(toIndentedString(template)).append("\n");
sb.append(" test: ").append(toIndentedString(test)).append("\n");
sb.append(" defaultContent: ").append(toIndentedString(defaultContent)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy