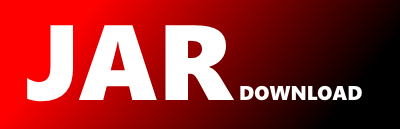
com.okta.sdk.resource.model.EmailServerRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* EmailServerRequest
*/
@JsonPropertyOrder({ EmailServerRequest.JSON_PROPERTY_ALIAS, EmailServerRequest.JSON_PROPERTY_ENABLED,
EmailServerRequest.JSON_PROPERTY_HOST, EmailServerRequest.JSON_PROPERTY_PORT,
EmailServerRequest.JSON_PROPERTY_USERNAME, EmailServerRequest.JSON_PROPERTY_PASSWORD })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class EmailServerRequest implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ALIAS = "alias";
private String alias;
public static final String JSON_PROPERTY_ENABLED = "enabled";
private Boolean enabled;
public static final String JSON_PROPERTY_HOST = "host";
private String host;
public static final String JSON_PROPERTY_PORT = "port";
private Integer port;
public static final String JSON_PROPERTY_USERNAME = "username";
private String username;
public static final String JSON_PROPERTY_PASSWORD = "password";
private String password;
public EmailServerRequest() {
}
public EmailServerRequest alias(String alias) {
this.alias = alias;
return this;
}
/**
* Human-readable name for your SMTP server
*
* @return alias
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "CustomServer1", value = "Human-readable name for your SMTP server")
@JsonProperty(JSON_PROPERTY_ALIAS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAlias() {
return alias;
}
@JsonProperty(JSON_PROPERTY_ALIAS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAlias(String alias) {
this.alias = alias;
}
public EmailServerRequest enabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* If `true`, routes all email traffic through your SMTP server
*
* @return enabled
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If `true`, routes all email traffic through your SMTP server")
@JsonProperty(JSON_PROPERTY_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getEnabled() {
return enabled;
}
@JsonProperty(JSON_PROPERTY_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
public EmailServerRequest host(String host) {
this.host = host;
return this;
}
/**
* Hostname or IP address of your SMTP server
*
* @return host
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "192.168.160.1", value = "Hostname or IP address of your SMTP server")
@JsonProperty(JSON_PROPERTY_HOST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getHost() {
return host;
}
@JsonProperty(JSON_PROPERTY_HOST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHost(String host) {
this.host = host;
}
public EmailServerRequest port(Integer port) {
this.port = port;
return this;
}
/**
* Port number of your SMTP server
*
* @return port
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "587", value = "Port number of your SMTP server")
@JsonProperty(JSON_PROPERTY_PORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPort() {
return port;
}
@JsonProperty(JSON_PROPERTY_PORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPort(Integer port) {
this.port = port;
}
public EmailServerRequest username(String username) {
this.username = username;
return this;
}
/**
* Username used to access your SMTP server
*
* @return username
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "aUser", value = "Username used to access your SMTP server")
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUsername() {
return username;
}
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUsername(String username) {
this.username = username;
}
public EmailServerRequest password(String password) {
this.password = password;
return this;
}
/**
* Password used to access your SMTP server
*
* @return password
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Password used to access your SMTP server")
@JsonProperty(JSON_PROPERTY_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPassword() {
return password;
}
@JsonProperty(JSON_PROPERTY_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPassword(String password) {
this.password = password;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailServerRequest emailServerRequest = (EmailServerRequest) o;
return Objects.equals(this.alias, emailServerRequest.alias)
&& Objects.equals(this.enabled, emailServerRequest.enabled)
&& Objects.equals(this.host, emailServerRequest.host)
&& Objects.equals(this.port, emailServerRequest.port)
&& Objects.equals(this.username, emailServerRequest.username)
&& Objects.equals(this.password, emailServerRequest.password);
// ;
}
@Override
public int hashCode() {
return Objects.hash(alias, enabled, host, port, username, password);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailServerRequest {\n");
sb.append(" alias: ").append(toIndentedString(alias)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" host: ").append(toIndentedString(host)).append("\n");
sb.append(" port: ").append(toIndentedString(port)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" password: ").append(toIndentedString(password)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy