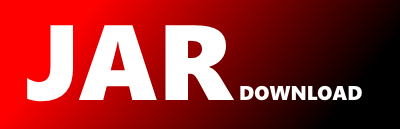
com.okta.sdk.resource.model.Embedded Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* The Public Key Details are defined in the `_embedded` property of the Key object.
*/
@ApiModel(description = "The Public Key Details are defined in the `_embedded` property of the Key object.")
@JsonPropertyOrder({ Embedded.JSON_PROPERTY_ALG, Embedded.JSON_PROPERTY_E, Embedded.JSON_PROPERTY_KID,
Embedded.JSON_PROPERTY_KTY, Embedded.JSON_PROPERTY_N, Embedded.JSON_PROPERTY_USE })
@JsonTypeName("_embedded")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class Embedded implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ALG = "alg";
private String alg;
public static final String JSON_PROPERTY_E = "e";
private String e;
public static final String JSON_PROPERTY_KID = "kid";
private String kid;
public static final String JSON_PROPERTY_KTY = "kty";
private String kty;
public static final String JSON_PROPERTY_N = "n";
private String n;
public static final String JSON_PROPERTY_USE = "use";
private JsonNullable use = JsonNullable. undefined();
public Embedded() {
}
/*
* @JsonCreator public Embedded(
*
* @JsonProperty(JSON_PROPERTY_ALG) String alg,
*
* @JsonProperty(JSON_PROPERTY_E) String e,
*
* @JsonProperty(JSON_PROPERTY_KID) String kid,
*
* @JsonProperty(JSON_PROPERTY_KTY) String kty,
*
* @JsonProperty(JSON_PROPERTY_N) String n,
*
* @JsonProperty(JSON_PROPERTY_USE) String use ) { this(); this.alg = alg; this.e = e; this.kid = kid; this.kty =
* kty; this.n = n; this.use = use; }
*/
/**
* Algorithm used in the key
*
* @return alg
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Algorithm used in the key")
@JsonProperty(JSON_PROPERTY_ALG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAlg() {
return alg;
}
/**
* RSA key value (exponent) for key binding
*
* @return e
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "RSA key value (exponent) for key binding")
@JsonProperty(JSON_PROPERTY_E)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getE() {
return e;
}
/**
* Unique identifier for the certificate
*
* @return kid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the certificate")
@JsonProperty(JSON_PROPERTY_KID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getKid() {
return kid;
}
/**
* Cryptographic algorithm family for the certificate's keypair
*
* @return kty
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Cryptographic algorithm family for the certificate's keypair")
@JsonProperty(JSON_PROPERTY_KTY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getKty() {
return kty;
}
/**
* RSA key value (modulus) for key binding
*
* @return n
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "RSA key value (modulus) for key binding")
@JsonProperty(JSON_PROPERTY_N)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getN() {
return n;
}
/**
* Acceptable use of the certificate
*
* @return use
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Acceptable use of the certificate")
@JsonIgnore
public String getUse() {
if (use == null) {
use = JsonNullable. undefined();
}
return use.orElse(null);
}
@JsonProperty(JSON_PROPERTY_USE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getUse_JsonNullable() {
return use;
}
@JsonProperty(JSON_PROPERTY_USE)
private void setUse_JsonNullable(JsonNullable use) {
this.use = use;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Embedded embedded = (Embedded) o;
return Objects.equals(this.alg, embedded.alg) && Objects.equals(this.e, embedded.e)
&& Objects.equals(this.kid, embedded.kid) && Objects.equals(this.kty, embedded.kty)
&& Objects.equals(this.n, embedded.n) && equalsNullable(this.use, embedded.use);
// ;
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(alg, e, kid, kty, n, hashCodeNullable(use));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Embedded {\n");
sb.append(" alg: ").append(toIndentedString(alg)).append("\n");
sb.append(" e: ").append(toIndentedString(e)).append("\n");
sb.append(" kid: ").append(toIndentedString(kid)).append("\n");
sb.append(" kty: ").append(toIndentedString(kty)).append("\n");
sb.append(" n: ").append(toIndentedString(n)).append("\n");
sb.append(" use: ").append(toIndentedString(use)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy