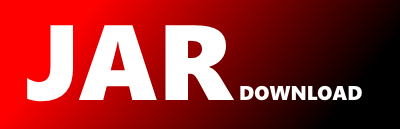
com.okta.sdk.resource.model.EventHookChannelConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.EventHookChannelConfigAuthScheme;
import com.okta.sdk.resource.model.EventHookChannelConfigHeader;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* EventHookChannelConfig
*/
@JsonPropertyOrder({ EventHookChannelConfig.JSON_PROPERTY_AUTH_SCHEME, EventHookChannelConfig.JSON_PROPERTY_HEADERS,
EventHookChannelConfig.JSON_PROPERTY_METHOD, EventHookChannelConfig.JSON_PROPERTY_URI })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class EventHookChannelConfig implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AUTH_SCHEME = "authScheme";
private EventHookChannelConfigAuthScheme authScheme;
public static final String JSON_PROPERTY_HEADERS = "headers";
private List headers = null;
public static final String JSON_PROPERTY_METHOD = "method";
private String method;
public static final String JSON_PROPERTY_URI = "uri";
private String uri;
public EventHookChannelConfig() {
}
/*
* @JsonCreator public EventHookChannelConfig(
*
* @JsonProperty(JSON_PROPERTY_METHOD) String method ) { this(); this.method = method; }
*/
public EventHookChannelConfig authScheme(EventHookChannelConfigAuthScheme authScheme) {
this.authScheme = authScheme;
return this;
}
/**
* Get authScheme
*
* @return authScheme
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AUTH_SCHEME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EventHookChannelConfigAuthScheme getAuthScheme() {
return authScheme;
}
@JsonProperty(JSON_PROPERTY_AUTH_SCHEME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthScheme(EventHookChannelConfigAuthScheme authScheme) {
this.authScheme = authScheme;
}
public EventHookChannelConfig headers(List headers) {
this.headers = headers;
return this;
}
public EventHookChannelConfig addheadersItem(EventHookChannelConfigHeader headersItem) {
if (this.headers == null) {
this.headers = new ArrayList<>();
}
this.headers.add(headersItem);
return this;
}
/**
* Optional list of key/value pairs for headers that can be sent with the request to the external service. For
* example, `X-Other-Header` is an example of an optional header, with a value of
* `my-header-value`, that you want Okta to pass to your external service.
*
* @return headers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Optional list of key/value pairs for headers that can be sent with the request to the external service. For example, `X-Other-Header` is an example of an optional header, with a value of `my-header-value`, that you want Okta to pass to your external service.")
@JsonProperty(JSON_PROPERTY_HEADERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getHeaders() {
return headers;
}
@JsonProperty(JSON_PROPERTY_HEADERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHeaders(List headers) {
this.headers = headers;
}
/**
* The method of the Okta event hook request
*
* @return method
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The method of the Okta event hook request")
@JsonProperty(JSON_PROPERTY_METHOD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMethod() {
return method;
}
public EventHookChannelConfig uri(String uri) {
this.uri = uri;
return this;
}
/**
* The external service endpoint called to execute the event hook handler
*
* @return uri
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The external service endpoint called to execute the event hook handler")
@JsonProperty(JSON_PROPERTY_URI)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getUri() {
return uri;
}
@JsonProperty(JSON_PROPERTY_URI)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUri(String uri) {
this.uri = uri;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EventHookChannelConfig eventHookChannelConfig = (EventHookChannelConfig) o;
return Objects.equals(this.authScheme, eventHookChannelConfig.authScheme)
&& Objects.equals(this.headers, eventHookChannelConfig.headers)
&& Objects.equals(this.method, eventHookChannelConfig.method)
&& Objects.equals(this.uri, eventHookChannelConfig.uri);
// ;
}
@Override
public int hashCode() {
return Objects.hash(authScheme, headers, method, uri);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EventHookChannelConfig {\n");
sb.append(" authScheme: ").append(toIndentedString(authScheme)).append("\n");
sb.append(" headers: ").append(toIndentedString(headers)).append("\n");
sb.append(" method: ").append(toIndentedString(method)).append("\n");
sb.append(" uri: ").append(toIndentedString(uri)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy