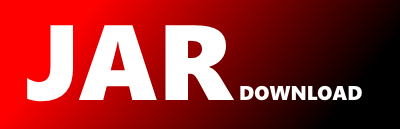
com.okta.sdk.resource.model.EventSubscriptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.EventHookFilters;
import com.okta.sdk.resource.model.EventSubscriptionType;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* EventSubscriptions
*/
@JsonPropertyOrder({ EventSubscriptions.JSON_PROPERTY_FILTER, EventSubscriptions.JSON_PROPERTY_ITEMS,
EventSubscriptions.JSON_PROPERTY_TYPE })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class EventSubscriptions implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_FILTER = "filter";
private JsonNullable filter = JsonNullable. undefined();
public static final String JSON_PROPERTY_ITEMS = "items";
private List items = new ArrayList<>();
public static final String JSON_PROPERTY_TYPE = "type";
private EventSubscriptionType type;
public EventSubscriptions() {
}
public EventSubscriptions filter(EventHookFilters filter) {
this.filter = JsonNullable. of(filter);
return this;
}
/**
* Get filter
*
* @return filter
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonIgnore
public EventHookFilters getFilter() {
return filter.orElse(null);
}
@JsonProperty(JSON_PROPERTY_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getFilter_JsonNullable() {
return filter;
}
@JsonProperty(JSON_PROPERTY_FILTER)
public void setFilter_JsonNullable(JsonNullable filter) {
this.filter = filter;
}
public void setFilter(EventHookFilters filter) {
this.filter = JsonNullable. of(filter);
}
public EventSubscriptions items(List items) {
this.items = items;
return this;
}
public EventSubscriptions additemsItem(String itemsItem) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.add(itemsItem);
return this;
}
/**
* The subscribed event types that trigger the event hook. When you register an event hook you need to specify which
* events you want to subscribe to. To see the list of event types currently eligible for use in event hooks, use
* the [Event Types catalog](https://developer.okta.com/docs/reference/api/event-types/#catalog) and search with the
* parameter `event-hook-eligible`.
*
* @return items
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The subscribed event types that trigger the event hook. When you register an event hook you need to specify which events you want to subscribe to. To see the list of event types currently eligible for use in event hooks, use the [Event Types catalog](https://developer.okta.com/docs/reference/api/event-types/#catalog) and search with the parameter `event-hook-eligible`.")
@JsonProperty(JSON_PROPERTY_ITEMS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getItems() {
return items;
}
@JsonProperty(JSON_PROPERTY_ITEMS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setItems(List items) {
this.items = items;
}
public EventSubscriptions type(EventSubscriptionType type) {
this.type = type;
return this;
}
/**
* Get type
*
* @return type
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public EventSubscriptionType getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setType(EventSubscriptionType type) {
this.type = type;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EventSubscriptions eventSubscriptions = (EventSubscriptions) o;
return equalsNullable(this.filter, eventSubscriptions.filter)
&& Objects.equals(this.items, eventSubscriptions.items)
&& Objects.equals(this.type, eventSubscriptions.type);
// ;
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(filter), items, type);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EventSubscriptions {\n");
sb.append(" filter: ").append(toIndentedString(filter)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy