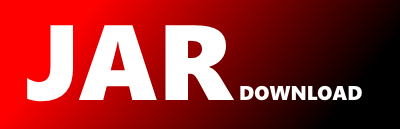
com.okta.sdk.resource.model.GoogleApplicationSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.ApplicationSettingsNotes;
import com.okta.sdk.resource.model.ApplicationSettingsNotifications;
import com.okta.sdk.resource.model.GoogleApplicationSettingsApplication;
import com.okta.sdk.resource.model.OINSaml20ApplicationSettingsSignOn;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* GoogleApplicationSettings
*/
@JsonPropertyOrder({ GoogleApplicationSettings.JSON_PROPERTY_IDENTITY_STORE_ID,
GoogleApplicationSettings.JSON_PROPERTY_IMPLICIT_ASSIGNMENT,
GoogleApplicationSettings.JSON_PROPERTY_INLINE_HOOK_ID, GoogleApplicationSettings.JSON_PROPERTY_NOTES,
GoogleApplicationSettings.JSON_PROPERTY_NOTIFICATIONS, GoogleApplicationSettings.JSON_PROPERTY_SIGN_ON,
GoogleApplicationSettings.JSON_PROPERTY_APP })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class GoogleApplicationSettings implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_IDENTITY_STORE_ID = "identityStoreId";
private String identityStoreId;
public static final String JSON_PROPERTY_IMPLICIT_ASSIGNMENT = "implicitAssignment";
private Boolean implicitAssignment;
public static final String JSON_PROPERTY_INLINE_HOOK_ID = "inlineHookId";
private String inlineHookId;
public static final String JSON_PROPERTY_NOTES = "notes";
private ApplicationSettingsNotes notes;
public static final String JSON_PROPERTY_NOTIFICATIONS = "notifications";
private ApplicationSettingsNotifications notifications;
public static final String JSON_PROPERTY_SIGN_ON = "signOn";
private OINSaml20ApplicationSettingsSignOn signOn;
public static final String JSON_PROPERTY_APP = "app";
private GoogleApplicationSettingsApplication app;
public GoogleApplicationSettings() {
}
public GoogleApplicationSettings identityStoreId(String identityStoreId) {
this.identityStoreId = identityStoreId;
return this;
}
/**
* Identifies an additional identity store app, if your app supports it. The `identityStoreId` value must
* be a valid identity store app ID. This identity store app must be created in the same org as your app.
*
* @return identityStoreId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifies an additional identity store app, if your app supports it. The `identityStoreId` value must be a valid identity store app ID. This identity store app must be created in the same org as your app.")
@JsonProperty(JSON_PROPERTY_IDENTITY_STORE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIdentityStoreId() {
return identityStoreId;
}
@JsonProperty(JSON_PROPERTY_IDENTITY_STORE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIdentityStoreId(String identityStoreId) {
this.identityStoreId = identityStoreId;
}
public GoogleApplicationSettings implicitAssignment(Boolean implicitAssignment) {
this.implicitAssignment = implicitAssignment;
return this;
}
/**
* Controls whether Okta automatically assigns users to the app based on the user's role or group membership.
*
* @return implicitAssignment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Controls whether Okta automatically assigns users to the app based on the user's role or group membership.")
@JsonProperty(JSON_PROPERTY_IMPLICIT_ASSIGNMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getImplicitAssignment() {
return implicitAssignment;
}
@JsonProperty(JSON_PROPERTY_IMPLICIT_ASSIGNMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setImplicitAssignment(Boolean implicitAssignment) {
this.implicitAssignment = implicitAssignment;
}
public GoogleApplicationSettings inlineHookId(String inlineHookId) {
this.inlineHookId = inlineHookId;
return this;
}
/**
* Identifier of an inline hook. Inline hooks are outbound calls from Okta to your own custom code, triggered at
* specific points in Okta process flows. They allow you to integrate custom functionality into those flows. See
* [Inline hooks](/openapi/okta-management/management/tag/InlineHook/).
*
* @return inlineHookId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of an inline hook. Inline hooks are outbound calls from Okta to your own custom code, triggered at specific points in Okta process flows. They allow you to integrate custom functionality into those flows. See [Inline hooks](/openapi/okta-management/management/tag/InlineHook/).")
@JsonProperty(JSON_PROPERTY_INLINE_HOOK_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getInlineHookId() {
return inlineHookId;
}
@JsonProperty(JSON_PROPERTY_INLINE_HOOK_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInlineHookId(String inlineHookId) {
this.inlineHookId = inlineHookId;
}
public GoogleApplicationSettings notes(ApplicationSettingsNotes notes) {
this.notes = notes;
return this;
}
/**
* Get notes
*
* @return notes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NOTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationSettingsNotes getNotes() {
return notes;
}
@JsonProperty(JSON_PROPERTY_NOTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNotes(ApplicationSettingsNotes notes) {
this.notes = notes;
}
public GoogleApplicationSettings notifications(ApplicationSettingsNotifications notifications) {
this.notifications = notifications;
return this;
}
/**
* Get notifications
*
* @return notifications
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NOTIFICATIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationSettingsNotifications getNotifications() {
return notifications;
}
@JsonProperty(JSON_PROPERTY_NOTIFICATIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNotifications(ApplicationSettingsNotifications notifications) {
this.notifications = notifications;
}
public GoogleApplicationSettings signOn(OINSaml20ApplicationSettingsSignOn signOn) {
this.signOn = signOn;
return this;
}
/**
* Get signOn
*
* @return signOn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SIGN_ON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OINSaml20ApplicationSettingsSignOn getSignOn() {
return signOn;
}
@JsonProperty(JSON_PROPERTY_SIGN_ON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSignOn(OINSaml20ApplicationSettingsSignOn signOn) {
this.signOn = signOn;
}
public GoogleApplicationSettings app(GoogleApplicationSettingsApplication app) {
this.app = app;
return this;
}
/**
* Get app
*
* @return app
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_APP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public GoogleApplicationSettingsApplication getApp() {
return app;
}
@JsonProperty(JSON_PROPERTY_APP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setApp(GoogleApplicationSettingsApplication app) {
this.app = app;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GoogleApplicationSettings googleApplicationSettings = (GoogleApplicationSettings) o;
return Objects.equals(this.identityStoreId, googleApplicationSettings.identityStoreId)
&& Objects.equals(this.implicitAssignment, googleApplicationSettings.implicitAssignment)
&& Objects.equals(this.inlineHookId, googleApplicationSettings.inlineHookId)
&& Objects.equals(this.notes, googleApplicationSettings.notes)
&& Objects.equals(this.notifications, googleApplicationSettings.notifications)
&& Objects.equals(this.signOn, googleApplicationSettings.signOn)
&& Objects.equals(this.app, googleApplicationSettings.app);
// ;
}
@Override
public int hashCode() {
return Objects.hash(identityStoreId, implicitAssignment, inlineHookId, notes, notifications, signOn, app);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GoogleApplicationSettings {\n");
sb.append(" identityStoreId: ").append(toIndentedString(identityStoreId)).append("\n");
sb.append(" implicitAssignment: ").append(toIndentedString(implicitAssignment)).append("\n");
sb.append(" inlineHookId: ").append(toIndentedString(inlineHookId)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" notifications: ").append(toIndentedString(notifications)).append("\n");
sb.append(" signOn: ").append(toIndentedString(signOn)).append("\n");
sb.append(" app: ").append(toIndentedString(app)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy