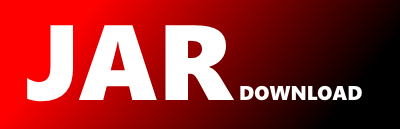
com.okta.sdk.resource.model.GroupSchemaAttribute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.GroupSchemaAttributeEnumInner;
import com.okta.sdk.resource.model.UserSchemaAttributeEnum;
import com.okta.sdk.resource.model.UserSchemaAttributeFormat;
import com.okta.sdk.resource.model.UserSchemaAttributeItems;
import com.okta.sdk.resource.model.UserSchemaAttributeMaster;
import com.okta.sdk.resource.model.UserSchemaAttributeMutabilityString;
import com.okta.sdk.resource.model.UserSchemaAttributePermission;
import com.okta.sdk.resource.model.UserSchemaAttributeScope;
import com.okta.sdk.resource.model.UserSchemaAttributeType;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* GroupSchemaAttribute
*/
@JsonPropertyOrder({ GroupSchemaAttribute.JSON_PROPERTY_DESCRIPTION, GroupSchemaAttribute.JSON_PROPERTY_ENUM,
GroupSchemaAttribute.JSON_PROPERTY_EXTERNAL_NAME, GroupSchemaAttribute.JSON_PROPERTY_EXTERNAL_NAMESPACE,
GroupSchemaAttribute.JSON_PROPERTY_FORMAT, GroupSchemaAttribute.JSON_PROPERTY_ITEMS,
GroupSchemaAttribute.JSON_PROPERTY_MASTER, GroupSchemaAttribute.JSON_PROPERTY_MAX_LENGTH,
GroupSchemaAttribute.JSON_PROPERTY_MIN_LENGTH, GroupSchemaAttribute.JSON_PROPERTY_MUTABILITY,
GroupSchemaAttribute.JSON_PROPERTY_ONE_OF, GroupSchemaAttribute.JSON_PROPERTY_PERMISSIONS,
GroupSchemaAttribute.JSON_PROPERTY_REQUIRED, GroupSchemaAttribute.JSON_PROPERTY_SCOPE,
GroupSchemaAttribute.JSON_PROPERTY_TITLE, GroupSchemaAttribute.JSON_PROPERTY_TYPE,
GroupSchemaAttribute.JSON_PROPERTY_UNIQUE })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class GroupSchemaAttribute implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_ENUM = "enum";
private JsonNullable> _enum = JsonNullable
.> undefined();
public static final String JSON_PROPERTY_EXTERNAL_NAME = "externalName";
private String externalName;
public static final String JSON_PROPERTY_EXTERNAL_NAMESPACE = "externalNamespace";
private String externalNamespace;
public static final String JSON_PROPERTY_FORMAT = "format";
private UserSchemaAttributeFormat format;
public static final String JSON_PROPERTY_ITEMS = "items";
private UserSchemaAttributeItems items;
public static final String JSON_PROPERTY_MASTER = "master";
private JsonNullable master = JsonNullable. undefined();
public static final String JSON_PROPERTY_MAX_LENGTH = "maxLength";
private JsonNullable maxLength = JsonNullable. undefined();
public static final String JSON_PROPERTY_MIN_LENGTH = "minLength";
private JsonNullable minLength = JsonNullable. undefined();
public static final String JSON_PROPERTY_MUTABILITY = "mutability";
private UserSchemaAttributeMutabilityString mutability;
public static final String JSON_PROPERTY_ONE_OF = "oneOf";
private JsonNullable> oneOf = JsonNullable
.> undefined();
public static final String JSON_PROPERTY_PERMISSIONS = "permissions";
private JsonNullable> permissions = JsonNullable
.> undefined();
public static final String JSON_PROPERTY_REQUIRED = "required";
private JsonNullable required = JsonNullable. undefined();
public static final String JSON_PROPERTY_SCOPE = "scope";
private UserSchemaAttributeScope scope;
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public static final String JSON_PROPERTY_TYPE = "type";
private UserSchemaAttributeType type;
public static final String JSON_PROPERTY_UNIQUE = "unique";
private String unique;
public GroupSchemaAttribute() {
}
public GroupSchemaAttribute description(String description) {
this.description = description;
return this;
}
/**
* Description of the property
*
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Description of the property")
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public GroupSchemaAttribute _enum(List _enum) {
this._enum = JsonNullable.> of(_enum);
return this;
}
public GroupSchemaAttribute addenumItem(GroupSchemaAttributeEnumInner _enumItem) {
if (this._enum == null || !this._enum.isPresent()) {
this._enum = JsonNullable.> of(new ArrayList<>());
}
try {
this._enum.get().add(_enumItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Enumerated value of the property. The value of the property is limited to one of the values specified in the enum
* definition. The list of values for the enum must consist of unique elements.
*
* @return _enum
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Enumerated value of the property. The value of the property is limited to one of the values specified in the enum definition. The list of values for the enum must consist of unique elements.")
@JsonIgnore
public List getEnum() {
return _enum.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ENUM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getEnum_JsonNullable() {
return _enum;
}
@JsonProperty(JSON_PROPERTY_ENUM)
public void setEnum_JsonNullable(JsonNullable> _enum) {
this._enum = _enum;
}
public void setEnum(List _enum) {
this._enum = JsonNullable.> of(_enum);
}
public GroupSchemaAttribute externalName(String externalName) {
this.externalName = externalName;
return this;
}
/**
* Name of the property as it exists in an external application
*
* @return externalName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the property as it exists in an external application")
@JsonProperty(JSON_PROPERTY_EXTERNAL_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalName() {
return externalName;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExternalName(String externalName) {
this.externalName = externalName;
}
public GroupSchemaAttribute externalNamespace(String externalNamespace) {
this.externalNamespace = externalNamespace;
return this;
}
/**
* Namespace from the external application
*
* @return externalNamespace
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Namespace from the external application")
@JsonProperty(JSON_PROPERTY_EXTERNAL_NAMESPACE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalNamespace() {
return externalNamespace;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_NAMESPACE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExternalNamespace(String externalNamespace) {
this.externalNamespace = externalNamespace;
}
public GroupSchemaAttribute format(UserSchemaAttributeFormat format) {
this.format = format;
return this;
}
/**
* Identifies the type of data represented by the string
*
* @return format
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifies the type of data represented by the string")
@JsonProperty(JSON_PROPERTY_FORMAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserSchemaAttributeFormat getFormat() {
return format;
}
@JsonProperty(JSON_PROPERTY_FORMAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFormat(UserSchemaAttributeFormat format) {
this.format = format;
}
public GroupSchemaAttribute items(UserSchemaAttributeItems items) {
this.items = items;
return this;
}
/**
* Get items
*
* @return items
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ITEMS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserSchemaAttributeItems getItems() {
return items;
}
@JsonProperty(JSON_PROPERTY_ITEMS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setItems(UserSchemaAttributeItems items) {
this.items = items;
}
public GroupSchemaAttribute master(UserSchemaAttributeMaster master) {
this.master = JsonNullable. of(master);
return this;
}
/**
* Identifies where the property is mastered
*
* @return master
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifies where the property is mastered")
@JsonIgnore
public UserSchemaAttributeMaster getMaster() {
return master.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MASTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getMaster_JsonNullable() {
return master;
}
@JsonProperty(JSON_PROPERTY_MASTER)
public void setMaster_JsonNullable(JsonNullable master) {
this.master = master;
}
public void setMaster(UserSchemaAttributeMaster master) {
this.master = JsonNullable. of(master);
}
public GroupSchemaAttribute maxLength(Integer maxLength) {
this.maxLength = JsonNullable. of(maxLength);
return this;
}
/**
* Maximum character length of a string property
*
* @return maxLength
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Maximum character length of a string property")
@JsonIgnore
public Integer getMaxLength() {
return maxLength.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MAX_LENGTH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getMaxLength_JsonNullable() {
return maxLength;
}
@JsonProperty(JSON_PROPERTY_MAX_LENGTH)
public void setMaxLength_JsonNullable(JsonNullable maxLength) {
this.maxLength = maxLength;
}
public void setMaxLength(Integer maxLength) {
this.maxLength = JsonNullable. of(maxLength);
}
public GroupSchemaAttribute minLength(Integer minLength) {
this.minLength = JsonNullable. of(minLength);
return this;
}
/**
* Minimum character length of a string property
*
* @return minLength
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Minimum character length of a string property")
@JsonIgnore
public Integer getMinLength() {
return minLength.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MIN_LENGTH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getMinLength_JsonNullable() {
return minLength;
}
@JsonProperty(JSON_PROPERTY_MIN_LENGTH)
public void setMinLength_JsonNullable(JsonNullable minLength) {
this.minLength = minLength;
}
public void setMinLength(Integer minLength) {
this.minLength = JsonNullable. of(minLength);
}
public GroupSchemaAttribute mutability(UserSchemaAttributeMutabilityString mutability) {
this.mutability = mutability;
return this;
}
/**
* Defines the mutability of the property
*
* @return mutability
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Defines the mutability of the property")
@JsonProperty(JSON_PROPERTY_MUTABILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserSchemaAttributeMutabilityString getMutability() {
return mutability;
}
@JsonProperty(JSON_PROPERTY_MUTABILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMutability(UserSchemaAttributeMutabilityString mutability) {
this.mutability = mutability;
}
public GroupSchemaAttribute oneOf(List oneOf) {
this.oneOf = JsonNullable.> of(oneOf);
return this;
}
public GroupSchemaAttribute addoneOfItem(UserSchemaAttributeEnum oneOfItem) {
if (this.oneOf == null || !this.oneOf.isPresent()) {
this.oneOf = JsonNullable.> of(new ArrayList<>());
}
try {
this.oneOf.get().add(oneOfItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Non-empty array of valid JSON schemas. Okta only supports `oneOf` for specifying display names for an
* `enum`. Each schema has the following format: ``` { \"const\":
* \"enumValue\", \"title\": \"display name\" } ```json When
* `enum` is used in conjunction with `oneOf`, you must keep the set of enumerated values and
* their order. ``` {\"enum\":
* [\"S\",\"M\",\"L\",\"XL\"], \"oneOf\": [ {\"const\":
* \"S\", \"title\": \"Small\"}, {\"const\": \"M\",
* \"title\": \"Medium\"}, {\"const\": \"L\", \"title\":
* \"Large\"}, {\"const\": \"XL\", \"title\": \"Extra Large\"} ] }
* ```json The `oneOf` key is only supported in conjunction with `enum` and
* provides a mechanism to return a display name for the `enum` value.
*
* @return oneOf
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Non-empty array of valid JSON schemas. Okta only supports `oneOf` for specifying display names for an `enum`. Each schema has the following format: ``` { \"const\": \"enumValue\", \"title\": \"display name\" } ```json When `enum` is used in conjunction with `oneOf`, you must keep the set of enumerated values and their order. ``` {\"enum\": [\"S\",\"M\",\"L\",\"XL\"], \"oneOf\": [ {\"const\": \"S\", \"title\": \"Small\"}, {\"const\": \"M\", \"title\": \"Medium\"}, {\"const\": \"L\", \"title\": \"Large\"}, {\"const\": \"XL\", \"title\": \"Extra Large\"} ] } ```json The `oneOf` key is only supported in conjunction with `enum` and provides a mechanism to return a display name for the `enum` value. ")
@JsonIgnore
public List getOneOf() {
return oneOf.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ONE_OF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getOneOf_JsonNullable() {
return oneOf;
}
@JsonProperty(JSON_PROPERTY_ONE_OF)
public void setOneOf_JsonNullable(JsonNullable> oneOf) {
this.oneOf = oneOf;
}
public void setOneOf(List oneOf) {
this.oneOf = JsonNullable.> of(oneOf);
}
public GroupSchemaAttribute permissions(List permissions) {
this.permissions = JsonNullable.> of(permissions);
return this;
}
public GroupSchemaAttribute addpermissionsItem(UserSchemaAttributePermission permissionsItem) {
if (this.permissions == null || !this.permissions.isPresent()) {
this.permissions = JsonNullable.> of(new ArrayList<>());
}
try {
this.permissions.get().add(permissionsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Access control permissions for the property
*
* @return permissions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Access control permissions for the property")
@JsonIgnore
public List getPermissions() {
return permissions.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PERMISSIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getPermissions_JsonNullable() {
return permissions;
}
@JsonProperty(JSON_PROPERTY_PERMISSIONS)
public void setPermissions_JsonNullable(JsonNullable> permissions) {
this.permissions = permissions;
}
public void setPermissions(List permissions) {
this.permissions = JsonNullable.> of(permissions);
}
public GroupSchemaAttribute required(Boolean required) {
this.required = JsonNullable. of(required);
return this;
}
/**
* Determines whether the property is required
*
* @return required
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Determines whether the property is required")
@JsonIgnore
public Boolean getRequired() {
return required.orElse(null);
}
@JsonProperty(JSON_PROPERTY_REQUIRED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getRequired_JsonNullable() {
return required;
}
@JsonProperty(JSON_PROPERTY_REQUIRED)
public void setRequired_JsonNullable(JsonNullable required) {
this.required = required;
}
public void setRequired(Boolean required) {
this.required = JsonNullable. of(required);
}
public GroupSchemaAttribute scope(UserSchemaAttributeScope scope) {
this.scope = scope;
return this;
}
/**
* Determines whether a group attribute can be set at the individual or group level
*
* @return scope
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Determines whether a group attribute can be set at the individual or group level")
@JsonProperty(JSON_PROPERTY_SCOPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserSchemaAttributeScope getScope() {
return scope;
}
@JsonProperty(JSON_PROPERTY_SCOPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setScope(UserSchemaAttributeScope scope) {
this.scope = scope;
}
public GroupSchemaAttribute title(String title) {
this.title = title;
return this;
}
/**
* User-defined display name for the property
*
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "User-defined display name for the property")
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
public GroupSchemaAttribute type(UserSchemaAttributeType type) {
this.type = type;
return this;
}
/**
* Type of property
*
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Type of property")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserSchemaAttributeType getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(UserSchemaAttributeType type) {
this.type = type;
}
public GroupSchemaAttribute unique(String unique) {
this.unique = unique;
return this;
}
/**
* Get unique
*
* @return unique
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_UNIQUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUnique() {
return unique;
}
@JsonProperty(JSON_PROPERTY_UNIQUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUnique(String unique) {
this.unique = unique;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GroupSchemaAttribute groupSchemaAttribute = (GroupSchemaAttribute) o;
return Objects.equals(this.description, groupSchemaAttribute.description)
&& equalsNullable(this._enum, groupSchemaAttribute._enum)
&& Objects.equals(this.externalName, groupSchemaAttribute.externalName)
&& Objects.equals(this.externalNamespace, groupSchemaAttribute.externalNamespace)
&& Objects.equals(this.format, groupSchemaAttribute.format)
&& Objects.equals(this.items, groupSchemaAttribute.items)
&& equalsNullable(this.master, groupSchemaAttribute.master)
&& equalsNullable(this.maxLength, groupSchemaAttribute.maxLength)
&& equalsNullable(this.minLength, groupSchemaAttribute.minLength)
&& Objects.equals(this.mutability, groupSchemaAttribute.mutability)
&& equalsNullable(this.oneOf, groupSchemaAttribute.oneOf)
&& equalsNullable(this.permissions, groupSchemaAttribute.permissions)
&& equalsNullable(this.required, groupSchemaAttribute.required)
&& Objects.equals(this.scope, groupSchemaAttribute.scope)
&& Objects.equals(this.title, groupSchemaAttribute.title)
&& Objects.equals(this.type, groupSchemaAttribute.type)
&& Objects.equals(this.unique, groupSchemaAttribute.unique);
// ;
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(description, hashCodeNullable(_enum), externalName, externalNamespace, format, items,
hashCodeNullable(master), hashCodeNullable(maxLength), hashCodeNullable(minLength), mutability,
hashCodeNullable(oneOf), hashCodeNullable(permissions), hashCodeNullable(required), scope, title, type,
unique);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GroupSchemaAttribute {\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" _enum: ").append(toIndentedString(_enum)).append("\n");
sb.append(" externalName: ").append(toIndentedString(externalName)).append("\n");
sb.append(" externalNamespace: ").append(toIndentedString(externalNamespace)).append("\n");
sb.append(" format: ").append(toIndentedString(format)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append(" master: ").append(toIndentedString(master)).append("\n");
sb.append(" maxLength: ").append(toIndentedString(maxLength)).append("\n");
sb.append(" minLength: ").append(toIndentedString(minLength)).append("\n");
sb.append(" mutability: ").append(toIndentedString(mutability)).append("\n");
sb.append(" oneOf: ").append(toIndentedString(oneOf)).append("\n");
sb.append(" permissions: ").append(toIndentedString(permissions)).append("\n");
sb.append(" required: ").append(toIndentedString(required)).append("\n");
sb.append(" scope: ").append(toIndentedString(scope)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" unique: ").append(toIndentedString(unique)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy