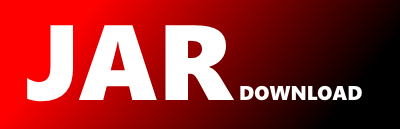
com.okta.sdk.resource.model.HookKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* The `id` property in the response as `id` serves as the unique ID for the key, which you can
* specify when invoking other CRUD operations. The `keyId` provided in the response is the alias of the
* public key that you can use to get details of the public key data in a separate call.
*/
@ApiModel(description = "The `id` property in the response as `id` serves as the unique ID for the key, which you can specify when invoking other CRUD operations. The `keyId` provided in the response is the alias of the public key that you can use to get details of the public key data in a separate call.")
@JsonPropertyOrder({ HookKey.JSON_PROPERTY_CREATED, HookKey.JSON_PROPERTY_ID, HookKey.JSON_PROPERTY_IS_USED,
HookKey.JSON_PROPERTY_KEY_ID, HookKey.JSON_PROPERTY_LAST_UPDATED, HookKey.JSON_PROPERTY_NAME })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class HookKey implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_CREATED = "created";
private JsonNullable created = JsonNullable. undefined();
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IS_USED = "isUsed";
private Boolean isUsed;
public static final String JSON_PROPERTY_KEY_ID = "keyId";
private String keyId;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private JsonNullable lastUpdated = JsonNullable. undefined();
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public HookKey() {
}
/*
* @JsonCreator public HookKey(
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_IS_USED) Boolean isUsed,
*
* @JsonProperty(JSON_PROPERTY_KEY_ID) String keyId,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated ) { this(); this.created = created; this.id
* = id; this.isUsed = isUsed; this.keyId = keyId; this.lastUpdated = lastUpdated; }
*/
/**
* Timestamp when the key was created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Timestamp when the key was created")
@JsonIgnore
public OffsetDateTime getCreated() {
if (created == null) {
created = JsonNullable. undefined();
}
return created.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCreated_JsonNullable() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
private void setCreated_JsonNullable(JsonNullable created) {
this.created = created;
}
/**
* The unique identifier for the key
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique identifier for the key")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
/**
* Whether this key is currently in use by other applications
*
* @return isUsed
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether this key is currently in use by other applications")
@JsonProperty(JSON_PROPERTY_IS_USED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsUsed() {
return isUsed;
}
/**
* The alias of the public key
*
* @return keyId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The alias of the public key")
@JsonProperty(JSON_PROPERTY_KEY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getKeyId() {
return keyId;
}
/**
* Timestamp when the key was updated
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Timestamp when the key was updated")
@JsonIgnore
public OffsetDateTime getLastUpdated() {
if (lastUpdated == null) {
lastUpdated = JsonNullable. undefined();
}
return lastUpdated.orElse(null);
}
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getLastUpdated_JsonNullable() {
return lastUpdated;
}
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
private void setLastUpdated_JsonNullable(JsonNullable lastUpdated) {
this.lastUpdated = lastUpdated;
}
public HookKey name(String name) {
this.name = name;
return this;
}
/**
* Display name of the key
*
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Display name of the key")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HookKey hookKey = (HookKey) o;
return equalsNullable(this.created, hookKey.created) && Objects.equals(this.id, hookKey.id)
&& Objects.equals(this.isUsed, hookKey.isUsed) && Objects.equals(this.keyId, hookKey.keyId)
&& equalsNullable(this.lastUpdated, hookKey.lastUpdated) && Objects.equals(this.name, hookKey.name);
// ;
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(created), id, isUsed, keyId, hashCodeNullable(lastUpdated), name);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HookKey {\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isUsed: ").append(toIndentedString(isUsed)).append("\n");
sb.append(" keyId: ").append(toIndentedString(keyId)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy