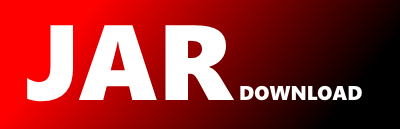
com.okta.sdk.resource.model.IdentityProviderPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.PolicyAccountLink;
import com.okta.sdk.resource.model.PolicySubject;
import com.okta.sdk.resource.model.Provisioning;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Policy settings for the IdP. The following provisioning and account linking actions are supported by each IdP
* provider: | IdP type | User provisioning actions | Group provisioning actions | Account link actions | Account link
* filters | | ----------------------------------------------------------------- | ------------------------- |
* ------------------------------------- | -------------------- | -------------------- | | `SAML2` |
* `AUTO` or `DISABLED` | `NONE`, `ASSIGN`, `APPEND`, or
* `SYNC` | `AUTO`, `DISABLED` | `groups` | | `X509` |
* `DISABLED` | No support for JIT provisioning | | | | All social IdP types (any IdP type that isn't
* `SAML2` or `X509`) | `AUTO`, `DISABLED` | `NONE` or
* `ASSIGN` | `AUTO`, `DISABLED` | `groups` |
*/
@ApiModel(description = "Policy settings for the IdP. The following provisioning and account linking actions are supported by each IdP provider: | IdP type | User provisioning actions | Group provisioning actions | Account link actions | Account link filters | | ----------------------------------------------------------------- | ------------------------- | ------------------------------------- | -------------------- | -------------------- | | `SAML2` | `AUTO` or `DISABLED` | `NONE`, `ASSIGN`, `APPEND`, or `SYNC` | `AUTO`, `DISABLED` | `groups` | | `X509` | `DISABLED` | No support for JIT provisioning | | | | All social IdP types (any IdP type that isn't `SAML2` or `X509`) | `AUTO`, `DISABLED` | `NONE` or `ASSIGN` | `AUTO`, `DISABLED` | `groups` |")
@JsonPropertyOrder({ IdentityProviderPolicy.JSON_PROPERTY_ACCOUNT_LINK,
IdentityProviderPolicy.JSON_PROPERTY_MAX_CLOCK_SKEW, IdentityProviderPolicy.JSON_PROPERTY_PROVISIONING,
IdentityProviderPolicy.JSON_PROPERTY_SUBJECT })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class IdentityProviderPolicy implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACCOUNT_LINK = "accountLink";
private PolicyAccountLink accountLink;
public static final String JSON_PROPERTY_MAX_CLOCK_SKEW = "maxClockSkew";
private Integer maxClockSkew;
public static final String JSON_PROPERTY_PROVISIONING = "provisioning";
private Provisioning provisioning;
public static final String JSON_PROPERTY_SUBJECT = "subject";
private PolicySubject subject;
public IdentityProviderPolicy() {
}
public IdentityProviderPolicy accountLink(PolicyAccountLink accountLink) {
this.accountLink = accountLink;
return this;
}
/**
* Get accountLink
*
* @return accountLink
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACCOUNT_LINK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PolicyAccountLink getAccountLink() {
return accountLink;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_LINK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountLink(PolicyAccountLink accountLink) {
this.accountLink = accountLink;
}
public IdentityProviderPolicy maxClockSkew(Integer maxClockSkew) {
this.maxClockSkew = maxClockSkew;
return this;
}
/**
* Maximum allowable clock skew when processing messages from the IdP
*
* @return maxClockSkew
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "120000", value = "Maximum allowable clock skew when processing messages from the IdP")
@JsonProperty(JSON_PROPERTY_MAX_CLOCK_SKEW)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxClockSkew() {
return maxClockSkew;
}
@JsonProperty(JSON_PROPERTY_MAX_CLOCK_SKEW)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxClockSkew(Integer maxClockSkew) {
this.maxClockSkew = maxClockSkew;
}
public IdentityProviderPolicy provisioning(Provisioning provisioning) {
this.provisioning = provisioning;
return this;
}
/**
* Get provisioning
*
* @return provisioning
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PROVISIONING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Provisioning getProvisioning() {
return provisioning;
}
@JsonProperty(JSON_PROPERTY_PROVISIONING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProvisioning(Provisioning provisioning) {
this.provisioning = provisioning;
}
public IdentityProviderPolicy subject(PolicySubject subject) {
this.subject = subject;
return this;
}
/**
* Get subject
*
* @return subject
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PolicySubject getSubject() {
return subject;
}
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSubject(PolicySubject subject) {
this.subject = subject;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IdentityProviderPolicy identityProviderPolicy = (IdentityProviderPolicy) o;
return Objects.equals(this.accountLink, identityProviderPolicy.accountLink)
&& Objects.equals(this.maxClockSkew, identityProviderPolicy.maxClockSkew)
&& Objects.equals(this.provisioning, identityProviderPolicy.provisioning)
&& Objects.equals(this.subject, identityProviderPolicy.subject);
// ;
}
@Override
public int hashCode() {
return Objects.hash(accountLink, maxClockSkew, provisioning, subject);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class IdentityProviderPolicy {\n");
sb.append(" accountLink: ").append(toIndentedString(accountLink)).append("\n");
sb.append(" maxClockSkew: ").append(toIndentedString(maxClockSkew)).append("\n");
sb.append(" provisioning: ").append(toIndentedString(provisioning)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy