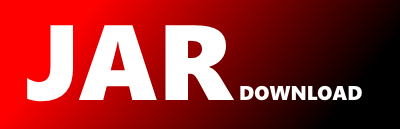
com.okta.sdk.resource.model.LogAuthenticationContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.LogAuthenticationProvider;
import com.okta.sdk.resource.model.LogCredentialProvider;
import com.okta.sdk.resource.model.LogCredentialType;
import com.okta.sdk.resource.model.LogIssuer;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* All authentication relies on validating one or more credentials that prove the authenticity of the actor's
* identity. Credentials are sometimes provided by the actor, as is the case with passwords, and at other times provided
* by a third party, and validated by the authentication provider. The authenticationContext contains metadata about how
* the actor is authenticated. For example, an authenticationContext for an event, where a user authenticates with
* Integrated Windows Authentication (IWA), looks like the following: ``` {
* \"authenticationProvider\": \"ACTIVE_DIRECTORY\", \"authenticationStep\": 0,
* \"credentialProvider\": null, \"credentialType\": \"IWA\",
* \"externalSessionId\": \"102N1EKyPFERROGvK9wizMAPQ\", \"interface\": null,
* \"issuer\": null } ``` In this case, the user enters an IWA credential to authenticate
* against an Active Directory instance. All of the user's future-generated events in this sign-in session are going
* to share the same `externalSessionId`. Among other operations, this response object can be used to scan for
* suspicious sign-in activity or perform analytics on user authentication habits (for example, how often authentication
* scheme X is used versus authentication scheme Y).
*/
@ApiModel(description = "All authentication relies on validating one or more credentials that prove the authenticity of the actor's identity. Credentials are sometimes provided by the actor, as is the case with passwords, and at other times provided by a third party, and validated by the authentication provider. The authenticationContext contains metadata about how the actor is authenticated. For example, an authenticationContext for an event, where a user authenticates with Integrated Windows Authentication (IWA), looks like the following: ``` { \"authenticationProvider\": \"ACTIVE_DIRECTORY\", \"authenticationStep\": 0, \"credentialProvider\": null, \"credentialType\": \"IWA\", \"externalSessionId\": \"102N1EKyPFERROGvK9wizMAPQ\", \"interface\": null, \"issuer\": null } ``` In this case, the user enters an IWA credential to authenticate against an Active Directory instance. All of the user's future-generated events in this sign-in session are going to share the same `externalSessionId`. Among other operations, this response object can be used to scan for suspicious sign-in activity or perform analytics on user authentication habits (for example, how often authentication scheme X is used versus authentication scheme Y).")
@JsonPropertyOrder({ LogAuthenticationContext.JSON_PROPERTY_AUTHENTICATION_PROVIDER,
LogAuthenticationContext.JSON_PROPERTY_AUTHENTICATION_STEP,
LogAuthenticationContext.JSON_PROPERTY_CREDENTIAL_PROVIDER,
LogAuthenticationContext.JSON_PROPERTY_CREDENTIAL_TYPE,
LogAuthenticationContext.JSON_PROPERTY_EXTERNAL_SESSION_ID, LogAuthenticationContext.JSON_PROPERTY_INTERFACE,
LogAuthenticationContext.JSON_PROPERTY_ISSUER })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class LogAuthenticationContext implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AUTHENTICATION_PROVIDER = "authenticationProvider";
private LogAuthenticationProvider authenticationProvider;
public static final String JSON_PROPERTY_AUTHENTICATION_STEP = "authenticationStep";
private Integer authenticationStep;
public static final String JSON_PROPERTY_CREDENTIAL_PROVIDER = "credentialProvider";
private LogCredentialProvider credentialProvider;
public static final String JSON_PROPERTY_CREDENTIAL_TYPE = "credentialType";
private LogCredentialType credentialType;
public static final String JSON_PROPERTY_EXTERNAL_SESSION_ID = "externalSessionId";
private String externalSessionId;
public static final String JSON_PROPERTY_INTERFACE = "interface";
private String _interface;
public static final String JSON_PROPERTY_ISSUER = "issuer";
private LogIssuer issuer;
public LogAuthenticationContext() {
}
/*
* @JsonCreator public LogAuthenticationContext(
*
* @JsonProperty(JSON_PROPERTY_AUTHENTICATION_STEP) Integer authenticationStep,
*
* @JsonProperty(JSON_PROPERTY_EXTERNAL_SESSION_ID) String externalSessionId,
*
* @JsonProperty(JSON_PROPERTY_INTERFACE) String _interface ) { this(); this.authenticationStep =
* authenticationStep; this.externalSessionId = externalSessionId; this._interface = _interface; }
*/
public LogAuthenticationContext authenticationProvider(LogAuthenticationProvider authenticationProvider) {
this.authenticationProvider = authenticationProvider;
return this;
}
/**
* Get authenticationProvider
*
* @return authenticationProvider
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AUTHENTICATION_PROVIDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogAuthenticationProvider getAuthenticationProvider() {
return authenticationProvider;
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATION_PROVIDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthenticationProvider(LogAuthenticationProvider authenticationProvider) {
this.authenticationProvider = authenticationProvider;
}
/**
* The zero-based step number in the authentication pipeline. Currently unused and always set to `0`.
*
* @return authenticationStep
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The zero-based step number in the authentication pipeline. Currently unused and always set to `0`.")
@JsonProperty(JSON_PROPERTY_AUTHENTICATION_STEP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getAuthenticationStep() {
return authenticationStep;
}
public LogAuthenticationContext credentialProvider(LogCredentialProvider credentialProvider) {
this.credentialProvider = credentialProvider;
return this;
}
/**
* Get credentialProvider
*
* @return credentialProvider
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREDENTIAL_PROVIDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogCredentialProvider getCredentialProvider() {
return credentialProvider;
}
@JsonProperty(JSON_PROPERTY_CREDENTIAL_PROVIDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCredentialProvider(LogCredentialProvider credentialProvider) {
this.credentialProvider = credentialProvider;
}
public LogAuthenticationContext credentialType(LogCredentialType credentialType) {
this.credentialType = credentialType;
return this;
}
/**
* Get credentialType
*
* @return credentialType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREDENTIAL_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogCredentialType getCredentialType() {
return credentialType;
}
@JsonProperty(JSON_PROPERTY_CREDENTIAL_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCredentialType(LogCredentialType credentialType) {
this.credentialType = credentialType;
}
/**
* A proxy for the actor's [session
* ID](https://cheatsheetseries.owasp.org/cheatsheets/Session_Management_Cheat_Sheet.html)
*
* @return externalSessionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A proxy for the actor's [session ID](https://cheatsheetseries.owasp.org/cheatsheets/Session_Management_Cheat_Sheet.html)")
@JsonProperty(JSON_PROPERTY_EXTERNAL_SESSION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalSessionId() {
return externalSessionId;
}
/**
* The third-party user interface that the actor authenticates through, if any.
*
* @return _interface
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The third-party user interface that the actor authenticates through, if any.")
@JsonProperty(JSON_PROPERTY_INTERFACE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getInterface() {
return _interface;
}
public LogAuthenticationContext issuer(LogIssuer issuer) {
this.issuer = issuer;
return this;
}
/**
* Get issuer
*
* @return issuer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ISSUER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogIssuer getIssuer() {
return issuer;
}
@JsonProperty(JSON_PROPERTY_ISSUER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIssuer(LogIssuer issuer) {
this.issuer = issuer;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LogAuthenticationContext logAuthenticationContext = (LogAuthenticationContext) o;
return Objects.equals(this.authenticationProvider, logAuthenticationContext.authenticationProvider)
&& Objects.equals(this.authenticationStep, logAuthenticationContext.authenticationStep)
&& Objects.equals(this.credentialProvider, logAuthenticationContext.credentialProvider)
&& Objects.equals(this.credentialType, logAuthenticationContext.credentialType)
&& Objects.equals(this.externalSessionId, logAuthenticationContext.externalSessionId)
&& Objects.equals(this._interface, logAuthenticationContext._interface)
&& Objects.equals(this.issuer, logAuthenticationContext.issuer);
// ;
}
@Override
public int hashCode() {
return Objects.hash(authenticationProvider, authenticationStep, credentialProvider, credentialType,
externalSessionId, _interface, issuer);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LogAuthenticationContext {\n");
sb.append(" authenticationProvider: ").append(toIndentedString(authenticationProvider)).append("\n");
sb.append(" authenticationStep: ").append(toIndentedString(authenticationStep)).append("\n");
sb.append(" credentialProvider: ").append(toIndentedString(credentialProvider)).append("\n");
sb.append(" credentialType: ").append(toIndentedString(credentialType)).append("\n");
sb.append(" externalSessionId: ").append(toIndentedString(externalSessionId)).append("\n");
sb.append(" _interface: ").append(toIndentedString(_interface)).append("\n");
sb.append(" issuer: ").append(toIndentedString(issuer)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy