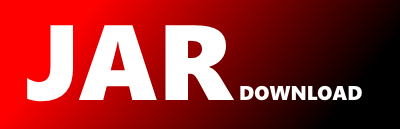
com.okta.sdk.resource.model.LogDebugContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.HashMap;
import java.util.Map;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* For some kinds of events (for example, OLM provisioning, sign-in request, second factor SMS, and so on), the fields
* that are provided in other response objects aren't sufficient to adequately describe the operations that the
* event has performed. In such cases, the `debugContext` object provides a way to store additional
* information. For example, an event where a second factor SMS token is sent to a user may have a
* `debugContext` that looks like the following: ``` { \"debugData\": {
* \"requestUri\": \"/api/v1/users/00u3gjksoiRGRAZHLSYV/factors/smsf8luacpZJAva10x45/verify\",
* \"smsProvider\": \"TELESIGN\", \"transactionId\":
* \"268632458E3C100F5F5F594C6DC689D4\" } } ``` By inspecting the debugData field, you can find
* the URI that is used to trigger the second factor SMS
* (`/api/v1/users/00u3gjksoiRGRAZHLSYV/factors/smsf8luacpZJAva10x45/verify`), the SMS provider
* (`TELESIGN`), and the ID used by Telesign to identify this transaction
* (`268632458E3C100F5F5F594C6DC689D4`). If for some reason the information that is needed to implement a
* feature isn't provided in other response objects, you should scan the `debugContext.debugData` field
* for potentially useful fields. > **Important:** The information contained in `debugContext.debugData` is
* intended to add context when troubleshooting customer platform issues. Both key names and values may change from
* release to release and aren't guaranteed to be stable. Therefore, they shouldn't be viewed as a data contract
* but as a debugging aid instead.
*/
@ApiModel(description = "For some kinds of events (for example, OLM provisioning, sign-in request, second factor SMS, and so on), the fields that are provided in other response objects aren't sufficient to adequately describe the operations that the event has performed. In such cases, the `debugContext` object provides a way to store additional information. For example, an event where a second factor SMS token is sent to a user may have a `debugContext` that looks like the following: ``` { \"debugData\": { \"requestUri\": \"/api/v1/users/00u3gjksoiRGRAZHLSYV/factors/smsf8luacpZJAva10x45/verify\", \"smsProvider\": \"TELESIGN\", \"transactionId\": \"268632458E3C100F5F5F594C6DC689D4\" } } ``` By inspecting the debugData field, you can find the URI that is used to trigger the second factor SMS (`/api/v1/users/00u3gjksoiRGRAZHLSYV/factors/smsf8luacpZJAva10x45/verify`), the SMS provider (`TELESIGN`), and the ID used by Telesign to identify this transaction (`268632458E3C100F5F5F594C6DC689D4`). If for some reason the information that is needed to implement a feature isn't provided in other response objects, you should scan the `debugContext.debugData` field for potentially useful fields. > **Important:** The information contained in `debugContext.debugData` is intended to add context when troubleshooting customer platform issues. Both key names and values may change from release to release and aren't guaranteed to be stable. Therefore, they shouldn't be viewed as a data contract but as a debugging aid instead.")
@JsonPropertyOrder({ LogDebugContext.JSON_PROPERTY_DEBUG_DATA })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class LogDebugContext implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_DEBUG_DATA = "debugData";
private Map debugData = null;
public LogDebugContext() {
}
/*
* @JsonCreator public LogDebugContext(
*
* @JsonProperty(JSON_PROPERTY_DEBUG_DATA) Map debugData ) { this(); this.debugData = debugData; }
*/
/**
* A dynamic field that contains miscellaneous information that is dependent on the event type.
*
* @return debugData
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A dynamic field that contains miscellaneous information that is dependent on the event type.")
@JsonProperty(JSON_PROPERTY_DEBUG_DATA)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getDebugData() {
return debugData;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LogDebugContext logDebugContext = (LogDebugContext) o;
return Objects.equals(this.debugData, logDebugContext.debugData);
// ;
}
@Override
public int hashCode() {
return Objects.hash(debugData);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LogDebugContext {\n");
sb.append(" debugData: ").append(toIndentedString(debugData)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy