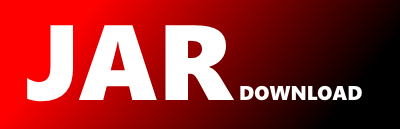
com.okta.sdk.resource.model.LogEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.LogActor;
import com.okta.sdk.resource.model.LogAuthenticationContext;
import com.okta.sdk.resource.model.LogClient;
import com.okta.sdk.resource.model.LogDebugContext;
import com.okta.sdk.resource.model.LogOutcome;
import com.okta.sdk.resource.model.LogRequest;
import com.okta.sdk.resource.model.LogSecurityContext;
import com.okta.sdk.resource.model.LogSeverity;
import com.okta.sdk.resource.model.LogTarget;
import com.okta.sdk.resource.model.LogTransaction;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* LogEvent
*/
@JsonPropertyOrder({ LogEvent.JSON_PROPERTY_ACTOR, LogEvent.JSON_PROPERTY_AUTHENTICATION_CONTEXT,
LogEvent.JSON_PROPERTY_CLIENT, LogEvent.JSON_PROPERTY_DEBUG_CONTEXT, LogEvent.JSON_PROPERTY_DISPLAY_MESSAGE,
LogEvent.JSON_PROPERTY_EVENT_TYPE, LogEvent.JSON_PROPERTY_LEGACY_EVENT_TYPE, LogEvent.JSON_PROPERTY_OUTCOME,
LogEvent.JSON_PROPERTY_PUBLISHED, LogEvent.JSON_PROPERTY_REQUEST, LogEvent.JSON_PROPERTY_SECURITY_CONTEXT,
LogEvent.JSON_PROPERTY_SEVERITY, LogEvent.JSON_PROPERTY_TARGET, LogEvent.JSON_PROPERTY_TRANSACTION,
LogEvent.JSON_PROPERTY_UUID, LogEvent.JSON_PROPERTY_VERSION })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class LogEvent implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACTOR = "actor";
private LogActor actor;
public static final String JSON_PROPERTY_AUTHENTICATION_CONTEXT = "authenticationContext";
private LogAuthenticationContext authenticationContext;
public static final String JSON_PROPERTY_CLIENT = "client";
private LogClient client;
public static final String JSON_PROPERTY_DEBUG_CONTEXT = "debugContext";
private LogDebugContext debugContext;
public static final String JSON_PROPERTY_DISPLAY_MESSAGE = "displayMessage";
private String displayMessage;
public static final String JSON_PROPERTY_EVENT_TYPE = "eventType";
private String eventType;
public static final String JSON_PROPERTY_LEGACY_EVENT_TYPE = "legacyEventType";
private String legacyEventType;
public static final String JSON_PROPERTY_OUTCOME = "outcome";
private LogOutcome outcome;
public static final String JSON_PROPERTY_PUBLISHED = "published";
private OffsetDateTime published;
public static final String JSON_PROPERTY_REQUEST = "request";
private LogRequest request;
public static final String JSON_PROPERTY_SECURITY_CONTEXT = "securityContext";
private LogSecurityContext securityContext;
public static final String JSON_PROPERTY_SEVERITY = "severity";
private LogSeverity severity;
public static final String JSON_PROPERTY_TARGET = "target";
private List target = null;
public static final String JSON_PROPERTY_TRANSACTION = "transaction";
private LogTransaction transaction;
public static final String JSON_PROPERTY_UUID = "uuid";
private String uuid;
public static final String JSON_PROPERTY_VERSION = "version";
private String version;
public LogEvent() {
}
/*
* @JsonCreator public LogEvent(
*
* @JsonProperty(JSON_PROPERTY_DISPLAY_MESSAGE) String displayMessage,
*
* @JsonProperty(JSON_PROPERTY_EVENT_TYPE) String eventType,
*
* @JsonProperty(JSON_PROPERTY_LEGACY_EVENT_TYPE) String legacyEventType,
*
* @JsonProperty(JSON_PROPERTY_PUBLISHED) OffsetDateTime published,
*
* @JsonProperty(JSON_PROPERTY_TARGET) List target,
*
* @JsonProperty(JSON_PROPERTY_UUID) String uuid,
*
* @JsonProperty(JSON_PROPERTY_VERSION) String version ) { this(); this.displayMessage = displayMessage;
* this.eventType = eventType; this.legacyEventType = legacyEventType; this.published = published; this.target =
* target; this.uuid = uuid; this.version = version; }
*/
public LogEvent actor(LogActor actor) {
this.actor = actor;
return this;
}
/**
* Get actor
*
* @return actor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogActor getActor() {
return actor;
}
@JsonProperty(JSON_PROPERTY_ACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActor(LogActor actor) {
this.actor = actor;
}
public LogEvent authenticationContext(LogAuthenticationContext authenticationContext) {
this.authenticationContext = authenticationContext;
return this;
}
/**
* Get authenticationContext
*
* @return authenticationContext
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AUTHENTICATION_CONTEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogAuthenticationContext getAuthenticationContext() {
return authenticationContext;
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATION_CONTEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthenticationContext(LogAuthenticationContext authenticationContext) {
this.authenticationContext = authenticationContext;
}
public LogEvent client(LogClient client) {
this.client = client;
return this;
}
/**
* Get client
*
* @return client
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CLIENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogClient getClient() {
return client;
}
@JsonProperty(JSON_PROPERTY_CLIENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setClient(LogClient client) {
this.client = client;
}
public LogEvent debugContext(LogDebugContext debugContext) {
this.debugContext = debugContext;
return this;
}
/**
* Get debugContext
*
* @return debugContext
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DEBUG_CONTEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogDebugContext getDebugContext() {
return debugContext;
}
@JsonProperty(JSON_PROPERTY_DEBUG_CONTEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDebugContext(LogDebugContext debugContext) {
this.debugContext = debugContext;
}
/**
* The display message for an event
*
* @return displayMessage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The display message for an event")
@JsonProperty(JSON_PROPERTY_DISPLAY_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDisplayMessage() {
return displayMessage;
}
/**
* Type of event that is published
*
* @return eventType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Type of event that is published")
@JsonProperty(JSON_PROPERTY_EVENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEventType() {
return eventType;
}
/**
* Associated Events API Action `objectType` attribute value
*
* @return legacyEventType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Associated Events API Action `objectType` attribute value")
@JsonProperty(JSON_PROPERTY_LEGACY_EVENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLegacyEventType() {
return legacyEventType;
}
public LogEvent outcome(LogOutcome outcome) {
this.outcome = outcome;
return this;
}
/**
* Get outcome
*
* @return outcome
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_OUTCOME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogOutcome getOutcome() {
return outcome;
}
@JsonProperty(JSON_PROPERTY_OUTCOME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOutcome(LogOutcome outcome) {
this.outcome = outcome;
}
/**
* Timestamp when the event is published
*
* @return published
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Timestamp when the event is published")
@JsonProperty(JSON_PROPERTY_PUBLISHED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getPublished() {
return published;
}
public LogEvent request(LogRequest request) {
this.request = request;
return this;
}
/**
* Get request
*
* @return request
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REQUEST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogRequest getRequest() {
return request;
}
@JsonProperty(JSON_PROPERTY_REQUEST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRequest(LogRequest request) {
this.request = request;
}
public LogEvent securityContext(LogSecurityContext securityContext) {
this.securityContext = securityContext;
return this;
}
/**
* Get securityContext
*
* @return securityContext
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SECURITY_CONTEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogSecurityContext getSecurityContext() {
return securityContext;
}
@JsonProperty(JSON_PROPERTY_SECURITY_CONTEXT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSecurityContext(LogSecurityContext securityContext) {
this.securityContext = securityContext;
}
public LogEvent severity(LogSeverity severity) {
this.severity = severity;
return this;
}
/**
* Get severity
*
* @return severity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SEVERITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogSeverity getSeverity() {
return severity;
}
@JsonProperty(JSON_PROPERTY_SEVERITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSeverity(LogSeverity severity) {
this.severity = severity;
}
/**
* Get target
*
* @return target
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TARGET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTarget() {
return target;
}
public LogEvent transaction(LogTransaction transaction) {
this.transaction = transaction;
return this;
}
/**
* Get transaction
*
* @return transaction
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TRANSACTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LogTransaction getTransaction() {
return transaction;
}
@JsonProperty(JSON_PROPERTY_TRANSACTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTransaction(LogTransaction transaction) {
this.transaction = transaction;
}
/**
* Unique identifier for an individual event
*
* @return uuid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for an individual event")
@JsonProperty(JSON_PROPERTY_UUID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUuid() {
return uuid;
}
/**
* Versioning indicator
*
* @return version
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Versioning indicator")
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getVersion() {
return version;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LogEvent logEvent = (LogEvent) o;
return Objects.equals(this.actor, logEvent.actor)
&& Objects.equals(this.authenticationContext, logEvent.authenticationContext)
&& Objects.equals(this.client, logEvent.client)
&& Objects.equals(this.debugContext, logEvent.debugContext)
&& Objects.equals(this.displayMessage, logEvent.displayMessage)
&& Objects.equals(this.eventType, logEvent.eventType)
&& Objects.equals(this.legacyEventType, logEvent.legacyEventType)
&& Objects.equals(this.outcome, logEvent.outcome) && Objects.equals(this.published, logEvent.published)
&& Objects.equals(this.request, logEvent.request)
&& Objects.equals(this.securityContext, logEvent.securityContext)
&& Objects.equals(this.severity, logEvent.severity) && Objects.equals(this.target, logEvent.target)
&& Objects.equals(this.transaction, logEvent.transaction) && Objects.equals(this.uuid, logEvent.uuid)
&& Objects.equals(this.version, logEvent.version);
// ;
}
@Override
public int hashCode() {
return Objects.hash(actor, authenticationContext, client, debugContext, displayMessage, eventType,
legacyEventType, outcome, published, request, securityContext, severity, target, transaction, uuid,
version);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LogEvent {\n");
sb.append(" actor: ").append(toIndentedString(actor)).append("\n");
sb.append(" authenticationContext: ").append(toIndentedString(authenticationContext)).append("\n");
sb.append(" client: ").append(toIndentedString(client)).append("\n");
sb.append(" debugContext: ").append(toIndentedString(debugContext)).append("\n");
sb.append(" displayMessage: ").append(toIndentedString(displayMessage)).append("\n");
sb.append(" eventType: ").append(toIndentedString(eventType)).append("\n");
sb.append(" legacyEventType: ").append(toIndentedString(legacyEventType)).append("\n");
sb.append(" outcome: ").append(toIndentedString(outcome)).append("\n");
sb.append(" published: ").append(toIndentedString(published)).append("\n");
sb.append(" request: ").append(toIndentedString(request)).append("\n");
sb.append(" securityContext: ").append(toIndentedString(securityContext)).append("\n");
sb.append(" severity: ").append(toIndentedString(severity)).append("\n");
sb.append(" target: ").append(toIndentedString(target)).append("\n");
sb.append(" transaction: ").append(toIndentedString(transaction)).append("\n");
sb.append(" uuid: ").append(toIndentedString(uuid)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy