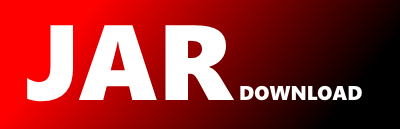
com.okta.sdk.resource.model.OAuthMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.CodeChallengeMethod;
import com.okta.sdk.resource.model.EndpointAuthMethod;
import com.okta.sdk.resource.model.GrantType;
import com.okta.sdk.resource.model.ResponseMode;
import com.okta.sdk.resource.model.ResponseTypesSupported;
import com.okta.sdk.resource.model.SigningAlgorithm;
import com.okta.sdk.resource.model.SubjectType;
import com.okta.sdk.resource.model.TokenDeliveryMode;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* OAuthMetadata
*/
@JsonPropertyOrder({ OAuthMetadata.JSON_PROPERTY_AUTHORIZATION_ENDPOINT,
OAuthMetadata.JSON_PROPERTY_BACKCHANNEL_AUTHENTICATION_REQUEST_SIGNING_ALG_VALUES_SUPPORTED,
OAuthMetadata.JSON_PROPERTY_BACKCHANNEL_TOKEN_DELIVERY_MODES_SUPPORTED,
OAuthMetadata.JSON_PROPERTY_CLAIMS_SUPPORTED, OAuthMetadata.JSON_PROPERTY_CODE_CHALLENGE_METHODS_SUPPORTED,
OAuthMetadata.JSON_PROPERTY_DEVICE_AUTHORIZATION_ENDPOINT,
OAuthMetadata.JSON_PROPERTY_DPOP_SIGNING_ALG_VALUES_SUPPORTED, OAuthMetadata.JSON_PROPERTY_END_SESSION_ENDPOINT,
OAuthMetadata.JSON_PROPERTY_GRANT_TYPES_SUPPORTED, OAuthMetadata.JSON_PROPERTY_INTROSPECTION_ENDPOINT,
OAuthMetadata.JSON_PROPERTY_INTROSPECTION_ENDPOINT_AUTH_METHODS_SUPPORTED, OAuthMetadata.JSON_PROPERTY_ISSUER,
OAuthMetadata.JSON_PROPERTY_JWKS_URI, OAuthMetadata.JSON_PROPERTY_PUSHED_AUTHORIZATION_REQUEST_ENDPOINT,
OAuthMetadata.JSON_PROPERTY_REGISTRATION_ENDPOINT,
OAuthMetadata.JSON_PROPERTY_REQUEST_OBJECT_SIGNING_ALG_VALUES_SUPPORTED,
OAuthMetadata.JSON_PROPERTY_REQUEST_PARAMETER_SUPPORTED, OAuthMetadata.JSON_PROPERTY_RESPONSE_MODES_SUPPORTED,
OAuthMetadata.JSON_PROPERTY_RESPONSE_TYPES_SUPPORTED, OAuthMetadata.JSON_PROPERTY_REVOCATION_ENDPOINT,
OAuthMetadata.JSON_PROPERTY_REVOCATION_ENDPOINT_AUTH_METHODS_SUPPORTED,
OAuthMetadata.JSON_PROPERTY_SCOPES_SUPPORTED, OAuthMetadata.JSON_PROPERTY_SUBJECT_TYPES_SUPPORTED,
OAuthMetadata.JSON_PROPERTY_TOKEN_ENDPOINT, OAuthMetadata.JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHODS_SUPPORTED })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class OAuthMetadata implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AUTHORIZATION_ENDPOINT = "authorization_endpoint";
private String authorizationEndpoint;
public static final String JSON_PROPERTY_BACKCHANNEL_AUTHENTICATION_REQUEST_SIGNING_ALG_VALUES_SUPPORTED = "backchannel_authentication_request_signing_alg_values_supported";
private List backchannelAuthenticationRequestSigningAlgValuesSupported = null;
public static final String JSON_PROPERTY_BACKCHANNEL_TOKEN_DELIVERY_MODES_SUPPORTED = "backchannel_token_delivery_modes_supported";
private List backchannelTokenDeliveryModesSupported = null;
public static final String JSON_PROPERTY_CLAIMS_SUPPORTED = "claims_supported";
private List claimsSupported = null;
public static final String JSON_PROPERTY_CODE_CHALLENGE_METHODS_SUPPORTED = "code_challenge_methods_supported";
private List codeChallengeMethodsSupported = null;
public static final String JSON_PROPERTY_DEVICE_AUTHORIZATION_ENDPOINT = "device_authorization_endpoint";
private String deviceAuthorizationEndpoint;
/**
* Gets or Sets dpopSigningAlgValuesSupported
*/
public enum DpopSigningAlgValuesSupportedEnum {
ES256("ES256"),
ES384("ES384"),
ES512("ES512"),
RS256("RS256"),
RS384("RS384"),
RS512("RS512"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
DpopSigningAlgValuesSupportedEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static DpopSigningAlgValuesSupportedEnum fromValue(String value) {
for (DpopSigningAlgValuesSupportedEnum b : DpopSigningAlgValuesSupportedEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_DPOP_SIGNING_ALG_VALUES_SUPPORTED = "dpop_signing_alg_values_supported";
private List dpopSigningAlgValuesSupported = null;
public static final String JSON_PROPERTY_END_SESSION_ENDPOINT = "end_session_endpoint";
private String endSessionEndpoint;
public static final String JSON_PROPERTY_GRANT_TYPES_SUPPORTED = "grant_types_supported";
private List grantTypesSupported = null;
public static final String JSON_PROPERTY_INTROSPECTION_ENDPOINT = "introspection_endpoint";
private String introspectionEndpoint;
public static final String JSON_PROPERTY_INTROSPECTION_ENDPOINT_AUTH_METHODS_SUPPORTED = "introspection_endpoint_auth_methods_supported";
private List introspectionEndpointAuthMethodsSupported = null;
public static final String JSON_PROPERTY_ISSUER = "issuer";
private String issuer;
public static final String JSON_PROPERTY_JWKS_URI = "jwks_uri";
private String jwksUri;
public static final String JSON_PROPERTY_PUSHED_AUTHORIZATION_REQUEST_ENDPOINT = "pushed_authorization_request_endpoint";
private String pushedAuthorizationRequestEndpoint;
public static final String JSON_PROPERTY_REGISTRATION_ENDPOINT = "registration_endpoint";
private String registrationEndpoint;
public static final String JSON_PROPERTY_REQUEST_OBJECT_SIGNING_ALG_VALUES_SUPPORTED = "request_object_signing_alg_values_supported";
private List requestObjectSigningAlgValuesSupported = null;
public static final String JSON_PROPERTY_REQUEST_PARAMETER_SUPPORTED = "request_parameter_supported";
private Boolean requestParameterSupported;
public static final String JSON_PROPERTY_RESPONSE_MODES_SUPPORTED = "response_modes_supported";
private List responseModesSupported = null;
public static final String JSON_PROPERTY_RESPONSE_TYPES_SUPPORTED = "response_types_supported";
private List responseTypesSupported = null;
public static final String JSON_PROPERTY_REVOCATION_ENDPOINT = "revocation_endpoint";
private String revocationEndpoint;
public static final String JSON_PROPERTY_REVOCATION_ENDPOINT_AUTH_METHODS_SUPPORTED = "revocation_endpoint_auth_methods_supported";
private List revocationEndpointAuthMethodsSupported = null;
public static final String JSON_PROPERTY_SCOPES_SUPPORTED = "scopes_supported";
private List scopesSupported = null;
public static final String JSON_PROPERTY_SUBJECT_TYPES_SUPPORTED = "subject_types_supported";
private List subjectTypesSupported = null;
public static final String JSON_PROPERTY_TOKEN_ENDPOINT = "token_endpoint";
private String tokenEndpoint;
public static final String JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHODS_SUPPORTED = "token_endpoint_auth_methods_supported";
private List tokenEndpointAuthMethodsSupported = null;
public OAuthMetadata() {
}
public OAuthMetadata authorizationEndpoint(String authorizationEndpoint) {
this.authorizationEndpoint = authorizationEndpoint;
return this;
}
/**
* URL of the authorization server's authorization endpoint.
*
* @return authorizationEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL of the authorization server's authorization endpoint.")
@JsonProperty(JSON_PROPERTY_AUTHORIZATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuthorizationEndpoint() {
return authorizationEndpoint;
}
@JsonProperty(JSON_PROPERTY_AUTHORIZATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthorizationEndpoint(String authorizationEndpoint) {
this.authorizationEndpoint = authorizationEndpoint;
}
public OAuthMetadata backchannelAuthenticationRequestSigningAlgValuesSupported(
List backchannelAuthenticationRequestSigningAlgValuesSupported) {
this.backchannelAuthenticationRequestSigningAlgValuesSupported = backchannelAuthenticationRequestSigningAlgValuesSupported;
return this;
}
public OAuthMetadata addbackchannelAuthenticationRequestSigningAlgValuesSupportedItem(
SigningAlgorithm backchannelAuthenticationRequestSigningAlgValuesSupportedItem) {
if (this.backchannelAuthenticationRequestSigningAlgValuesSupported == null) {
this.backchannelAuthenticationRequestSigningAlgValuesSupported = new ArrayList<>();
}
this.backchannelAuthenticationRequestSigningAlgValuesSupported
.add(backchannelAuthenticationRequestSigningAlgValuesSupportedItem);
return this;
}
/**
* <div class=\"x-lifecycle-container\"><x-lifecycle
* class=\"lea\"></x-lifecycle> <x-lifecycle
* class=\"oie\"></x-lifecycle></div>A list of signing algorithms that this
* authorization server supports for signed requests.
*
* @return backchannelAuthenticationRequestSigningAlgValuesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " A list of signing algorithms that this authorization server supports for signed requests.")
@JsonProperty(JSON_PROPERTY_BACKCHANNEL_AUTHENTICATION_REQUEST_SIGNING_ALG_VALUES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getBackchannelAuthenticationRequestSigningAlgValuesSupported() {
return backchannelAuthenticationRequestSigningAlgValuesSupported;
}
@JsonProperty(JSON_PROPERTY_BACKCHANNEL_AUTHENTICATION_REQUEST_SIGNING_ALG_VALUES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBackchannelAuthenticationRequestSigningAlgValuesSupported(
List backchannelAuthenticationRequestSigningAlgValuesSupported) {
this.backchannelAuthenticationRequestSigningAlgValuesSupported = backchannelAuthenticationRequestSigningAlgValuesSupported;
}
public OAuthMetadata backchannelTokenDeliveryModesSupported(
List backchannelTokenDeliveryModesSupported) {
this.backchannelTokenDeliveryModesSupported = backchannelTokenDeliveryModesSupported;
return this;
}
public OAuthMetadata addbackchannelTokenDeliveryModesSupportedItem(
TokenDeliveryMode backchannelTokenDeliveryModesSupportedItem) {
if (this.backchannelTokenDeliveryModesSupported == null) {
this.backchannelTokenDeliveryModesSupported = new ArrayList<>();
}
this.backchannelTokenDeliveryModesSupported.add(backchannelTokenDeliveryModesSupportedItem);
return this;
}
/**
* <div class=\"x-lifecycle-container\"><x-lifecycle
* class=\"lea\"></x-lifecycle> <x-lifecycle
* class=\"oie\"></x-lifecycle></div>The delivery modes that this authorization server
* supports for Client-Initiated Backchannel Authentication.
*
* @return backchannelTokenDeliveryModesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " The delivery modes that this authorization server supports for Client-Initiated Backchannel Authentication.")
@JsonProperty(JSON_PROPERTY_BACKCHANNEL_TOKEN_DELIVERY_MODES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getBackchannelTokenDeliveryModesSupported() {
return backchannelTokenDeliveryModesSupported;
}
@JsonProperty(JSON_PROPERTY_BACKCHANNEL_TOKEN_DELIVERY_MODES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBackchannelTokenDeliveryModesSupported(
List backchannelTokenDeliveryModesSupported) {
this.backchannelTokenDeliveryModesSupported = backchannelTokenDeliveryModesSupported;
}
public OAuthMetadata claimsSupported(List claimsSupported) {
this.claimsSupported = claimsSupported;
return this;
}
public OAuthMetadata addclaimsSupportedItem(String claimsSupportedItem) {
if (this.claimsSupported == null) {
this.claimsSupported = new ArrayList<>();
}
this.claimsSupported.add(claimsSupportedItem);
return this;
}
/**
* A list of the claims supported by this authorization server.
*
* @return claimsSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of the claims supported by this authorization server.")
@JsonProperty(JSON_PROPERTY_CLAIMS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getClaimsSupported() {
return claimsSupported;
}
@JsonProperty(JSON_PROPERTY_CLAIMS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setClaimsSupported(List claimsSupported) {
this.claimsSupported = claimsSupported;
}
public OAuthMetadata codeChallengeMethodsSupported(List codeChallengeMethodsSupported) {
this.codeChallengeMethodsSupported = codeChallengeMethodsSupported;
return this;
}
public OAuthMetadata addcodeChallengeMethodsSupportedItem(CodeChallengeMethod codeChallengeMethodsSupportedItem) {
if (this.codeChallengeMethodsSupported == null) {
this.codeChallengeMethodsSupported = new ArrayList<>();
}
this.codeChallengeMethodsSupported.add(codeChallengeMethodsSupportedItem);
return this;
}
/**
* A list of PKCE code challenge methods supported by this authorization server.
*
* @return codeChallengeMethodsSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of PKCE code challenge methods supported by this authorization server.")
@JsonProperty(JSON_PROPERTY_CODE_CHALLENGE_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCodeChallengeMethodsSupported() {
return codeChallengeMethodsSupported;
}
@JsonProperty(JSON_PROPERTY_CODE_CHALLENGE_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCodeChallengeMethodsSupported(List codeChallengeMethodsSupported) {
this.codeChallengeMethodsSupported = codeChallengeMethodsSupported;
}
public OAuthMetadata deviceAuthorizationEndpoint(String deviceAuthorizationEndpoint) {
this.deviceAuthorizationEndpoint = deviceAuthorizationEndpoint;
return this;
}
/**
* Get deviceAuthorizationEndpoint
*
* @return deviceAuthorizationEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DEVICE_AUTHORIZATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDeviceAuthorizationEndpoint() {
return deviceAuthorizationEndpoint;
}
@JsonProperty(JSON_PROPERTY_DEVICE_AUTHORIZATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeviceAuthorizationEndpoint(String deviceAuthorizationEndpoint) {
this.deviceAuthorizationEndpoint = deviceAuthorizationEndpoint;
}
public OAuthMetadata dpopSigningAlgValuesSupported(
List dpopSigningAlgValuesSupported) {
this.dpopSigningAlgValuesSupported = dpopSigningAlgValuesSupported;
return this;
}
public OAuthMetadata adddpopSigningAlgValuesSupportedItem(
DpopSigningAlgValuesSupportedEnum dpopSigningAlgValuesSupportedItem) {
if (this.dpopSigningAlgValuesSupported == null) {
this.dpopSigningAlgValuesSupported = new ArrayList<>();
}
this.dpopSigningAlgValuesSupported.add(dpopSigningAlgValuesSupportedItem);
return this;
}
/**
* A list of signing algorithms supported by this authorization server for Demonstrating Proof-of-Possession (DPoP)
* JWTs.
*
* @return dpopSigningAlgValuesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of signing algorithms supported by this authorization server for Demonstrating Proof-of-Possession (DPoP) JWTs.")
@JsonProperty(JSON_PROPERTY_DPOP_SIGNING_ALG_VALUES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getDpopSigningAlgValuesSupported() {
return dpopSigningAlgValuesSupported;
}
@JsonProperty(JSON_PROPERTY_DPOP_SIGNING_ALG_VALUES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDpopSigningAlgValuesSupported(
List dpopSigningAlgValuesSupported) {
this.dpopSigningAlgValuesSupported = dpopSigningAlgValuesSupported;
}
public OAuthMetadata endSessionEndpoint(String endSessionEndpoint) {
this.endSessionEndpoint = endSessionEndpoint;
return this;
}
/**
* URL of the authorization server's logout endpoint.
*
* @return endSessionEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL of the authorization server's logout endpoint.")
@JsonProperty(JSON_PROPERTY_END_SESSION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEndSessionEndpoint() {
return endSessionEndpoint;
}
@JsonProperty(JSON_PROPERTY_END_SESSION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEndSessionEndpoint(String endSessionEndpoint) {
this.endSessionEndpoint = endSessionEndpoint;
}
public OAuthMetadata grantTypesSupported(List grantTypesSupported) {
this.grantTypesSupported = grantTypesSupported;
return this;
}
public OAuthMetadata addgrantTypesSupportedItem(GrantType grantTypesSupportedItem) {
if (this.grantTypesSupported == null) {
this.grantTypesSupported = new ArrayList<>();
}
this.grantTypesSupported.add(grantTypesSupportedItem);
return this;
}
/**
* A list of the grant type values that this authorization server supports.
*
* @return grantTypesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of the grant type values that this authorization server supports.")
@JsonProperty(JSON_PROPERTY_GRANT_TYPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getGrantTypesSupported() {
return grantTypesSupported;
}
@JsonProperty(JSON_PROPERTY_GRANT_TYPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGrantTypesSupported(List grantTypesSupported) {
this.grantTypesSupported = grantTypesSupported;
}
public OAuthMetadata introspectionEndpoint(String introspectionEndpoint) {
this.introspectionEndpoint = introspectionEndpoint;
return this;
}
/**
* URL of the authorization server's introspection endpoint.
*
* @return introspectionEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL of the authorization server's introspection endpoint.")
@JsonProperty(JSON_PROPERTY_INTROSPECTION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIntrospectionEndpoint() {
return introspectionEndpoint;
}
@JsonProperty(JSON_PROPERTY_INTROSPECTION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIntrospectionEndpoint(String introspectionEndpoint) {
this.introspectionEndpoint = introspectionEndpoint;
}
public OAuthMetadata introspectionEndpointAuthMethodsSupported(
List introspectionEndpointAuthMethodsSupported) {
this.introspectionEndpointAuthMethodsSupported = introspectionEndpointAuthMethodsSupported;
return this;
}
public OAuthMetadata addintrospectionEndpointAuthMethodsSupportedItem(
EndpointAuthMethod introspectionEndpointAuthMethodsSupportedItem) {
if (this.introspectionEndpointAuthMethodsSupported == null) {
this.introspectionEndpointAuthMethodsSupported = new ArrayList<>();
}
this.introspectionEndpointAuthMethodsSupported.add(introspectionEndpointAuthMethodsSupportedItem);
return this;
}
/**
* A list of client authentication methods supported by this introspection endpoint.
*
* @return introspectionEndpointAuthMethodsSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of client authentication methods supported by this introspection endpoint.")
@JsonProperty(JSON_PROPERTY_INTROSPECTION_ENDPOINT_AUTH_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getIntrospectionEndpointAuthMethodsSupported() {
return introspectionEndpointAuthMethodsSupported;
}
@JsonProperty(JSON_PROPERTY_INTROSPECTION_ENDPOINT_AUTH_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIntrospectionEndpointAuthMethodsSupported(
List introspectionEndpointAuthMethodsSupported) {
this.introspectionEndpointAuthMethodsSupported = introspectionEndpointAuthMethodsSupported;
}
public OAuthMetadata issuer(String issuer) {
this.issuer = issuer;
return this;
}
/**
* The authorization server's issuer identifier. In the context of this document, this is your authorization
* server's base URL. This becomes the `iss` claim in an access token.
*
* @return issuer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The authorization server's issuer identifier. In the context of this document, this is your authorization server's base URL. This becomes the `iss` claim in an access token.")
@JsonProperty(JSON_PROPERTY_ISSUER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIssuer() {
return issuer;
}
@JsonProperty(JSON_PROPERTY_ISSUER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIssuer(String issuer) {
this.issuer = issuer;
}
public OAuthMetadata jwksUri(String jwksUri) {
this.jwksUri = jwksUri;
return this;
}
/**
* URL of the authorization server's JSON Web Key Set document.
*
* @return jwksUri
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL of the authorization server's JSON Web Key Set document.")
@JsonProperty(JSON_PROPERTY_JWKS_URI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getJwksUri() {
return jwksUri;
}
@JsonProperty(JSON_PROPERTY_JWKS_URI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setJwksUri(String jwksUri) {
this.jwksUri = jwksUri;
}
public OAuthMetadata pushedAuthorizationRequestEndpoint(String pushedAuthorizationRequestEndpoint) {
this.pushedAuthorizationRequestEndpoint = pushedAuthorizationRequestEndpoint;
return this;
}
/**
* Get pushedAuthorizationRequestEndpoint
*
* @return pushedAuthorizationRequestEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PUSHED_AUTHORIZATION_REQUEST_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPushedAuthorizationRequestEndpoint() {
return pushedAuthorizationRequestEndpoint;
}
@JsonProperty(JSON_PROPERTY_PUSHED_AUTHORIZATION_REQUEST_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPushedAuthorizationRequestEndpoint(String pushedAuthorizationRequestEndpoint) {
this.pushedAuthorizationRequestEndpoint = pushedAuthorizationRequestEndpoint;
}
public OAuthMetadata registrationEndpoint(String registrationEndpoint) {
this.registrationEndpoint = registrationEndpoint;
return this;
}
/**
* URL of the authorization server's JSON Web Key Set document.
*
* @return registrationEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL of the authorization server's JSON Web Key Set document.")
@JsonProperty(JSON_PROPERTY_REGISTRATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRegistrationEndpoint() {
return registrationEndpoint;
}
@JsonProperty(JSON_PROPERTY_REGISTRATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRegistrationEndpoint(String registrationEndpoint) {
this.registrationEndpoint = registrationEndpoint;
}
public OAuthMetadata requestObjectSigningAlgValuesSupported(
List requestObjectSigningAlgValuesSupported) {
this.requestObjectSigningAlgValuesSupported = requestObjectSigningAlgValuesSupported;
return this;
}
public OAuthMetadata addrequestObjectSigningAlgValuesSupportedItem(
SigningAlgorithm requestObjectSigningAlgValuesSupportedItem) {
if (this.requestObjectSigningAlgValuesSupported == null) {
this.requestObjectSigningAlgValuesSupported = new ArrayList<>();
}
this.requestObjectSigningAlgValuesSupported.add(requestObjectSigningAlgValuesSupportedItem);
return this;
}
/**
* A list of signing algorithms that this authorization server supports for signed requests.
*
* @return requestObjectSigningAlgValuesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of signing algorithms that this authorization server supports for signed requests.")
@JsonProperty(JSON_PROPERTY_REQUEST_OBJECT_SIGNING_ALG_VALUES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getRequestObjectSigningAlgValuesSupported() {
return requestObjectSigningAlgValuesSupported;
}
@JsonProperty(JSON_PROPERTY_REQUEST_OBJECT_SIGNING_ALG_VALUES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRequestObjectSigningAlgValuesSupported(
List requestObjectSigningAlgValuesSupported) {
this.requestObjectSigningAlgValuesSupported = requestObjectSigningAlgValuesSupported;
}
public OAuthMetadata requestParameterSupported(Boolean requestParameterSupported) {
this.requestParameterSupported = requestParameterSupported;
return this;
}
/**
* Indicates if Request Parameters are supported by this authorization server.
*
* @return requestParameterSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if Request Parameters are supported by this authorization server.")
@JsonProperty(JSON_PROPERTY_REQUEST_PARAMETER_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getRequestParameterSupported() {
return requestParameterSupported;
}
@JsonProperty(JSON_PROPERTY_REQUEST_PARAMETER_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRequestParameterSupported(Boolean requestParameterSupported) {
this.requestParameterSupported = requestParameterSupported;
}
public OAuthMetadata responseModesSupported(List responseModesSupported) {
this.responseModesSupported = responseModesSupported;
return this;
}
public OAuthMetadata addresponseModesSupportedItem(ResponseMode responseModesSupportedItem) {
if (this.responseModesSupported == null) {
this.responseModesSupported = new ArrayList<>();
}
this.responseModesSupported.add(responseModesSupportedItem);
return this;
}
/**
* A list of the `response_mode` values that this authorization server supports. More information here.
*
* @return responseModesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of the `response_mode` values that this authorization server supports. More information here.")
@JsonProperty(JSON_PROPERTY_RESPONSE_MODES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getResponseModesSupported() {
return responseModesSupported;
}
@JsonProperty(JSON_PROPERTY_RESPONSE_MODES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResponseModesSupported(List responseModesSupported) {
this.responseModesSupported = responseModesSupported;
}
public OAuthMetadata responseTypesSupported(List responseTypesSupported) {
this.responseTypesSupported = responseTypesSupported;
return this;
}
public OAuthMetadata addresponseTypesSupportedItem(ResponseTypesSupported responseTypesSupportedItem) {
if (this.responseTypesSupported == null) {
this.responseTypesSupported = new ArrayList<>();
}
this.responseTypesSupported.add(responseTypesSupportedItem);
return this;
}
/**
* A list of the `response_type` values that this authorization server supports. Can be a combination of
* `code`, `token`, and `id_token`.
*
* @return responseTypesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of the `response_type` values that this authorization server supports. Can be a combination of `code`, `token`, and `id_token`.")
@JsonProperty(JSON_PROPERTY_RESPONSE_TYPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getResponseTypesSupported() {
return responseTypesSupported;
}
@JsonProperty(JSON_PROPERTY_RESPONSE_TYPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResponseTypesSupported(List responseTypesSupported) {
this.responseTypesSupported = responseTypesSupported;
}
public OAuthMetadata revocationEndpoint(String revocationEndpoint) {
this.revocationEndpoint = revocationEndpoint;
return this;
}
/**
* URL of the authorization server's revocation endpoint.
*
* @return revocationEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL of the authorization server's revocation endpoint.")
@JsonProperty(JSON_PROPERTY_REVOCATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRevocationEndpoint() {
return revocationEndpoint;
}
@JsonProperty(JSON_PROPERTY_REVOCATION_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRevocationEndpoint(String revocationEndpoint) {
this.revocationEndpoint = revocationEndpoint;
}
public OAuthMetadata revocationEndpointAuthMethodsSupported(
List revocationEndpointAuthMethodsSupported) {
this.revocationEndpointAuthMethodsSupported = revocationEndpointAuthMethodsSupported;
return this;
}
public OAuthMetadata addrevocationEndpointAuthMethodsSupportedItem(
EndpointAuthMethod revocationEndpointAuthMethodsSupportedItem) {
if (this.revocationEndpointAuthMethodsSupported == null) {
this.revocationEndpointAuthMethodsSupported = new ArrayList<>();
}
this.revocationEndpointAuthMethodsSupported.add(revocationEndpointAuthMethodsSupportedItem);
return this;
}
/**
* A list of client authentication methods supported by this revocation endpoint.
*
* @return revocationEndpointAuthMethodsSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of client authentication methods supported by this revocation endpoint.")
@JsonProperty(JSON_PROPERTY_REVOCATION_ENDPOINT_AUTH_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getRevocationEndpointAuthMethodsSupported() {
return revocationEndpointAuthMethodsSupported;
}
@JsonProperty(JSON_PROPERTY_REVOCATION_ENDPOINT_AUTH_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRevocationEndpointAuthMethodsSupported(
List revocationEndpointAuthMethodsSupported) {
this.revocationEndpointAuthMethodsSupported = revocationEndpointAuthMethodsSupported;
}
public OAuthMetadata scopesSupported(List scopesSupported) {
this.scopesSupported = scopesSupported;
return this;
}
public OAuthMetadata addscopesSupportedItem(String scopesSupportedItem) {
if (this.scopesSupported == null) {
this.scopesSupported = new ArrayList<>();
}
this.scopesSupported.add(scopesSupportedItem);
return this;
}
/**
* A list of the scope values that this authorization server supports.
*
* @return scopesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of the scope values that this authorization server supports.")
@JsonProperty(JSON_PROPERTY_SCOPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getScopesSupported() {
return scopesSupported;
}
@JsonProperty(JSON_PROPERTY_SCOPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setScopesSupported(List scopesSupported) {
this.scopesSupported = scopesSupported;
}
public OAuthMetadata subjectTypesSupported(List subjectTypesSupported) {
this.subjectTypesSupported = subjectTypesSupported;
return this;
}
public OAuthMetadata addsubjectTypesSupportedItem(SubjectType subjectTypesSupportedItem) {
if (this.subjectTypesSupported == null) {
this.subjectTypesSupported = new ArrayList<>();
}
this.subjectTypesSupported.add(subjectTypesSupportedItem);
return this;
}
/**
* A list of the Subject Identifier types that this authorization server supports. Valid types include
* `pairwise` and `public`, but only `public` is currently supported. See the [Subject
* Identifier Types](https://openid.net/specs/openid-connect-core-1_0.html#SubjectIDTypes) section in the OpenID
* Connect specification.
*
* @return subjectTypesSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of the Subject Identifier types that this authorization server supports. Valid types include `pairwise` and `public`, but only `public` is currently supported. See the [Subject Identifier Types](https://openid.net/specs/openid-connect-core-1_0.html#SubjectIDTypes) section in the OpenID Connect specification.")
@JsonProperty(JSON_PROPERTY_SUBJECT_TYPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSubjectTypesSupported() {
return subjectTypesSupported;
}
@JsonProperty(JSON_PROPERTY_SUBJECT_TYPES_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSubjectTypesSupported(List subjectTypesSupported) {
this.subjectTypesSupported = subjectTypesSupported;
}
public OAuthMetadata tokenEndpoint(String tokenEndpoint) {
this.tokenEndpoint = tokenEndpoint;
return this;
}
/**
* URL of the authorization server's token endpoint.
*
* @return tokenEndpoint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL of the authorization server's token endpoint.")
@JsonProperty(JSON_PROPERTY_TOKEN_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTokenEndpoint() {
return tokenEndpoint;
}
@JsonProperty(JSON_PROPERTY_TOKEN_ENDPOINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTokenEndpoint(String tokenEndpoint) {
this.tokenEndpoint = tokenEndpoint;
}
public OAuthMetadata tokenEndpointAuthMethodsSupported(List tokenEndpointAuthMethodsSupported) {
this.tokenEndpointAuthMethodsSupported = tokenEndpointAuthMethodsSupported;
return this;
}
public OAuthMetadata addtokenEndpointAuthMethodsSupportedItem(
EndpointAuthMethod tokenEndpointAuthMethodsSupportedItem) {
if (this.tokenEndpointAuthMethodsSupported == null) {
this.tokenEndpointAuthMethodsSupported = new ArrayList<>();
}
this.tokenEndpointAuthMethodsSupported.add(tokenEndpointAuthMethodsSupportedItem);
return this;
}
/**
* A list of client authentication methods supported by this token endpoint.
*
* @return tokenEndpointAuthMethodsSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of client authentication methods supported by this token endpoint.")
@JsonProperty(JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTokenEndpointAuthMethodsSupported() {
return tokenEndpointAuthMethodsSupported;
}
@JsonProperty(JSON_PROPERTY_TOKEN_ENDPOINT_AUTH_METHODS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTokenEndpointAuthMethodsSupported(List tokenEndpointAuthMethodsSupported) {
this.tokenEndpointAuthMethodsSupported = tokenEndpointAuthMethodsSupported;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OAuthMetadata oauthMetadata = (OAuthMetadata) o;
return Objects.equals(this.authorizationEndpoint, oauthMetadata.authorizationEndpoint)
&& Objects.equals(this.backchannelAuthenticationRequestSigningAlgValuesSupported,
oauthMetadata.backchannelAuthenticationRequestSigningAlgValuesSupported)
&& Objects.equals(this.backchannelTokenDeliveryModesSupported,
oauthMetadata.backchannelTokenDeliveryModesSupported)
&& Objects.equals(this.claimsSupported, oauthMetadata.claimsSupported)
&& Objects.equals(this.codeChallengeMethodsSupported, oauthMetadata.codeChallengeMethodsSupported)
&& Objects.equals(this.deviceAuthorizationEndpoint, oauthMetadata.deviceAuthorizationEndpoint)
&& Objects.equals(this.dpopSigningAlgValuesSupported, oauthMetadata.dpopSigningAlgValuesSupported)
&& Objects.equals(this.endSessionEndpoint, oauthMetadata.endSessionEndpoint)
&& Objects.equals(this.grantTypesSupported, oauthMetadata.grantTypesSupported)
&& Objects.equals(this.introspectionEndpoint, oauthMetadata.introspectionEndpoint)
&& Objects.equals(this.introspectionEndpointAuthMethodsSupported,
oauthMetadata.introspectionEndpointAuthMethodsSupported)
&& Objects.equals(this.issuer, oauthMetadata.issuer)
&& Objects.equals(this.jwksUri, oauthMetadata.jwksUri)
&& Objects.equals(this.pushedAuthorizationRequestEndpoint,
oauthMetadata.pushedAuthorizationRequestEndpoint)
&& Objects.equals(this.registrationEndpoint, oauthMetadata.registrationEndpoint)
&& Objects.equals(this.requestObjectSigningAlgValuesSupported,
oauthMetadata.requestObjectSigningAlgValuesSupported)
&& Objects.equals(this.requestParameterSupported, oauthMetadata.requestParameterSupported)
&& Objects.equals(this.responseModesSupported, oauthMetadata.responseModesSupported)
&& Objects.equals(this.responseTypesSupported, oauthMetadata.responseTypesSupported)
&& Objects.equals(this.revocationEndpoint, oauthMetadata.revocationEndpoint)
&& Objects.equals(this.revocationEndpointAuthMethodsSupported,
oauthMetadata.revocationEndpointAuthMethodsSupported)
&& Objects.equals(this.scopesSupported, oauthMetadata.scopesSupported)
&& Objects.equals(this.subjectTypesSupported, oauthMetadata.subjectTypesSupported)
&& Objects.equals(this.tokenEndpoint, oauthMetadata.tokenEndpoint) && Objects.equals(
this.tokenEndpointAuthMethodsSupported, oauthMetadata.tokenEndpointAuthMethodsSupported);
// ;
}
@Override
public int hashCode() {
return Objects.hash(authorizationEndpoint, backchannelAuthenticationRequestSigningAlgValuesSupported,
backchannelTokenDeliveryModesSupported, claimsSupported, codeChallengeMethodsSupported,
deviceAuthorizationEndpoint, dpopSigningAlgValuesSupported, endSessionEndpoint, grantTypesSupported,
introspectionEndpoint, introspectionEndpointAuthMethodsSupported, issuer, jwksUri,
pushedAuthorizationRequestEndpoint, registrationEndpoint, requestObjectSigningAlgValuesSupported,
requestParameterSupported, responseModesSupported, responseTypesSupported, revocationEndpoint,
revocationEndpointAuthMethodsSupported, scopesSupported, subjectTypesSupported, tokenEndpoint,
tokenEndpointAuthMethodsSupported);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OAuthMetadata {\n");
sb.append(" authorizationEndpoint: ").append(toIndentedString(authorizationEndpoint)).append("\n");
sb.append(" backchannelAuthenticationRequestSigningAlgValuesSupported: ")
.append(toIndentedString(backchannelAuthenticationRequestSigningAlgValuesSupported)).append("\n");
sb.append(" backchannelTokenDeliveryModesSupported: ")
.append(toIndentedString(backchannelTokenDeliveryModesSupported)).append("\n");
sb.append(" claimsSupported: ").append(toIndentedString(claimsSupported)).append("\n");
sb.append(" codeChallengeMethodsSupported: ").append(toIndentedString(codeChallengeMethodsSupported))
.append("\n");
sb.append(" deviceAuthorizationEndpoint: ").append(toIndentedString(deviceAuthorizationEndpoint))
.append("\n");
sb.append(" dpopSigningAlgValuesSupported: ").append(toIndentedString(dpopSigningAlgValuesSupported))
.append("\n");
sb.append(" endSessionEndpoint: ").append(toIndentedString(endSessionEndpoint)).append("\n");
sb.append(" grantTypesSupported: ").append(toIndentedString(grantTypesSupported)).append("\n");
sb.append(" introspectionEndpoint: ").append(toIndentedString(introspectionEndpoint)).append("\n");
sb.append(" introspectionEndpointAuthMethodsSupported: ")
.append(toIndentedString(introspectionEndpointAuthMethodsSupported)).append("\n");
sb.append(" issuer: ").append(toIndentedString(issuer)).append("\n");
sb.append(" jwksUri: ").append(toIndentedString(jwksUri)).append("\n");
sb.append(" pushedAuthorizationRequestEndpoint: ")
.append(toIndentedString(pushedAuthorizationRequestEndpoint)).append("\n");
sb.append(" registrationEndpoint: ").append(toIndentedString(registrationEndpoint)).append("\n");
sb.append(" requestObjectSigningAlgValuesSupported: ")
.append(toIndentedString(requestObjectSigningAlgValuesSupported)).append("\n");
sb.append(" requestParameterSupported: ").append(toIndentedString(requestParameterSupported)).append("\n");
sb.append(" responseModesSupported: ").append(toIndentedString(responseModesSupported)).append("\n");
sb.append(" responseTypesSupported: ").append(toIndentedString(responseTypesSupported)).append("\n");
sb.append(" revocationEndpoint: ").append(toIndentedString(revocationEndpoint)).append("\n");
sb.append(" revocationEndpointAuthMethodsSupported: ")
.append(toIndentedString(revocationEndpointAuthMethodsSupported)).append("\n");
sb.append(" scopesSupported: ").append(toIndentedString(scopesSupported)).append("\n");
sb.append(" subjectTypesSupported: ").append(toIndentedString(subjectTypesSupported)).append("\n");
sb.append(" tokenEndpoint: ").append(toIndentedString(tokenEndpoint)).append("\n");
sb.append(" tokenEndpointAuthMethodsSupported: ").append(toIndentedString(tokenEndpointAuthMethodsSupported))
.append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy