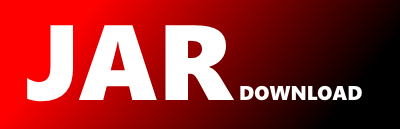
com.okta.sdk.resource.model.OrgPreferencesLinksHideEndUserFooter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.HrefHints;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* OrgPreferencesLinksHideEndUserFooter
*/
@JsonPropertyOrder({ OrgPreferencesLinksHideEndUserFooter.JSON_PROPERTY_HINTS,
OrgPreferencesLinksHideEndUserFooter.JSON_PROPERTY_HREF,
OrgPreferencesLinksHideEndUserFooter.JSON_PROPERTY_NAME,
OrgPreferencesLinksHideEndUserFooter.JSON_PROPERTY_TEMPLATED,
OrgPreferencesLinksHideEndUserFooter.JSON_PROPERTY_TYPE })
@JsonTypeName("OrgPreferences__links_hideEndUserFooter")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class OrgPreferencesLinksHideEndUserFooter implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_HINTS = "hints";
private HrefHints hints;
public static final String JSON_PROPERTY_HREF = "href";
private String href;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_TEMPLATED = "templated";
private Boolean templated;
public static final String JSON_PROPERTY_TYPE = "type";
private String type;
public OrgPreferencesLinksHideEndUserFooter() {
}
public OrgPreferencesLinksHideEndUserFooter hints(HrefHints hints) {
this.hints = hints;
return this;
}
/**
* Get hints
*
* @return hints
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefHints getHints() {
return hints;
}
@JsonProperty(JSON_PROPERTY_HINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHints(HrefHints hints) {
this.hints = hints;
}
public OrgPreferencesLinksHideEndUserFooter href(String href) {
this.href = href;
return this;
}
/**
* Link URI
*
* @return href
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Link URI")
@JsonProperty(JSON_PROPERTY_HREF)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getHref() {
return href;
}
@JsonProperty(JSON_PROPERTY_HREF)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHref(String href) {
this.href = href;
}
public OrgPreferencesLinksHideEndUserFooter name(String name) {
this.name = name;
return this;
}
/**
* Link name
*
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Link name")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public OrgPreferencesLinksHideEndUserFooter templated(Boolean templated) {
this.templated = templated;
return this;
}
/**
* Indicates whether the Link Object's `href` property is a URI template.
*
* @return templated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether the Link Object's `href` property is a URI template.")
@JsonProperty(JSON_PROPERTY_TEMPLATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getTemplated() {
return templated;
}
@JsonProperty(JSON_PROPERTY_TEMPLATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTemplated(Boolean templated) {
this.templated = templated;
}
public OrgPreferencesLinksHideEndUserFooter type(String type) {
this.type = type;
return this;
}
/**
* The media type of the link. If omitted, it is implicitly `application/json`.
*
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The media type of the link. If omitted, it is implicitly `application/json`.")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(String type) {
this.type = type;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrgPreferencesLinksHideEndUserFooter orgPreferencesLinksHideEndUserFooter = (OrgPreferencesLinksHideEndUserFooter) o;
return Objects.equals(this.hints, orgPreferencesLinksHideEndUserFooter.hints)
&& Objects.equals(this.href, orgPreferencesLinksHideEndUserFooter.href)
&& Objects.equals(this.name, orgPreferencesLinksHideEndUserFooter.name)
&& Objects.equals(this.templated, orgPreferencesLinksHideEndUserFooter.templated)
&& Objects.equals(this.type, orgPreferencesLinksHideEndUserFooter.type);
// ;
}
@Override
public int hashCode() {
return Objects.hash(hints, href, name, templated, type);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrgPreferencesLinksHideEndUserFooter {\n");
sb.append(" hints: ").append(toIndentedString(hints)).append("\n");
sb.append(" href: ").append(toIndentedString(href)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" templated: ").append(toIndentedString(templated)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy