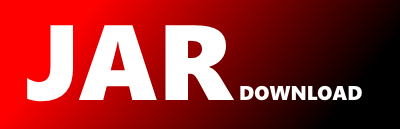
com.okta.sdk.resource.model.OrgSetting Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.OrgGeneralSettingLinks;
import java.time.OffsetDateTime;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* OrgSetting
*/
@JsonPropertyOrder({ OrgSetting.JSON_PROPERTY_ADDRESS1, OrgSetting.JSON_PROPERTY_ADDRESS2,
OrgSetting.JSON_PROPERTY_CITY, OrgSetting.JSON_PROPERTY_COMPANY_NAME, OrgSetting.JSON_PROPERTY_COUNTRY,
OrgSetting.JSON_PROPERTY_CREATED, OrgSetting.JSON_PROPERTY_END_USER_SUPPORT_HELP_U_R_L,
OrgSetting.JSON_PROPERTY_EXPIRES_AT, OrgSetting.JSON_PROPERTY_ID, OrgSetting.JSON_PROPERTY_LAST_UPDATED,
OrgSetting.JSON_PROPERTY_PHONE_NUMBER, OrgSetting.JSON_PROPERTY_POSTAL_CODE, OrgSetting.JSON_PROPERTY_STATE,
OrgSetting.JSON_PROPERTY_STATUS, OrgSetting.JSON_PROPERTY_SUBDOMAIN,
OrgSetting.JSON_PROPERTY_SUPPORT_PHONE_NUMBER, OrgSetting.JSON_PROPERTY_WEBSITE,
OrgSetting.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class OrgSetting implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ADDRESS1 = "address1";
private String address1;
public static final String JSON_PROPERTY_ADDRESS2 = "address2";
private String address2;
public static final String JSON_PROPERTY_CITY = "city";
private String city;
public static final String JSON_PROPERTY_COMPANY_NAME = "companyName";
private String companyName;
public static final String JSON_PROPERTY_COUNTRY = "country";
private String country;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_END_USER_SUPPORT_HELP_U_R_L = "endUserSupportHelpURL";
private String endUserSupportHelpURL;
public static final String JSON_PROPERTY_EXPIRES_AT = "expiresAt";
private OffsetDateTime expiresAt;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_PHONE_NUMBER = "phoneNumber";
private String phoneNumber;
public static final String JSON_PROPERTY_POSTAL_CODE = "postalCode";
private String postalCode;
public static final String JSON_PROPERTY_STATE = "state";
private String state;
/**
* Status of org
*/
public enum StatusEnum {
ACTIVE("ACTIVE"),
INACTIVE("INACTIVE"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_STATUS = "status";
private StatusEnum status;
public static final String JSON_PROPERTY_SUBDOMAIN = "subdomain";
private String subdomain;
public static final String JSON_PROPERTY_SUPPORT_PHONE_NUMBER = "supportPhoneNumber";
private String supportPhoneNumber;
public static final String JSON_PROPERTY_WEBSITE = "website";
private String website;
public static final String JSON_PROPERTY_LINKS = "_links";
private OrgGeneralSettingLinks links;
public OrgSetting() {
}
/*
* @JsonCreator public OrgSetting(
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_EXPIRES_AT) OffsetDateTime expiresAt,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated,
*
* @JsonProperty(JSON_PROPERTY_STATUS) StatusEnum status,
*
* @JsonProperty(JSON_PROPERTY_SUBDOMAIN) String subdomain ) { this(); this.created = created; this.expiresAt =
* expiresAt; this.id = id; this.lastUpdated = lastUpdated; this.status = status; this.subdomain = subdomain; }
*/
public OrgSetting address1(String address1) {
this.address1 = address1;
return this;
}
/**
* Primary address of the organization associated with the org
*
* @return address1
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Primary address of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_ADDRESS1)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAddress1() {
return address1;
}
@JsonProperty(JSON_PROPERTY_ADDRESS1)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAddress1(String address1) {
this.address1 = address1;
}
public OrgSetting address2(String address2) {
this.address2 = address2;
return this;
}
/**
* Secondary address of the organization associated with the org
*
* @return address2
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Secondary address of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_ADDRESS2)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAddress2() {
return address2;
}
@JsonProperty(JSON_PROPERTY_ADDRESS2)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAddress2(String address2) {
this.address2 = address2;
}
public OrgSetting city(String city) {
this.city = city;
return this;
}
/**
* City of the organization associated with the org
*
* @return city
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "City of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_CITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCity() {
return city;
}
@JsonProperty(JSON_PROPERTY_CITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCity(String city) {
this.city = city;
}
public OrgSetting companyName(String companyName) {
this.companyName = companyName;
return this;
}
/**
* Name of org
*
* @return companyName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of org")
@JsonProperty(JSON_PROPERTY_COMPANY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCompanyName() {
return companyName;
}
@JsonProperty(JSON_PROPERTY_COMPANY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public OrgSetting country(String country) {
this.country = country;
return this;
}
/**
* County of the organization associated with the org
*
* @return country
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "County of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_COUNTRY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCountry() {
return country;
}
@JsonProperty(JSON_PROPERTY_COUNTRY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCountry(String country) {
this.country = country;
}
/**
* When org was created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "When org was created")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
public OrgSetting endUserSupportHelpURL(String endUserSupportHelpURL) {
this.endUserSupportHelpURL = endUserSupportHelpURL;
return this;
}
/**
* Support link of org
*
* @return endUserSupportHelpURL
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Support link of org")
@JsonProperty(JSON_PROPERTY_END_USER_SUPPORT_HELP_U_R_L)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEndUserSupportHelpURL() {
return endUserSupportHelpURL;
}
@JsonProperty(JSON_PROPERTY_END_USER_SUPPORT_HELP_U_R_L)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEndUserSupportHelpURL(String endUserSupportHelpURL) {
this.endUserSupportHelpURL = endUserSupportHelpURL;
}
/**
* Expiration of org
*
* @return expiresAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Expiration of org")
@JsonProperty(JSON_PROPERTY_EXPIRES_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getExpiresAt() {
return expiresAt;
}
/**
* Org ID
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Org ID")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
/**
* When org was last updated
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "When org was last updated")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
public OrgSetting phoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* Phone number of the organization associated with the org
*
* @return phoneNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Phone number of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_PHONE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPhoneNumber() {
return phoneNumber;
}
@JsonProperty(JSON_PROPERTY_PHONE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
public OrgSetting postalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
/**
* Postal code of the organization associated with the org
*
* @return postalCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Postal code of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_POSTAL_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPostalCode() {
return postalCode;
}
@JsonProperty(JSON_PROPERTY_POSTAL_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
public OrgSetting state(String state) {
this.state = state;
return this;
}
/**
* State of the organization associated with the org
*
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "State of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getState() {
return state;
}
@JsonProperty(JSON_PROPERTY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setState(String state) {
this.state = state;
}
/**
* Status of org
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Status of org")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public StatusEnum getStatus() {
return status;
}
/**
* Subdomain of org
*
* @return subdomain
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Subdomain of org")
@JsonProperty(JSON_PROPERTY_SUBDOMAIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSubdomain() {
return subdomain;
}
public OrgSetting supportPhoneNumber(String supportPhoneNumber) {
this.supportPhoneNumber = supportPhoneNumber;
return this;
}
/**
* Support help phone of the organization associated with the org
*
* @return supportPhoneNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Support help phone of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_SUPPORT_PHONE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSupportPhoneNumber() {
return supportPhoneNumber;
}
@JsonProperty(JSON_PROPERTY_SUPPORT_PHONE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSupportPhoneNumber(String supportPhoneNumber) {
this.supportPhoneNumber = supportPhoneNumber;
}
public OrgSetting website(String website) {
this.website = website;
return this;
}
/**
* Website of the organization associated with the org
*
* @return website
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Website of the organization associated with the org")
@JsonProperty(JSON_PROPERTY_WEBSITE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getWebsite() {
return website;
}
@JsonProperty(JSON_PROPERTY_WEBSITE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWebsite(String website) {
this.website = website;
}
public OrgSetting links(OrgGeneralSettingLinks links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OrgGeneralSettingLinks getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(OrgGeneralSettingLinks links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OrgSetting orgSetting = (OrgSetting) o;
return Objects.equals(this.address1, orgSetting.address1) && Objects.equals(this.address2, orgSetting.address2)
&& Objects.equals(this.city, orgSetting.city)
&& Objects.equals(this.companyName, orgSetting.companyName)
&& Objects.equals(this.country, orgSetting.country) && Objects.equals(this.created, orgSetting.created)
&& Objects.equals(this.endUserSupportHelpURL, orgSetting.endUserSupportHelpURL)
&& Objects.equals(this.expiresAt, orgSetting.expiresAt) && Objects.equals(this.id, orgSetting.id)
&& Objects.equals(this.lastUpdated, orgSetting.lastUpdated)
&& Objects.equals(this.phoneNumber, orgSetting.phoneNumber)
&& Objects.equals(this.postalCode, orgSetting.postalCode)
&& Objects.equals(this.state, orgSetting.state) && Objects.equals(this.status, orgSetting.status)
&& Objects.equals(this.subdomain, orgSetting.subdomain)
&& Objects.equals(this.supportPhoneNumber, orgSetting.supportPhoneNumber)
&& Objects.equals(this.website, orgSetting.website) && Objects.equals(this.links, orgSetting.links);
// ;
}
@Override
public int hashCode() {
return Objects.hash(address1, address2, city, companyName, country, created, endUserSupportHelpURL, expiresAt,
id, lastUpdated, phoneNumber, postalCode, state, status, subdomain, supportPhoneNumber, website, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OrgSetting {\n");
sb.append(" address1: ").append(toIndentedString(address1)).append("\n");
sb.append(" address2: ").append(toIndentedString(address2)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" companyName: ").append(toIndentedString(companyName)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" endUserSupportHelpURL: ").append(toIndentedString(endUserSupportHelpURL)).append("\n");
sb.append(" expiresAt: ").append(toIndentedString(expiresAt)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" phoneNumber: ").append(toIndentedString(phoneNumber)).append("\n");
sb.append(" postalCode: ").append(toIndentedString(postalCode)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" subdomain: ").append(toIndentedString(subdomain)).append("\n");
sb.append(" supportPhoneNumber: ").append(toIndentedString(supportPhoneNumber)).append("\n");
sb.append(" website: ").append(toIndentedString(website)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy