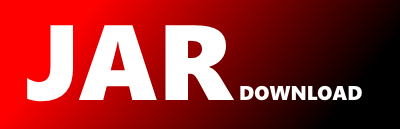
com.okta.sdk.resource.model.PasswordCredentialHash Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.DigestAlgorithm;
import com.okta.sdk.resource.model.PasswordCredentialHashAlgorithm;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Specifies a hashed password to import into Okta. This allows an existing password to be imported into Okta directly
* from some other store. Okta supports the BCRYPT, SHA-512, SHA-256, SHA-1, MD5, and PBKDF2 hash functions for password
* import. A hashed password may be specified in a Password object when creating or updating a user, but not for other
* operations. See [Create User with Imported Hashed
* Password](https://developer.okta.com/docs/reference/api/users/#create-user-with-imported-hashed-password) for
* information on using this object when creating a user. When updating a User with a hashed password, the User must be
* in the `STAGED` status.
*/
@ApiModel(description = "Specifies a hashed password to import into Okta. This allows an existing password to be imported into Okta directly from some other store. Okta supports the BCRYPT, SHA-512, SHA-256, SHA-1, MD5, and PBKDF2 hash functions for password import. A hashed password may be specified in a Password object when creating or updating a user, but not for other operations. See [Create User with Imported Hashed Password](https://developer.okta.com/docs/reference/api/users/#create-user-with-imported-hashed-password) for information on using this object when creating a user. When updating a User with a hashed password, the User must be in the `STAGED` status.")
@JsonPropertyOrder({ PasswordCredentialHash.JSON_PROPERTY_ALGORITHM,
PasswordCredentialHash.JSON_PROPERTY_DIGEST_ALGORITHM, PasswordCredentialHash.JSON_PROPERTY_ITERATION_COUNT,
PasswordCredentialHash.JSON_PROPERTY_KEY_SIZE, PasswordCredentialHash.JSON_PROPERTY_SALT,
PasswordCredentialHash.JSON_PROPERTY_SALT_ORDER, PasswordCredentialHash.JSON_PROPERTY_VALUE,
PasswordCredentialHash.JSON_PROPERTY_WORK_FACTOR })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class PasswordCredentialHash implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ALGORITHM = "algorithm";
private PasswordCredentialHashAlgorithm algorithm;
public static final String JSON_PROPERTY_DIGEST_ALGORITHM = "digestAlgorithm";
private DigestAlgorithm digestAlgorithm;
public static final String JSON_PROPERTY_ITERATION_COUNT = "iterationCount";
private Integer iterationCount;
public static final String JSON_PROPERTY_KEY_SIZE = "keySize";
private Integer keySize;
public static final String JSON_PROPERTY_SALT = "salt";
private String salt;
public static final String JSON_PROPERTY_SALT_ORDER = "saltOrder";
private String saltOrder;
public static final String JSON_PROPERTY_VALUE = "value";
private String value;
public static final String JSON_PROPERTY_WORK_FACTOR = "workFactor";
private Integer workFactor;
public PasswordCredentialHash() {
}
public PasswordCredentialHash algorithm(PasswordCredentialHashAlgorithm algorithm) {
this.algorithm = algorithm;
return this;
}
/**
* Get algorithm
*
* @return algorithm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PasswordCredentialHashAlgorithm getAlgorithm() {
return algorithm;
}
@JsonProperty(JSON_PROPERTY_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAlgorithm(PasswordCredentialHashAlgorithm algorithm) {
this.algorithm = algorithm;
}
public PasswordCredentialHash digestAlgorithm(DigestAlgorithm digestAlgorithm) {
this.digestAlgorithm = digestAlgorithm;
return this;
}
/**
* Get digestAlgorithm
*
* @return digestAlgorithm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DIGEST_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DigestAlgorithm getDigestAlgorithm() {
return digestAlgorithm;
}
@JsonProperty(JSON_PROPERTY_DIGEST_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDigestAlgorithm(DigestAlgorithm digestAlgorithm) {
this.digestAlgorithm = digestAlgorithm;
}
public PasswordCredentialHash iterationCount(Integer iterationCount) {
this.iterationCount = iterationCount;
return this;
}
/**
* The number of iterations used when hashing passwords using PBKDF2. Must be >= 4096. Only required for
* PBKDF2 algorithm.
*
* @return iterationCount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of iterations used when hashing passwords using PBKDF2. Must be >= 4096. Only required for PBKDF2 algorithm.")
@JsonProperty(JSON_PROPERTY_ITERATION_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getIterationCount() {
return iterationCount;
}
@JsonProperty(JSON_PROPERTY_ITERATION_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIterationCount(Integer iterationCount) {
this.iterationCount = iterationCount;
}
public PasswordCredentialHash keySize(Integer keySize) {
this.keySize = keySize;
return this;
}
/**
* Size of the derived key in bytes. Only required for PBKDF2 algorithm.
*
* @return keySize
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Size of the derived key in bytes. Only required for PBKDF2 algorithm.")
@JsonProperty(JSON_PROPERTY_KEY_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getKeySize() {
return keySize;
}
@JsonProperty(JSON_PROPERTY_KEY_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setKeySize(Integer keySize) {
this.keySize = keySize;
}
public PasswordCredentialHash salt(String salt) {
this.salt = salt;
return this;
}
/**
* Only required for salted hashes. For BCRYPT, this specifies Radix-64 as the encoded salt used to generate the
* hash, which must be 22 characters long. For other salted hashes, this specifies the Base64-encoded salt used to
* generate the hash.
*
* @return salt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Only required for salted hashes. For BCRYPT, this specifies Radix-64 as the encoded salt used to generate the hash, which must be 22 characters long. For other salted hashes, this specifies the Base64-encoded salt used to generate the hash.")
@JsonProperty(JSON_PROPERTY_SALT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSalt() {
return salt;
}
@JsonProperty(JSON_PROPERTY_SALT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSalt(String salt) {
this.salt = salt;
}
public PasswordCredentialHash saltOrder(String saltOrder) {
this.saltOrder = saltOrder;
return this;
}
/**
* Specifies whether salt was pre- or postfixed to the password before hashing. Only required for salted algorithms.
*
* @return saltOrder
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies whether salt was pre- or postfixed to the password before hashing. Only required for salted algorithms.")
@JsonProperty(JSON_PROPERTY_SALT_ORDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSaltOrder() {
return saltOrder;
}
@JsonProperty(JSON_PROPERTY_SALT_ORDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSaltOrder(String saltOrder) {
this.saltOrder = saltOrder;
}
public PasswordCredentialHash value(String value) {
this.value = value;
return this;
}
/**
* For SHA-512, SHA-256, SHA-1, MD5, and PBKDF2, this is the actual base64-encoded hash of the password (and salt,
* if used). This is the Base64-encoded `value` of the SHA-512/SHA-256/SHA-1/MD5/PBKDF2 digest that was
* computed by either pre-fixing or post-fixing the `salt` to the `password`, depending on the
* `saltOrder`. If a `salt` was not used in the `source` system, then this should just
* be the Base64-encoded `value` of the password's SHA-512/SHA-256/SHA-1/MD5/PBKDF2 digest. For
* BCRYPT, this is the actual Radix-64 encoded hashed password.
*
* @return value
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "For SHA-512, SHA-256, SHA-1, MD5, and PBKDF2, this is the actual base64-encoded hash of the password (and salt, if used). This is the Base64-encoded `value` of the SHA-512/SHA-256/SHA-1/MD5/PBKDF2 digest that was computed by either pre-fixing or post-fixing the `salt` to the `password`, depending on the `saltOrder`. If a `salt` was not used in the `source` system, then this should just be the Base64-encoded `value` of the password's SHA-512/SHA-256/SHA-1/MD5/PBKDF2 digest. For BCRYPT, this is the actual Radix-64 encoded hashed password.")
@JsonProperty(JSON_PROPERTY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getValue() {
return value;
}
@JsonProperty(JSON_PROPERTY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setValue(String value) {
this.value = value;
}
public PasswordCredentialHash workFactor(Integer workFactor) {
this.workFactor = workFactor;
return this;
}
/**
* Governs the strength of the hash and the time required to compute it. Only required for BCRYPT algorithm.
* minimum: 1 maximum: 20
*
* @return workFactor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Governs the strength of the hash and the time required to compute it. Only required for BCRYPT algorithm.")
@JsonProperty(JSON_PROPERTY_WORK_FACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getWorkFactor() {
return workFactor;
}
@JsonProperty(JSON_PROPERTY_WORK_FACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWorkFactor(Integer workFactor) {
this.workFactor = workFactor;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PasswordCredentialHash passwordCredentialHash = (PasswordCredentialHash) o;
return Objects.equals(this.algorithm, passwordCredentialHash.algorithm)
&& Objects.equals(this.digestAlgorithm, passwordCredentialHash.digestAlgorithm)
&& Objects.equals(this.iterationCount, passwordCredentialHash.iterationCount)
&& Objects.equals(this.keySize, passwordCredentialHash.keySize)
&& Objects.equals(this.salt, passwordCredentialHash.salt)
&& Objects.equals(this.saltOrder, passwordCredentialHash.saltOrder)
&& Objects.equals(this.value, passwordCredentialHash.value)
&& Objects.equals(this.workFactor, passwordCredentialHash.workFactor);
// ;
}
@Override
public int hashCode() {
return Objects.hash(algorithm, digestAlgorithm, iterationCount, keySize, salt, saltOrder, value, workFactor);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PasswordCredentialHash {\n");
sb.append(" algorithm: ").append(toIndentedString(algorithm)).append("\n");
sb.append(" digestAlgorithm: ").append(toIndentedString(digestAlgorithm)).append("\n");
sb.append(" iterationCount: ").append(toIndentedString(iterationCount)).append("\n");
sb.append(" keySize: ").append(toIndentedString(keySize)).append("\n");
sb.append(" salt: ").append(toIndentedString(salt)).append("\n");
sb.append(" saltOrder: ").append(toIndentedString(saltOrder)).append("\n");
sb.append(" value: ").append(toIndentedString(value)).append("\n");
sb.append(" workFactor: ").append(toIndentedString(workFactor)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy