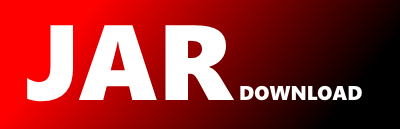
com.okta.sdk.resource.model.PolicySubject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.PolicySubjectMatchType;
import com.okta.sdk.resource.model.PolicyUserNameTemplate;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Specifies the behavior for establishing, validating, and matching a username for an IdP User
*/
@ApiModel(description = "Specifies the behavior for establishing, validating, and matching a username for an IdP User")
@JsonPropertyOrder({ PolicySubject.JSON_PROPERTY_FILTER, PolicySubject.JSON_PROPERTY_MATCH_ATTRIBUTE,
PolicySubject.JSON_PROPERTY_MATCH_TYPE, PolicySubject.JSON_PROPERTY_USER_NAME_TEMPLATE })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class PolicySubject implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_FILTER = "filter";
private String filter;
public static final String JSON_PROPERTY_MATCH_ATTRIBUTE = "matchAttribute";
private String matchAttribute;
public static final String JSON_PROPERTY_MATCH_TYPE = "matchType";
private PolicySubjectMatchType matchType;
public static final String JSON_PROPERTY_USER_NAME_TEMPLATE = "userNameTemplate";
private PolicyUserNameTemplate userNameTemplate;
public PolicySubject() {
}
public PolicySubject filter(String filter) {
this.filter = filter;
return this;
}
/**
* Optional [regular expression
* pattern](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Regular_expressions) used to filter
* untrusted IdP usernames. * As a best security practice, you should define a regular expression pattern to filter
* untrusted IdP usernames. This is especially important if multiple IdPs are connected to your org. The filter
* prevents an IdP from issuing an assertion for any User, including partners or directory Users in your Okta org. *
* For example, the filter pattern `(\\S+@example\\.com)` allows only Users that have an
* `@example.com` username suffix. It rejects assertions that have any other suffix such as
* `@corp.example.com` or `@partner.com`. * Only `SAML2` and `OIDC` IdP
* providers support the `filter` property.
*
* @return filter
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "(\\S+@example\\.com)", value = "Optional [regular expression pattern](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Regular_expressions) used to filter untrusted IdP usernames. * As a best security practice, you should define a regular expression pattern to filter untrusted IdP usernames. This is especially important if multiple IdPs are connected to your org. The filter prevents an IdP from issuing an assertion for any User, including partners or directory Users in your Okta org. * For example, the filter pattern `(\\S+@example\\.com)` allows only Users that have an `@example.com` username suffix. It rejects assertions that have any other suffix such as `@corp.example.com` or `@partner.com`. * Only `SAML2` and `OIDC` IdP providers support the `filter` property.")
@JsonProperty(JSON_PROPERTY_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFilter() {
return filter;
}
@JsonProperty(JSON_PROPERTY_FILTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFilter(String filter) {
this.filter = filter;
}
public PolicySubject matchAttribute(String matchAttribute) {
this.matchAttribute = matchAttribute;
return this;
}
/**
* Okta User profile attribute for matching a transformed IdP username. Only for matchType
* `CUSTOM_ATTRIBUTE`. The `matchAttribute` must be a valid Okta User profile attribute of one
* of the following types: * String (with no format or 'email' format only) * Integer * Number
*
* @return matchAttribute
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "login", value = "Okta User profile attribute for matching a transformed IdP username. Only for matchType `CUSTOM_ATTRIBUTE`. The `matchAttribute` must be a valid Okta User profile attribute of one of the following types: * String (with no format or 'email' format only) * Integer * Number")
@JsonProperty(JSON_PROPERTY_MATCH_ATTRIBUTE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMatchAttribute() {
return matchAttribute;
}
@JsonProperty(JSON_PROPERTY_MATCH_ATTRIBUTE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMatchAttribute(String matchAttribute) {
this.matchAttribute = matchAttribute;
}
public PolicySubject matchType(PolicySubjectMatchType matchType) {
this.matchType = matchType;
return this;
}
/**
* Get matchType
*
* @return matchType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_MATCH_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PolicySubjectMatchType getMatchType() {
return matchType;
}
@JsonProperty(JSON_PROPERTY_MATCH_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMatchType(PolicySubjectMatchType matchType) {
this.matchType = matchType;
}
public PolicySubject userNameTemplate(PolicyUserNameTemplate userNameTemplate) {
this.userNameTemplate = userNameTemplate;
return this;
}
/**
* Get userNameTemplate
*
* @return userNameTemplate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_USER_NAME_TEMPLATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PolicyUserNameTemplate getUserNameTemplate() {
return userNameTemplate;
}
@JsonProperty(JSON_PROPERTY_USER_NAME_TEMPLATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserNameTemplate(PolicyUserNameTemplate userNameTemplate) {
this.userNameTemplate = userNameTemplate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PolicySubject policySubject = (PolicySubject) o;
return Objects.equals(this.filter, policySubject.filter)
&& Objects.equals(this.matchAttribute, policySubject.matchAttribute)
&& Objects.equals(this.matchType, policySubject.matchType)
&& Objects.equals(this.userNameTemplate, policySubject.userNameTemplate);
// ;
}
@Override
public int hashCode() {
return Objects.hash(filter, matchAttribute, matchType, userNameTemplate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PolicySubject {\n");
sb.append(" filter: ").append(toIndentedString(filter)).append("\n");
sb.append(" matchAttribute: ").append(toIndentedString(matchAttribute)).append("\n");
sb.append(" matchType: ").append(toIndentedString(matchType)).append("\n");
sb.append(" userNameTemplate: ").append(toIndentedString(userNameTemplate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy