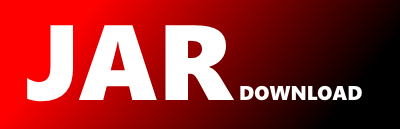
com.okta.sdk.resource.model.PossessionConstraint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.AuthenticationMethodObject;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* PossessionConstraint
*/
@JsonPropertyOrder({ PossessionConstraint.JSON_PROPERTY_AUTHENTICATION_METHODS,
PossessionConstraint.JSON_PROPERTY_EXCLUDED_AUTHENTICATION_METHODS, PossessionConstraint.JSON_PROPERTY_METHODS,
PossessionConstraint.JSON_PROPERTY_REAUTHENTICATE_IN, PossessionConstraint.JSON_PROPERTY_REQUIRED,
PossessionConstraint.JSON_PROPERTY_TYPES, PossessionConstraint.JSON_PROPERTY_DEVICE_BOUND,
PossessionConstraint.JSON_PROPERTY_HARDWARE_PROTECTION, PossessionConstraint.JSON_PROPERTY_PHISHING_RESISTANT,
PossessionConstraint.JSON_PROPERTY_USER_PRESENCE, PossessionConstraint.JSON_PROPERTY_USER_VERIFICATION })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class PossessionConstraint implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AUTHENTICATION_METHODS = "authenticationMethods";
private List authenticationMethods = null;
public static final String JSON_PROPERTY_EXCLUDED_AUTHENTICATION_METHODS = "excludedAuthenticationMethods";
private List excludedAuthenticationMethods = null;
/**
* Gets or Sets methods
*/
public enum MethodsEnum {
PASSWORD("PASSWORD"),
SECURITY_QUESTION("SECURITY_QUESTION"),
SMS("SMS"),
VOICE("VOICE"),
EMAIL("EMAIL"),
PUSH("PUSH"),
SIGNED_NONCE("SIGNED_NONCE"),
OTP("OTP"),
TOTP("TOTP"),
WEBAUTHN("WEBAUTHN"),
DUO("DUO"),
IDP("IDP"),
CERT("CERT"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
MethodsEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static MethodsEnum fromValue(String value) {
for (MethodsEnum b : MethodsEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_METHODS = "methods";
private List methods = null;
public static final String JSON_PROPERTY_REAUTHENTICATE_IN = "reauthenticateIn";
private String reauthenticateIn;
public static final String JSON_PROPERTY_REQUIRED = "required";
private Boolean required;
/**
* Gets or Sets types
*/
public enum TypesEnum {
SECURITY_KEY("SECURITY_KEY"),
PHONE("PHONE"),
EMAIL("EMAIL"),
PASSWORD("PASSWORD"),
SECURITY_QUESTION("SECURITY_QUESTION"),
APP("APP"),
FEDERATED("FEDERATED"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypesEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypesEnum fromValue(String value) {
for (TypesEnum b : TypesEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_TYPES = "types";
private List types = null;
/**
* Indicates if device-bound Factors are required. This property is only set for `POSSESSION` constraints.
*/
public enum DeviceBoundEnum {
OPTIONAL("OPTIONAL"),
REQUIRED("REQUIRED"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
DeviceBoundEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static DeviceBoundEnum fromValue(String value) {
for (DeviceBoundEnum b : DeviceBoundEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_DEVICE_BOUND = "deviceBound";
private DeviceBoundEnum deviceBound = DeviceBoundEnum.OPTIONAL;
/**
* Indicates if any secrets or private keys used during authentication must be hardware protected and not
* exportable. This property is only set for `POSSESSION` constraints.
*/
public enum HardwareProtectionEnum {
OPTIONAL("OPTIONAL"),
REQUIRED("REQUIRED"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
HardwareProtectionEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static HardwareProtectionEnum fromValue(String value) {
for (HardwareProtectionEnum b : HardwareProtectionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_HARDWARE_PROTECTION = "hardwareProtection";
private HardwareProtectionEnum hardwareProtection = HardwareProtectionEnum.OPTIONAL;
/**
* Indicates if phishing-resistant Factors are required. This property is only set for `POSSESSION`
* constraints.
*/
public enum PhishingResistantEnum {
OPTIONAL("OPTIONAL"),
REQUIRED("REQUIRED"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
PhishingResistantEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static PhishingResistantEnum fromValue(String value) {
for (PhishingResistantEnum b : PhishingResistantEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_PHISHING_RESISTANT = "phishingResistant";
private PhishingResistantEnum phishingResistant = PhishingResistantEnum.OPTIONAL;
/**
* Indicates if the user needs to approve an Okta Verify prompt or provide biometrics (meets NIST AAL2
* requirements). This property is only set for `POSSESSION` constraints.
*/
public enum UserPresenceEnum {
OPTIONAL("OPTIONAL"),
REQUIRED("REQUIRED"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
UserPresenceEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static UserPresenceEnum fromValue(String value) {
for (UserPresenceEnum b : UserPresenceEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_USER_PRESENCE = "userPresence";
private UserPresenceEnum userPresence = UserPresenceEnum.REQUIRED;
/**
* Indicates the user interaction requirement (PIN or biometrics) to ensure verification of a possession factor
*/
public enum UserVerificationEnum {
OPTIONAL("OPTIONAL"),
REQUIRED("REQUIRED"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
UserVerificationEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static UserVerificationEnum fromValue(String value) {
for (UserVerificationEnum b : UserVerificationEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_USER_VERIFICATION = "userVerification";
private UserVerificationEnum userVerification = UserVerificationEnum.OPTIONAL;
public PossessionConstraint() {
}
public PossessionConstraint authenticationMethods(List authenticationMethods) {
this.authenticationMethods = authenticationMethods;
return this;
}
public PossessionConstraint addauthenticationMethodsItem(AuthenticationMethodObject authenticationMethodsItem) {
if (this.authenticationMethods == null) {
this.authenticationMethods = new ArrayList<>();
}
this.authenticationMethods.add(authenticationMethodsItem);
return this;
}
/**
* This property specifies the precise authenticator and method for authentication. <x-lifecycle
* class=\"oie\"></x-lifecycle>
*
* @return authenticationMethods
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This property specifies the precise authenticator and method for authentication. ")
@JsonProperty(JSON_PROPERTY_AUTHENTICATION_METHODS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAuthenticationMethods() {
return authenticationMethods;
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATION_METHODS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthenticationMethods(List authenticationMethods) {
this.authenticationMethods = authenticationMethods;
}
public PossessionConstraint excludedAuthenticationMethods(
List excludedAuthenticationMethods) {
this.excludedAuthenticationMethods = excludedAuthenticationMethods;
return this;
}
public PossessionConstraint addexcludedAuthenticationMethodsItem(
AuthenticationMethodObject excludedAuthenticationMethodsItem) {
if (this.excludedAuthenticationMethods == null) {
this.excludedAuthenticationMethods = new ArrayList<>();
}
this.excludedAuthenticationMethods.add(excludedAuthenticationMethodsItem);
return this;
}
/**
* This property specifies the precise authenticator and method to exclude from authentication. <x-lifecycle
* class=\"oie\"></x-lifecycle>
*
* @return excludedAuthenticationMethods
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This property specifies the precise authenticator and method to exclude from authentication. ")
@JsonProperty(JSON_PROPERTY_EXCLUDED_AUTHENTICATION_METHODS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getExcludedAuthenticationMethods() {
return excludedAuthenticationMethods;
}
@JsonProperty(JSON_PROPERTY_EXCLUDED_AUTHENTICATION_METHODS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExcludedAuthenticationMethods(List excludedAuthenticationMethods) {
this.excludedAuthenticationMethods = excludedAuthenticationMethods;
}
public PossessionConstraint methods(List methods) {
this.methods = methods;
return this;
}
public PossessionConstraint addmethodsItem(MethodsEnum methodsItem) {
if (this.methods == null) {
this.methods = new ArrayList<>();
}
this.methods.add(methodsItem);
return this;
}
/**
* The Authenticator methods that are permitted
*
* @return methods
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Authenticator methods that are permitted")
@JsonProperty(JSON_PROPERTY_METHODS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMethods() {
return methods;
}
@JsonProperty(JSON_PROPERTY_METHODS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMethods(List methods) {
this.methods = methods;
}
public PossessionConstraint reauthenticateIn(String reauthenticateIn) {
this.reauthenticateIn = reauthenticateIn;
return this;
}
/**
* The duration after which the user must re-authenticate regardless of user activity. This re-authentication
* interval overrides the Verification Method object's `reauthenticateIn` interval. The supported
* values use ISO 8601 period format for recurring time intervals (for example, `PT1H`).
*
* @return reauthenticateIn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The duration after which the user must re-authenticate regardless of user activity. This re-authentication interval overrides the Verification Method object's `reauthenticateIn` interval. The supported values use ISO 8601 period format for recurring time intervals (for example, `PT1H`).")
@JsonProperty(JSON_PROPERTY_REAUTHENTICATE_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getReauthenticateIn() {
return reauthenticateIn;
}
@JsonProperty(JSON_PROPERTY_REAUTHENTICATE_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReauthenticateIn(String reauthenticateIn) {
this.reauthenticateIn = reauthenticateIn;
}
public PossessionConstraint required(Boolean required) {
this.required = required;
return this;
}
/**
* This property indicates whether the knowledge or possession factor is required by the assurance. It's
* optional in the request, but is always returned in the response. By default, this field is `true`. If
* the knowledge or possession constraint has values for `excludedAuthenticationMethods` the
* `required` value is false. <x-lifecycle class=\"oie\"></x-lifecycle>
*
* @return required
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This property indicates whether the knowledge or possession factor is required by the assurance. It's optional in the request, but is always returned in the response. By default, this field is `true`. If the knowledge or possession constraint has values for `excludedAuthenticationMethods` the `required` value is false. ")
@JsonProperty(JSON_PROPERTY_REQUIRED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getRequired() {
return required;
}
@JsonProperty(JSON_PROPERTY_REQUIRED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRequired(Boolean required) {
this.required = required;
}
public PossessionConstraint types(List types) {
this.types = types;
return this;
}
public PossessionConstraint addtypesItem(TypesEnum typesItem) {
if (this.types == null) {
this.types = new ArrayList<>();
}
this.types.add(typesItem);
return this;
}
/**
* The Authenticator types that are permitted
*
* @return types
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Authenticator types that are permitted")
@JsonProperty(JSON_PROPERTY_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTypes() {
return types;
}
@JsonProperty(JSON_PROPERTY_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTypes(List types) {
this.types = types;
}
public PossessionConstraint deviceBound(DeviceBoundEnum deviceBound) {
this.deviceBound = deviceBound;
return this;
}
/**
* Indicates if device-bound Factors are required. This property is only set for `POSSESSION` constraints.
*
* @return deviceBound
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if device-bound Factors are required. This property is only set for `POSSESSION` constraints.")
@JsonProperty(JSON_PROPERTY_DEVICE_BOUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DeviceBoundEnum getDeviceBound() {
return deviceBound;
}
@JsonProperty(JSON_PROPERTY_DEVICE_BOUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeviceBound(DeviceBoundEnum deviceBound) {
this.deviceBound = deviceBound;
}
public PossessionConstraint hardwareProtection(HardwareProtectionEnum hardwareProtection) {
this.hardwareProtection = hardwareProtection;
return this;
}
/**
* Indicates if any secrets or private keys used during authentication must be hardware protected and not
* exportable. This property is only set for `POSSESSION` constraints.
*
* @return hardwareProtection
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if any secrets or private keys used during authentication must be hardware protected and not exportable. This property is only set for `POSSESSION` constraints.")
@JsonProperty(JSON_PROPERTY_HARDWARE_PROTECTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HardwareProtectionEnum getHardwareProtection() {
return hardwareProtection;
}
@JsonProperty(JSON_PROPERTY_HARDWARE_PROTECTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHardwareProtection(HardwareProtectionEnum hardwareProtection) {
this.hardwareProtection = hardwareProtection;
}
public PossessionConstraint phishingResistant(PhishingResistantEnum phishingResistant) {
this.phishingResistant = phishingResistant;
return this;
}
/**
* Indicates if phishing-resistant Factors are required. This property is only set for `POSSESSION`
* constraints.
*
* @return phishingResistant
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if phishing-resistant Factors are required. This property is only set for `POSSESSION` constraints.")
@JsonProperty(JSON_PROPERTY_PHISHING_RESISTANT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PhishingResistantEnum getPhishingResistant() {
return phishingResistant;
}
@JsonProperty(JSON_PROPERTY_PHISHING_RESISTANT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPhishingResistant(PhishingResistantEnum phishingResistant) {
this.phishingResistant = phishingResistant;
}
public PossessionConstraint userPresence(UserPresenceEnum userPresence) {
this.userPresence = userPresence;
return this;
}
/**
* Indicates if the user needs to approve an Okta Verify prompt or provide biometrics (meets NIST AAL2
* requirements). This property is only set for `POSSESSION` constraints.
*
* @return userPresence
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if the user needs to approve an Okta Verify prompt or provide biometrics (meets NIST AAL2 requirements). This property is only set for `POSSESSION` constraints.")
@JsonProperty(JSON_PROPERTY_USER_PRESENCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserPresenceEnum getUserPresence() {
return userPresence;
}
@JsonProperty(JSON_PROPERTY_USER_PRESENCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserPresence(UserPresenceEnum userPresence) {
this.userPresence = userPresence;
}
public PossessionConstraint userVerification(UserVerificationEnum userVerification) {
this.userVerification = userVerification;
return this;
}
/**
* Indicates the user interaction requirement (PIN or biometrics) to ensure verification of a possession factor
*
* @return userVerification
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates the user interaction requirement (PIN or biometrics) to ensure verification of a possession factor")
@JsonProperty(JSON_PROPERTY_USER_VERIFICATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserVerificationEnum getUserVerification() {
return userVerification;
}
@JsonProperty(JSON_PROPERTY_USER_VERIFICATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserVerification(UserVerificationEnum userVerification) {
this.userVerification = userVerification;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PossessionConstraint possessionConstraint = (PossessionConstraint) o;
return Objects.equals(this.authenticationMethods, possessionConstraint.authenticationMethods)
&& Objects.equals(this.excludedAuthenticationMethods,
possessionConstraint.excludedAuthenticationMethods)
&& Objects.equals(this.methods, possessionConstraint.methods)
&& Objects.equals(this.reauthenticateIn, possessionConstraint.reauthenticateIn)
&& Objects.equals(this.required, possessionConstraint.required)
&& Objects.equals(this.types, possessionConstraint.types)
&& Objects.equals(this.deviceBound, possessionConstraint.deviceBound)
&& Objects.equals(this.hardwareProtection, possessionConstraint.hardwareProtection)
&& Objects.equals(this.phishingResistant, possessionConstraint.phishingResistant)
&& Objects.equals(this.userPresence, possessionConstraint.userPresence)
&& Objects.equals(this.userVerification, possessionConstraint.userVerification);
// ;
}
@Override
public int hashCode() {
return Objects.hash(authenticationMethods, excludedAuthenticationMethods, methods, reauthenticateIn, required,
types, deviceBound, hardwareProtection, phishingResistant, userPresence, userVerification);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PossessionConstraint {\n");
sb.append(" authenticationMethods: ").append(toIndentedString(authenticationMethods)).append("\n");
sb.append(" excludedAuthenticationMethods: ").append(toIndentedString(excludedAuthenticationMethods))
.append("\n");
sb.append(" methods: ").append(toIndentedString(methods)).append("\n");
sb.append(" reauthenticateIn: ").append(toIndentedString(reauthenticateIn)).append("\n");
sb.append(" required: ").append(toIndentedString(required)).append("\n");
sb.append(" types: ").append(toIndentedString(types)).append("\n");
sb.append(" deviceBound: ").append(toIndentedString(deviceBound)).append("\n");
sb.append(" hardwareProtection: ").append(toIndentedString(hardwareProtection)).append("\n");
sb.append(" phishingResistant: ").append(toIndentedString(phishingResistant)).append("\n");
sb.append(" userPresence: ").append(toIndentedString(userPresence)).append("\n");
sb.append(" userVerification: ").append(toIndentedString(userVerification)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy