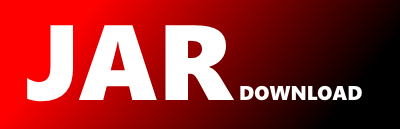
com.okta.sdk.resource.model.ProfileEnrollmentPolicyRuleAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.PreRegistrationInlineHook;
import com.okta.sdk.resource.model.ProfileEnrollmentPolicyRuleActivationRequirement;
import com.okta.sdk.resource.model.ProfileEnrollmentPolicyRuleProfileAttribute;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* ProfileEnrollmentPolicyRuleAction
*/
@JsonPropertyOrder({ ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_ACCESS,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_ACTIVATION_REQUIREMENTS,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_ALLOWED_IDENTIFIERS,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_ENROLL_AUTHENTICATOR_TYPES,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_PRE_REGISTRATION_INLINE_HOOKS,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_PROFILE_ATTRIBUTES,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_PROGRESSIVE_PROFILING_ACTION,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_TARGET_GROUP_IDS,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_UI_SCHEMA_ID,
ProfileEnrollmentPolicyRuleAction.JSON_PROPERTY_UNKNOWN_USER_ACTION })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ProfileEnrollmentPolicyRuleAction implements Serializable {
private static final long serialVersionUID = 1L;
/**
* **Note:** The Profile Enrollment Action object can't be modified to set the `access` property to
* `DENY` after the policy is created.
*/
public enum AccessEnum {
ALLOW("ALLOW"),
DENY("DENY"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
AccessEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static AccessEnum fromValue(String value) {
for (AccessEnum b : AccessEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_ACCESS = "access";
private AccessEnum access;
public static final String JSON_PROPERTY_ACTIVATION_REQUIREMENTS = "activationRequirements";
private ProfileEnrollmentPolicyRuleActivationRequirement activationRequirements;
public static final String JSON_PROPERTY_ALLOWED_IDENTIFIERS = "allowedIdentifiers";
private List allowedIdentifiers = null;
public static final String JSON_PROPERTY_ENROLL_AUTHENTICATOR_TYPES = "enrollAuthenticatorTypes";
private List enrollAuthenticatorTypes = null;
public static final String JSON_PROPERTY_PRE_REGISTRATION_INLINE_HOOKS = "preRegistrationInlineHooks";
private List preRegistrationInlineHooks = null;
public static final String JSON_PROPERTY_PROFILE_ATTRIBUTES = "profileAttributes";
private List profileAttributes = null;
/**
* Progressive profile enrollment helps evaluate the profile enrollment policy at every user login. Users can be
* prompted to provide input for newly required attributes.
*/
public enum ProgressiveProfilingActionEnum {
ENABLED("ENABLED"),
DISABLED("DISABLED"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ProgressiveProfilingActionEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ProgressiveProfilingActionEnum fromValue(String value) {
for (ProgressiveProfilingActionEnum b : ProgressiveProfilingActionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_PROGRESSIVE_PROFILING_ACTION = "progressiveProfilingAction";
private ProgressiveProfilingActionEnum progressiveProfilingAction;
public static final String JSON_PROPERTY_TARGET_GROUP_IDS = "targetGroupIds";
private List targetGroupIds = null;
public static final String JSON_PROPERTY_UI_SCHEMA_ID = "uiSchemaId";
private String uiSchemaId;
/**
* Which action should be taken if this User is new
*/
public enum UnknownUserActionEnum {
DENY("DENY"),
REGISTER("REGISTER"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
UnknownUserActionEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static UnknownUserActionEnum fromValue(String value) {
for (UnknownUserActionEnum b : UnknownUserActionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_UNKNOWN_USER_ACTION = "unknownUserAction";
private UnknownUserActionEnum unknownUserAction;
public ProfileEnrollmentPolicyRuleAction() {
}
public ProfileEnrollmentPolicyRuleAction access(AccessEnum access) {
this.access = access;
return this;
}
/**
* **Note:** The Profile Enrollment Action object can't be modified to set the `access` property to
* `DENY` after the policy is created.
*
* @return access
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "**Note:** The Profile Enrollment Action object can't be modified to set the `access` property to `DENY` after the policy is created.")
@JsonProperty(JSON_PROPERTY_ACCESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AccessEnum getAccess() {
return access;
}
@JsonProperty(JSON_PROPERTY_ACCESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccess(AccessEnum access) {
this.access = access;
}
public ProfileEnrollmentPolicyRuleAction activationRequirements(
ProfileEnrollmentPolicyRuleActivationRequirement activationRequirements) {
this.activationRequirements = activationRequirements;
return this;
}
/**
* Get activationRequirements
*
* @return activationRequirements
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACTIVATION_REQUIREMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProfileEnrollmentPolicyRuleActivationRequirement getActivationRequirements() {
return activationRequirements;
}
@JsonProperty(JSON_PROPERTY_ACTIVATION_REQUIREMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActivationRequirements(ProfileEnrollmentPolicyRuleActivationRequirement activationRequirements) {
this.activationRequirements = activationRequirements;
}
public ProfileEnrollmentPolicyRuleAction allowedIdentifiers(List allowedIdentifiers) {
this.allowedIdentifiers = allowedIdentifiers;
return this;
}
public ProfileEnrollmentPolicyRuleAction addallowedIdentifiersItem(String allowedIdentifiersItem) {
if (this.allowedIdentifiers == null) {
this.allowedIdentifiers = new ArrayList<>(Arrays.asList("login"));
}
this.allowedIdentifiers.add(allowedIdentifiersItem);
return this;
}
/**
* <x-lifecycle class=\"ea\"></x-lifecycle> A list of attributes to identify an end user.
* Can be used across Okta sign-in, unlock, and recovery flows.
*
* @return allowedIdentifiers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = " A list of attributes to identify an end user. Can be used across Okta sign-in, unlock, and recovery flows.")
@JsonProperty(JSON_PROPERTY_ALLOWED_IDENTIFIERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAllowedIdentifiers() {
return allowedIdentifiers;
}
@JsonProperty(JSON_PROPERTY_ALLOWED_IDENTIFIERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAllowedIdentifiers(List allowedIdentifiers) {
this.allowedIdentifiers = allowedIdentifiers;
}
public ProfileEnrollmentPolicyRuleAction enrollAuthenticatorTypes(List enrollAuthenticatorTypes) {
this.enrollAuthenticatorTypes = enrollAuthenticatorTypes;
return this;
}
public ProfileEnrollmentPolicyRuleAction addenrollAuthenticatorTypesItem(String enrollAuthenticatorTypesItem) {
if (this.enrollAuthenticatorTypes == null) {
this.enrollAuthenticatorTypes = new ArrayList<>();
}
this.enrollAuthenticatorTypes.add(enrollAuthenticatorTypesItem);
return this;
}
/**
* Additional authenticator fields that can be used on the first page of user registration. Valid values only
* includes `'password'`.
*
* @return enrollAuthenticatorTypes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Additional authenticator fields that can be used on the first page of user registration. Valid values only includes `'password'`.")
@JsonProperty(JSON_PROPERTY_ENROLL_AUTHENTICATOR_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getEnrollAuthenticatorTypes() {
return enrollAuthenticatorTypes;
}
@JsonProperty(JSON_PROPERTY_ENROLL_AUTHENTICATOR_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnrollAuthenticatorTypes(List enrollAuthenticatorTypes) {
this.enrollAuthenticatorTypes = enrollAuthenticatorTypes;
}
public ProfileEnrollmentPolicyRuleAction preRegistrationInlineHooks(
List preRegistrationInlineHooks) {
this.preRegistrationInlineHooks = preRegistrationInlineHooks;
return this;
}
public ProfileEnrollmentPolicyRuleAction addpreRegistrationInlineHooksItem(
PreRegistrationInlineHook preRegistrationInlineHooksItem) {
if (this.preRegistrationInlineHooks == null) {
this.preRegistrationInlineHooks = new ArrayList<>();
}
this.preRegistrationInlineHooks.add(preRegistrationInlineHooksItem);
return this;
}
/**
* (Optional) The `id` of at most one registration inline hook
*
* @return preRegistrationInlineHooks
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "(Optional) The `id` of at most one registration inline hook")
@JsonProperty(JSON_PROPERTY_PRE_REGISTRATION_INLINE_HOOKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getPreRegistrationInlineHooks() {
return preRegistrationInlineHooks;
}
@JsonProperty(JSON_PROPERTY_PRE_REGISTRATION_INLINE_HOOKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPreRegistrationInlineHooks(List preRegistrationInlineHooks) {
this.preRegistrationInlineHooks = preRegistrationInlineHooks;
}
public ProfileEnrollmentPolicyRuleAction profileAttributes(
List profileAttributes) {
this.profileAttributes = profileAttributes;
return this;
}
public ProfileEnrollmentPolicyRuleAction addprofileAttributesItem(
ProfileEnrollmentPolicyRuleProfileAttribute profileAttributesItem) {
if (this.profileAttributes == null) {
this.profileAttributes = new ArrayList<>();
}
this.profileAttributes.add(profileAttributesItem);
return this;
}
/**
* A list of attributes to prompt the user during registration or progressive profiling. Where defined on the User
* schema, these attributes are persisted in the User profile. Non-schema attributes can also be added, which
* aren't persisted to the User's profile, but are included in requests to the registration inline hook. A
* maximum of 10 Profile properties is supported.
*
* @return profileAttributes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of attributes to prompt the user during registration or progressive profiling. Where defined on the User schema, these attributes are persisted in the User profile. Non-schema attributes can also be added, which aren't persisted to the User's profile, but are included in requests to the registration inline hook. A maximum of 10 Profile properties is supported.")
@JsonProperty(JSON_PROPERTY_PROFILE_ATTRIBUTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getProfileAttributes() {
return profileAttributes;
}
@JsonProperty(JSON_PROPERTY_PROFILE_ATTRIBUTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProfileAttributes(List profileAttributes) {
this.profileAttributes = profileAttributes;
}
public ProfileEnrollmentPolicyRuleAction progressiveProfilingAction(
ProgressiveProfilingActionEnum progressiveProfilingAction) {
this.progressiveProfilingAction = progressiveProfilingAction;
return this;
}
/**
* Progressive profile enrollment helps evaluate the profile enrollment policy at every user login. Users can be
* prompted to provide input for newly required attributes.
*
* @return progressiveProfilingAction
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Progressive profile enrollment helps evaluate the profile enrollment policy at every user login. Users can be prompted to provide input for newly required attributes.")
@JsonProperty(JSON_PROPERTY_PROGRESSIVE_PROFILING_ACTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProgressiveProfilingActionEnum getProgressiveProfilingAction() {
return progressiveProfilingAction;
}
@JsonProperty(JSON_PROPERTY_PROGRESSIVE_PROFILING_ACTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProgressiveProfilingAction(ProgressiveProfilingActionEnum progressiveProfilingAction) {
this.progressiveProfilingAction = progressiveProfilingAction;
}
public ProfileEnrollmentPolicyRuleAction targetGroupIds(List targetGroupIds) {
this.targetGroupIds = targetGroupIds;
return this;
}
public ProfileEnrollmentPolicyRuleAction addtargetGroupIdsItem(String targetGroupIdsItem) {
if (this.targetGroupIds == null) {
this.targetGroupIds = new ArrayList<>();
}
this.targetGroupIds.add(targetGroupIdsItem);
return this;
}
/**
* (Optional, max 1 entry) The `id` of a Group that this User should be added to
*
* @return targetGroupIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "(Optional, max 1 entry) The `id` of a Group that this User should be added to")
@JsonProperty(JSON_PROPERTY_TARGET_GROUP_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTargetGroupIds() {
return targetGroupIds;
}
@JsonProperty(JSON_PROPERTY_TARGET_GROUP_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTargetGroupIds(List targetGroupIds) {
this.targetGroupIds = targetGroupIds;
}
public ProfileEnrollmentPolicyRuleAction uiSchemaId(String uiSchemaId) {
this.uiSchemaId = uiSchemaId;
return this;
}
/**
* Value created by the backend. If present, all policy updates must include this attribute/value.
*
* @return uiSchemaId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Value created by the backend. If present, all policy updates must include this attribute/value.")
@JsonProperty(JSON_PROPERTY_UI_SCHEMA_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUiSchemaId() {
return uiSchemaId;
}
@JsonProperty(JSON_PROPERTY_UI_SCHEMA_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUiSchemaId(String uiSchemaId) {
this.uiSchemaId = uiSchemaId;
}
public ProfileEnrollmentPolicyRuleAction unknownUserAction(UnknownUserActionEnum unknownUserAction) {
this.unknownUserAction = unknownUserAction;
return this;
}
/**
* Which action should be taken if this User is new
*
* @return unknownUserAction
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Which action should be taken if this User is new")
@JsonProperty(JSON_PROPERTY_UNKNOWN_USER_ACTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UnknownUserActionEnum getUnknownUserAction() {
return unknownUserAction;
}
@JsonProperty(JSON_PROPERTY_UNKNOWN_USER_ACTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUnknownUserAction(UnknownUserActionEnum unknownUserAction) {
this.unknownUserAction = unknownUserAction;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProfileEnrollmentPolicyRuleAction profileEnrollmentPolicyRuleAction = (ProfileEnrollmentPolicyRuleAction) o;
return Objects.equals(this.access, profileEnrollmentPolicyRuleAction.access)
&& Objects.equals(this.activationRequirements, profileEnrollmentPolicyRuleAction.activationRequirements)
&& Objects.equals(this.allowedIdentifiers, profileEnrollmentPolicyRuleAction.allowedIdentifiers)
&& Objects.equals(this.enrollAuthenticatorTypes,
profileEnrollmentPolicyRuleAction.enrollAuthenticatorTypes)
&& Objects.equals(this.preRegistrationInlineHooks,
profileEnrollmentPolicyRuleAction.preRegistrationInlineHooks)
&& Objects.equals(this.profileAttributes, profileEnrollmentPolicyRuleAction.profileAttributes)
&& Objects.equals(this.progressiveProfilingAction,
profileEnrollmentPolicyRuleAction.progressiveProfilingAction)
&& Objects.equals(this.targetGroupIds, profileEnrollmentPolicyRuleAction.targetGroupIds)
&& Objects.equals(this.uiSchemaId, profileEnrollmentPolicyRuleAction.uiSchemaId)
&& Objects.equals(this.unknownUserAction, profileEnrollmentPolicyRuleAction.unknownUserAction);
// ;
}
@Override
public int hashCode() {
return Objects.hash(access, activationRequirements, allowedIdentifiers, enrollAuthenticatorTypes,
preRegistrationInlineHooks, profileAttributes, progressiveProfilingAction, targetGroupIds, uiSchemaId,
unknownUserAction);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ProfileEnrollmentPolicyRuleAction {\n");
sb.append(" access: ").append(toIndentedString(access)).append("\n");
sb.append(" activationRequirements: ").append(toIndentedString(activationRequirements)).append("\n");
sb.append(" allowedIdentifiers: ").append(toIndentedString(allowedIdentifiers)).append("\n");
sb.append(" enrollAuthenticatorTypes: ").append(toIndentedString(enrollAuthenticatorTypes)).append("\n");
sb.append(" preRegistrationInlineHooks: ").append(toIndentedString(preRegistrationInlineHooks)).append("\n");
sb.append(" profileAttributes: ").append(toIndentedString(profileAttributes)).append("\n");
sb.append(" progressiveProfilingAction: ").append(toIndentedString(progressiveProfilingAction)).append("\n");
sb.append(" targetGroupIds: ").append(toIndentedString(targetGroupIds)).append("\n");
sb.append(" uiSchemaId: ").append(toIndentedString(uiSchemaId)).append("\n");
sb.append(" unknownUserAction: ").append(toIndentedString(unknownUserAction)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy