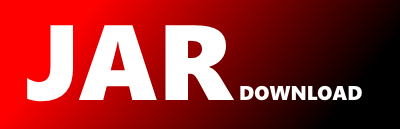
com.okta.sdk.resource.model.ProtocolEndpoints Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.ProtocolEndpoint;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* ProtocolEndpoints
*/
@JsonPropertyOrder({ ProtocolEndpoints.JSON_PROPERTY_ACS, ProtocolEndpoints.JSON_PROPERTY_AUTHORIZATION,
ProtocolEndpoints.JSON_PROPERTY_JWKS, ProtocolEndpoints.JSON_PROPERTY_METADATA,
ProtocolEndpoints.JSON_PROPERTY_SLO, ProtocolEndpoints.JSON_PROPERTY_SSO, ProtocolEndpoints.JSON_PROPERTY_TOKEN,
ProtocolEndpoints.JSON_PROPERTY_USER_INFO })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ProtocolEndpoints implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACS = "acs";
private ProtocolEndpoint acs;
public static final String JSON_PROPERTY_AUTHORIZATION = "authorization";
private ProtocolEndpoint authorization;
public static final String JSON_PROPERTY_JWKS = "jwks";
private ProtocolEndpoint jwks;
public static final String JSON_PROPERTY_METADATA = "metadata";
private ProtocolEndpoint metadata;
public static final String JSON_PROPERTY_SLO = "slo";
private ProtocolEndpoint slo;
public static final String JSON_PROPERTY_SSO = "sso";
private ProtocolEndpoint sso;
public static final String JSON_PROPERTY_TOKEN = "token";
private ProtocolEndpoint token;
public static final String JSON_PROPERTY_USER_INFO = "userInfo";
private ProtocolEndpoint userInfo;
public ProtocolEndpoints() {
}
public ProtocolEndpoints acs(ProtocolEndpoint acs) {
this.acs = acs;
return this;
}
/**
* Get acs
*
* @return acs
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getAcs() {
return acs;
}
@JsonProperty(JSON_PROPERTY_ACS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAcs(ProtocolEndpoint acs) {
this.acs = acs;
}
public ProtocolEndpoints authorization(ProtocolEndpoint authorization) {
this.authorization = authorization;
return this;
}
/**
* Get authorization
*
* @return authorization
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AUTHORIZATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getAuthorization() {
return authorization;
}
@JsonProperty(JSON_PROPERTY_AUTHORIZATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthorization(ProtocolEndpoint authorization) {
this.authorization = authorization;
}
public ProtocolEndpoints jwks(ProtocolEndpoint jwks) {
this.jwks = jwks;
return this;
}
/**
* Get jwks
*
* @return jwks
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_JWKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getJwks() {
return jwks;
}
@JsonProperty(JSON_PROPERTY_JWKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setJwks(ProtocolEndpoint jwks) {
this.jwks = jwks;
}
public ProtocolEndpoints metadata(ProtocolEndpoint metadata) {
this.metadata = metadata;
return this;
}
/**
* Get metadata
*
* @return metadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(ProtocolEndpoint metadata) {
this.metadata = metadata;
}
public ProtocolEndpoints slo(ProtocolEndpoint slo) {
this.slo = slo;
return this;
}
/**
* Get slo
*
* @return slo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SLO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getSlo() {
return slo;
}
@JsonProperty(JSON_PROPERTY_SLO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSlo(ProtocolEndpoint slo) {
this.slo = slo;
}
public ProtocolEndpoints sso(ProtocolEndpoint sso) {
this.sso = sso;
return this;
}
/**
* Get sso
*
* @return sso
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SSO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getSso() {
return sso;
}
@JsonProperty(JSON_PROPERTY_SSO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSso(ProtocolEndpoint sso) {
this.sso = sso;
}
public ProtocolEndpoints token(ProtocolEndpoint token) {
this.token = token;
return this;
}
/**
* Get token
*
* @return token
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getToken() {
return token;
}
@JsonProperty(JSON_PROPERTY_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setToken(ProtocolEndpoint token) {
this.token = token;
}
public ProtocolEndpoints userInfo(ProtocolEndpoint userInfo) {
this.userInfo = userInfo;
return this;
}
/**
* Get userInfo
*
* @return userInfo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_USER_INFO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ProtocolEndpoint getUserInfo() {
return userInfo;
}
@JsonProperty(JSON_PROPERTY_USER_INFO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserInfo(ProtocolEndpoint userInfo) {
this.userInfo = userInfo;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProtocolEndpoints protocolEndpoints = (ProtocolEndpoints) o;
return Objects.equals(this.acs, protocolEndpoints.acs)
&& Objects.equals(this.authorization, protocolEndpoints.authorization)
&& Objects.equals(this.jwks, protocolEndpoints.jwks)
&& Objects.equals(this.metadata, protocolEndpoints.metadata)
&& Objects.equals(this.slo, protocolEndpoints.slo) && Objects.equals(this.sso, protocolEndpoints.sso)
&& Objects.equals(this.token, protocolEndpoints.token)
&& Objects.equals(this.userInfo, protocolEndpoints.userInfo);
// ;
}
@Override
public int hashCode() {
return Objects.hash(acs, authorization, jwks, metadata, slo, sso, token, userInfo);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ProtocolEndpoints {\n");
sb.append(" acs: ").append(toIndentedString(acs)).append("\n");
sb.append(" authorization: ").append(toIndentedString(authorization)).append("\n");
sb.append(" jwks: ").append(toIndentedString(jwks)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" slo: ").append(toIndentedString(slo)).append("\n");
sb.append(" sso: ").append(toIndentedString(sso)).append("\n");
sb.append(" token: ").append(toIndentedString(token)).append("\n");
sb.append(" userInfo: ").append(toIndentedString(userInfo)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy