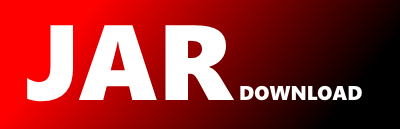
com.okta.sdk.resource.model.ProtocolSaml Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.SamlAlgorithms;
import com.okta.sdk.resource.model.SamlCredentials;
import com.okta.sdk.resource.model.SamlEndpoints;
import com.okta.sdk.resource.model.SamlRelayState;
import com.okta.sdk.resource.model.SamlSettings;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Protocol settings for the [SAML 2.0 Authentication Request
* Protocol](http://docs.oasis-open.org/security/saml/v2.0/saml-core-2.0-os.pdf)
*/
@ApiModel(description = "Protocol settings for the [SAML 2.0 Authentication Request Protocol](http://docs.oasis-open.org/security/saml/v2.0/saml-core-2.0-os.pdf)")
@JsonPropertyOrder({ ProtocolSaml.JSON_PROPERTY_ALGORITHMS, ProtocolSaml.JSON_PROPERTY_CREDENTIALS,
ProtocolSaml.JSON_PROPERTY_ENDPOINTS, ProtocolSaml.JSON_PROPERTY_RELAY_STATE,
ProtocolSaml.JSON_PROPERTY_SETTINGS, ProtocolSaml.JSON_PROPERTY_TYPE })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ProtocolSaml implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ALGORITHMS = "algorithms";
private SamlAlgorithms algorithms;
public static final String JSON_PROPERTY_CREDENTIALS = "credentials";
private SamlCredentials credentials;
public static final String JSON_PROPERTY_ENDPOINTS = "endpoints";
private SamlEndpoints endpoints;
public static final String JSON_PROPERTY_RELAY_STATE = "relayState";
private SamlRelayState relayState;
public static final String JSON_PROPERTY_SETTINGS = "settings";
private SamlSettings settings;
/**
* SAML 2.0 protocol
*/
public enum TypeEnum {
SAML2("SAML2"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_TYPE = "type";
private TypeEnum type;
public ProtocolSaml() {
}
public ProtocolSaml algorithms(SamlAlgorithms algorithms) {
this.algorithms = algorithms;
return this;
}
/**
* Get algorithms
*
* @return algorithms
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ALGORITHMS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SamlAlgorithms getAlgorithms() {
return algorithms;
}
@JsonProperty(JSON_PROPERTY_ALGORITHMS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAlgorithms(SamlAlgorithms algorithms) {
this.algorithms = algorithms;
}
public ProtocolSaml credentials(SamlCredentials credentials) {
this.credentials = credentials;
return this;
}
/**
* Get credentials
*
* @return credentials
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SamlCredentials getCredentials() {
return credentials;
}
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCredentials(SamlCredentials credentials) {
this.credentials = credentials;
}
public ProtocolSaml endpoints(SamlEndpoints endpoints) {
this.endpoints = endpoints;
return this;
}
/**
* Get endpoints
*
* @return endpoints
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENDPOINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SamlEndpoints getEndpoints() {
return endpoints;
}
@JsonProperty(JSON_PROPERTY_ENDPOINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEndpoints(SamlEndpoints endpoints) {
this.endpoints = endpoints;
}
public ProtocolSaml relayState(SamlRelayState relayState) {
this.relayState = relayState;
return this;
}
/**
* Get relayState
*
* @return relayState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_RELAY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SamlRelayState getRelayState() {
return relayState;
}
@JsonProperty(JSON_PROPERTY_RELAY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRelayState(SamlRelayState relayState) {
this.relayState = relayState;
}
public ProtocolSaml settings(SamlSettings settings) {
this.settings = settings;
return this;
}
/**
* Get settings
*
* @return settings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SETTINGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SamlSettings getSettings() {
return settings;
}
@JsonProperty(JSON_PROPERTY_SETTINGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSettings(SamlSettings settings) {
this.settings = settings;
}
public ProtocolSaml type(TypeEnum type) {
this.type = type;
return this;
}
/**
* SAML 2.0 protocol
*
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "SAML 2.0 protocol")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TypeEnum getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(TypeEnum type) {
this.type = type;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProtocolSaml protocolSaml = (ProtocolSaml) o;
return Objects.equals(this.algorithms, protocolSaml.algorithms)
&& Objects.equals(this.credentials, protocolSaml.credentials)
&& Objects.equals(this.endpoints, protocolSaml.endpoints)
&& Objects.equals(this.relayState, protocolSaml.relayState)
&& Objects.equals(this.settings, protocolSaml.settings) && Objects.equals(this.type, protocolSaml.type);
// ;
}
@Override
public int hashCode() {
return Objects.hash(algorithms, credentials, endpoints, relayState, settings, type);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ProtocolSaml {\n");
sb.append(" algorithms: ").append(toIndentedString(algorithms)).append("\n");
sb.append(" credentials: ").append(toIndentedString(credentials)).append("\n");
sb.append(" endpoints: ").append(toIndentedString(endpoints)).append("\n");
sb.append(" relayState: ").append(toIndentedString(relayState)).append("\n");
sb.append(" settings: ").append(toIndentedString(settings)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy