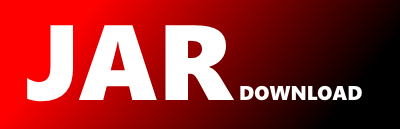
com.okta.sdk.resource.model.RealmAssignment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.Actions;
import com.okta.sdk.resource.model.Conditions;
import com.okta.sdk.resource.model.LifecycleStatus;
import com.okta.sdk.resource.model.LinksSelf;
import java.time.OffsetDateTime;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* RealmAssignment
*/
@JsonPropertyOrder({ RealmAssignment.JSON_PROPERTY_ACTIONS, RealmAssignment.JSON_PROPERTY_CONDITIONS,
RealmAssignment.JSON_PROPERTY_CREATED, RealmAssignment.JSON_PROPERTY_ID,
RealmAssignment.JSON_PROPERTY_IS_DEFAULT, RealmAssignment.JSON_PROPERTY_LAST_UPDATED,
RealmAssignment.JSON_PROPERTY_NAME, RealmAssignment.JSON_PROPERTY_PRIORITY,
RealmAssignment.JSON_PROPERTY_STATUS, RealmAssignment.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class RealmAssignment implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACTIONS = "actions";
private Actions actions;
public static final String JSON_PROPERTY_CONDITIONS = "conditions";
private Conditions conditions;
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IS_DEFAULT = "isDefault";
private Boolean isDefault;
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_PRIORITY = "priority";
private Integer priority;
public static final String JSON_PROPERTY_STATUS = "status";
private LifecycleStatus status;
public static final String JSON_PROPERTY_LINKS = "_links";
private LinksSelf links;
public RealmAssignment() {
}
/*
* @JsonCreator public RealmAssignment(
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_IS_DEFAULT) Boolean isDefault,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated ) { this(); this.created = created; this.id
* = id; this.isDefault = isDefault; this.lastUpdated = lastUpdated; }
*/
public RealmAssignment actions(Actions actions) {
this.actions = actions;
return this;
}
/**
* Get actions
*
* @return actions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Actions getActions() {
return actions;
}
@JsonProperty(JSON_PROPERTY_ACTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActions(Actions actions) {
this.actions = actions;
}
public RealmAssignment conditions(Conditions conditions) {
this.conditions = conditions;
return this;
}
/**
* Get conditions
*
* @return conditions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CONDITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Conditions getConditions() {
return conditions;
}
@JsonProperty(JSON_PROPERTY_CONDITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setConditions(Conditions conditions) {
this.conditions = conditions;
}
/**
* Get created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
/**
* Get id
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
/**
* Get isDefault
*
* @return isDefault
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_IS_DEFAULT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsDefault() {
return isDefault;
}
/**
* Get lastUpdated
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
public RealmAssignment name(String name) {
this.name = name;
return this;
}
/**
* Get name
*
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public RealmAssignment priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* Get priority
*
* @return priority
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PRIORITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPriority() {
return priority;
}
@JsonProperty(JSON_PROPERTY_PRIORITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPriority(Integer priority) {
this.priority = priority;
}
public RealmAssignment status(LifecycleStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LifecycleStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatus(LifecycleStatus status) {
this.status = status;
}
public RealmAssignment links(LinksSelf links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksSelf getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(LinksSelf links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RealmAssignment realmAssignment = (RealmAssignment) o;
return Objects.equals(this.actions, realmAssignment.actions)
&& Objects.equals(this.conditions, realmAssignment.conditions)
&& Objects.equals(this.created, realmAssignment.created) && Objects.equals(this.id, realmAssignment.id)
&& Objects.equals(this.isDefault, realmAssignment.isDefault)
&& Objects.equals(this.lastUpdated, realmAssignment.lastUpdated)
&& Objects.equals(this.name, realmAssignment.name)
&& Objects.equals(this.priority, realmAssignment.priority)
&& Objects.equals(this.status, realmAssignment.status)
&& Objects.equals(this.links, realmAssignment.links);
// ;
}
@Override
public int hashCode() {
return Objects.hash(actions, conditions, created, id, isDefault, lastUpdated, name, priority, status, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RealmAssignment {\n");
sb.append(" actions: ").append(toIndentedString(actions)).append("\n");
sb.append(" conditions: ").append(toIndentedString(conditions)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isDefault: ").append(toIndentedString(isDefault)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy