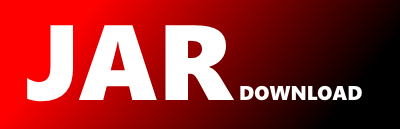
com.okta.sdk.resource.model.SAMLHookResponseCommandsInnerValueInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.SAMLHookResponseCommandsInnerValueInnerValue;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* SAMLHookResponseCommandsInnerValueInner
*/
@JsonPropertyOrder({ SAMLHookResponseCommandsInnerValueInner.JSON_PROPERTY_OP,
SAMLHookResponseCommandsInnerValueInner.JSON_PROPERTY_PATH,
SAMLHookResponseCommandsInnerValueInner.JSON_PROPERTY_VALUE })
@JsonTypeName("SAMLHookResponse_commands_inner_value_inner")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class SAMLHookResponseCommandsInnerValueInner implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_OP = "op";
private String op;
public static final String JSON_PROPERTY_PATH = "path";
private String path;
public static final String JSON_PROPERTY_VALUE = "value";
private SAMLHookResponseCommandsInnerValueInnerValue value;
public SAMLHookResponseCommandsInnerValueInner() {
}
public SAMLHookResponseCommandsInnerValueInner op(String op) {
this.op = op;
return this;
}
/**
* The name of one of the supported ops: `add`: Add a new claim to the assertion `replace`:
* Modify any element of the assertion > **Note:** If a response to the SAML assertion inline hook request
* isn't received from your external service within three seconds, a timeout occurs. In this scenario, the Okta
* process flow continues with the original SAML assertion returned.
*
* @return op
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of one of the supported ops: `add`: Add a new claim to the assertion `replace`: Modify any element of the assertion > **Note:** If a response to the SAML assertion inline hook request isn't received from your external service within three seconds, a timeout occurs. In this scenario, the Okta process flow continues with the original SAML assertion returned.")
@JsonProperty(JSON_PROPERTY_OP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOp() {
return op;
}
@JsonProperty(JSON_PROPERTY_OP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOp(String op) {
this.op = op;
}
public SAMLHookResponseCommandsInnerValueInner path(String path) {
this.path = path;
return this;
}
/**
* Location, within the assertion, to apply the operation
*
* @return path
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Location, within the assertion, to apply the operation")
@JsonProperty(JSON_PROPERTY_PATH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPath() {
return path;
}
@JsonProperty(JSON_PROPERTY_PATH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPath(String path) {
this.path = path;
}
public SAMLHookResponseCommandsInnerValueInner value(SAMLHookResponseCommandsInnerValueInnerValue value) {
this.value = value;
return this;
}
/**
* Get value
*
* @return value
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SAMLHookResponseCommandsInnerValueInnerValue getValue() {
return value;
}
@JsonProperty(JSON_PROPERTY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setValue(SAMLHookResponseCommandsInnerValueInnerValue value) {
this.value = value;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SAMLHookResponseCommandsInnerValueInner saMLHookResponseCommandsInnerValueInner = (SAMLHookResponseCommandsInnerValueInner) o;
return Objects.equals(this.op, saMLHookResponseCommandsInnerValueInner.op)
&& Objects.equals(this.path, saMLHookResponseCommandsInnerValueInner.path)
&& Objects.equals(this.value, saMLHookResponseCommandsInnerValueInner.value);
// ;
}
@Override
public int hashCode() {
return Objects.hash(op, path, value);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SAMLHookResponseCommandsInnerValueInner {\n");
sb.append(" op: ").append(toIndentedString(op)).append("\n");
sb.append(" path: ").append(toIndentedString(path)).append("\n");
sb.append(" value: ").append(toIndentedString(value)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy