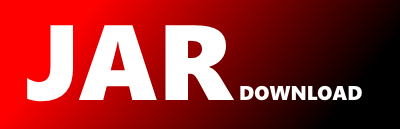
com.okta.sdk.resource.model.SamlApplicationSettingsSignOn Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.AcsEndpoint;
import com.okta.sdk.resource.model.SamlAttributeStatement;
import com.okta.sdk.resource.model.SamlSpCertificate;
import com.okta.sdk.resource.model.SignOnInlineHook;
import com.okta.sdk.resource.model.SingleLogout;
import com.okta.sdk.resource.model.SloParticipate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* SAML 2.0 sign-on attributes. > **Note:** Set either `destinationOverride` or `ssoAcsUrl` to
* configure any other SAML 2.0 attributes in this section.
*/
@ApiModel(description = "SAML 2.0 sign-on attributes. > **Note:** Set either `destinationOverride` or `ssoAcsUrl` to configure any other SAML 2.0 attributes in this section.")
@JsonPropertyOrder({ SamlApplicationSettingsSignOn.JSON_PROPERTY_ACS_ENDPOINTS,
SamlApplicationSettingsSignOn.JSON_PROPERTY_ALLOW_MULTIPLE_ACS_ENDPOINTS,
SamlApplicationSettingsSignOn.JSON_PROPERTY_ASSERTION_SIGNED,
SamlApplicationSettingsSignOn.JSON_PROPERTY_ATTRIBUTE_STATEMENTS,
SamlApplicationSettingsSignOn.JSON_PROPERTY_AUDIENCE,
SamlApplicationSettingsSignOn.JSON_PROPERTY_AUDIENCE_OVERRIDE,
SamlApplicationSettingsSignOn.JSON_PROPERTY_AUTHN_CONTEXT_CLASS_REF,
SamlApplicationSettingsSignOn.JSON_PROPERTY_CONFIGURED_ATTRIBUTE_STATEMENTS,
SamlApplicationSettingsSignOn.JSON_PROPERTY_DEFAULT_RELAY_STATE,
SamlApplicationSettingsSignOn.JSON_PROPERTY_DESTINATION,
SamlApplicationSettingsSignOn.JSON_PROPERTY_DESTINATION_OVERRIDE,
SamlApplicationSettingsSignOn.JSON_PROPERTY_DIGEST_ALGORITHM,
SamlApplicationSettingsSignOn.JSON_PROPERTY_HONOR_FORCE_AUTHN,
SamlApplicationSettingsSignOn.JSON_PROPERTY_IDP_ISSUER,
SamlApplicationSettingsSignOn.JSON_PROPERTY_INLINE_HOOKS,
SamlApplicationSettingsSignOn.JSON_PROPERTY_PARTICIPATE_SLO,
SamlApplicationSettingsSignOn.JSON_PROPERTY_RECIPIENT,
SamlApplicationSettingsSignOn.JSON_PROPERTY_RECIPIENT_OVERRIDE,
SamlApplicationSettingsSignOn.JSON_PROPERTY_REQUEST_COMPRESSED,
SamlApplicationSettingsSignOn.JSON_PROPERTY_RESPONSE_SIGNED,
SamlApplicationSettingsSignOn.JSON_PROPERTY_SAML_ASSERTION_LIFETIME_SECONDS,
SamlApplicationSettingsSignOn.JSON_PROPERTY_SIGNATURE_ALGORITHM,
SamlApplicationSettingsSignOn.JSON_PROPERTY_SLO, SamlApplicationSettingsSignOn.JSON_PROPERTY_SP_CERTIFICATE,
SamlApplicationSettingsSignOn.JSON_PROPERTY_SP_ISSUER, SamlApplicationSettingsSignOn.JSON_PROPERTY_SSO_ACS_URL,
SamlApplicationSettingsSignOn.JSON_PROPERTY_SSO_ACS_URL_OVERRIDE,
SamlApplicationSettingsSignOn.JSON_PROPERTY_SUBJECT_NAME_ID_FORMAT,
SamlApplicationSettingsSignOn.JSON_PROPERTY_SUBJECT_NAME_ID_TEMPLATE })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class SamlApplicationSettingsSignOn implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACS_ENDPOINTS = "acsEndpoints";
private List acsEndpoints = null;
public static final String JSON_PROPERTY_ALLOW_MULTIPLE_ACS_ENDPOINTS = "allowMultipleAcsEndpoints";
private Boolean allowMultipleAcsEndpoints;
public static final String JSON_PROPERTY_ASSERTION_SIGNED = "assertionSigned";
private Boolean assertionSigned;
public static final String JSON_PROPERTY_ATTRIBUTE_STATEMENTS = "attributeStatements";
private List attributeStatements = null;
public static final String JSON_PROPERTY_AUDIENCE = "audience";
private String audience;
public static final String JSON_PROPERTY_AUDIENCE_OVERRIDE = "audienceOverride";
private String audienceOverride;
public static final String JSON_PROPERTY_AUTHN_CONTEXT_CLASS_REF = "authnContextClassRef";
private String authnContextClassRef;
public static final String JSON_PROPERTY_CONFIGURED_ATTRIBUTE_STATEMENTS = "configuredAttributeStatements";
private List configuredAttributeStatements = null;
public static final String JSON_PROPERTY_DEFAULT_RELAY_STATE = "defaultRelayState";
private String defaultRelayState;
public static final String JSON_PROPERTY_DESTINATION = "destination";
private String destination;
public static final String JSON_PROPERTY_DESTINATION_OVERRIDE = "destinationOverride";
private String destinationOverride;
public static final String JSON_PROPERTY_DIGEST_ALGORITHM = "digestAlgorithm";
private String digestAlgorithm;
public static final String JSON_PROPERTY_HONOR_FORCE_AUTHN = "honorForceAuthn";
private Boolean honorForceAuthn;
public static final String JSON_PROPERTY_IDP_ISSUER = "idpIssuer";
private String idpIssuer;
public static final String JSON_PROPERTY_INLINE_HOOKS = "inlineHooks";
private List inlineHooks = null;
public static final String JSON_PROPERTY_PARTICIPATE_SLO = "participateSlo";
private SloParticipate participateSlo;
public static final String JSON_PROPERTY_RECIPIENT = "recipient";
private String recipient;
public static final String JSON_PROPERTY_RECIPIENT_OVERRIDE = "recipientOverride";
private String recipientOverride;
public static final String JSON_PROPERTY_REQUEST_COMPRESSED = "requestCompressed";
private Boolean requestCompressed;
public static final String JSON_PROPERTY_RESPONSE_SIGNED = "responseSigned";
private Boolean responseSigned;
public static final String JSON_PROPERTY_SAML_ASSERTION_LIFETIME_SECONDS = "samlAssertionLifetimeSeconds";
private Integer samlAssertionLifetimeSeconds;
public static final String JSON_PROPERTY_SIGNATURE_ALGORITHM = "signatureAlgorithm";
private String signatureAlgorithm;
public static final String JSON_PROPERTY_SLO = "slo";
private SingleLogout slo;
public static final String JSON_PROPERTY_SP_CERTIFICATE = "spCertificate";
private SamlSpCertificate spCertificate;
public static final String JSON_PROPERTY_SP_ISSUER = "spIssuer";
private String spIssuer;
public static final String JSON_PROPERTY_SSO_ACS_URL = "ssoAcsUrl";
private String ssoAcsUrl;
public static final String JSON_PROPERTY_SSO_ACS_URL_OVERRIDE = "ssoAcsUrlOverride";
private String ssoAcsUrlOverride;
public static final String JSON_PROPERTY_SUBJECT_NAME_ID_FORMAT = "subjectNameIdFormat";
private String subjectNameIdFormat;
public static final String JSON_PROPERTY_SUBJECT_NAME_ID_TEMPLATE = "subjectNameIdTemplate";
private String subjectNameIdTemplate;
public SamlApplicationSettingsSignOn() {
}
public SamlApplicationSettingsSignOn acsEndpoints(List acsEndpoints) {
this.acsEndpoints = acsEndpoints;
return this;
}
public SamlApplicationSettingsSignOn addacsEndpointsItem(AcsEndpoint acsEndpointsItem) {
if (this.acsEndpoints == null) {
this.acsEndpoints = new ArrayList<>();
}
this.acsEndpoints.add(acsEndpointsItem);
return this;
}
/**
* An array of ACS endpoints. You can configure a maximum of 100 endpoints.
*
* @return acsEndpoints
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of ACS endpoints. You can configure a maximum of 100 endpoints.")
@JsonProperty(JSON_PROPERTY_ACS_ENDPOINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAcsEndpoints() {
return acsEndpoints;
}
@JsonProperty(JSON_PROPERTY_ACS_ENDPOINTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAcsEndpoints(List acsEndpoints) {
this.acsEndpoints = acsEndpoints;
}
public SamlApplicationSettingsSignOn allowMultipleAcsEndpoints(Boolean allowMultipleAcsEndpoints) {
this.allowMultipleAcsEndpoints = allowMultipleAcsEndpoints;
return this;
}
/**
* Determines whether the app allows you to configure multiple ACS URIs
*
* @return allowMultipleAcsEndpoints
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Determines whether the app allows you to configure multiple ACS URIs")
@JsonProperty(JSON_PROPERTY_ALLOW_MULTIPLE_ACS_ENDPOINTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getAllowMultipleAcsEndpoints() {
return allowMultipleAcsEndpoints;
}
@JsonProperty(JSON_PROPERTY_ALLOW_MULTIPLE_ACS_ENDPOINTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAllowMultipleAcsEndpoints(Boolean allowMultipleAcsEndpoints) {
this.allowMultipleAcsEndpoints = allowMultipleAcsEndpoints;
}
public SamlApplicationSettingsSignOn assertionSigned(Boolean assertionSigned) {
this.assertionSigned = assertionSigned;
return this;
}
/**
* Determines whether the SAML assertion is digitally signed
*
* @return assertionSigned
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Determines whether the SAML assertion is digitally signed")
@JsonProperty(JSON_PROPERTY_ASSERTION_SIGNED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getAssertionSigned() {
return assertionSigned;
}
@JsonProperty(JSON_PROPERTY_ASSERTION_SIGNED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAssertionSigned(Boolean assertionSigned) {
this.assertionSigned = assertionSigned;
}
public SamlApplicationSettingsSignOn attributeStatements(List attributeStatements) {
this.attributeStatements = attributeStatements;
return this;
}
public SamlApplicationSettingsSignOn addattributeStatementsItem(SamlAttributeStatement attributeStatementsItem) {
if (this.attributeStatements == null) {
this.attributeStatements = new ArrayList<>();
}
this.attributeStatements.add(attributeStatementsItem);
return this;
}
/**
* A list of custom attribute statements for the app's SAML assertion. See [SAML 2.0 Technical
* Overview](https://docs.oasis-open.org/security/saml/Post2.0/sstc-saml-tech-overview-2.0-cd-02.html). There are
* two types of attribute statements: | Type | Description | | ---- | ----------- | | EXPRESSION | Generic attribute
* statement that can be dynamic and supports [Okta Expression
* Language](https://developer.okta.com/docs/reference/okta-expression-language/) | | GROUP | Group attribute
* statement |
*
* @return attributeStatements
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of custom attribute statements for the app's SAML assertion. See [SAML 2.0 Technical Overview](https://docs.oasis-open.org/security/saml/Post2.0/sstc-saml-tech-overview-2.0-cd-02.html). There are two types of attribute statements: | Type | Description | | ---- | ----------- | | EXPRESSION | Generic attribute statement that can be dynamic and supports [Okta Expression Language](https://developer.okta.com/docs/reference/okta-expression-language/) | | GROUP | Group attribute statement | ")
@JsonProperty(JSON_PROPERTY_ATTRIBUTE_STATEMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAttributeStatements() {
return attributeStatements;
}
@JsonProperty(JSON_PROPERTY_ATTRIBUTE_STATEMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAttributeStatements(List attributeStatements) {
this.attributeStatements = attributeStatements;
}
public SamlApplicationSettingsSignOn audience(String audience) {
this.audience = audience;
return this;
}
/**
* The entity ID of the SP. Use the entity ID value exactly as provided by the SP.
*
* @return audience
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The entity ID of the SP. Use the entity ID value exactly as provided by the SP.")
@JsonProperty(JSON_PROPERTY_AUDIENCE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAudience() {
return audience;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAudience(String audience) {
this.audience = audience;
}
public SamlApplicationSettingsSignOn audienceOverride(String audienceOverride) {
this.audienceOverride = audienceOverride;
return this;
}
/**
* Audience override for CASB configuration. See [CASB config
* guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).
*
* @return audienceOverride
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Audience override for CASB configuration. See [CASB config guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).")
@JsonProperty(JSON_PROPERTY_AUDIENCE_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAudienceOverride() {
return audienceOverride;
}
@JsonProperty(JSON_PROPERTY_AUDIENCE_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAudienceOverride(String audienceOverride) {
this.audienceOverride = audienceOverride;
}
public SamlApplicationSettingsSignOn authnContextClassRef(String authnContextClassRef) {
this.authnContextClassRef = authnContextClassRef;
return this;
}
/**
* Identifies the SAML authentication context class for the assertion's authentication statement
*
* @return authnContextClassRef
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Identifies the SAML authentication context class for the assertion's authentication statement")
@JsonProperty(JSON_PROPERTY_AUTHN_CONTEXT_CLASS_REF)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAuthnContextClassRef() {
return authnContextClassRef;
}
@JsonProperty(JSON_PROPERTY_AUTHN_CONTEXT_CLASS_REF)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAuthnContextClassRef(String authnContextClassRef) {
this.authnContextClassRef = authnContextClassRef;
}
public SamlApplicationSettingsSignOn configuredAttributeStatements(
List configuredAttributeStatements) {
this.configuredAttributeStatements = configuredAttributeStatements;
return this;
}
public SamlApplicationSettingsSignOn addconfiguredAttributeStatementsItem(
SamlAttributeStatement configuredAttributeStatementsItem) {
if (this.configuredAttributeStatements == null) {
this.configuredAttributeStatements = new ArrayList<>();
}
this.configuredAttributeStatements.add(configuredAttributeStatementsItem);
return this;
}
/**
* The list of dynamic attribute statements for the SAML assertion inherited from app metadata (apps from the OIN)
* during app creation. There are two types of attribute statements: `EXPRESSION` and `GROUP`.
*
* @return configuredAttributeStatements
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The list of dynamic attribute statements for the SAML assertion inherited from app metadata (apps from the OIN) during app creation. There are two types of attribute statements: `EXPRESSION` and `GROUP`. ")
@JsonProperty(JSON_PROPERTY_CONFIGURED_ATTRIBUTE_STATEMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getConfiguredAttributeStatements() {
return configuredAttributeStatements;
}
@JsonProperty(JSON_PROPERTY_CONFIGURED_ATTRIBUTE_STATEMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setConfiguredAttributeStatements(List configuredAttributeStatements) {
this.configuredAttributeStatements = configuredAttributeStatements;
}
public SamlApplicationSettingsSignOn defaultRelayState(String defaultRelayState) {
this.defaultRelayState = defaultRelayState;
return this;
}
/**
* Identifies a specific application resource in an IdP-initiated SSO scenario
*
* @return defaultRelayState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifies a specific application resource in an IdP-initiated SSO scenario")
@JsonProperty(JSON_PROPERTY_DEFAULT_RELAY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDefaultRelayState() {
return defaultRelayState;
}
@JsonProperty(JSON_PROPERTY_DEFAULT_RELAY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDefaultRelayState(String defaultRelayState) {
this.defaultRelayState = defaultRelayState;
}
public SamlApplicationSettingsSignOn destination(String destination) {
this.destination = destination;
return this;
}
/**
* Identifies the location inside the SAML assertion where the SAML response should be sent
*
* @return destination
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Identifies the location inside the SAML assertion where the SAML response should be sent")
@JsonProperty(JSON_PROPERTY_DESTINATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDestination() {
return destination;
}
@JsonProperty(JSON_PROPERTY_DESTINATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDestination(String destination) {
this.destination = destination;
}
public SamlApplicationSettingsSignOn destinationOverride(String destinationOverride) {
this.destinationOverride = destinationOverride;
return this;
}
/**
* Destination override for CASB configuration. See [CASB config
* guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).
*
* @return destinationOverride
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Destination override for CASB configuration. See [CASB config guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).")
@JsonProperty(JSON_PROPERTY_DESTINATION_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDestinationOverride() {
return destinationOverride;
}
@JsonProperty(JSON_PROPERTY_DESTINATION_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDestinationOverride(String destinationOverride) {
this.destinationOverride = destinationOverride;
}
public SamlApplicationSettingsSignOn digestAlgorithm(String digestAlgorithm) {
this.digestAlgorithm = digestAlgorithm;
return this;
}
/**
* Determines the digest algorithm used to digitally sign the SAML assertion and response
*
* @return digestAlgorithm
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Determines the digest algorithm used to digitally sign the SAML assertion and response")
@JsonProperty(JSON_PROPERTY_DIGEST_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDigestAlgorithm() {
return digestAlgorithm;
}
@JsonProperty(JSON_PROPERTY_DIGEST_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDigestAlgorithm(String digestAlgorithm) {
this.digestAlgorithm = digestAlgorithm;
}
public SamlApplicationSettingsSignOn honorForceAuthn(Boolean honorForceAuthn) {
this.honorForceAuthn = honorForceAuthn;
return this;
}
/**
* Set to `true` to prompt users for their credentials when a SAML request has the `ForceAuthn`
* attribute set to `true`
*
* @return honorForceAuthn
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Set to `true` to prompt users for their credentials when a SAML request has the `ForceAuthn` attribute set to `true`")
@JsonProperty(JSON_PROPERTY_HONOR_FORCE_AUTHN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getHonorForceAuthn() {
return honorForceAuthn;
}
@JsonProperty(JSON_PROPERTY_HONOR_FORCE_AUTHN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHonorForceAuthn(Boolean honorForceAuthn) {
this.honorForceAuthn = honorForceAuthn;
}
public SamlApplicationSettingsSignOn idpIssuer(String idpIssuer) {
this.idpIssuer = idpIssuer;
return this;
}
/**
* SAML Issuer ID
*
* @return idpIssuer
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "SAML Issuer ID")
@JsonProperty(JSON_PROPERTY_IDP_ISSUER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getIdpIssuer() {
return idpIssuer;
}
@JsonProperty(JSON_PROPERTY_IDP_ISSUER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIdpIssuer(String idpIssuer) {
this.idpIssuer = idpIssuer;
}
public SamlApplicationSettingsSignOn inlineHooks(List inlineHooks) {
this.inlineHooks = inlineHooks;
return this;
}
public SamlApplicationSettingsSignOn addinlineHooksItem(SignOnInlineHook inlineHooksItem) {
if (this.inlineHooks == null) {
this.inlineHooks = new ArrayList<>();
}
this.inlineHooks.add(inlineHooksItem);
return this;
}
/**
* Associates the app with SAML inline hooks. See [the SAML assertion inline hook
* reference](https://developer.okta.com/docs/reference/saml-hook/).
*
* @return inlineHooks
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Associates the app with SAML inline hooks. See [the SAML assertion inline hook reference](https://developer.okta.com/docs/reference/saml-hook/).")
@JsonProperty(JSON_PROPERTY_INLINE_HOOKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getInlineHooks() {
return inlineHooks;
}
@JsonProperty(JSON_PROPERTY_INLINE_HOOKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInlineHooks(List inlineHooks) {
this.inlineHooks = inlineHooks;
}
public SamlApplicationSettingsSignOn participateSlo(SloParticipate participateSlo) {
this.participateSlo = participateSlo;
return this;
}
/**
* Get participateSlo
*
* @return participateSlo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PARTICIPATE_SLO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SloParticipate getParticipateSlo() {
return participateSlo;
}
@JsonProperty(JSON_PROPERTY_PARTICIPATE_SLO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setParticipateSlo(SloParticipate participateSlo) {
this.participateSlo = participateSlo;
}
public SamlApplicationSettingsSignOn recipient(String recipient) {
this.recipient = recipient;
return this;
}
/**
* The location where the app may present the SAML assertion
*
* @return recipient
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The location where the app may present the SAML assertion")
@JsonProperty(JSON_PROPERTY_RECIPIENT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getRecipient() {
return recipient;
}
@JsonProperty(JSON_PROPERTY_RECIPIENT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRecipient(String recipient) {
this.recipient = recipient;
}
public SamlApplicationSettingsSignOn recipientOverride(String recipientOverride) {
this.recipientOverride = recipientOverride;
return this;
}
/**
* Recipient override for CASB configuration. See [CASB config
* guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).
*
* @return recipientOverride
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Recipient override for CASB configuration. See [CASB config guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).")
@JsonProperty(JSON_PROPERTY_RECIPIENT_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRecipientOverride() {
return recipientOverride;
}
@JsonProperty(JSON_PROPERTY_RECIPIENT_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRecipientOverride(String recipientOverride) {
this.recipientOverride = recipientOverride;
}
public SamlApplicationSettingsSignOn requestCompressed(Boolean requestCompressed) {
this.requestCompressed = requestCompressed;
return this;
}
/**
* Determines whether the SAML request is expected to be compressed
*
* @return requestCompressed
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Determines whether the SAML request is expected to be compressed")
@JsonProperty(JSON_PROPERTY_REQUEST_COMPRESSED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getRequestCompressed() {
return requestCompressed;
}
@JsonProperty(JSON_PROPERTY_REQUEST_COMPRESSED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRequestCompressed(Boolean requestCompressed) {
this.requestCompressed = requestCompressed;
}
public SamlApplicationSettingsSignOn responseSigned(Boolean responseSigned) {
this.responseSigned = responseSigned;
return this;
}
/**
* Determines whether the SAML authentication response message is digitally signed by the IdP > **Note:** Either
* (or both) `responseSigned` or `assertionSigned` must be `TRUE`.
*
* @return responseSigned
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Determines whether the SAML authentication response message is digitally signed by the IdP > **Note:** Either (or both) `responseSigned` or `assertionSigned` must be `TRUE`.")
@JsonProperty(JSON_PROPERTY_RESPONSE_SIGNED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getResponseSigned() {
return responseSigned;
}
@JsonProperty(JSON_PROPERTY_RESPONSE_SIGNED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setResponseSigned(Boolean responseSigned) {
this.responseSigned = responseSigned;
}
public SamlApplicationSettingsSignOn samlAssertionLifetimeSeconds(Integer samlAssertionLifetimeSeconds) {
this.samlAssertionLifetimeSeconds = samlAssertionLifetimeSeconds;
return this;
}
/**
* Determines the SAML app session lifetimes with Okta
*
* @return samlAssertionLifetimeSeconds
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "3600", value = "Determines the SAML app session lifetimes with Okta")
@JsonProperty(JSON_PROPERTY_SAML_ASSERTION_LIFETIME_SECONDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getSamlAssertionLifetimeSeconds() {
return samlAssertionLifetimeSeconds;
}
@JsonProperty(JSON_PROPERTY_SAML_ASSERTION_LIFETIME_SECONDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSamlAssertionLifetimeSeconds(Integer samlAssertionLifetimeSeconds) {
this.samlAssertionLifetimeSeconds = samlAssertionLifetimeSeconds;
}
public SamlApplicationSettingsSignOn signatureAlgorithm(String signatureAlgorithm) {
this.signatureAlgorithm = signatureAlgorithm;
return this;
}
/**
* Determines the signing algorithm used to digitally sign the SAML assertion and response
*
* @return signatureAlgorithm
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Determines the signing algorithm used to digitally sign the SAML assertion and response")
@JsonProperty(JSON_PROPERTY_SIGNATURE_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSignatureAlgorithm() {
return signatureAlgorithm;
}
@JsonProperty(JSON_PROPERTY_SIGNATURE_ALGORITHM)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSignatureAlgorithm(String signatureAlgorithm) {
this.signatureAlgorithm = signatureAlgorithm;
}
public SamlApplicationSettingsSignOn slo(SingleLogout slo) {
this.slo = slo;
return this;
}
/**
* Get slo
*
* @return slo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SLO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SingleLogout getSlo() {
return slo;
}
@JsonProperty(JSON_PROPERTY_SLO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSlo(SingleLogout slo) {
this.slo = slo;
}
public SamlApplicationSettingsSignOn spCertificate(SamlSpCertificate spCertificate) {
this.spCertificate = spCertificate;
return this;
}
/**
* Get spCertificate
*
* @return spCertificate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SP_CERTIFICATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SamlSpCertificate getSpCertificate() {
return spCertificate;
}
@JsonProperty(JSON_PROPERTY_SP_CERTIFICATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSpCertificate(SamlSpCertificate spCertificate) {
this.spCertificate = spCertificate;
}
public SamlApplicationSettingsSignOn spIssuer(String spIssuer) {
this.spIssuer = spIssuer;
return this;
}
/**
* The issuer ID for the Service Provider. This property appears when SLO is enabled.
*
* @return spIssuer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The issuer ID for the Service Provider. This property appears when SLO is enabled.")
@JsonProperty(JSON_PROPERTY_SP_ISSUER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSpIssuer() {
return spIssuer;
}
@JsonProperty(JSON_PROPERTY_SP_ISSUER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSpIssuer(String spIssuer) {
this.spIssuer = spIssuer;
}
public SamlApplicationSettingsSignOn ssoAcsUrl(String ssoAcsUrl) {
this.ssoAcsUrl = ssoAcsUrl;
return this;
}
/**
* Single Sign-On Assertion Consumer Service (ACS) URL
*
* @return ssoAcsUrl
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Single Sign-On Assertion Consumer Service (ACS) URL")
@JsonProperty(JSON_PROPERTY_SSO_ACS_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSsoAcsUrl() {
return ssoAcsUrl;
}
@JsonProperty(JSON_PROPERTY_SSO_ACS_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSsoAcsUrl(String ssoAcsUrl) {
this.ssoAcsUrl = ssoAcsUrl;
}
public SamlApplicationSettingsSignOn ssoAcsUrlOverride(String ssoAcsUrlOverride) {
this.ssoAcsUrlOverride = ssoAcsUrlOverride;
return this;
}
/**
* Assertion Consumer Service (ACS) URL override for CASB configuration. See [CASB config
* guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).
*
* @return ssoAcsUrlOverride
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Assertion Consumer Service (ACS) URL override for CASB configuration. See [CASB config guide](https://help.okta.com/en-us/Content/Topics/Apps/CASB-config-guide.htm).")
@JsonProperty(JSON_PROPERTY_SSO_ACS_URL_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSsoAcsUrlOverride() {
return ssoAcsUrlOverride;
}
@JsonProperty(JSON_PROPERTY_SSO_ACS_URL_OVERRIDE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSsoAcsUrlOverride(String ssoAcsUrlOverride) {
this.ssoAcsUrlOverride = ssoAcsUrlOverride;
}
public SamlApplicationSettingsSignOn subjectNameIdFormat(String subjectNameIdFormat) {
this.subjectNameIdFormat = subjectNameIdFormat;
return this;
}
/**
* Get subjectNameIdFormat
*
* @return subjectNameIdFormat
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_SUBJECT_NAME_ID_FORMAT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSubjectNameIdFormat() {
return subjectNameIdFormat;
}
@JsonProperty(JSON_PROPERTY_SUBJECT_NAME_ID_FORMAT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSubjectNameIdFormat(String subjectNameIdFormat) {
this.subjectNameIdFormat = subjectNameIdFormat;
}
public SamlApplicationSettingsSignOn subjectNameIdTemplate(String subjectNameIdTemplate) {
this.subjectNameIdTemplate = subjectNameIdTemplate;
return this;
}
/**
* Template for app user's username when a user is assigned to the app
*
* @return subjectNameIdTemplate
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Template for app user's username when a user is assigned to the app")
@JsonProperty(JSON_PROPERTY_SUBJECT_NAME_ID_TEMPLATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSubjectNameIdTemplate() {
return subjectNameIdTemplate;
}
@JsonProperty(JSON_PROPERTY_SUBJECT_NAME_ID_TEMPLATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSubjectNameIdTemplate(String subjectNameIdTemplate) {
this.subjectNameIdTemplate = subjectNameIdTemplate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SamlApplicationSettingsSignOn samlApplicationSettingsSignOn = (SamlApplicationSettingsSignOn) o;
return Objects.equals(this.acsEndpoints, samlApplicationSettingsSignOn.acsEndpoints)
&& Objects.equals(this.allowMultipleAcsEndpoints,
samlApplicationSettingsSignOn.allowMultipleAcsEndpoints)
&& Objects.equals(this.assertionSigned, samlApplicationSettingsSignOn.assertionSigned)
&& Objects.equals(this.attributeStatements, samlApplicationSettingsSignOn.attributeStatements)
&& Objects.equals(this.audience, samlApplicationSettingsSignOn.audience)
&& Objects.equals(this.audienceOverride, samlApplicationSettingsSignOn.audienceOverride)
&& Objects.equals(this.authnContextClassRef, samlApplicationSettingsSignOn.authnContextClassRef)
&& Objects.equals(this.configuredAttributeStatements,
samlApplicationSettingsSignOn.configuredAttributeStatements)
&& Objects.equals(this.defaultRelayState, samlApplicationSettingsSignOn.defaultRelayState)
&& Objects.equals(this.destination, samlApplicationSettingsSignOn.destination)
&& Objects.equals(this.destinationOverride, samlApplicationSettingsSignOn.destinationOverride)
&& Objects.equals(this.digestAlgorithm, samlApplicationSettingsSignOn.digestAlgorithm)
&& Objects.equals(this.honorForceAuthn, samlApplicationSettingsSignOn.honorForceAuthn)
&& Objects.equals(this.idpIssuer, samlApplicationSettingsSignOn.idpIssuer)
&& Objects.equals(this.inlineHooks, samlApplicationSettingsSignOn.inlineHooks)
&& Objects.equals(this.participateSlo, samlApplicationSettingsSignOn.participateSlo)
&& Objects.equals(this.recipient, samlApplicationSettingsSignOn.recipient)
&& Objects.equals(this.recipientOverride, samlApplicationSettingsSignOn.recipientOverride)
&& Objects.equals(this.requestCompressed, samlApplicationSettingsSignOn.requestCompressed)
&& Objects.equals(this.responseSigned, samlApplicationSettingsSignOn.responseSigned)
&& Objects.equals(this.samlAssertionLifetimeSeconds,
samlApplicationSettingsSignOn.samlAssertionLifetimeSeconds)
&& Objects.equals(this.signatureAlgorithm, samlApplicationSettingsSignOn.signatureAlgorithm)
&& Objects.equals(this.slo, samlApplicationSettingsSignOn.slo)
&& Objects.equals(this.spCertificate, samlApplicationSettingsSignOn.spCertificate)
&& Objects.equals(this.spIssuer, samlApplicationSettingsSignOn.spIssuer)
&& Objects.equals(this.ssoAcsUrl, samlApplicationSettingsSignOn.ssoAcsUrl)
&& Objects.equals(this.ssoAcsUrlOverride, samlApplicationSettingsSignOn.ssoAcsUrlOverride)
&& Objects.equals(this.subjectNameIdFormat, samlApplicationSettingsSignOn.subjectNameIdFormat)
&& Objects.equals(this.subjectNameIdTemplate, samlApplicationSettingsSignOn.subjectNameIdTemplate);
// ;
}
@Override
public int hashCode() {
return Objects.hash(acsEndpoints, allowMultipleAcsEndpoints, assertionSigned, attributeStatements, audience,
audienceOverride, authnContextClassRef, configuredAttributeStatements, defaultRelayState, destination,
destinationOverride, digestAlgorithm, honorForceAuthn, idpIssuer, inlineHooks, participateSlo,
recipient, recipientOverride, requestCompressed, responseSigned, samlAssertionLifetimeSeconds,
signatureAlgorithm, slo, spCertificate, spIssuer, ssoAcsUrl, ssoAcsUrlOverride, subjectNameIdFormat,
subjectNameIdTemplate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SamlApplicationSettingsSignOn {\n");
sb.append(" acsEndpoints: ").append(toIndentedString(acsEndpoints)).append("\n");
sb.append(" allowMultipleAcsEndpoints: ").append(toIndentedString(allowMultipleAcsEndpoints)).append("\n");
sb.append(" assertionSigned: ").append(toIndentedString(assertionSigned)).append("\n");
sb.append(" attributeStatements: ").append(toIndentedString(attributeStatements)).append("\n");
sb.append(" audience: ").append(toIndentedString(audience)).append("\n");
sb.append(" audienceOverride: ").append(toIndentedString(audienceOverride)).append("\n");
sb.append(" authnContextClassRef: ").append(toIndentedString(authnContextClassRef)).append("\n");
sb.append(" configuredAttributeStatements: ").append(toIndentedString(configuredAttributeStatements))
.append("\n");
sb.append(" defaultRelayState: ").append(toIndentedString(defaultRelayState)).append("\n");
sb.append(" destination: ").append(toIndentedString(destination)).append("\n");
sb.append(" destinationOverride: ").append(toIndentedString(destinationOverride)).append("\n");
sb.append(" digestAlgorithm: ").append(toIndentedString(digestAlgorithm)).append("\n");
sb.append(" honorForceAuthn: ").append(toIndentedString(honorForceAuthn)).append("\n");
sb.append(" idpIssuer: ").append(toIndentedString(idpIssuer)).append("\n");
sb.append(" inlineHooks: ").append(toIndentedString(inlineHooks)).append("\n");
sb.append(" participateSlo: ").append(toIndentedString(participateSlo)).append("\n");
sb.append(" recipient: ").append(toIndentedString(recipient)).append("\n");
sb.append(" recipientOverride: ").append(toIndentedString(recipientOverride)).append("\n");
sb.append(" requestCompressed: ").append(toIndentedString(requestCompressed)).append("\n");
sb.append(" responseSigned: ").append(toIndentedString(responseSigned)).append("\n");
sb.append(" samlAssertionLifetimeSeconds: ").append(toIndentedString(samlAssertionLifetimeSeconds))
.append("\n");
sb.append(" signatureAlgorithm: ").append(toIndentedString(signatureAlgorithm)).append("\n");
sb.append(" slo: ").append(toIndentedString(slo)).append("\n");
sb.append(" spCertificate: ").append(toIndentedString(spCertificate)).append("\n");
sb.append(" spIssuer: ").append(toIndentedString(spIssuer)).append("\n");
sb.append(" ssoAcsUrl: ").append(toIndentedString(ssoAcsUrl)).append("\n");
sb.append(" ssoAcsUrlOverride: ").append(toIndentedString(ssoAcsUrlOverride)).append("\n");
sb.append(" subjectNameIdFormat: ").append(toIndentedString(subjectNameIdFormat)).append("\n");
sb.append(" subjectNameIdTemplate: ").append(toIndentedString(subjectNameIdTemplate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy