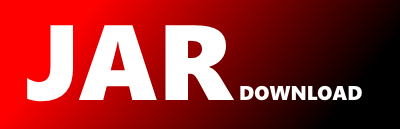
com.okta.sdk.resource.model.SecurityEventTokenRequestJwtEvents Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.CaepDeviceComplianceChangeEvent;
import com.okta.sdk.resource.model.CaepSessionRevokedEvent;
import com.okta.sdk.resource.model.OktaDeviceRiskChangeEvent;
import com.okta.sdk.resource.model.OktaIpChangeEvent;
import com.okta.sdk.resource.model.OktaUserRiskChangeEvent;
import com.okta.sdk.resource.model.RiscIdentifierChangedEvent;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* A non-empty collection of events
*/
@ApiModel(description = "A non-empty collection of events")
@JsonPropertyOrder({
SecurityEventTokenRequestJwtEvents.JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_DEVICE_RISK_CHANGE,
SecurityEventTokenRequestJwtEvents.JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_IP_CHANGE,
SecurityEventTokenRequestJwtEvents.JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_USER_RISK_CHANGE,
SecurityEventTokenRequestJwtEvents.JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_DEVICE_COMPLIANCE_CHANGE,
SecurityEventTokenRequestJwtEvents.JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_SESSION_REVOKED,
SecurityEventTokenRequestJwtEvents.JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_RISC_EVENT_TYPE_IDENTIFIER_CHANGED })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class SecurityEventTokenRequestJwtEvents implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_DEVICE_RISK_CHANGE = "https://schemas.okta.com/secevent/okta/event-type/device-risk-change";
private OktaDeviceRiskChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange;
public static final String JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_IP_CHANGE = "https://schemas.okta.com/secevent/okta/event-type/ip-change";
private OktaIpChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeIpChange;
public static final String JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_USER_RISK_CHANGE = "https://schemas.okta.com/secevent/okta/event-type/user-risk-change";
private OktaUserRiskChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange;
public static final String JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_DEVICE_COMPLIANCE_CHANGE = "https://schemas.openid.net/secevent/caep/event-type/device-compliance-change";
private CaepDeviceComplianceChangeEvent httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange;
public static final String JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_SESSION_REVOKED = "https://schemas.openid.net/secevent/caep/event-type/session-revoked";
private CaepSessionRevokedEvent httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked;
public static final String JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_RISC_EVENT_TYPE_IDENTIFIER_CHANGED = "https://schemas.openid.net/secevent/risc/event-type/identifier-changed";
private RiscIdentifierChangedEvent httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged;
public SecurityEventTokenRequestJwtEvents() {
}
public SecurityEventTokenRequestJwtEvents httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange(
OktaDeviceRiskChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange) {
this.httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange = httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange;
return this;
}
/**
* Get httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange
*
* @return httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_DEVICE_RISK_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OktaDeviceRiskChangeEvent getHttpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange() {
return httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange;
}
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_DEVICE_RISK_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHttpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange(
OktaDeviceRiskChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange) {
this.httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange = httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange;
}
public SecurityEventTokenRequestJwtEvents httpsColonSchemasOktaComSeceventOktaEventTypeIpChange(
OktaIpChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeIpChange) {
this.httpsColonSchemasOktaComSeceventOktaEventTypeIpChange = httpsColonSchemasOktaComSeceventOktaEventTypeIpChange;
return this;
}
/**
* Get httpsColonSchemasOktaComSeceventOktaEventTypeIpChange
*
* @return httpsColonSchemasOktaComSeceventOktaEventTypeIpChange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_IP_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OktaIpChangeEvent getHttpsColonSchemasOktaComSeceventOktaEventTypeIpChange() {
return httpsColonSchemasOktaComSeceventOktaEventTypeIpChange;
}
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_IP_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHttpsColonSchemasOktaComSeceventOktaEventTypeIpChange(
OktaIpChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeIpChange) {
this.httpsColonSchemasOktaComSeceventOktaEventTypeIpChange = httpsColonSchemasOktaComSeceventOktaEventTypeIpChange;
}
public SecurityEventTokenRequestJwtEvents httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange(
OktaUserRiskChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange) {
this.httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange = httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange;
return this;
}
/**
* Get httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange
*
* @return httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_USER_RISK_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OktaUserRiskChangeEvent getHttpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange() {
return httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange;
}
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OKTA_COM_SECEVENT_OKTA_EVENT_TYPE_USER_RISK_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHttpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange(
OktaUserRiskChangeEvent httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange) {
this.httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange = httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange;
}
public SecurityEventTokenRequestJwtEvents httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange(
CaepDeviceComplianceChangeEvent httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange) {
this.httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange = httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange;
return this;
}
/**
* Get httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange
*
* @return httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_DEVICE_COMPLIANCE_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CaepDeviceComplianceChangeEvent getHttpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange() {
return httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange;
}
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_DEVICE_COMPLIANCE_CHANGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHttpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange(
CaepDeviceComplianceChangeEvent httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange) {
this.httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange = httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange;
}
public SecurityEventTokenRequestJwtEvents httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked(
CaepSessionRevokedEvent httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked) {
this.httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked = httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked;
return this;
}
/**
* Get httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked
*
* @return httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_SESSION_REVOKED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CaepSessionRevokedEvent getHttpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked() {
return httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked;
}
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_CAEP_EVENT_TYPE_SESSION_REVOKED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHttpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked(
CaepSessionRevokedEvent httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked) {
this.httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked = httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked;
}
public SecurityEventTokenRequestJwtEvents httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged(
RiscIdentifierChangedEvent httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged) {
this.httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged = httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged;
return this;
}
/**
* Get httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged
*
* @return httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_RISC_EVENT_TYPE_IDENTIFIER_CHANGED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public RiscIdentifierChangedEvent getHttpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged() {
return httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged;
}
@JsonProperty(JSON_PROPERTY_HTTPS_COLON_SCHEMAS_OPENID_NET_SECEVENT_RISC_EVENT_TYPE_IDENTIFIER_CHANGED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHttpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged(
RiscIdentifierChangedEvent httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged) {
this.httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged = httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SecurityEventTokenRequestJwtEvents securityEventTokenRequestJwtEvents = (SecurityEventTokenRequestJwtEvents) o;
return Objects.equals(this.httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange,
securityEventTokenRequestJwtEvents.httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange)
&& Objects.equals(this.httpsColonSchemasOktaComSeceventOktaEventTypeIpChange,
securityEventTokenRequestJwtEvents.httpsColonSchemasOktaComSeceventOktaEventTypeIpChange)
&& Objects.equals(this.httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange,
securityEventTokenRequestJwtEvents.httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange)
&& Objects.equals(this.httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange,
securityEventTokenRequestJwtEvents.httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange)
&& Objects.equals(this.httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked,
securityEventTokenRequestJwtEvents.httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked)
&& Objects.equals(this.httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged,
securityEventTokenRequestJwtEvents.httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged);
// ;
}
@Override
public int hashCode() {
return Objects.hash(httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange,
httpsColonSchemasOktaComSeceventOktaEventTypeIpChange,
httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange,
httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange,
httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked,
httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SecurityEventTokenRequestJwtEvents {\n");
sb.append(" httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange: ")
.append(toIndentedString(httpsColonSchemasOktaComSeceventOktaEventTypeDeviceRiskChange)).append("\n");
sb.append(" httpsColonSchemasOktaComSeceventOktaEventTypeIpChange: ")
.append(toIndentedString(httpsColonSchemasOktaComSeceventOktaEventTypeIpChange)).append("\n");
sb.append(" httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange: ")
.append(toIndentedString(httpsColonSchemasOktaComSeceventOktaEventTypeUserRiskChange)).append("\n");
sb.append(" httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange: ")
.append(toIndentedString(httpsColonSchemasOpenidNetSeceventCaepEventTypeDeviceComplianceChange))
.append("\n");
sb.append(" httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked: ")
.append(toIndentedString(httpsColonSchemasOpenidNetSeceventCaepEventTypeSessionRevoked)).append("\n");
sb.append(" httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged: ")
.append(toIndentedString(httpsColonSchemasOpenidNetSeceventRiscEventTypeIdentifierChanged))
.append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy