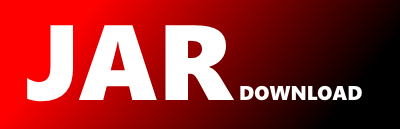
com.okta.sdk.resource.model.StreamConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.StreamConfigurationAud;
import com.okta.sdk.resource.model.StreamConfigurationDelivery;
import java.net.URI;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* StreamConfiguration
*/
@JsonPropertyOrder({ StreamConfiguration.JSON_PROPERTY_AUD, StreamConfiguration.JSON_PROPERTY_DELIVERY,
StreamConfiguration.JSON_PROPERTY_EVENTS_DELIVERED, StreamConfiguration.JSON_PROPERTY_EVENTS_REQUESTED,
StreamConfiguration.JSON_PROPERTY_EVENTS_SUPPORTED, StreamConfiguration.JSON_PROPERTY_FORMAT,
StreamConfiguration.JSON_PROPERTY_ISS, StreamConfiguration.JSON_PROPERTY_MIN_VERIFICATION_INTERVAL,
StreamConfiguration.JSON_PROPERTY_STREAM_ID })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class StreamConfiguration implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_AUD = "aud";
private StreamConfigurationAud aud;
public static final String JSON_PROPERTY_DELIVERY = "delivery";
private StreamConfigurationDelivery delivery;
public static final String JSON_PROPERTY_EVENTS_DELIVERED = "events_delivered";
private List eventsDelivered = null;
public static final String JSON_PROPERTY_EVENTS_REQUESTED = "events_requested";
private List eventsRequested = new ArrayList<>();
public static final String JSON_PROPERTY_EVENTS_SUPPORTED = "events_supported";
private List eventsSupported = null;
/**
* The Subject Identifier format expected for any SET transmitted.
*/
public enum FormatEnum {
ISS_SUB("iss_sub"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
FormatEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static FormatEnum fromValue(String value) {
for (FormatEnum b : FormatEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_FORMAT = "format";
private FormatEnum format;
public static final String JSON_PROPERTY_ISS = "iss";
private String iss;
public static final String JSON_PROPERTY_MIN_VERIFICATION_INTERVAL = "min_verification_interval";
private JsonNullable minVerificationInterval = JsonNullable. undefined();
public static final String JSON_PROPERTY_STREAM_ID = "stream_id";
private String streamId;
public StreamConfiguration() {
}
public StreamConfiguration aud(StreamConfigurationAud aud) {
this.aud = aud;
return this;
}
/**
* Get aud
*
* @return aud
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AUD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public StreamConfigurationAud getAud() {
return aud;
}
@JsonProperty(JSON_PROPERTY_AUD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAud(StreamConfigurationAud aud) {
this.aud = aud;
}
public StreamConfiguration delivery(StreamConfigurationDelivery delivery) {
this.delivery = delivery;
return this;
}
/**
* Get delivery
*
* @return delivery
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_DELIVERY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public StreamConfigurationDelivery getDelivery() {
return delivery;
}
@JsonProperty(JSON_PROPERTY_DELIVERY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDelivery(StreamConfigurationDelivery delivery) {
this.delivery = delivery;
}
public StreamConfiguration eventsDelivered(List eventsDelivered) {
this.eventsDelivered = eventsDelivered;
return this;
}
public StreamConfiguration addeventsDeliveredItem(URI eventsDeliveredItem) {
if (this.eventsDelivered == null) {
this.eventsDelivered = new ArrayList<>();
}
this.eventsDelivered.add(eventsDeliveredItem);
return this;
}
/**
* The events (mapped by the array of event type URIs) that the transmitter actually delivers to the SSF Stream. A
* read-only parameter that is set by the transmitter. If this parameter is included in the request, the value must
* match the expected value from the transmitter.
*
* @return eventsDelivered
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"https://schemas.openid.net/secevent/caep/event-type/session-revoked\",\"https://schemas.openid.net/secevent/caep/event-type/credential-change\"]", value = "The events (mapped by the array of event type URIs) that the transmitter actually delivers to the SSF Stream. A read-only parameter that is set by the transmitter. If this parameter is included in the request, the value must match the expected value from the transmitter.")
@JsonProperty(JSON_PROPERTY_EVENTS_DELIVERED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getEventsDelivered() {
return eventsDelivered;
}
@JsonProperty(JSON_PROPERTY_EVENTS_DELIVERED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEventsDelivered(List eventsDelivered) {
this.eventsDelivered = eventsDelivered;
}
public StreamConfiguration eventsRequested(List eventsRequested) {
this.eventsRequested = eventsRequested;
return this;
}
public StreamConfiguration addeventsRequestedItem(URI eventsRequestedItem) {
if (this.eventsRequested == null) {
this.eventsRequested = new ArrayList<>();
}
this.eventsRequested.add(eventsRequestedItem);
return this;
}
/**
* The events (mapped by the array of event type URIs) that the receiver wants to receive
*
* @return eventsRequested
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "[\"https://schemas.openid.net/secevent/caep/event-type/session-revoked\",\"https://schemas.openid.net/secevent/caep/event-type/credential-change\"]", required = true, value = "The events (mapped by the array of event type URIs) that the receiver wants to receive")
@JsonProperty(JSON_PROPERTY_EVENTS_REQUESTED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getEventsRequested() {
return eventsRequested;
}
@JsonProperty(JSON_PROPERTY_EVENTS_REQUESTED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEventsRequested(List eventsRequested) {
this.eventsRequested = eventsRequested;
}
public StreamConfiguration eventsSupported(List eventsSupported) {
this.eventsSupported = eventsSupported;
return this;
}
public StreamConfiguration addeventsSupportedItem(URI eventsSupportedItem) {
if (this.eventsSupported == null) {
this.eventsSupported = new ArrayList<>();
}
this.eventsSupported.add(eventsSupportedItem);
return this;
}
/**
* An array of event type URIs that the transmitter supports. A read-only parameter that is set by the transmitter.
* If this parameter is included in the request, the value must match the expected value from the transmitter.
*
* @return eventsSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"https://schemas.openid.net/secevent/caep/event-type/session-revoked\",\"https://schemas.openid.net/secevent/caep/event-type/credential-change\"]", value = "An array of event type URIs that the transmitter supports. A read-only parameter that is set by the transmitter. If this parameter is included in the request, the value must match the expected value from the transmitter.")
@JsonProperty(JSON_PROPERTY_EVENTS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getEventsSupported() {
return eventsSupported;
}
@JsonProperty(JSON_PROPERTY_EVENTS_SUPPORTED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEventsSupported(List eventsSupported) {
this.eventsSupported = eventsSupported;
}
public StreamConfiguration format(FormatEnum format) {
this.format = format;
return this;
}
/**
* The Subject Identifier format expected for any SET transmitted.
*
* @return format
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Subject Identifier format expected for any SET transmitted.")
@JsonProperty(JSON_PROPERTY_FORMAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public FormatEnum getFormat() {
return format;
}
@JsonProperty(JSON_PROPERTY_FORMAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFormat(FormatEnum format) {
this.format = format;
}
public StreamConfiguration iss(String iss) {
this.iss = iss;
return this;
}
/**
* The issuer used in Security Event Tokens (SETs). This value is set as `iss` in the claim. A read-only
* parameter that is set by the transmitter. If this parameter is included in the request, the value must match the
* expected value from the transmitter.
*
* @return iss
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "https://{yourOktaDomain}", value = "The issuer used in Security Event Tokens (SETs). This value is set as `iss` in the claim. A read-only parameter that is set by the transmitter. If this parameter is included in the request, the value must match the expected value from the transmitter.")
@JsonProperty(JSON_PROPERTY_ISS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIss() {
return iss;
}
@JsonProperty(JSON_PROPERTY_ISS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIss(String iss) {
this.iss = iss;
}
public StreamConfiguration minVerificationInterval(Integer minVerificationInterval) {
this.minVerificationInterval = JsonNullable. of(minVerificationInterval);
return this;
}
/**
* The minimum amount of time, in seconds, between two verification requests. A read-only parameter that is set by
* the transmitter. If this parameter is included in the request, the value must match the expected value from the
* transmitter.
*
* @return minVerificationInterval
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "60", value = "The minimum amount of time, in seconds, between two verification requests. A read-only parameter that is set by the transmitter. If this parameter is included in the request, the value must match the expected value from the transmitter.")
@JsonIgnore
public Integer getMinVerificationInterval() {
return minVerificationInterval.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MIN_VERIFICATION_INTERVAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getMinVerificationInterval_JsonNullable() {
return minVerificationInterval;
}
@JsonProperty(JSON_PROPERTY_MIN_VERIFICATION_INTERVAL)
public void setMinVerificationInterval_JsonNullable(JsonNullable minVerificationInterval) {
this.minVerificationInterval = minVerificationInterval;
}
public void setMinVerificationInterval(Integer minVerificationInterval) {
this.minVerificationInterval = JsonNullable. of(minVerificationInterval);
}
public StreamConfiguration streamId(String streamId) {
this.streamId = streamId;
return this;
}
/**
* The ID of the SSF Stream configuration
*
* @return streamId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "esc1k235GIIztAuGK0g5", value = "The ID of the SSF Stream configuration")
@JsonProperty(JSON_PROPERTY_STREAM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getStreamId() {
return streamId;
}
@JsonProperty(JSON_PROPERTY_STREAM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStreamId(String streamId) {
this.streamId = streamId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StreamConfiguration streamConfiguration = (StreamConfiguration) o;
return Objects.equals(this.aud, streamConfiguration.aud)
&& Objects.equals(this.delivery, streamConfiguration.delivery)
&& Objects.equals(this.eventsDelivered, streamConfiguration.eventsDelivered)
&& Objects.equals(this.eventsRequested, streamConfiguration.eventsRequested)
&& Objects.equals(this.eventsSupported, streamConfiguration.eventsSupported)
&& Objects.equals(this.format, streamConfiguration.format)
&& Objects.equals(this.iss, streamConfiguration.iss)
&& equalsNullable(this.minVerificationInterval, streamConfiguration.minVerificationInterval)
&& Objects.equals(this.streamId, streamConfiguration.streamId);
// ;
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(aud, delivery, eventsDelivered, eventsRequested, eventsSupported, format, iss,
hashCodeNullable(minVerificationInterval), streamId);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class StreamConfiguration {\n");
sb.append(" aud: ").append(toIndentedString(aud)).append("\n");
sb.append(" delivery: ").append(toIndentedString(delivery)).append("\n");
sb.append(" eventsDelivered: ").append(toIndentedString(eventsDelivered)).append("\n");
sb.append(" eventsRequested: ").append(toIndentedString(eventsRequested)).append("\n");
sb.append(" eventsSupported: ").append(toIndentedString(eventsSupported)).append("\n");
sb.append(" format: ").append(toIndentedString(format)).append("\n");
sb.append(" iss: ").append(toIndentedString(iss)).append("\n");
sb.append(" minVerificationInterval: ").append(toIndentedString(minVerificationInterval)).append("\n");
sb.append(" streamId: ").append(toIndentedString(streamId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy