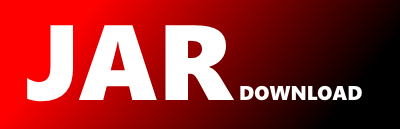
com.okta.sdk.resource.model.SwaApplicationSettingsApplication Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* SwaApplicationSettingsApplication
*/
@JsonPropertyOrder({ SwaApplicationSettingsApplication.JSON_PROPERTY_BUTTON_FIELD,
SwaApplicationSettingsApplication.JSON_PROPERTY_BUTTON_SELECTOR,
SwaApplicationSettingsApplication.JSON_PROPERTY_EXTRA_FIELD_SELECTOR,
SwaApplicationSettingsApplication.JSON_PROPERTY_EXTRA_FIELD_VALUE,
SwaApplicationSettingsApplication.JSON_PROPERTY_LOGIN_URL_REGEX,
SwaApplicationSettingsApplication.JSON_PROPERTY_PASSWORD_FIELD,
SwaApplicationSettingsApplication.JSON_PROPERTY_PASSWORD_SELECTOR,
SwaApplicationSettingsApplication.JSON_PROPERTY_TARGET_U_R_L,
SwaApplicationSettingsApplication.JSON_PROPERTY_URL,
SwaApplicationSettingsApplication.JSON_PROPERTY_USERNAME_FIELD,
SwaApplicationSettingsApplication.JSON_PROPERTY_USER_NAME_SELECTOR })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class SwaApplicationSettingsApplication implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_BUTTON_FIELD = "buttonField";
private String buttonField;
public static final String JSON_PROPERTY_BUTTON_SELECTOR = "buttonSelector";
private String buttonSelector;
public static final String JSON_PROPERTY_EXTRA_FIELD_SELECTOR = "extraFieldSelector";
private String extraFieldSelector;
public static final String JSON_PROPERTY_EXTRA_FIELD_VALUE = "extraFieldValue";
private String extraFieldValue;
public static final String JSON_PROPERTY_LOGIN_URL_REGEX = "loginUrlRegex";
private String loginUrlRegex;
public static final String JSON_PROPERTY_PASSWORD_FIELD = "passwordField";
private String passwordField;
public static final String JSON_PROPERTY_PASSWORD_SELECTOR = "passwordSelector";
private String passwordSelector;
public static final String JSON_PROPERTY_TARGET_U_R_L = "targetURL";
private String targetURL;
public static final String JSON_PROPERTY_URL = "url";
private String url;
public static final String JSON_PROPERTY_USERNAME_FIELD = "usernameField";
private String usernameField;
public static final String JSON_PROPERTY_USER_NAME_SELECTOR = "userNameSelector";
private String userNameSelector;
public SwaApplicationSettingsApplication() {
}
public SwaApplicationSettingsApplication buttonField(String buttonField) {
this.buttonField = buttonField;
return this;
}
/**
* CSS selector for the **Sign-In** button in the sign-in form (for SWA apps with the `template_swa` app
* name definition)
*
* @return buttonField
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "CSS selector for the **Sign-In** button in the sign-in form (for SWA apps with the `template_swa` app name definition)")
@JsonProperty(JSON_PROPERTY_BUTTON_FIELD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getButtonField() {
return buttonField;
}
@JsonProperty(JSON_PROPERTY_BUTTON_FIELD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setButtonField(String buttonField) {
this.buttonField = buttonField;
}
public SwaApplicationSettingsApplication buttonSelector(String buttonSelector) {
this.buttonSelector = buttonSelector;
return this;
}
/**
* CSS selector for the **Sign-In** button in the sign-in form (for three-field SWA apps with the
* `template_swa3field` app name definition)
*
* @return buttonSelector
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "CSS selector for the **Sign-In** button in the sign-in form (for three-field SWA apps with the `template_swa3field` app name definition)")
@JsonProperty(JSON_PROPERTY_BUTTON_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getButtonSelector() {
return buttonSelector;
}
@JsonProperty(JSON_PROPERTY_BUTTON_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setButtonSelector(String buttonSelector) {
this.buttonSelector = buttonSelector;
}
public SwaApplicationSettingsApplication extraFieldSelector(String extraFieldSelector) {
this.extraFieldSelector = extraFieldSelector;
return this;
}
/**
* Enter the CSS selector for the extra field (for three-field SWA apps with the `template_swa3field` app
* name definition).
*
* @return extraFieldSelector
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Enter the CSS selector for the extra field (for three-field SWA apps with the `template_swa3field` app name definition).")
@JsonProperty(JSON_PROPERTY_EXTRA_FIELD_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExtraFieldSelector() {
return extraFieldSelector;
}
@JsonProperty(JSON_PROPERTY_EXTRA_FIELD_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExtraFieldSelector(String extraFieldSelector) {
this.extraFieldSelector = extraFieldSelector;
}
public SwaApplicationSettingsApplication extraFieldValue(String extraFieldValue) {
this.extraFieldValue = extraFieldValue;
return this;
}
/**
* Enter the value for the extra field in the form (for three-field SWA apps with the `template_swa3field`
* app name definition).
*
* @return extraFieldValue
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Enter the value for the extra field in the form (for three-field SWA apps with the `template_swa3field` app name definition).")
@JsonProperty(JSON_PROPERTY_EXTRA_FIELD_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExtraFieldValue() {
return extraFieldValue;
}
@JsonProperty(JSON_PROPERTY_EXTRA_FIELD_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExtraFieldValue(String extraFieldValue) {
this.extraFieldValue = extraFieldValue;
}
public SwaApplicationSettingsApplication loginUrlRegex(String loginUrlRegex) {
this.loginUrlRegex = loginUrlRegex;
return this;
}
/**
* A regular expression that further restricts targetURL to the specified regular expression
*
* @return loginUrlRegex
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A regular expression that further restricts targetURL to the specified regular expression")
@JsonProperty(JSON_PROPERTY_LOGIN_URL_REGEX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLoginUrlRegex() {
return loginUrlRegex;
}
@JsonProperty(JSON_PROPERTY_LOGIN_URL_REGEX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLoginUrlRegex(String loginUrlRegex) {
this.loginUrlRegex = loginUrlRegex;
}
public SwaApplicationSettingsApplication passwordField(String passwordField) {
this.passwordField = passwordField;
return this;
}
/**
* CSS selector for the **Password** field in the sign-in form (for SWA apps with the `template_swa` app
* name definition)
*
* @return passwordField
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "CSS selector for the **Password** field in the sign-in form (for SWA apps with the `template_swa` app name definition)")
@JsonProperty(JSON_PROPERTY_PASSWORD_FIELD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getPasswordField() {
return passwordField;
}
@JsonProperty(JSON_PROPERTY_PASSWORD_FIELD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPasswordField(String passwordField) {
this.passwordField = passwordField;
}
public SwaApplicationSettingsApplication passwordSelector(String passwordSelector) {
this.passwordSelector = passwordSelector;
return this;
}
/**
* CSS selector for the **Password** field in the sign-in form (for three-field SWA apps with the
* `template_swa3field` app name definition)
*
* @return passwordSelector
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "CSS selector for the **Password** field in the sign-in form (for three-field SWA apps with the `template_swa3field` app name definition)")
@JsonProperty(JSON_PROPERTY_PASSWORD_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPasswordSelector() {
return passwordSelector;
}
@JsonProperty(JSON_PROPERTY_PASSWORD_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPasswordSelector(String passwordSelector) {
this.passwordSelector = passwordSelector;
}
public SwaApplicationSettingsApplication targetURL(String targetURL) {
this.targetURL = targetURL;
return this;
}
/**
* The URL of the sign-in page for this app (for three-field SWA apps with the `template_swa3field` app
* name definition)
*
* @return targetURL
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL of the sign-in page for this app (for three-field SWA apps with the `template_swa3field` app name definition)")
@JsonProperty(JSON_PROPERTY_TARGET_U_R_L)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTargetURL() {
return targetURL;
}
@JsonProperty(JSON_PROPERTY_TARGET_U_R_L)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTargetURL(String targetURL) {
this.targetURL = targetURL;
}
public SwaApplicationSettingsApplication url(String url) {
this.url = url;
return this;
}
/**
* The URL of the sign-in page for this app (for SWA apps with the `template_swa` app name definition)
*
* @return url
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The URL of the sign-in page for this app (for SWA apps with the `template_swa` app name definition)")
@JsonProperty(JSON_PROPERTY_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getUrl() {
return url;
}
@JsonProperty(JSON_PROPERTY_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUrl(String url) {
this.url = url;
}
public SwaApplicationSettingsApplication usernameField(String usernameField) {
this.usernameField = usernameField;
return this;
}
/**
* CSS selector for the **Username** field in the sign-in form (for SWA apps with the `template_swa` app
* name definition)
*
* @return usernameField
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "CSS selector for the **Username** field in the sign-in form (for SWA apps with the `template_swa` app name definition)")
@JsonProperty(JSON_PROPERTY_USERNAME_FIELD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getUsernameField() {
return usernameField;
}
@JsonProperty(JSON_PROPERTY_USERNAME_FIELD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUsernameField(String usernameField) {
this.usernameField = usernameField;
}
public SwaApplicationSettingsApplication userNameSelector(String userNameSelector) {
this.userNameSelector = userNameSelector;
return this;
}
/**
* CSS selector for the **Username** field in the sign-in form (for three-field SWA apps with the
* `template_swa3field` app name definition)
*
* @return userNameSelector
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "CSS selector for the **Username** field in the sign-in form (for three-field SWA apps with the `template_swa3field` app name definition)")
@JsonProperty(JSON_PROPERTY_USER_NAME_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUserNameSelector() {
return userNameSelector;
}
@JsonProperty(JSON_PROPERTY_USER_NAME_SELECTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserNameSelector(String userNameSelector) {
this.userNameSelector = userNameSelector;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SwaApplicationSettingsApplication swaApplicationSettingsApplication = (SwaApplicationSettingsApplication) o;
return Objects.equals(this.buttonField, swaApplicationSettingsApplication.buttonField)
&& Objects.equals(this.buttonSelector, swaApplicationSettingsApplication.buttonSelector)
&& Objects.equals(this.extraFieldSelector, swaApplicationSettingsApplication.extraFieldSelector)
&& Objects.equals(this.extraFieldValue, swaApplicationSettingsApplication.extraFieldValue)
&& Objects.equals(this.loginUrlRegex, swaApplicationSettingsApplication.loginUrlRegex)
&& Objects.equals(this.passwordField, swaApplicationSettingsApplication.passwordField)
&& Objects.equals(this.passwordSelector, swaApplicationSettingsApplication.passwordSelector)
&& Objects.equals(this.targetURL, swaApplicationSettingsApplication.targetURL)
&& Objects.equals(this.url, swaApplicationSettingsApplication.url)
&& Objects.equals(this.usernameField, swaApplicationSettingsApplication.usernameField)
&& Objects.equals(this.userNameSelector, swaApplicationSettingsApplication.userNameSelector);
// ;
}
@Override
public int hashCode() {
return Objects.hash(buttonField, buttonSelector, extraFieldSelector, extraFieldValue, loginUrlRegex,
passwordField, passwordSelector, targetURL, url, usernameField, userNameSelector);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SwaApplicationSettingsApplication {\n");
sb.append(" buttonField: ").append(toIndentedString(buttonField)).append("\n");
sb.append(" buttonSelector: ").append(toIndentedString(buttonSelector)).append("\n");
sb.append(" extraFieldSelector: ").append(toIndentedString(extraFieldSelector)).append("\n");
sb.append(" extraFieldValue: ").append(toIndentedString(extraFieldValue)).append("\n");
sb.append(" loginUrlRegex: ").append(toIndentedString(loginUrlRegex)).append("\n");
sb.append(" passwordField: ").append(toIndentedString(passwordField)).append("\n");
sb.append(" passwordSelector: ").append(toIndentedString(passwordSelector)).append("\n");
sb.append(" targetURL: ").append(toIndentedString(targetURL)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" usernameField: ").append(toIndentedString(usernameField)).append("\n");
sb.append(" userNameSelector: ").append(toIndentedString(userNameSelector)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy