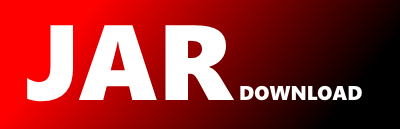
com.okta.sdk.resource.model.TokenResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.TokenResponseTokenType;
import com.okta.sdk.resource.model.TokenType;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* TokenResponse
*/
@JsonPropertyOrder({ TokenResponse.JSON_PROPERTY_ACCESS_TOKEN, TokenResponse.JSON_PROPERTY_DEVICE_SECRET,
TokenResponse.JSON_PROPERTY_EXPIRES_IN, TokenResponse.JSON_PROPERTY_ID_TOKEN,
TokenResponse.JSON_PROPERTY_ISSUED_TOKEN_TYPE, TokenResponse.JSON_PROPERTY_REFRESH_TOKEN,
TokenResponse.JSON_PROPERTY_SCOPE, TokenResponse.JSON_PROPERTY_TOKEN_TYPE })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class TokenResponse implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACCESS_TOKEN = "access_token";
private String accessToken;
public static final String JSON_PROPERTY_DEVICE_SECRET = "device_secret";
private String deviceSecret;
public static final String JSON_PROPERTY_EXPIRES_IN = "expires_in";
private Integer expiresIn;
public static final String JSON_PROPERTY_ID_TOKEN = "id_token";
private String idToken;
public static final String JSON_PROPERTY_ISSUED_TOKEN_TYPE = "issued_token_type";
private TokenType issuedTokenType;
public static final String JSON_PROPERTY_REFRESH_TOKEN = "refresh_token";
private String refreshToken;
public static final String JSON_PROPERTY_SCOPE = "scope";
private String scope;
public static final String JSON_PROPERTY_TOKEN_TYPE = "token_type";
private TokenResponseTokenType tokenType;
public TokenResponse() {
}
public TokenResponse accessToken(String accessToken) {
this.accessToken = accessToken;
return this;
}
/**
* An access token.
*
* @return accessToken
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An access token.")
@JsonProperty(JSON_PROPERTY_ACCESS_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAccessToken() {
return accessToken;
}
@JsonProperty(JSON_PROPERTY_ACCESS_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public TokenResponse deviceSecret(String deviceSecret) {
this.deviceSecret = deviceSecret;
return this;
}
/**
* An opaque device secret. This is returned if the `device_sso` scope is granted.
*
* @return deviceSecret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An opaque device secret. This is returned if the `device_sso` scope is granted.")
@JsonProperty(JSON_PROPERTY_DEVICE_SECRET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDeviceSecret() {
return deviceSecret;
}
@JsonProperty(JSON_PROPERTY_DEVICE_SECRET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeviceSecret(String deviceSecret) {
this.deviceSecret = deviceSecret;
}
public TokenResponse expiresIn(Integer expiresIn) {
this.expiresIn = expiresIn;
return this;
}
/**
* The expiration time of the access token in seconds.
*
* @return expiresIn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The expiration time of the access token in seconds.")
@JsonProperty(JSON_PROPERTY_EXPIRES_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getExpiresIn() {
return expiresIn;
}
@JsonProperty(JSON_PROPERTY_EXPIRES_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExpiresIn(Integer expiresIn) {
this.expiresIn = expiresIn;
}
public TokenResponse idToken(String idToken) {
this.idToken = idToken;
return this;
}
/**
* An ID token. This is returned if the `openid` scope is granted.
*
* @return idToken
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An ID token. This is returned if the `openid` scope is granted.")
@JsonProperty(JSON_PROPERTY_ID_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIdToken() {
return idToken;
}
@JsonProperty(JSON_PROPERTY_ID_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIdToken(String idToken) {
this.idToken = idToken;
}
public TokenResponse issuedTokenType(TokenType issuedTokenType) {
this.issuedTokenType = issuedTokenType;
return this;
}
/**
* Get issuedTokenType
*
* @return issuedTokenType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ISSUED_TOKEN_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TokenType getIssuedTokenType() {
return issuedTokenType;
}
@JsonProperty(JSON_PROPERTY_ISSUED_TOKEN_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIssuedTokenType(TokenType issuedTokenType) {
this.issuedTokenType = issuedTokenType;
}
public TokenResponse refreshToken(String refreshToken) {
this.refreshToken = refreshToken;
return this;
}
/**
* An opaque refresh token. This is returned if the `offline_access` scope is granted.
*
* @return refreshToken
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An opaque refresh token. This is returned if the `offline_access` scope is granted.")
@JsonProperty(JSON_PROPERTY_REFRESH_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRefreshToken() {
return refreshToken;
}
@JsonProperty(JSON_PROPERTY_REFRESH_TOKEN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRefreshToken(String refreshToken) {
this.refreshToken = refreshToken;
}
public TokenResponse scope(String scope) {
this.scope = scope;
return this;
}
/**
* The scopes contained in the access token.
*
* @return scope
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The scopes contained in the access token.")
@JsonProperty(JSON_PROPERTY_SCOPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getScope() {
return scope;
}
@JsonProperty(JSON_PROPERTY_SCOPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setScope(String scope) {
this.scope = scope;
}
public TokenResponse tokenType(TokenResponseTokenType tokenType) {
this.tokenType = tokenType;
return this;
}
/**
* Get tokenType
*
* @return tokenType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOKEN_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TokenResponseTokenType getTokenType() {
return tokenType;
}
@JsonProperty(JSON_PROPERTY_TOKEN_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTokenType(TokenResponseTokenType tokenType) {
this.tokenType = tokenType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TokenResponse tokenResponse = (TokenResponse) o;
return Objects.equals(this.accessToken, tokenResponse.accessToken)
&& Objects.equals(this.deviceSecret, tokenResponse.deviceSecret)
&& Objects.equals(this.expiresIn, tokenResponse.expiresIn)
&& Objects.equals(this.idToken, tokenResponse.idToken)
&& Objects.equals(this.issuedTokenType, tokenResponse.issuedTokenType)
&& Objects.equals(this.refreshToken, tokenResponse.refreshToken)
&& Objects.equals(this.scope, tokenResponse.scope)
&& Objects.equals(this.tokenType, tokenResponse.tokenType);
// ;
}
@Override
public int hashCode() {
return Objects.hash(accessToken, deviceSecret, expiresIn, idToken, issuedTokenType, refreshToken, scope,
tokenType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TokenResponse {\n");
sb.append(" accessToken: ").append(toIndentedString(accessToken)).append("\n");
sb.append(" deviceSecret: ").append(toIndentedString(deviceSecret)).append("\n");
sb.append(" expiresIn: ").append(toIndentedString(expiresIn)).append("\n");
sb.append(" idToken: ").append(toIndentedString(idToken)).append("\n");
sb.append(" issuedTokenType: ").append(toIndentedString(issuedTokenType)).append("\n");
sb.append(" refreshToken: ").append(toIndentedString(refreshToken)).append("\n");
sb.append(" scope: ").append(toIndentedString(scope)).append("\n");
sb.append(" tokenType: ").append(toIndentedString(tokenType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy