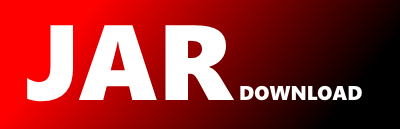
com.okta.sdk.resource.model.UserFactorLinks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.HrefObjectSelfLink;
import com.okta.sdk.resource.model.LinksActivateActivate;
import com.okta.sdk.resource.model.LinksCancelCancel;
import com.okta.sdk.resource.model.LinksDeactivateDeactivate;
import com.okta.sdk.resource.model.LinksEnrollEnroll;
import com.okta.sdk.resource.model.LinksFactorFactor;
import com.okta.sdk.resource.model.LinksPollPoll;
import com.okta.sdk.resource.model.LinksQrcodeQrcode;
import com.okta.sdk.resource.model.LinksQuestionsQuestion;
import com.okta.sdk.resource.model.LinksResendResend;
import com.okta.sdk.resource.model.LinksSendSend;
import com.okta.sdk.resource.model.LinksUserUser;
import com.okta.sdk.resource.model.LinksVerifyVerify;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* UserFactorLinks
*/
@JsonPropertyOrder({ UserFactorLinks.JSON_PROPERTY_ACTIVATE, UserFactorLinks.JSON_PROPERTY_CANCEL,
UserFactorLinks.JSON_PROPERTY_DEACTIVATE, UserFactorLinks.JSON_PROPERTY_ENROLL,
UserFactorLinks.JSON_PROPERTY_FACTOR, UserFactorLinks.JSON_PROPERTY_POLL, UserFactorLinks.JSON_PROPERTY_QRCODE,
UserFactorLinks.JSON_PROPERTY_QUESTION, UserFactorLinks.JSON_PROPERTY_RESEND,
UserFactorLinks.JSON_PROPERTY_SEND, UserFactorLinks.JSON_PROPERTY_SELF, UserFactorLinks.JSON_PROPERTY_USER,
UserFactorLinks.JSON_PROPERTY_VERIFY })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserFactorLinks implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACTIVATE = "activate";
private LinksActivateActivate activate;
public static final String JSON_PROPERTY_CANCEL = "cancel";
private LinksCancelCancel cancel;
public static final String JSON_PROPERTY_DEACTIVATE = "deactivate";
private LinksDeactivateDeactivate deactivate;
public static final String JSON_PROPERTY_ENROLL = "enroll";
private LinksEnrollEnroll enroll;
public static final String JSON_PROPERTY_FACTOR = "factor";
private LinksFactorFactor factor;
public static final String JSON_PROPERTY_POLL = "poll";
private LinksPollPoll poll;
public static final String JSON_PROPERTY_QRCODE = "qrcode";
private LinksQrcodeQrcode qrcode;
public static final String JSON_PROPERTY_QUESTION = "question";
private LinksQuestionsQuestion question;
public static final String JSON_PROPERTY_RESEND = "resend";
private LinksResendResend resend;
public static final String JSON_PROPERTY_SEND = "send";
private LinksSendSend send;
public static final String JSON_PROPERTY_SELF = "self";
private HrefObjectSelfLink self;
public static final String JSON_PROPERTY_USER = "user";
private LinksUserUser user;
public static final String JSON_PROPERTY_VERIFY = "verify";
private LinksVerifyVerify verify;
public UserFactorLinks() {
}
public UserFactorLinks activate(LinksActivateActivate activate) {
this.activate = activate;
return this;
}
/**
* Get activate
*
* @return activate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksActivateActivate getActivate() {
return activate;
}
@JsonProperty(JSON_PROPERTY_ACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActivate(LinksActivateActivate activate) {
this.activate = activate;
}
public UserFactorLinks cancel(LinksCancelCancel cancel) {
this.cancel = cancel;
return this;
}
/**
* Get cancel
*
* @return cancel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CANCEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksCancelCancel getCancel() {
return cancel;
}
@JsonProperty(JSON_PROPERTY_CANCEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancel(LinksCancelCancel cancel) {
this.cancel = cancel;
}
public UserFactorLinks deactivate(LinksDeactivateDeactivate deactivate) {
this.deactivate = deactivate;
return this;
}
/**
* Get deactivate
*
* @return deactivate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DEACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksDeactivateDeactivate getDeactivate() {
return deactivate;
}
@JsonProperty(JSON_PROPERTY_DEACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeactivate(LinksDeactivateDeactivate deactivate) {
this.deactivate = deactivate;
}
public UserFactorLinks enroll(LinksEnrollEnroll enroll) {
this.enroll = enroll;
return this;
}
/**
* Get enroll
*
* @return enroll
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ENROLL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksEnrollEnroll getEnroll() {
return enroll;
}
@JsonProperty(JSON_PROPERTY_ENROLL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnroll(LinksEnrollEnroll enroll) {
this.enroll = enroll;
}
public UserFactorLinks factor(LinksFactorFactor factor) {
this.factor = factor;
return this;
}
/**
* Get factor
*
* @return factor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_FACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksFactorFactor getFactor() {
return factor;
}
@JsonProperty(JSON_PROPERTY_FACTOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFactor(LinksFactorFactor factor) {
this.factor = factor;
}
public UserFactorLinks poll(LinksPollPoll poll) {
this.poll = poll;
return this;
}
/**
* Get poll
*
* @return poll
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_POLL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksPollPoll getPoll() {
return poll;
}
@JsonProperty(JSON_PROPERTY_POLL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPoll(LinksPollPoll poll) {
this.poll = poll;
}
public UserFactorLinks qrcode(LinksQrcodeQrcode qrcode) {
this.qrcode = qrcode;
return this;
}
/**
* Get qrcode
*
* @return qrcode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_QRCODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksQrcodeQrcode getQrcode() {
return qrcode;
}
@JsonProperty(JSON_PROPERTY_QRCODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setQrcode(LinksQrcodeQrcode qrcode) {
this.qrcode = qrcode;
}
public UserFactorLinks question(LinksQuestionsQuestion question) {
this.question = question;
return this;
}
/**
* Get question
*
* @return question
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_QUESTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksQuestionsQuestion getQuestion() {
return question;
}
@JsonProperty(JSON_PROPERTY_QUESTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setQuestion(LinksQuestionsQuestion question) {
this.question = question;
}
public UserFactorLinks resend(LinksResendResend resend) {
this.resend = resend;
return this;
}
/**
* Get resend
*
* @return resend
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_RESEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksResendResend getResend() {
return resend;
}
@JsonProperty(JSON_PROPERTY_RESEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResend(LinksResendResend resend) {
this.resend = resend;
}
public UserFactorLinks send(LinksSendSend send) {
this.send = send;
return this;
}
/**
* Get send
*
* @return send
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksSendSend getSend() {
return send;
}
@JsonProperty(JSON_PROPERTY_SEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSend(LinksSendSend send) {
this.send = send;
}
public UserFactorLinks self(HrefObjectSelfLink self) {
this.self = self;
return this;
}
/**
* Get self
*
* @return self
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObjectSelfLink getSelf() {
return self;
}
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSelf(HrefObjectSelfLink self) {
this.self = self;
}
public UserFactorLinks user(LinksUserUser user) {
this.user = user;
return this;
}
/**
* Get user
*
* @return user
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_USER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksUserUser getUser() {
return user;
}
@JsonProperty(JSON_PROPERTY_USER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUser(LinksUserUser user) {
this.user = user;
}
public UserFactorLinks verify(LinksVerifyVerify verify) {
this.verify = verify;
return this;
}
/**
* Get verify
*
* @return verify
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_VERIFY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LinksVerifyVerify getVerify() {
return verify;
}
@JsonProperty(JSON_PROPERTY_VERIFY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVerify(LinksVerifyVerify verify) {
this.verify = verify;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserFactorLinks userFactorLinks = (UserFactorLinks) o;
return Objects.equals(this.activate, userFactorLinks.activate)
&& Objects.equals(this.cancel, userFactorLinks.cancel)
&& Objects.equals(this.deactivate, userFactorLinks.deactivate)
&& Objects.equals(this.enroll, userFactorLinks.enroll)
&& Objects.equals(this.factor, userFactorLinks.factor)
&& Objects.equals(this.poll, userFactorLinks.poll)
&& Objects.equals(this.qrcode, userFactorLinks.qrcode)
&& Objects.equals(this.question, userFactorLinks.question)
&& Objects.equals(this.resend, userFactorLinks.resend)
&& Objects.equals(this.send, userFactorLinks.send) && Objects.equals(this.self, userFactorLinks.self)
&& Objects.equals(this.user, userFactorLinks.user)
&& Objects.equals(this.verify, userFactorLinks.verify);
// ;
}
@Override
public int hashCode() {
return Objects.hash(activate, cancel, deactivate, enroll, factor, poll, qrcode, question, resend, send, self,
user, verify);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserFactorLinks {\n");
sb.append(" activate: ").append(toIndentedString(activate)).append("\n");
sb.append(" cancel: ").append(toIndentedString(cancel)).append("\n");
sb.append(" deactivate: ").append(toIndentedString(deactivate)).append("\n");
sb.append(" enroll: ").append(toIndentedString(enroll)).append("\n");
sb.append(" factor: ").append(toIndentedString(factor)).append("\n");
sb.append(" poll: ").append(toIndentedString(poll)).append("\n");
sb.append(" qrcode: ").append(toIndentedString(qrcode)).append("\n");
sb.append(" question: ").append(toIndentedString(question)).append("\n");
sb.append(" resend: ").append(toIndentedString(resend)).append("\n");
sb.append(" send: ").append(toIndentedString(send)).append("\n");
sb.append(" self: ").append(toIndentedString(self)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" verify: ").append(toIndentedString(verify)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy