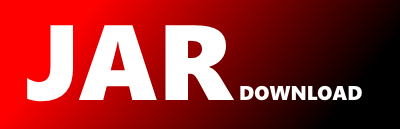
com.okta.sdk.resource.model.UserGetSingleton Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.UserCredentials;
import com.okta.sdk.resource.model.UserGetSingletonAllOfEmbedded;
import com.okta.sdk.resource.model.UserLinks;
import com.okta.sdk.resource.model.UserProfile;
import com.okta.sdk.resource.model.UserStatus;
import com.okta.sdk.resource.model.UserType;
import java.time.OffsetDateTime;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* UserGetSingleton
*/
@JsonPropertyOrder({ UserGetSingleton.JSON_PROPERTY_ACTIVATED, UserGetSingleton.JSON_PROPERTY_CREATED,
UserGetSingleton.JSON_PROPERTY_CREDENTIALS, UserGetSingleton.JSON_PROPERTY_ID,
UserGetSingleton.JSON_PROPERTY_LAST_LOGIN, UserGetSingleton.JSON_PROPERTY_LAST_UPDATED,
UserGetSingleton.JSON_PROPERTY_PASSWORD_CHANGED, UserGetSingleton.JSON_PROPERTY_PROFILE,
UserGetSingleton.JSON_PROPERTY_REALM_ID, UserGetSingleton.JSON_PROPERTY_STATUS,
UserGetSingleton.JSON_PROPERTY_STATUS_CHANGED, UserGetSingleton.JSON_PROPERTY_TRANSITIONING_TO_STATUS,
UserGetSingleton.JSON_PROPERTY_TYPE, UserGetSingleton.JSON_PROPERTY_EMBEDDED,
UserGetSingleton.JSON_PROPERTY_LINKS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserGetSingleton implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACTIVATED = "activated";
private JsonNullable activated = JsonNullable. undefined();
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_CREDENTIALS = "credentials";
private UserCredentials credentials;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LAST_LOGIN = "lastLogin";
private JsonNullable lastLogin = JsonNullable. undefined();
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private OffsetDateTime lastUpdated;
public static final String JSON_PROPERTY_PASSWORD_CHANGED = "passwordChanged";
private JsonNullable passwordChanged = JsonNullable. undefined();
public static final String JSON_PROPERTY_PROFILE = "profile";
private UserProfile profile;
public static final String JSON_PROPERTY_REALM_ID = "realmId";
private String realmId;
public static final String JSON_PROPERTY_STATUS = "status";
private UserStatus status;
public static final String JSON_PROPERTY_STATUS_CHANGED = "statusChanged";
private JsonNullable statusChanged = JsonNullable. undefined();
public static final String JSON_PROPERTY_TRANSITIONING_TO_STATUS = "transitioningToStatus";
private UserStatus transitioningToStatus;
public static final String JSON_PROPERTY_TYPE = "type";
private UserType type;
public static final String JSON_PROPERTY_EMBEDDED = "_embedded";
private UserGetSingletonAllOfEmbedded embedded;
public static final String JSON_PROPERTY_LINKS = "_links";
private UserLinks links;
public UserGetSingleton() {
}
/*
* @JsonCreator public UserGetSingleton(
*
* @JsonProperty(JSON_PROPERTY_ACTIVATED) OffsetDateTime activated,
*
* @JsonProperty(JSON_PROPERTY_CREATED) OffsetDateTime created,
*
* @JsonProperty(JSON_PROPERTY_ID) String id,
*
* @JsonProperty(JSON_PROPERTY_LAST_LOGIN) OffsetDateTime lastLogin,
*
* @JsonProperty(JSON_PROPERTY_LAST_UPDATED) OffsetDateTime lastUpdated,
*
* @JsonProperty(JSON_PROPERTY_PASSWORD_CHANGED) OffsetDateTime passwordChanged,
*
* @JsonProperty(JSON_PROPERTY_REALM_ID) String realmId,
*
* @JsonProperty(JSON_PROPERTY_STATUS_CHANGED) OffsetDateTime statusChanged ) { this(); this.activated = activated;
* this.created = created; this.id = id; this.lastLogin = lastLogin; this.lastUpdated = lastUpdated;
* this.passwordChanged = passwordChanged; this.realmId = realmId; this.statusChanged = statusChanged; }
*/
/**
* The timestamp when the User status transitioned to `ACTIVE`
*
* @return activated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The timestamp when the User status transitioned to `ACTIVE`")
@JsonIgnore
public OffsetDateTime getActivated() {
if (activated == null) {
activated = JsonNullable. undefined();
}
return activated.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getActivated_JsonNullable() {
return activated;
}
@JsonProperty(JSON_PROPERTY_ACTIVATED)
private void setActivated_JsonNullable(JsonNullable activated) {
this.activated = activated;
}
/**
* The timestamp when the User was created
*
* @return created
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The timestamp when the User was created")
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreated() {
return created;
}
public UserGetSingleton credentials(UserCredentials credentials) {
this.credentials = credentials;
return this;
}
/**
* Get credentials
*
* @return credentials
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserCredentials getCredentials() {
return credentials;
}
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCredentials(UserCredentials credentials) {
this.credentials = credentials;
}
/**
* The unique key for the user
*
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique key for the user")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
/**
* The timestamp of the last login
*
* @return lastLogin
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The timestamp of the last login")
@JsonIgnore
public OffsetDateTime getLastLogin() {
if (lastLogin == null) {
lastLogin = JsonNullable. undefined();
}
return lastLogin.orElse(null);
}
@JsonProperty(JSON_PROPERTY_LAST_LOGIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getLastLogin_JsonNullable() {
return lastLogin;
}
@JsonProperty(JSON_PROPERTY_LAST_LOGIN)
private void setLastLogin_JsonNullable(JsonNullable lastLogin) {
this.lastLogin = lastLogin;
}
/**
* The timestamp when the User was last updated
*
* @return lastUpdated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The timestamp when the User was last updated")
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
/**
* The timestamp when the User's password was last updated
*
* @return passwordChanged
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The timestamp when the User's password was last updated")
@JsonIgnore
public OffsetDateTime getPasswordChanged() {
if (passwordChanged == null) {
passwordChanged = JsonNullable. undefined();
}
return passwordChanged.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PASSWORD_CHANGED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getPasswordChanged_JsonNullable() {
return passwordChanged;
}
@JsonProperty(JSON_PROPERTY_PASSWORD_CHANGED)
private void setPasswordChanged_JsonNullable(JsonNullable passwordChanged) {
this.passwordChanged = passwordChanged;
}
public UserGetSingleton profile(UserProfile profile) {
this.profile = profile;
return this;
}
/**
* Get profile
*
* @return profile
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserProfile getProfile() {
return profile;
}
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProfile(UserProfile profile) {
this.profile = profile;
}
/**
* <div class=\"x-lifecycle-container\"><x-lifecycle
* class=\"ea\"></x-lifecycle></div>The ID of the Realm in which the User is residing
*
* @return realmId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "guo1bfiNtSnZYILxO0g4", value = " The ID of the Realm in which the User is residing")
@JsonProperty(JSON_PROPERTY_REALM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRealmId() {
return realmId;
}
public UserGetSingleton status(UserStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatus(UserStatus status) {
this.status = status;
}
/**
* The timestamp when the status of the User last changed
*
* @return statusChanged
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The timestamp when the status of the User last changed")
@JsonIgnore
public OffsetDateTime getStatusChanged() {
if (statusChanged == null) {
statusChanged = JsonNullable. undefined();
}
return statusChanged.orElse(null);
}
@JsonProperty(JSON_PROPERTY_STATUS_CHANGED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getStatusChanged_JsonNullable() {
return statusChanged;
}
@JsonProperty(JSON_PROPERTY_STATUS_CHANGED)
private void setStatusChanged_JsonNullable(JsonNullable statusChanged) {
this.statusChanged = statusChanged;
}
public UserGetSingleton transitioningToStatus(UserStatus transitioningToStatus) {
this.transitioningToStatus = transitioningToStatus;
return this;
}
/**
* Get transitioningToStatus
*
* @return transitioningToStatus
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TRANSITIONING_TO_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserStatus getTransitioningToStatus() {
return transitioningToStatus;
}
@JsonProperty(JSON_PROPERTY_TRANSITIONING_TO_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTransitioningToStatus(UserStatus transitioningToStatus) {
this.transitioningToStatus = transitioningToStatus;
}
public UserGetSingleton type(UserType type) {
this.type = type;
return this;
}
/**
* Get type
*
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserType getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(UserType type) {
this.type = type;
}
public UserGetSingleton embedded(UserGetSingletonAllOfEmbedded embedded) {
this.embedded = embedded;
return this;
}
/**
* Get embedded
*
* @return embedded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserGetSingletonAllOfEmbedded getEmbedded() {
return embedded;
}
@JsonProperty(JSON_PROPERTY_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEmbedded(UserGetSingletonAllOfEmbedded embedded) {
this.embedded = embedded;
}
public UserGetSingleton links(UserLinks links) {
this.links = links;
return this;
}
/**
* Get links
*
* @return links
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UserLinks getLinks() {
return links;
}
@JsonProperty(JSON_PROPERTY_LINKS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLinks(UserLinks links) {
this.links = links;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserGetSingleton userGetSingleton = (UserGetSingleton) o;
return equalsNullable(this.activated, userGetSingleton.activated)
&& Objects.equals(this.created, userGetSingleton.created)
&& Objects.equals(this.credentials, userGetSingleton.credentials)
&& Objects.equals(this.id, userGetSingleton.id)
&& equalsNullable(this.lastLogin, userGetSingleton.lastLogin)
&& Objects.equals(this.lastUpdated, userGetSingleton.lastUpdated)
&& equalsNullable(this.passwordChanged, userGetSingleton.passwordChanged)
&& Objects.equals(this.profile, userGetSingleton.profile)
&& Objects.equals(this.realmId, userGetSingleton.realmId)
&& Objects.equals(this.status, userGetSingleton.status)
&& equalsNullable(this.statusChanged, userGetSingleton.statusChanged)
&& Objects.equals(this.transitioningToStatus, userGetSingleton.transitioningToStatus)
&& Objects.equals(this.type, userGetSingleton.type)
&& Objects.equals(this.embedded, userGetSingleton.embedded)
&& Objects.equals(this.links, userGetSingleton.links);
// ;
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(activated), created, credentials, id, hashCodeNullable(lastLogin),
lastUpdated, hashCodeNullable(passwordChanged), profile, realmId, status,
hashCodeNullable(statusChanged), transitioningToStatus, type, embedded, links);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserGetSingleton {\n");
sb.append(" activated: ").append(toIndentedString(activated)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" credentials: ").append(toIndentedString(credentials)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lastLogin: ").append(toIndentedString(lastLogin)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" passwordChanged: ").append(toIndentedString(passwordChanged)).append("\n");
sb.append(" profile: ").append(toIndentedString(profile)).append("\n");
sb.append(" realmId: ").append(toIndentedString(realmId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" statusChanged: ").append(toIndentedString(statusChanged)).append("\n");
sb.append(" transitioningToStatus: ").append(toIndentedString(transitioningToStatus)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" embedded: ").append(toIndentedString(embedded)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy