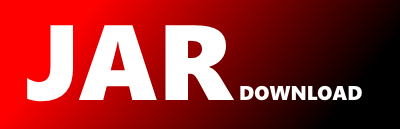
com.okta.sdk.resource.model.UserLinks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.HrefObject;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Specifies link relations (see [Web Linking](https://datatracker.ietf.org/doc/html/rfc8288) available for the current
* status of a user. The Links object is used for dynamic discovery of related resources, lifecycle operations, and
* credential operations. The Links object is read-only. For an individual User result, the Links object contains a full
* set of link relations available for that User as determined by your policies. For a collection of users, the Links
* object contains only the `self` link. Operations that return a collection of Users include List Users and
* List Group Members.
*/
@ApiModel(description = "Specifies link relations (see [Web Linking](https://datatracker.ietf.org/doc/html/rfc8288) available for the current status of a user. The Links object is used for dynamic discovery of related resources, lifecycle operations, and credential operations. The Links object is read-only. For an individual User result, the Links object contains a full set of link relations available for that User as determined by your policies. For a collection of users, the Links object contains only the `self` link. Operations that return a collection of Users include List Users and List Group Members.")
@JsonPropertyOrder({ UserLinks.JSON_PROPERTY_SELF, UserLinks.JSON_PROPERTY_ACTIVATE,
UserLinks.JSON_PROPERTY_RESET_PASSWORD, UserLinks.JSON_PROPERTY_RESET_FACTORS,
UserLinks.JSON_PROPERTY_EXPIRE_PASSWORD, UserLinks.JSON_PROPERTY_FORGOT_PASSWORD,
UserLinks.JSON_PROPERTY_CHANGE_RECOVERY_QUESTION, UserLinks.JSON_PROPERTY_DEACTIVATE,
UserLinks.JSON_PROPERTY_REACTIVATE, UserLinks.JSON_PROPERTY_CHANGE_PASSWORD, UserLinks.JSON_PROPERTY_SCHEMA,
UserLinks.JSON_PROPERTY_SUSPEND, UserLinks.JSON_PROPERTY_UNSUSPEND, UserLinks.JSON_PROPERTY_UNLOCK,
UserLinks.JSON_PROPERTY_TYPE })
@JsonTypeName("User__links")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserLinks implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_SELF = "self";
private HrefObject self;
public static final String JSON_PROPERTY_ACTIVATE = "activate";
private HrefObject activate;
public static final String JSON_PROPERTY_RESET_PASSWORD = "resetPassword";
private HrefObject resetPassword;
public static final String JSON_PROPERTY_RESET_FACTORS = "resetFactors";
private HrefObject resetFactors;
public static final String JSON_PROPERTY_EXPIRE_PASSWORD = "expirePassword";
private HrefObject expirePassword;
public static final String JSON_PROPERTY_FORGOT_PASSWORD = "forgotPassword";
private HrefObject forgotPassword;
public static final String JSON_PROPERTY_CHANGE_RECOVERY_QUESTION = "changeRecoveryQuestion";
private HrefObject changeRecoveryQuestion;
public static final String JSON_PROPERTY_DEACTIVATE = "deactivate";
private HrefObject deactivate;
public static final String JSON_PROPERTY_REACTIVATE = "reactivate";
private HrefObject reactivate;
public static final String JSON_PROPERTY_CHANGE_PASSWORD = "changePassword";
private HrefObject changePassword;
public static final String JSON_PROPERTY_SCHEMA = "schema";
private HrefObject schema;
public static final String JSON_PROPERTY_SUSPEND = "suspend";
private HrefObject suspend;
public static final String JSON_PROPERTY_UNSUSPEND = "unsuspend";
private HrefObject unsuspend;
public static final String JSON_PROPERTY_UNLOCK = "unlock";
private HrefObject unlock;
public static final String JSON_PROPERTY_TYPE = "type";
private HrefObject type;
public UserLinks() {
}
public UserLinks self(HrefObject self) {
this.self = self;
return this;
}
/**
* URL to the individual user
*
* @return self
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to the individual user")
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getSelf() {
return self;
}
@JsonProperty(JSON_PROPERTY_SELF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSelf(HrefObject self) {
this.self = self;
}
public UserLinks activate(HrefObject activate) {
this.activate = activate;
return this;
}
/**
* URL to activate the user
*
* @return activate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to activate the user")
@JsonProperty(JSON_PROPERTY_ACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getActivate() {
return activate;
}
@JsonProperty(JSON_PROPERTY_ACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActivate(HrefObject activate) {
this.activate = activate;
}
public UserLinks resetPassword(HrefObject resetPassword) {
this.resetPassword = resetPassword;
return this;
}
/**
* URL to reset the User's password
*
* @return resetPassword
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to reset the User's password")
@JsonProperty(JSON_PROPERTY_RESET_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getResetPassword() {
return resetPassword;
}
@JsonProperty(JSON_PROPERTY_RESET_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResetPassword(HrefObject resetPassword) {
this.resetPassword = resetPassword;
}
public UserLinks resetFactors(HrefObject resetFactors) {
this.resetFactors = resetFactors;
return this;
}
/**
* URL to reset the User's factors
*
* @return resetFactors
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to reset the User's factors")
@JsonProperty(JSON_PROPERTY_RESET_FACTORS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getResetFactors() {
return resetFactors;
}
@JsonProperty(JSON_PROPERTY_RESET_FACTORS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResetFactors(HrefObject resetFactors) {
this.resetFactors = resetFactors;
}
public UserLinks expirePassword(HrefObject expirePassword) {
this.expirePassword = expirePassword;
return this;
}
/**
* URL to expire the User's password
*
* @return expirePassword
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to expire the User's password")
@JsonProperty(JSON_PROPERTY_EXPIRE_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getExpirePassword() {
return expirePassword;
}
@JsonProperty(JSON_PROPERTY_EXPIRE_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExpirePassword(HrefObject expirePassword) {
this.expirePassword = expirePassword;
}
public UserLinks forgotPassword(HrefObject forgotPassword) {
this.forgotPassword = forgotPassword;
return this;
}
/**
* URL to initiate a forgot password operation
*
* @return forgotPassword
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to initiate a forgot password operation")
@JsonProperty(JSON_PROPERTY_FORGOT_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getForgotPassword() {
return forgotPassword;
}
@JsonProperty(JSON_PROPERTY_FORGOT_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setForgotPassword(HrefObject forgotPassword) {
this.forgotPassword = forgotPassword;
}
public UserLinks changeRecoveryQuestion(HrefObject changeRecoveryQuestion) {
this.changeRecoveryQuestion = changeRecoveryQuestion;
return this;
}
/**
* URL to change the User's recovery question
*
* @return changeRecoveryQuestion
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to change the User's recovery question")
@JsonProperty(JSON_PROPERTY_CHANGE_RECOVERY_QUESTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getChangeRecoveryQuestion() {
return changeRecoveryQuestion;
}
@JsonProperty(JSON_PROPERTY_CHANGE_RECOVERY_QUESTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setChangeRecoveryQuestion(HrefObject changeRecoveryQuestion) {
this.changeRecoveryQuestion = changeRecoveryQuestion;
}
public UserLinks deactivate(HrefObject deactivate) {
this.deactivate = deactivate;
return this;
}
/**
* URL to deactivate a user
*
* @return deactivate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to deactivate a user")
@JsonProperty(JSON_PROPERTY_DEACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getDeactivate() {
return deactivate;
}
@JsonProperty(JSON_PROPERTY_DEACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeactivate(HrefObject deactivate) {
this.deactivate = deactivate;
}
public UserLinks reactivate(HrefObject reactivate) {
this.reactivate = reactivate;
return this;
}
/**
* URL to reactivate the user
*
* @return reactivate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to reactivate the user")
@JsonProperty(JSON_PROPERTY_REACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getReactivate() {
return reactivate;
}
@JsonProperty(JSON_PROPERTY_REACTIVATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReactivate(HrefObject reactivate) {
this.reactivate = reactivate;
}
public UserLinks changePassword(HrefObject changePassword) {
this.changePassword = changePassword;
return this;
}
/**
* URL to change the User's password
*
* @return changePassword
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to change the User's password")
@JsonProperty(JSON_PROPERTY_CHANGE_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getChangePassword() {
return changePassword;
}
@JsonProperty(JSON_PROPERTY_CHANGE_PASSWORD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setChangePassword(HrefObject changePassword) {
this.changePassword = changePassword;
}
public UserLinks schema(HrefObject schema) {
this.schema = schema;
return this;
}
/**
* URL to the User's profile schema
*
* @return schema
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to the User's profile schema")
@JsonProperty(JSON_PROPERTY_SCHEMA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getSchema() {
return schema;
}
@JsonProperty(JSON_PROPERTY_SCHEMA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSchema(HrefObject schema) {
this.schema = schema;
}
public UserLinks suspend(HrefObject suspend) {
this.suspend = suspend;
return this;
}
/**
* URL to suspend the user
*
* @return suspend
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to suspend the user")
@JsonProperty(JSON_PROPERTY_SUSPEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getSuspend() {
return suspend;
}
@JsonProperty(JSON_PROPERTY_SUSPEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSuspend(HrefObject suspend) {
this.suspend = suspend;
}
public UserLinks unsuspend(HrefObject unsuspend) {
this.unsuspend = unsuspend;
return this;
}
/**
* URL to unsuspend the user
*
* @return unsuspend
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to unsuspend the user")
@JsonProperty(JSON_PROPERTY_UNSUSPEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getUnsuspend() {
return unsuspend;
}
@JsonProperty(JSON_PROPERTY_UNSUSPEND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUnsuspend(HrefObject unsuspend) {
this.unsuspend = unsuspend;
}
public UserLinks unlock(HrefObject unlock) {
this.unlock = unlock;
return this;
}
/**
* URL to unlock the locked-out user
*
* @return unlock
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to unlock the locked-out user")
@JsonProperty(JSON_PROPERTY_UNLOCK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getUnlock() {
return unlock;
}
@JsonProperty(JSON_PROPERTY_UNLOCK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUnlock(HrefObject unlock) {
this.unlock = unlock;
}
public UserLinks type(HrefObject type) {
this.type = type;
return this;
}
/**
* URL to the User Type
*
* @return type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to the User Type")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HrefObject getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(HrefObject type) {
this.type = type;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserLinks userLinks = (UserLinks) o;
return Objects.equals(this.self, userLinks.self) && Objects.equals(this.activate, userLinks.activate)
&& Objects.equals(this.resetPassword, userLinks.resetPassword)
&& Objects.equals(this.resetFactors, userLinks.resetFactors)
&& Objects.equals(this.expirePassword, userLinks.expirePassword)
&& Objects.equals(this.forgotPassword, userLinks.forgotPassword)
&& Objects.equals(this.changeRecoveryQuestion, userLinks.changeRecoveryQuestion)
&& Objects.equals(this.deactivate, userLinks.deactivate)
&& Objects.equals(this.reactivate, userLinks.reactivate)
&& Objects.equals(this.changePassword, userLinks.changePassword)
&& Objects.equals(this.schema, userLinks.schema) && Objects.equals(this.suspend, userLinks.suspend)
&& Objects.equals(this.unsuspend, userLinks.unsuspend) && Objects.equals(this.unlock, userLinks.unlock)
&& Objects.equals(this.type, userLinks.type);
// ;
}
@Override
public int hashCode() {
return Objects.hash(self, activate, resetPassword, resetFactors, expirePassword, forgotPassword,
changeRecoveryQuestion, deactivate, reactivate, changePassword, schema, suspend, unsuspend, unlock,
type);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserLinks {\n");
sb.append(" self: ").append(toIndentedString(self)).append("\n");
sb.append(" activate: ").append(toIndentedString(activate)).append("\n");
sb.append(" resetPassword: ").append(toIndentedString(resetPassword)).append("\n");
sb.append(" resetFactors: ").append(toIndentedString(resetFactors)).append("\n");
sb.append(" expirePassword: ").append(toIndentedString(expirePassword)).append("\n");
sb.append(" forgotPassword: ").append(toIndentedString(forgotPassword)).append("\n");
sb.append(" changeRecoveryQuestion: ").append(toIndentedString(changeRecoveryQuestion)).append("\n");
sb.append(" deactivate: ").append(toIndentedString(deactivate)).append("\n");
sb.append(" reactivate: ").append(toIndentedString(reactivate)).append("\n");
sb.append(" changePassword: ").append(toIndentedString(changePassword)).append("\n");
sb.append(" schema: ").append(toIndentedString(schema)).append("\n");
sb.append(" suspend: ").append(toIndentedString(suspend)).append("\n");
sb.append(" unsuspend: ").append(toIndentedString(unsuspend)).append("\n");
sb.append(" unlock: ").append(toIndentedString(unlock)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy