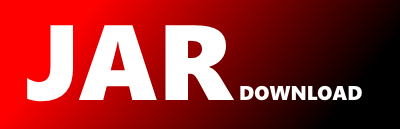
com.okta.sdk.resource.model.UserProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.HashMap;
import java.util.Map;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Specifies the default and custom profile properties for a user. The default user profile is based on the [System for
* Cross-domain Identity Management: Core Schema](https://datatracker.ietf.org/doc/html/rfc7643). The only permitted
* customizations of the default profile are to update permissions, change whether the `firstName` and
* `lastName` properties are nullable, and specify a
* [pattern](https://developer.okta.com/docs/reference/api/schemas/#login-pattern-validation) for `login`. You
* can use the Profile Editor in the Admin Console or the [Schemas
* API](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UISchema/#tag/UISchema) to make
* schema modifications. You can extend user profiles with custom properties. You must first add the custom property to
* the user profile schema before you reference it. You can use the Profile Editor in the Admin console or the [Schemas
* API](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UISchema/#tag/UISchema) to manage
* schema extensions. Custom attributes can contain HTML tags. It's the client's responsibility to escape or
* encode this data before displaying it. Use
* [best-practices](https://cheatsheetseries.owasp.org/cheatsheets/Cross_Site_Scripting_Prevention_Cheat_Sheet.html) to
* prevent cross-site scripting.
*/
@ApiModel(description = "Specifies the default and custom profile properties for a user. The default user profile is based on the [System for Cross-domain Identity Management: Core Schema](https://datatracker.ietf.org/doc/html/rfc7643). The only permitted customizations of the default profile are to update permissions, change whether the `firstName` and `lastName` properties are nullable, and specify a [pattern](https://developer.okta.com/docs/reference/api/schemas/#login-pattern-validation) for `login`. You can use the Profile Editor in the Admin Console or the [Schemas API](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UISchema/#tag/UISchema) to make schema modifications. You can extend user profiles with custom properties. You must first add the custom property to the user profile schema before you reference it. You can use the Profile Editor in the Admin console or the [Schemas API](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UISchema/#tag/UISchema) to manage schema extensions. Custom attributes can contain HTML tags. It's the client's responsibility to escape or encode this data before displaying it. Use [best-practices](https://cheatsheetseries.owasp.org/cheatsheets/Cross_Site_Scripting_Prevention_Cheat_Sheet.html) to prevent cross-site scripting.")
@JsonPropertyOrder({ UserProfile.JSON_PROPERTY_CITY, UserProfile.JSON_PROPERTY_COST_CENTER,
UserProfile.JSON_PROPERTY_COUNTRY_CODE, UserProfile.JSON_PROPERTY_DEPARTMENT,
UserProfile.JSON_PROPERTY_DISPLAY_NAME, UserProfile.JSON_PROPERTY_DIVISION, UserProfile.JSON_PROPERTY_EMAIL,
UserProfile.JSON_PROPERTY_EMPLOYEE_NUMBER, UserProfile.JSON_PROPERTY_FIRST_NAME,
UserProfile.JSON_PROPERTY_HONORIFIC_PREFIX, UserProfile.JSON_PROPERTY_HONORIFIC_SUFFIX,
UserProfile.JSON_PROPERTY_LAST_NAME, UserProfile.JSON_PROPERTY_LOCALE, UserProfile.JSON_PROPERTY_LOGIN,
UserProfile.JSON_PROPERTY_MANAGER, UserProfile.JSON_PROPERTY_MANAGER_ID, UserProfile.JSON_PROPERTY_MIDDLE_NAME,
UserProfile.JSON_PROPERTY_MOBILE_PHONE, UserProfile.JSON_PROPERTY_NICK_NAME,
UserProfile.JSON_PROPERTY_ORGANIZATION, UserProfile.JSON_PROPERTY_POSTAL_ADDRESS,
UserProfile.JSON_PROPERTY_PREFERRED_LANGUAGE, UserProfile.JSON_PROPERTY_PRIMARY_PHONE,
UserProfile.JSON_PROPERTY_PROFILE_URL, UserProfile.JSON_PROPERTY_SECOND_EMAIL, UserProfile.JSON_PROPERTY_STATE,
UserProfile.JSON_PROPERTY_STREET_ADDRESS, UserProfile.JSON_PROPERTY_TIMEZONE, UserProfile.JSON_PROPERTY_TITLE,
UserProfile.JSON_PROPERTY_USER_TYPE, UserProfile.JSON_PROPERTY_ZIP_CODE })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class UserProfile implements Serializable {
private static final long serialVersionUID = 1L;
public Map additionalProperties = new java.util.LinkedHashMap<>();
public Map getAdditionalProperties() {
return additionalProperties;
}
public void setAdditionalProperties(Map additionalProperties) {
this.additionalProperties = additionalProperties;
}
public static final String JSON_PROPERTY_CITY = "city";
private JsonNullable city = JsonNullable. undefined();
public static final String JSON_PROPERTY_COST_CENTER = "costCenter";
private JsonNullable costCenter = JsonNullable. undefined();
public static final String JSON_PROPERTY_COUNTRY_CODE = "countryCode";
private JsonNullable countryCode = JsonNullable. undefined();
public static final String JSON_PROPERTY_DEPARTMENT = "department";
private String department;
public static final String JSON_PROPERTY_DISPLAY_NAME = "displayName";
private JsonNullable displayName = JsonNullable. undefined();
public static final String JSON_PROPERTY_DIVISION = "division";
private JsonNullable division = JsonNullable. undefined();
public static final String JSON_PROPERTY_EMAIL = "email";
private String email;
public static final String JSON_PROPERTY_EMPLOYEE_NUMBER = "employeeNumber";
private String employeeNumber;
public static final String JSON_PROPERTY_FIRST_NAME = "firstName";
private JsonNullable firstName = JsonNullable. undefined();
public static final String JSON_PROPERTY_HONORIFIC_PREFIX = "honorificPrefix";
private JsonNullable honorificPrefix = JsonNullable. undefined();
public static final String JSON_PROPERTY_HONORIFIC_SUFFIX = "honorificSuffix";
private JsonNullable honorificSuffix = JsonNullable. undefined();
public static final String JSON_PROPERTY_LAST_NAME = "lastName";
private JsonNullable lastName = JsonNullable. undefined();
public static final String JSON_PROPERTY_LOCALE = "locale";
private String locale;
public static final String JSON_PROPERTY_LOGIN = "login";
private String login;
public static final String JSON_PROPERTY_MANAGER = "manager";
private JsonNullable manager = JsonNullable. undefined();
public static final String JSON_PROPERTY_MANAGER_ID = "managerId";
private JsonNullable managerId = JsonNullable. undefined();
public static final String JSON_PROPERTY_MIDDLE_NAME = "middleName";
private JsonNullable middleName = JsonNullable. undefined();
public static final String JSON_PROPERTY_MOBILE_PHONE = "mobilePhone";
private JsonNullable mobilePhone = JsonNullable. undefined();
public static final String JSON_PROPERTY_NICK_NAME = "nickName";
private JsonNullable nickName = JsonNullable. undefined();
public static final String JSON_PROPERTY_ORGANIZATION = "organization";
private JsonNullable organization = JsonNullable. undefined();
public static final String JSON_PROPERTY_POSTAL_ADDRESS = "postalAddress";
private JsonNullable postalAddress = JsonNullable. undefined();
public static final String JSON_PROPERTY_PREFERRED_LANGUAGE = "preferredLanguage";
private JsonNullable preferredLanguage = JsonNullable. undefined();
public static final String JSON_PROPERTY_PRIMARY_PHONE = "primaryPhone";
private JsonNullable primaryPhone = JsonNullable. undefined();
public static final String JSON_PROPERTY_PROFILE_URL = "profileUrl";
private JsonNullable profileUrl = JsonNullable. undefined();
public static final String JSON_PROPERTY_SECOND_EMAIL = "secondEmail";
private JsonNullable secondEmail = JsonNullable. undefined();
public static final String JSON_PROPERTY_STATE = "state";
private JsonNullable state = JsonNullable. undefined();
public static final String JSON_PROPERTY_STREET_ADDRESS = "streetAddress";
private JsonNullable streetAddress = JsonNullable. undefined();
public static final String JSON_PROPERTY_TIMEZONE = "timezone";
private JsonNullable timezone = JsonNullable. undefined();
public static final String JSON_PROPERTY_TITLE = "title";
private JsonNullable title = JsonNullable. undefined();
public static final String JSON_PROPERTY_USER_TYPE = "userType";
private JsonNullable userType = JsonNullable. undefined();
public static final String JSON_PROPERTY_ZIP_CODE = "zipCode";
private JsonNullable zipCode = JsonNullable. undefined();
public UserProfile() {
}
public UserProfile city(String city) {
this.city = JsonNullable. of(city);
return this;
}
/**
* The city or locality of the User's address (`locality`)
*
* @return city
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The city or locality of the User's address (`locality`)")
@JsonIgnore
public String getCity() {
return city.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCity_JsonNullable() {
return city;
}
@JsonProperty(JSON_PROPERTY_CITY)
public void setCity_JsonNullable(JsonNullable city) {
this.city = city;
}
public void setCity(String city) {
this.city = JsonNullable. of(city);
}
public UserProfile costCenter(String costCenter) {
this.costCenter = JsonNullable. of(costCenter);
return this;
}
/**
* Name of the cost center assigned to a user
*
* @return costCenter
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the cost center assigned to a user")
@JsonIgnore
public String getCostCenter() {
return costCenter.orElse(null);
}
@JsonProperty(JSON_PROPERTY_COST_CENTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCostCenter_JsonNullable() {
return costCenter;
}
@JsonProperty(JSON_PROPERTY_COST_CENTER)
public void setCostCenter_JsonNullable(JsonNullable costCenter) {
this.costCenter = costCenter;
}
public void setCostCenter(String costCenter) {
this.costCenter = JsonNullable. of(costCenter);
}
public UserProfile countryCode(String countryCode) {
this.countryCode = JsonNullable. of(countryCode);
return this;
}
/**
* The country name component of the User's address (`country`). For validation, see [ISO 3166-1 alpha
* 2 \"short\" code
* format](https://datatracker.ietf.org/doc/html/draft-ietf-scim-core-schema-22#ref-ISO3166).
*
* @return countryCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The country name component of the User's address (`country`). For validation, see [ISO 3166-1 alpha 2 \"short\" code format](https://datatracker.ietf.org/doc/html/draft-ietf-scim-core-schema-22#ref-ISO3166).")
@JsonIgnore
public String getCountryCode() {
return countryCode.orElse(null);
}
@JsonProperty(JSON_PROPERTY_COUNTRY_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCountryCode_JsonNullable() {
return countryCode;
}
@JsonProperty(JSON_PROPERTY_COUNTRY_CODE)
public void setCountryCode_JsonNullable(JsonNullable countryCode) {
this.countryCode = countryCode;
}
public void setCountryCode(String countryCode) {
this.countryCode = JsonNullable. of(countryCode);
}
public UserProfile department(String department) {
this.department = department;
return this;
}
/**
* Name of the User's department
*
* @return department
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the User's department")
@JsonProperty(JSON_PROPERTY_DEPARTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDepartment() {
return department;
}
@JsonProperty(JSON_PROPERTY_DEPARTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDepartment(String department) {
this.department = department;
}
public UserProfile displayName(String displayName) {
this.displayName = JsonNullable. of(displayName);
return this;
}
/**
* Name of the User suitable for display to end users
*
* @return displayName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the User suitable for display to end users")
@JsonIgnore
public String getDisplayName() {
return displayName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getDisplayName_JsonNullable() {
return displayName;
}
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
public void setDisplayName_JsonNullable(JsonNullable displayName) {
this.displayName = displayName;
}
public void setDisplayName(String displayName) {
this.displayName = JsonNullable. of(displayName);
}
public UserProfile division(String division) {
this.division = JsonNullable. of(division);
return this;
}
/**
* Name of the User's division
*
* @return division
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the User's division")
@JsonIgnore
public String getDivision() {
return division.orElse(null);
}
@JsonProperty(JSON_PROPERTY_DIVISION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getDivision_JsonNullable() {
return division;
}
@JsonProperty(JSON_PROPERTY_DIVISION)
public void setDivision_JsonNullable(JsonNullable division) {
this.division = division;
}
public void setDivision(String division) {
this.division = JsonNullable. of(division);
}
public UserProfile email(String email) {
this.email = email;
return this;
}
/**
* The primary email address of the user. For validation, see [RFC 5322 Section
* 3.2.3](https://datatracker.ietf.org/doc/html/rfc5322#section-3.2.3).
*
* @return email
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The primary email address of the user. For validation, see [RFC 5322 Section 3.2.3](https://datatracker.ietf.org/doc/html/rfc5322#section-3.2.3).")
@JsonProperty(JSON_PROPERTY_EMAIL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEmail() {
return email;
}
@JsonProperty(JSON_PROPERTY_EMAIL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEmail(String email) {
this.email = email;
}
public UserProfile employeeNumber(String employeeNumber) {
this.employeeNumber = employeeNumber;
return this;
}
/**
* The organization or company assigned unique identifier for the user
*
* @return employeeNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The organization or company assigned unique identifier for the user")
@JsonProperty(JSON_PROPERTY_EMPLOYEE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEmployeeNumber() {
return employeeNumber;
}
@JsonProperty(JSON_PROPERTY_EMPLOYEE_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEmployeeNumber(String employeeNumber) {
this.employeeNumber = employeeNumber;
}
public UserProfile firstName(String firstName) {
this.firstName = JsonNullable. of(firstName);
return this;
}
/**
* Given name of the User (`givenName`)
*
* @return firstName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Given name of the User (`givenName`)")
@JsonIgnore
public String getFirstName() {
return firstName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_FIRST_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getFirstName_JsonNullable() {
return firstName;
}
@JsonProperty(JSON_PROPERTY_FIRST_NAME)
public void setFirstName_JsonNullable(JsonNullable firstName) {
this.firstName = firstName;
}
public void setFirstName(String firstName) {
this.firstName = JsonNullable. of(firstName);
}
public UserProfile honorificPrefix(String honorificPrefix) {
this.honorificPrefix = JsonNullable. of(honorificPrefix);
return this;
}
/**
* Honorific prefix(es) of the user, or title in most Western languages
*
* @return honorificPrefix
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Honorific prefix(es) of the user, or title in most Western languages")
@JsonIgnore
public String getHonorificPrefix() {
return honorificPrefix.orElse(null);
}
@JsonProperty(JSON_PROPERTY_HONORIFIC_PREFIX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getHonorificPrefix_JsonNullable() {
return honorificPrefix;
}
@JsonProperty(JSON_PROPERTY_HONORIFIC_PREFIX)
public void setHonorificPrefix_JsonNullable(JsonNullable honorificPrefix) {
this.honorificPrefix = honorificPrefix;
}
public void setHonorificPrefix(String honorificPrefix) {
this.honorificPrefix = JsonNullable. of(honorificPrefix);
}
public UserProfile honorificSuffix(String honorificSuffix) {
this.honorificSuffix = JsonNullable. of(honorificSuffix);
return this;
}
/**
* Honorific suffix(es) of the User
*
* @return honorificSuffix
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Honorific suffix(es) of the User")
@JsonIgnore
public String getHonorificSuffix() {
return honorificSuffix.orElse(null);
}
@JsonProperty(JSON_PROPERTY_HONORIFIC_SUFFIX)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getHonorificSuffix_JsonNullable() {
return honorificSuffix;
}
@JsonProperty(JSON_PROPERTY_HONORIFIC_SUFFIX)
public void setHonorificSuffix_JsonNullable(JsonNullable honorificSuffix) {
this.honorificSuffix = honorificSuffix;
}
public void setHonorificSuffix(String honorificSuffix) {
this.honorificSuffix = JsonNullable. of(honorificSuffix);
}
public UserProfile lastName(String lastName) {
this.lastName = JsonNullable. of(lastName);
return this;
}
/**
* The family name of the User (`familyName`)
*
* @return lastName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The family name of the User (`familyName`)")
@JsonIgnore
public String getLastName() {
return lastName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_LAST_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getLastName_JsonNullable() {
return lastName;
}
@JsonProperty(JSON_PROPERTY_LAST_NAME)
public void setLastName_JsonNullable(JsonNullable lastName) {
this.lastName = lastName;
}
public void setLastName(String lastName) {
this.lastName = JsonNullable. of(lastName);
}
public UserProfile locale(String locale) {
this.locale = locale;
return this;
}
/**
* The User's default location for purposes of localizing items such as currency, date time format, numerical
* representations, and so on. A locale value is a concatenation of the ISO 639-1 two-letter language code, an
* underscore, and the ISO 3166-1 two-letter country code. For example, en_US specifies the language English and
* country US. This value is `en_US` by default.
*
* @return locale
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The User's default location for purposes of localizing items such as currency, date time format, numerical representations, and so on. A locale value is a concatenation of the ISO 639-1 two-letter language code, an underscore, and the ISO 3166-1 two-letter country code. For example, en_US specifies the language English and country US. This value is `en_US` by default.")
@JsonProperty(JSON_PROPERTY_LOCALE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLocale() {
return locale;
}
@JsonProperty(JSON_PROPERTY_LOCALE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLocale(String locale) {
this.locale = locale;
}
public UserProfile login(String login) {
this.login = login;
return this;
}
/**
* The unique identifier for the User (`username`). For validation, see [Login pattern
* validation](https://developer.okta.com/docs/reference/api/schemas/#login-pattern-validation). Every user within
* your Okta org must have a unique identifier for a login. This constraint applies to all users you import from
* other systems or applications such as Active Directory. Your organization is the top-level namespace to mix and
* match logins from all your connected applications or directories. Careful consideration of naming conventions for
* your login identifier will make it easier to onboard new applications in the future. Logins are not considered
* unique if they differ only in case and/or diacritical marks. If one of your users has a login of
* [email protected], there cannot be another user whose login is [email protected], nor
* isáàc.brö[email protected]. Okta has a default ambiguous name resolution policy for usernames that include @-signs.
* (By default, usernames must be formatted as email addresses and thus always include @-signs. You can remove that
* restriction using either the Admin Console or the Schemas API (opens new window).) Users can sign in with their
* non-qualified short name (for example: isaac.brock with username [email protected]) as long as the short
* name is still unique within the organization. maxLength: 100
*
* @return login
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The unique identifier for the User (`username`). For validation, see [Login pattern validation](https://developer.okta.com/docs/reference/api/schemas/#login-pattern-validation). Every user within your Okta org must have a unique identifier for a login. This constraint applies to all users you import from other systems or applications such as Active Directory. Your organization is the top-level namespace to mix and match logins from all your connected applications or directories. Careful consideration of naming conventions for your login identifier will make it easier to onboard new applications in the future. Logins are not considered unique if they differ only in case and/or diacritical marks. If one of your users has a login of [email protected], there cannot be another user whose login is [email protected], nor isáàc.brö[email protected]. Okta has a default ambiguous name resolution policy for usernames that include @-signs. (By default, usernames must be formatted as email addresses and thus always include @-signs. You can remove that restriction using either the Admin Console or the Schemas API (opens new window).) Users can sign in with their non-qualified short name (for example: isaac.brock with username [email protected]) as long as the short name is still unique within the organization. maxLength: 100")
@JsonProperty(JSON_PROPERTY_LOGIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLogin() {
return login;
}
@JsonProperty(JSON_PROPERTY_LOGIN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLogin(String login) {
this.login = login;
}
public UserProfile manager(String manager) {
this.manager = JsonNullable. of(manager);
return this;
}
/**
* The `displayName` of the User's manager
*
* @return manager
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The `displayName` of the User's manager")
@JsonIgnore
public String getManager() {
return manager.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MANAGER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getManager_JsonNullable() {
return manager;
}
@JsonProperty(JSON_PROPERTY_MANAGER)
public void setManager_JsonNullable(JsonNullable manager) {
this.manager = manager;
}
public void setManager(String manager) {
this.manager = JsonNullable. of(manager);
}
public UserProfile managerId(String managerId) {
this.managerId = JsonNullable. of(managerId);
return this;
}
/**
* The `id` of the User's manager
*
* @return managerId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The `id` of the User's manager")
@JsonIgnore
public String getManagerId() {
return managerId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MANAGER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getManagerId_JsonNullable() {
return managerId;
}
@JsonProperty(JSON_PROPERTY_MANAGER_ID)
public void setManagerId_JsonNullable(JsonNullable managerId) {
this.managerId = managerId;
}
public void setManagerId(String managerId) {
this.managerId = JsonNullable. of(managerId);
}
public UserProfile middleName(String middleName) {
this.middleName = JsonNullable. of(middleName);
return this;
}
/**
* The middle name of the user
*
* @return middleName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The middle name of the user")
@JsonIgnore
public String getMiddleName() {
return middleName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MIDDLE_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getMiddleName_JsonNullable() {
return middleName;
}
@JsonProperty(JSON_PROPERTY_MIDDLE_NAME)
public void setMiddleName_JsonNullable(JsonNullable middleName) {
this.middleName = middleName;
}
public void setMiddleName(String middleName) {
this.middleName = JsonNullable. of(middleName);
}
public UserProfile mobilePhone(String mobilePhone) {
this.mobilePhone = JsonNullable. of(mobilePhone);
return this;
}
/**
* The mobile phone number of the user
*
* @return mobilePhone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The mobile phone number of the user")
@JsonIgnore
public String getMobilePhone() {
return mobilePhone.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MOBILE_PHONE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getMobilePhone_JsonNullable() {
return mobilePhone;
}
@JsonProperty(JSON_PROPERTY_MOBILE_PHONE)
public void setMobilePhone_JsonNullable(JsonNullable mobilePhone) {
this.mobilePhone = mobilePhone;
}
public void setMobilePhone(String mobilePhone) {
this.mobilePhone = JsonNullable. of(mobilePhone);
}
public UserProfile nickName(String nickName) {
this.nickName = JsonNullable. of(nickName);
return this;
}
/**
* The casual way to address the User in real life
*
* @return nickName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The casual way to address the User in real life")
@JsonIgnore
public String getNickName() {
return nickName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_NICK_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getNickName_JsonNullable() {
return nickName;
}
@JsonProperty(JSON_PROPERTY_NICK_NAME)
public void setNickName_JsonNullable(JsonNullable nickName) {
this.nickName = nickName;
}
public void setNickName(String nickName) {
this.nickName = JsonNullable. of(nickName);
}
public UserProfile organization(String organization) {
this.organization = JsonNullable. of(organization);
return this;
}
/**
* Name of the the User's organization
*
* @return organization
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the the User's organization")
@JsonIgnore
public String getOrganization() {
return organization.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ORGANIZATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getOrganization_JsonNullable() {
return organization;
}
@JsonProperty(JSON_PROPERTY_ORGANIZATION)
public void setOrganization_JsonNullable(JsonNullable organization) {
this.organization = organization;
}
public void setOrganization(String organization) {
this.organization = JsonNullable. of(organization);
}
public UserProfile postalAddress(String postalAddress) {
this.postalAddress = JsonNullable. of(postalAddress);
return this;
}
/**
* Mailing address component of the User's address
*
* @return postalAddress
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Mailing address component of the User's address")
@JsonIgnore
public String getPostalAddress() {
return postalAddress.orElse(null);
}
@JsonProperty(JSON_PROPERTY_POSTAL_ADDRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getPostalAddress_JsonNullable() {
return postalAddress;
}
@JsonProperty(JSON_PROPERTY_POSTAL_ADDRESS)
public void setPostalAddress_JsonNullable(JsonNullable postalAddress) {
this.postalAddress = postalAddress;
}
public void setPostalAddress(String postalAddress) {
this.postalAddress = JsonNullable. of(postalAddress);
}
public UserProfile preferredLanguage(String preferredLanguage) {
this.preferredLanguage = JsonNullable. of(preferredLanguage);
return this;
}
/**
* The User's preferred written or spoken language. For validation, see [RFC 7231 Section
* 5.3.5](https://datatracker.ietf.org/doc/html/rfc7231#section-5.3.5).
*
* @return preferredLanguage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The User's preferred written or spoken language. For validation, see [RFC 7231 Section 5.3.5](https://datatracker.ietf.org/doc/html/rfc7231#section-5.3.5).")
@JsonIgnore
public String getPreferredLanguage() {
return preferredLanguage.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PREFERRED_LANGUAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getPreferredLanguage_JsonNullable() {
return preferredLanguage;
}
@JsonProperty(JSON_PROPERTY_PREFERRED_LANGUAGE)
public void setPreferredLanguage_JsonNullable(JsonNullable preferredLanguage) {
this.preferredLanguage = preferredLanguage;
}
public void setPreferredLanguage(String preferredLanguage) {
this.preferredLanguage = JsonNullable. of(preferredLanguage);
}
public UserProfile primaryPhone(String primaryPhone) {
this.primaryPhone = JsonNullable. of(primaryPhone);
return this;
}
/**
* The primary phone number of the User such as a home number
*
* @return primaryPhone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The primary phone number of the User such as a home number")
@JsonIgnore
public String getPrimaryPhone() {
return primaryPhone.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PRIMARY_PHONE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getPrimaryPhone_JsonNullable() {
return primaryPhone;
}
@JsonProperty(JSON_PROPERTY_PRIMARY_PHONE)
public void setPrimaryPhone_JsonNullable(JsonNullable primaryPhone) {
this.primaryPhone = primaryPhone;
}
public void setPrimaryPhone(String primaryPhone) {
this.primaryPhone = JsonNullable. of(primaryPhone);
}
public UserProfile profileUrl(String profileUrl) {
this.profileUrl = JsonNullable. of(profileUrl);
return this;
}
/**
* The URL of the User's online profile. For example, a web page. See
* [URL](https://datatracker.ietf.org/doc/html/rfc1808).
*
* @return profileUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL of the User's online profile. For example, a web page. See [URL](https://datatracker.ietf.org/doc/html/rfc1808).")
@JsonIgnore
public String getProfileUrl() {
return profileUrl.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROFILE_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProfileUrl_JsonNullable() {
return profileUrl;
}
@JsonProperty(JSON_PROPERTY_PROFILE_URL)
public void setProfileUrl_JsonNullable(JsonNullable profileUrl) {
this.profileUrl = profileUrl;
}
public void setProfileUrl(String profileUrl) {
this.profileUrl = JsonNullable. of(profileUrl);
}
public UserProfile secondEmail(String secondEmail) {
this.secondEmail = JsonNullable. of(secondEmail);
return this;
}
/**
* The secondary email address of the User typically used for account recovery. For validation, see [RFC 5322
* Section 3.2.3](https://datatracker.ietf.org/doc/html/rfc5322#section-3.2.3).
*
* @return secondEmail
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The secondary email address of the User typically used for account recovery. For validation, see [RFC 5322 Section 3.2.3](https://datatracker.ietf.org/doc/html/rfc5322#section-3.2.3).")
@JsonIgnore
public String getSecondEmail() {
return secondEmail.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SECOND_EMAIL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSecondEmail_JsonNullable() {
return secondEmail;
}
@JsonProperty(JSON_PROPERTY_SECOND_EMAIL)
public void setSecondEmail_JsonNullable(JsonNullable secondEmail) {
this.secondEmail = secondEmail;
}
public void setSecondEmail(String secondEmail) {
this.secondEmail = JsonNullable. of(secondEmail);
}
public UserProfile state(String state) {
this.state = JsonNullable. of(state);
return this;
}
/**
* The state or region component of the User's address (`region`)
*
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The state or region component of the User's address (`region`)")
@JsonIgnore
public String getState() {
return state.orElse(null);
}
@JsonProperty(JSON_PROPERTY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getState_JsonNullable() {
return state;
}
@JsonProperty(JSON_PROPERTY_STATE)
public void setState_JsonNullable(JsonNullable state) {
this.state = state;
}
public void setState(String state) {
this.state = JsonNullable. of(state);
}
public UserProfile streetAddress(String streetAddress) {
this.streetAddress = JsonNullable. of(streetAddress);
return this;
}
/**
* The full street address component of the User's address
*
* @return streetAddress
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The full street address component of the User's address")
@JsonIgnore
public String getStreetAddress() {
return streetAddress.orElse(null);
}
@JsonProperty(JSON_PROPERTY_STREET_ADDRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getStreetAddress_JsonNullable() {
return streetAddress;
}
@JsonProperty(JSON_PROPERTY_STREET_ADDRESS)
public void setStreetAddress_JsonNullable(JsonNullable streetAddress) {
this.streetAddress = streetAddress;
}
public void setStreetAddress(String streetAddress) {
this.streetAddress = JsonNullable. of(streetAddress);
}
public UserProfile timezone(String timezone) {
this.timezone = JsonNullable. of(timezone);
return this;
}
/**
* The User's time zone
*
* @return timezone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The User's time zone")
@JsonIgnore
public String getTimezone() {
return timezone.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TIMEZONE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getTimezone_JsonNullable() {
return timezone;
}
@JsonProperty(JSON_PROPERTY_TIMEZONE)
public void setTimezone_JsonNullable(JsonNullable timezone) {
this.timezone = timezone;
}
public void setTimezone(String timezone) {
this.timezone = JsonNullable. of(timezone);
}
public UserProfile title(String title) {
this.title = JsonNullable. of(title);
return this;
}
/**
* The User's title, such as Vice President
*
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The User's title, such as Vice President")
@JsonIgnore
public String getTitle() {
return title.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getTitle_JsonNullable() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
public void setTitle_JsonNullable(JsonNullable title) {
this.title = title;
}
public void setTitle(String title) {
this.title = JsonNullable. of(title);
}
public UserProfile userType(String userType) {
this.userType = JsonNullable. of(userType);
return this;
}
/**
* The property used to describe the organization-to-user relationship, such as employee or contractor
*
* @return userType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The property used to describe the organization-to-user relationship, such as employee or contractor")
@JsonIgnore
public String getUserType() {
return userType.orElse(null);
}
@JsonProperty(JSON_PROPERTY_USER_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getUserType_JsonNullable() {
return userType;
}
@JsonProperty(JSON_PROPERTY_USER_TYPE)
public void setUserType_JsonNullable(JsonNullable userType) {
this.userType = userType;
}
public void setUserType(String userType) {
this.userType = JsonNullable. of(userType);
}
public UserProfile zipCode(String zipCode) {
this.zipCode = JsonNullable. of(zipCode);
return this;
}
/**
* The ZIP code or postal code component of the User's address (`postalCode`)
*
* @return zipCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The ZIP code or postal code component of the User's address (`postalCode`)")
@JsonIgnore
public String getZipCode() {
return zipCode.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ZIP_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getZipCode_JsonNullable() {
return zipCode;
}
@JsonProperty(JSON_PROPERTY_ZIP_CODE)
public void setZipCode_JsonNullable(JsonNullable zipCode) {
this.zipCode = zipCode;
}
public void setZipCode(String zipCode) {
this.zipCode = JsonNullable. of(zipCode);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserProfile userProfile = (UserProfile) o;
return equalsNullable(this.city, userProfile.city) && equalsNullable(this.costCenter, userProfile.costCenter)
&& equalsNullable(this.countryCode, userProfile.countryCode)
&& Objects.equals(this.department, userProfile.department)
&& equalsNullable(this.displayName, userProfile.displayName)
&& equalsNullable(this.division, userProfile.division) && Objects.equals(this.email, userProfile.email)
&& Objects.equals(this.employeeNumber, userProfile.employeeNumber)
&& equalsNullable(this.firstName, userProfile.firstName)
&& equalsNullable(this.honorificPrefix, userProfile.honorificPrefix)
&& equalsNullable(this.honorificSuffix, userProfile.honorificSuffix)
&& equalsNullable(this.lastName, userProfile.lastName)
&& Objects.equals(this.locale, userProfile.locale) && Objects.equals(this.login, userProfile.login)
&& equalsNullable(this.manager, userProfile.manager)
&& equalsNullable(this.managerId, userProfile.managerId)
&& equalsNullable(this.middleName, userProfile.middleName)
&& equalsNullable(this.mobilePhone, userProfile.mobilePhone)
&& equalsNullable(this.nickName, userProfile.nickName)
&& equalsNullable(this.organization, userProfile.organization)
&& equalsNullable(this.postalAddress, userProfile.postalAddress)
&& equalsNullable(this.preferredLanguage, userProfile.preferredLanguage)
&& equalsNullable(this.primaryPhone, userProfile.primaryPhone)
&& equalsNullable(this.profileUrl, userProfile.profileUrl)
&& equalsNullable(this.secondEmail, userProfile.secondEmail)
&& equalsNullable(this.state, userProfile.state)
&& equalsNullable(this.streetAddress, userProfile.streetAddress)
&& equalsNullable(this.timezone, userProfile.timezone) && equalsNullable(this.title, userProfile.title)
&& equalsNullable(this.userType, userProfile.userType)
&& equalsNullable(this.zipCode, userProfile.zipCode);
// && super.equals(o);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b
|| (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(city), hashCodeNullable(costCenter), hashCodeNullable(countryCode),
department, hashCodeNullable(displayName), hashCodeNullable(division), email, employeeNumber,
hashCodeNullable(firstName), hashCodeNullable(honorificPrefix), hashCodeNullable(honorificSuffix),
hashCodeNullable(lastName), locale, login, hashCodeNullable(manager), hashCodeNullable(managerId),
hashCodeNullable(middleName), hashCodeNullable(mobilePhone), hashCodeNullable(nickName),
hashCodeNullable(organization), hashCodeNullable(postalAddress), hashCodeNullable(preferredLanguage),
hashCodeNullable(primaryPhone), hashCodeNullable(profileUrl), hashCodeNullable(secondEmail),
hashCodeNullable(state), hashCodeNullable(streetAddress), hashCodeNullable(timezone),
hashCodeNullable(title), hashCodeNullable(userType), hashCodeNullable(zipCode), super.hashCode());
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[] { a.get() }) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserProfile {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" costCenter: ").append(toIndentedString(costCenter)).append("\n");
sb.append(" countryCode: ").append(toIndentedString(countryCode)).append("\n");
sb.append(" department: ").append(toIndentedString(department)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" division: ").append(toIndentedString(division)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" employeeNumber: ").append(toIndentedString(employeeNumber)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" honorificPrefix: ").append(toIndentedString(honorificPrefix)).append("\n");
sb.append(" honorificSuffix: ").append(toIndentedString(honorificSuffix)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" locale: ").append(toIndentedString(locale)).append("\n");
sb.append(" login: ").append(toIndentedString(login)).append("\n");
sb.append(" manager: ").append(toIndentedString(manager)).append("\n");
sb.append(" managerId: ").append(toIndentedString(managerId)).append("\n");
sb.append(" middleName: ").append(toIndentedString(middleName)).append("\n");
sb.append(" mobilePhone: ").append(toIndentedString(mobilePhone)).append("\n");
sb.append(" nickName: ").append(toIndentedString(nickName)).append("\n");
sb.append(" organization: ").append(toIndentedString(organization)).append("\n");
sb.append(" postalAddress: ").append(toIndentedString(postalAddress)).append("\n");
sb.append(" preferredLanguage: ").append(toIndentedString(preferredLanguage)).append("\n");
sb.append(" primaryPhone: ").append(toIndentedString(primaryPhone)).append("\n");
sb.append(" profileUrl: ").append(toIndentedString(profileUrl)).append("\n");
sb.append(" secondEmail: ").append(toIndentedString(secondEmail)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" streetAddress: ").append(toIndentedString(streetAddress)).append("\n");
sb.append(" timezone: ").append(toIndentedString(timezone)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" userType: ").append(toIndentedString(userType)).append("\n");
sb.append(" zipCode: ").append(toIndentedString(zipCode)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy