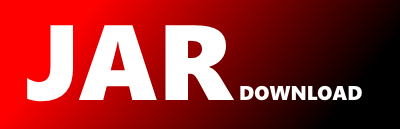
com.okta.sdk.resource.model.ZscalerbyzApplication Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
package com.okta.sdk.resource.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.okta.sdk.resource.model.ApplicationAccessibility;
import com.okta.sdk.resource.model.ApplicationLicensing;
import com.okta.sdk.resource.model.ApplicationLifecycleStatus;
import com.okta.sdk.resource.model.ApplicationVisibility;
import com.okta.sdk.resource.model.SchemeApplicationCredentials;
import com.okta.sdk.resource.model.ZscalerbyzApplicationSettings;
import java.util.HashMap;
import java.util.Map;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.annotations.ApiModel;
/**
* Schema for the Zscaler 2.0 app (key name: `zscalerbyz`) To create a Zscaler 2.0 app, use the [Create an
* Application](/openapi/okta-management/management/tag/Application/#tag/Application/operation/createApplication)
* request with the following parameters in the request body. > **Note:** The Zscaler 2.0 app only supports
* `BROWSER_PLUGIN` and `SAML_2_0` sign-on modes.
*/
@ApiModel(description = "Schema for the Zscaler 2.0 app (key name: `zscalerbyz`) To create a Zscaler 2.0 app, use the [Create an Application](/openapi/okta-management/management/tag/Application/#tag/Application/operation/createApplication) request with the following parameters in the request body. > **Note:** The Zscaler 2.0 app only supports `BROWSER_PLUGIN` and `SAML_2_0` sign-on modes. ")
@JsonPropertyOrder({ ZscalerbyzApplication.JSON_PROPERTY_ACCESSIBILITY, ZscalerbyzApplication.JSON_PROPERTY_CREDENTIALS,
ZscalerbyzApplication.JSON_PROPERTY_LABEL, ZscalerbyzApplication.JSON_PROPERTY_LICENSING,
ZscalerbyzApplication.JSON_PROPERTY_NAME, ZscalerbyzApplication.JSON_PROPERTY_PROFILE,
ZscalerbyzApplication.JSON_PROPERTY_SIGN_ON_MODE, ZscalerbyzApplication.JSON_PROPERTY_STATUS,
ZscalerbyzApplication.JSON_PROPERTY_VISIBILITY, ZscalerbyzApplication.JSON_PROPERTY_SETTINGS })
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-15T08:48:47.130589-06:00[America/Chicago]", comments = "Generator version: 7.8.0")
public class ZscalerbyzApplication implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ACCESSIBILITY = "accessibility";
private ApplicationAccessibility accessibility;
public static final String JSON_PROPERTY_CREDENTIALS = "credentials";
private SchemeApplicationCredentials credentials;
public static final String JSON_PROPERTY_LABEL = "label";
private String label;
public static final String JSON_PROPERTY_LICENSING = "licensing";
private ApplicationLicensing licensing;
/**
* Gets or Sets name
*/
public enum NameEnum {
ZSCALERBYZ("zscalerbyz"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
NameEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static NameEnum fromValue(String value) {
for (NameEnum b : NameEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_NAME = "name";
private NameEnum name;
public static final String JSON_PROPERTY_PROFILE = "profile";
private Map profile = null;
/**
* Gets or Sets signOnMode
*/
public enum SignOnModeEnum {
BROWSER_PLUGIN("BROWSER_PLUGIN"),
SAML_2_0("SAML_2_0"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
SignOnModeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SignOnModeEnum fromValue(String value) {
for (SignOnModeEnum b : SignOnModeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_SIGN_ON_MODE = "signOnMode";
private SignOnModeEnum signOnMode;
public static final String JSON_PROPERTY_STATUS = "status";
private ApplicationLifecycleStatus status;
public static final String JSON_PROPERTY_VISIBILITY = "visibility";
private ApplicationVisibility visibility;
public static final String JSON_PROPERTY_SETTINGS = "settings";
private ZscalerbyzApplicationSettings settings;
public ZscalerbyzApplication() {
}
public ZscalerbyzApplication accessibility(ApplicationAccessibility accessibility) {
this.accessibility = accessibility;
return this;
}
/**
* Get accessibility
*
* @return accessibility
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ACCESSIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationAccessibility getAccessibility() {
return accessibility;
}
@JsonProperty(JSON_PROPERTY_ACCESSIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccessibility(ApplicationAccessibility accessibility) {
this.accessibility = accessibility;
}
public ZscalerbyzApplication credentials(SchemeApplicationCredentials credentials) {
this.credentials = credentials;
return this;
}
/**
* Get credentials
*
* @return credentials
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SchemeApplicationCredentials getCredentials() {
return credentials;
}
@JsonProperty(JSON_PROPERTY_CREDENTIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCredentials(SchemeApplicationCredentials credentials) {
this.credentials = credentials;
}
public ZscalerbyzApplication label(String label) {
this.label = label;
return this;
}
/**
* User-defined display name for app
*
* @return label
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "User-defined display name for app")
@JsonProperty(JSON_PROPERTY_LABEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getLabel() {
return label;
}
@JsonProperty(JSON_PROPERTY_LABEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLabel(String label) {
this.label = label;
}
public ZscalerbyzApplication licensing(ApplicationLicensing licensing) {
this.licensing = licensing;
return this;
}
/**
* Get licensing
*
* @return licensing
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LICENSING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationLicensing getLicensing() {
return licensing;
}
@JsonProperty(JSON_PROPERTY_LICENSING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLicensing(ApplicationLicensing licensing) {
this.licensing = licensing;
}
public ZscalerbyzApplication name(NameEnum name) {
this.name = name;
return this;
}
/**
* Get name
*
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "zscalerbyz", required = true, value = "")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public NameEnum getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(NameEnum name) {
this.name = name;
}
public ZscalerbyzApplication profile(Map profile) {
this.profile = profile;
return this;
}
public ZscalerbyzApplication putprofileItem(String key, Object profileItem) {
if (this.profile == null) {
this.profile = new HashMap<>();
}
this.profile.put(key, profileItem);
return this;
}
/**
* Contains any valid JSON schema for specifying properties that can be referenced from a request (only available to
* OAuth 2.0 client apps)
*
* @return profile
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Contains any valid JSON schema for specifying properties that can be referenced from a request (only available to OAuth 2.0 client apps)")
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getProfile() {
return profile;
}
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProfile(Map profile) {
this.profile = profile;
}
public ZscalerbyzApplication signOnMode(SignOnModeEnum signOnMode) {
this.signOnMode = signOnMode;
return this;
}
/**
* Get signOnMode
*
* @return signOnMode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SIGN_ON_MODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SignOnModeEnum getSignOnMode() {
return signOnMode;
}
@JsonProperty(JSON_PROPERTY_SIGN_ON_MODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSignOnMode(SignOnModeEnum signOnMode) {
this.signOnMode = signOnMode;
}
public ZscalerbyzApplication status(ApplicationLifecycleStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationLifecycleStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStatus(ApplicationLifecycleStatus status) {
this.status = status;
}
public ZscalerbyzApplication visibility(ApplicationVisibility visibility) {
this.visibility = visibility;
return this;
}
/**
* Get visibility
*
* @return visibility
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ApplicationVisibility getVisibility() {
return visibility;
}
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVisibility(ApplicationVisibility visibility) {
this.visibility = visibility;
}
public ZscalerbyzApplication settings(ZscalerbyzApplicationSettings settings) {
this.settings = settings;
return this;
}
/**
* Get settings
*
* @return settings
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_SETTINGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ZscalerbyzApplicationSettings getSettings() {
return settings;
}
@JsonProperty(JSON_PROPERTY_SETTINGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSettings(ZscalerbyzApplicationSettings settings) {
this.settings = settings;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ZscalerbyzApplication zscalerbyzApplication = (ZscalerbyzApplication) o;
return Objects.equals(this.accessibility, zscalerbyzApplication.accessibility)
&& Objects.equals(this.credentials, zscalerbyzApplication.credentials)
&& Objects.equals(this.label, zscalerbyzApplication.label)
&& Objects.equals(this.licensing, zscalerbyzApplication.licensing)
&& Objects.equals(this.name, zscalerbyzApplication.name)
&& Objects.equals(this.profile, zscalerbyzApplication.profile)
&& Objects.equals(this.signOnMode, zscalerbyzApplication.signOnMode)
&& Objects.equals(this.status, zscalerbyzApplication.status)
&& Objects.equals(this.visibility, zscalerbyzApplication.visibility)
&& Objects.equals(this.settings, zscalerbyzApplication.settings);
// ;
}
@Override
public int hashCode() {
return Objects.hash(accessibility, credentials, label, licensing, name, profile, signOnMode, status, visibility,
settings);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ZscalerbyzApplication {\n");
sb.append(" accessibility: ").append(toIndentedString(accessibility)).append("\n");
sb.append(" credentials: ").append(toIndentedString(credentials)).append("\n");
sb.append(" label: ").append(toIndentedString(label)).append("\n");
sb.append(" licensing: ").append(toIndentedString(licensing)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" profile: ").append(toIndentedString(profile)).append("\n");
sb.append(" signOnMode: ").append(toIndentedString(signOnMode)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append(" settings: ").append(toIndentedString(settings)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy