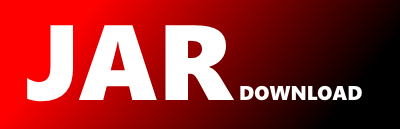
docs.AgentPoolsApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# AgentPoolsApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activateAgentPoolsUpdate**](AgentPoolsApi.md#activateAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates/{updateId}/activate | Activate an Agent Pool update |
| [**createAgentPoolsUpdate**](AgentPoolsApi.md#createAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates | Create an Agent Pool update |
| [**deactivateAgentPoolsUpdate**](AgentPoolsApi.md#deactivateAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates/{updateId}/deactivate | Deactivate an Agent Pool update |
| [**deleteAgentPoolsUpdate**](AgentPoolsApi.md#deleteAgentPoolsUpdate) | **DELETE** /api/v1/agentPools/{poolId}/updates/{updateId} | Delete an Agent Pool update |
| [**getAgentPoolsUpdateInstance**](AgentPoolsApi.md#getAgentPoolsUpdateInstance) | **GET** /api/v1/agentPools/{poolId}/updates/{updateId} | Retrieve an Agent Pool update by id |
| [**getAgentPoolsUpdateSettings**](AgentPoolsApi.md#getAgentPoolsUpdateSettings) | **GET** /api/v1/agentPools/{poolId}/updates/settings | Retrieve an Agent Pool update's settings |
| [**listAgentPools**](AgentPoolsApi.md#listAgentPools) | **GET** /api/v1/agentPools | List all Agent Pools |
| [**listAgentPoolsUpdates**](AgentPoolsApi.md#listAgentPoolsUpdates) | **GET** /api/v1/agentPools/{poolId}/updates | List all Agent Pool updates |
| [**pauseAgentPoolsUpdate**](AgentPoolsApi.md#pauseAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates/{updateId}/pause | Pause an Agent Pool update |
| [**resumeAgentPoolsUpdate**](AgentPoolsApi.md#resumeAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates/{updateId}/resume | Resume an Agent Pool update |
| [**retryAgentPoolsUpdate**](AgentPoolsApi.md#retryAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates/{updateId}/retry | Retry an Agent Pool update |
| [**stopAgentPoolsUpdate**](AgentPoolsApi.md#stopAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates/{updateId}/stop | Stop an Agent Pool update |
| [**updateAgentPoolsUpdate**](AgentPoolsApi.md#updateAgentPoolsUpdate) | **POST** /api/v1/agentPools/{poolId}/updates/{updateId} | Update an Agent Pool update by id |
| [**updateAgentPoolsUpdateSettings**](AgentPoolsApi.md#updateAgentPoolsUpdateSettings) | **POST** /api/v1/agentPools/{poolId}/updates/settings | Update an Agent Pool update settings |
## activateAgentPoolsUpdate
> AgentPoolUpdate activateAgentPoolsUpdate(poolId, updateId)
Activate an Agent Pool update
Activates scheduled Agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
AgentPoolUpdate result = apiInstance.activateAgentPoolsUpdate(poolId, updateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#activateAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Activated | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## createAgentPoolsUpdate
> AgentPoolUpdate createAgentPoolsUpdate(poolId, agentPoolUpdate)
Create an Agent Pool update
Creates an Agent pool update \\n For user flow 2 manual update, starts the update immediately. \\n For user flow 3, schedules the update based on the configured update window and delay.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
AgentPoolUpdate agentPoolUpdate = new AgentPoolUpdate(); // AgentPoolUpdate |
try {
AgentPoolUpdate result = apiInstance.createAgentPoolsUpdate(poolId, agentPoolUpdate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#createAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **agentPoolUpdate** | [**AgentPoolUpdate**](AgentPoolUpdate.md)| | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deactivateAgentPoolsUpdate
> AgentPoolUpdate deactivateAgentPoolsUpdate(poolId, updateId)
Deactivate an Agent Pool update
Deactivates scheduled Agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
AgentPoolUpdate result = apiInstance.deactivateAgentPoolsUpdate(poolId, updateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#deactivateAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Deactivated | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteAgentPoolsUpdate
> deleteAgentPoolsUpdate(poolId, updateId)
Delete an Agent Pool update
Deletes Agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
apiInstance.deleteAgentPoolsUpdate(poolId, updateId);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#deleteAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | Deleted | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getAgentPoolsUpdateInstance
> AgentPoolUpdate getAgentPoolsUpdateInstance(poolId, updateId)
Retrieve an Agent Pool update by id
Retrieves Agent pool update from updateId
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
AgentPoolUpdate result = apiInstance.getAgentPoolsUpdateInstance(poolId, updateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#getAgentPoolsUpdateInstance");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getAgentPoolsUpdateSettings
> AgentPoolUpdateSetting getAgentPoolsUpdateSettings(poolId)
Retrieve an Agent Pool update's settings
Retrieves the current state of the agent pool update instance settings
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
try {
AgentPoolUpdateSetting result = apiInstance.getAgentPoolsUpdateSettings(poolId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#getAgentPoolsUpdateSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
### Return type
[**AgentPoolUpdateSetting**](AgentPoolUpdateSetting.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listAgentPools
> List<AgentPool> listAgentPools(limitPerPoolType, poolType, after)
List all Agent Pools
Lists all agent pools with pagination support
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
Integer limitPerPoolType = 5; // Integer | Maximum number of AgentPools being returned
AgentType poolType = AgentType.fromValue("AD"); // AgentType | Agent type to search for
String after = "after_example"; // String | The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination).
try {
List result = apiInstance.listAgentPools(limitPerPoolType, poolType, after);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#listAgentPools");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **limitPerPoolType** | **Integer**| Maximum number of AgentPools being returned | [optional] [default to 5] |
| **poolType** | [**AgentType**](.md)| Agent type to search for | [optional] [enum: AD, IWA, LDAP, MFA, OPP, RUM, Radius] |
| **after** | **String**| The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
### Return type
[**List<AgentPool>**](AgentPool.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## listAgentPoolsUpdates
> List<AgentPoolUpdate> listAgentPoolsUpdates(poolId, scheduled)
List all Agent Pool updates
Lists all agent pool updates
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
Boolean scheduled = true; // Boolean | Scope the list only to scheduled or ad-hoc updates. If the parameter is not provided we will return the whole list of updates.
try {
List result = apiInstance.listAgentPoolsUpdates(poolId, scheduled);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#listAgentPoolsUpdates");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **scheduled** | **Boolean**| Scope the list only to scheduled or ad-hoc updates. If the parameter is not provided we will return the whole list of updates. | [optional] |
### Return type
[**List<AgentPoolUpdate>**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## pauseAgentPoolsUpdate
> AgentPoolUpdate pauseAgentPoolsUpdate(poolId, updateId)
Pause an Agent Pool update
Pauses running or queued Agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
AgentPoolUpdate result = apiInstance.pauseAgentPoolsUpdate(poolId, updateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#pauseAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Paused | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## resumeAgentPoolsUpdate
> AgentPoolUpdate resumeAgentPoolsUpdate(poolId, updateId)
Resume an Agent Pool update
Resumes running or queued Agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
AgentPoolUpdate result = apiInstance.resumeAgentPoolsUpdate(poolId, updateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#resumeAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Resumed | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## retryAgentPoolsUpdate
> AgentPoolUpdate retryAgentPoolsUpdate(poolId, updateId)
Retry an Agent Pool update
Retries Agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
AgentPoolUpdate result = apiInstance.retryAgentPoolsUpdate(poolId, updateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#retryAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Retried | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## stopAgentPoolsUpdate
> AgentPoolUpdate stopAgentPoolsUpdate(poolId, updateId)
Stop an Agent Pool update
Stops Agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
try {
AgentPoolUpdate result = apiInstance.stopAgentPoolsUpdate(poolId, updateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#stopAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Stopped | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## updateAgentPoolsUpdate
> AgentPoolUpdate updateAgentPoolsUpdate(poolId, updateId, agentPoolUpdate)
Update an Agent Pool update by id
Updates Agent pool update and return latest agent pool update
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
String updateId = "updateId_example"; // String | Id of the update
AgentPoolUpdate agentPoolUpdate = new AgentPoolUpdate(); // AgentPoolUpdate |
try {
AgentPoolUpdate result = apiInstance.updateAgentPoolsUpdate(poolId, updateId, agentPoolUpdate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#updateAgentPoolsUpdate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **updateId** | **String**| Id of the update | |
| **agentPoolUpdate** | [**AgentPoolUpdate**](AgentPoolUpdate.md)| | |
### Return type
[**AgentPoolUpdate**](AgentPoolUpdate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Updated | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## updateAgentPoolsUpdateSettings
> AgentPoolUpdateSetting updateAgentPoolsUpdateSettings(poolId, agentPoolUpdateSetting)
Update an Agent Pool update settings
Updates an agent pool update settings
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AgentPoolsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AgentPoolsApi apiInstance = new AgentPoolsApi(defaultClient);
String poolId = "poolId_example"; // String | Id of the agent pool for which the settings will apply
AgentPoolUpdateSetting agentPoolUpdateSetting = new AgentPoolUpdateSetting(); // AgentPoolUpdateSetting |
try {
AgentPoolUpdateSetting result = apiInstance.updateAgentPoolsUpdateSettings(poolId, agentPoolUpdateSetting);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AgentPoolsApi#updateAgentPoolsUpdateSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **poolId** | **String**| Id of the agent pool for which the settings will apply | |
| **agentPoolUpdateSetting** | [**AgentPoolUpdateSetting**](AgentPoolUpdateSetting.md)| | |
### Return type
[**AgentPoolUpdateSetting**](AgentPoolUpdateSetting.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Updated | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy