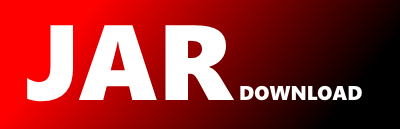
docs.ApplicationSsoCredentialOAuth2ClientAuthApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
# ApplicationSsoCredentialOAuth2ClientAuthApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activateOAuth2ClientJsonWebKey**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#activateOAuth2ClientJsonWebKey) | **POST** /api/v1/apps/{appId}/credentials/jwks/{keyId}/lifecycle/activate | Activate an OAuth 2.0 Client JSON Web Key |
| [**activateOAuth2ClientSecret**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#activateOAuth2ClientSecret) | **POST** /api/v1/apps/{appId}/credentials/secrets/{secretId}/lifecycle/activate | Activate an OAuth 2.0 Client Secret |
| [**addJwk**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#addJwk) | **POST** /api/v1/apps/{appId}/credentials/jwks | Add a JSON Web Key |
| [**createOAuth2ClientSecret**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#createOAuth2ClientSecret) | **POST** /api/v1/apps/{appId}/credentials/secrets | Create an OAuth 2.0 Client Secret |
| [**deactivateOAuth2ClientJsonWebKey**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#deactivateOAuth2ClientJsonWebKey) | **POST** /api/v1/apps/{appId}/credentials/jwks/{keyId}/lifecycle/deactivate | Deactivate an OAuth 2.0 Client JSON Web Key |
| [**deactivateOAuth2ClientSecret**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#deactivateOAuth2ClientSecret) | **POST** /api/v1/apps/{appId}/credentials/secrets/{secretId}/lifecycle/deactivate | Deactivate an OAuth 2.0 Client Secret |
| [**deleteOAuth2ClientSecret**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#deleteOAuth2ClientSecret) | **DELETE** /api/v1/apps/{appId}/credentials/secrets/{secretId} | Delete an OAuth 2.0 Client Secret |
| [**deletejwk**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#deletejwk) | **DELETE** /api/v1/apps/{appId}/credentials/jwks/{keyId} | Delete an OAuth 2.0 Client JSON Web Key |
| [**getJwk**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#getJwk) | **GET** /api/v1/apps/{appId}/credentials/jwks/{keyId} | Retrieve an OAuth 2.0 Client JSON Web Key |
| [**getOAuth2ClientSecret**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#getOAuth2ClientSecret) | **GET** /api/v1/apps/{appId}/credentials/secrets/{secretId} | Retrieve an OAuth 2.0 Client Secret |
| [**listJwk**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#listJwk) | **GET** /api/v1/apps/{appId}/credentials/jwks | List all the OAuth 2.0 Client JSON Web Keys |
| [**listOAuth2ClientSecrets**](ApplicationSsoCredentialOAuth2ClientAuthApi.md#listOAuth2ClientSecrets) | **GET** /api/v1/apps/{appId}/credentials/secrets | List all OAuth 2.0 Client Secrets |
## activateOAuth2ClientJsonWebKey
> OAuth2ClientJsonWebKey activateOAuth2ClientJsonWebKey(appId, keyId)
Activate an OAuth 2.0 Client JSON Web Key
Activates an OAuth 2.0 Client JSON Web Key by `keyId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String keyId = "pks2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client JSON Web Key
try {
OAuth2ClientJsonWebKey result = apiInstance.activateOAuth2ClientJsonWebKey(appId, keyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#activateOAuth2ClientJsonWebKey");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **keyId** | **String**| Unique `id` of the OAuth 2.0 Client JSON Web Key | |
### Return type
[**OAuth2ClientJsonWebKey**](OAuth2ClientJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## activateOAuth2ClientSecret
> OAuth2ClientSecret activateOAuth2ClientSecret(appId, secretId)
Activate an OAuth 2.0 Client Secret
Activates an OAuth 2.0 Client Secret by `secretId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String secretId = "ocs2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client Secret
try {
OAuth2ClientSecret result = apiInstance.activateOAuth2ClientSecret(appId, secretId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#activateOAuth2ClientSecret");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **secretId** | **String**| Unique `id` of the OAuth 2.0 Client Secret | |
### Return type
[**OAuth2ClientSecret**](OAuth2ClientSecret.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## addJwk
> OAuth2ClientJsonWebKey addJwk(appId, oauth2ClientJsonWebKeyRequestBody)
Add a JSON Web Key
Adds a new JSON Web Key to the client’s JSON Web Keys. > **Note:** This API doesn't allow you to add a key if the existing key doesn't have a `kid`. This is also consistent with how the [Dynamic Client Registration](/openapi/okta-oauth/oauth/tag/Client/) or [Applications](/openapi/okta-management/management/tag/Application/) APIs behave, as they don't allow the creation of multiple keys without `kids`. Use the [Replace an Application](/openapi/okta-management/management/tag/Application/#tag/Application/operation/replaceApplication) or the [Replace a Client Application](/openapi/okta-oauth/oauth/tag/Client/#tag/Client/operation/replaceClient) operation to update the JWKS or [Delete an OAuth 2.0 Client JSON Web Key](/openapi/okta-management/management/tag/ApplicationSSOCredentialOAuth2ClientAuth/#tag/ApplicationSSOCredentialOAuth2ClientAuth/operation/deletejwk) and re-add the key with a `kid`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
OAuth2ClientJsonWebKeyRequestBody oauth2ClientJsonWebKeyRequestBody = new OAuth2ClientJsonWebKeyRequestBody(); // OAuth2ClientJsonWebKeyRequestBody |
try {
OAuth2ClientJsonWebKey result = apiInstance.addJwk(appId, oauth2ClientJsonWebKeyRequestBody);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#addJwk");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **oauth2ClientJsonWebKeyRequestBody** | [**OAuth2ClientJsonWebKeyRequestBody**](OAuth2ClientJsonWebKeyRequestBody.md)| | |
### Return type
[**OAuth2ClientJsonWebKey**](OAuth2ClientJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## createOAuth2ClientSecret
> OAuth2ClientSecret createOAuth2ClientSecret(appId, oauth2ClientSecretRequestBody)
Create an OAuth 2.0 Client Secret
Creates an OAuth 2.0 Client Secret object with a new active client secret. You can create up to two Secret objects. An error is returned if you attempt to create more than two Secret objects. > **Note:** This API lets you bring your own secret. If [token_endpoint_auth_method](/openapi/okta-management/management/tag/Application/#tag/Application/operation/createApplication!path=4/credentials/oauthClient/token_endpoint_auth_method&t=request) of the app is `client_secret_jwt`, then the minimum length of `client_secret` is 32 characters. If no secret is specified in the request, Okta adds a new system-generated secret.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
OAuth2ClientSecretRequestBody oauth2ClientSecretRequestBody = new OAuth2ClientSecretRequestBody(); // OAuth2ClientSecretRequestBody |
try {
OAuth2ClientSecret result = apiInstance.createOAuth2ClientSecret(appId, oauth2ClientSecretRequestBody);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#createOAuth2ClientSecret");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **oauth2ClientSecretRequestBody** | [**OAuth2ClientSecretRequestBody**](OAuth2ClientSecretRequestBody.md)| | [optional] |
### Return type
[**OAuth2ClientSecret**](OAuth2ClientSecret.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## deactivateOAuth2ClientJsonWebKey
> OAuth2ClientJsonWebKey deactivateOAuth2ClientJsonWebKey(appId, keyId)
Deactivate an OAuth 2.0 Client JSON Web Key
Deactivates an OAuth 2.0 Client JSON Web Key by `keyId`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String keyId = "pks2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client JSON Web Key
try {
OAuth2ClientJsonWebKey result = apiInstance.deactivateOAuth2ClientJsonWebKey(appId, keyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#deactivateOAuth2ClientJsonWebKey");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **keyId** | **String**| Unique `id` of the OAuth 2.0 Client JSON Web Key | |
### Return type
[**OAuth2ClientJsonWebKey**](OAuth2ClientJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **400** | Bad Request | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deactivateOAuth2ClientSecret
> OAuth2ClientSecret deactivateOAuth2ClientSecret(appId, secretId)
Deactivate an OAuth 2.0 Client Secret
Deactivates an OAuth 2.0 Client Secret by `secretId`. You can't deactivate a secret if it's the only secret of the client.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String secretId = "ocs2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client Secret
try {
OAuth2ClientSecret result = apiInstance.deactivateOAuth2ClientSecret(appId, secretId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#deactivateOAuth2ClientSecret");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **secretId** | **String**| Unique `id` of the OAuth 2.0 Client Secret | |
### Return type
[**OAuth2ClientSecret**](OAuth2ClientSecret.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **400** | Bad Request | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteOAuth2ClientSecret
> deleteOAuth2ClientSecret(appId, secretId)
Delete an OAuth 2.0 Client Secret
Deletes an OAuth 2.0 Client Secret by `secretId`. You can only delete an inactive Secret.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String secretId = "ocs2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client Secret
try {
apiInstance.deleteOAuth2ClientSecret(appId, secretId);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#deleteOAuth2ClientSecret");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **secretId** | **String**| Unique `id` of the OAuth 2.0 Client Secret | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **400** | Bad Request | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deletejwk
> deletejwk(appId, keyId)
Delete an OAuth 2.0 Client JSON Web Key
Deletes an OAuth 2.0 Client JSON Web Key by `keyId`. You can only delete an inactive key.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String keyId = "pks2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client JSON Web Key
try {
apiInstance.deletejwk(appId, keyId);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#deletejwk");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **keyId** | **String**| Unique `id` of the OAuth 2.0 Client JSON Web Key | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **400** | Bad Request | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getJwk
> OAuth2ClientJsonWebKey getJwk(appId, keyId)
Retrieve an OAuth 2.0 Client JSON Web Key
Retrieves an OAuth 2.0 Client JSON Web Key by `keyId`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String keyId = "pks2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client JSON Web Key
try {
OAuth2ClientJsonWebKey result = apiInstance.getJwk(appId, keyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#getJwk");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **keyId** | **String**| Unique `id` of the OAuth 2.0 Client JSON Web Key | |
### Return type
[**OAuth2ClientJsonWebKey**](OAuth2ClientJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getOAuth2ClientSecret
> OAuth2ClientSecret getOAuth2ClientSecret(appId, secretId)
Retrieve an OAuth 2.0 Client Secret
Retrieves an OAuth 2.0 Client Secret by `secretId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
String secretId = "ocs2f4zrZbs8nUa7p0g4"; // String | Unique `id` of the OAuth 2.0 Client Secret
try {
OAuth2ClientSecret result = apiInstance.getOAuth2ClientSecret(appId, secretId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#getOAuth2ClientSecret");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **secretId** | **String**| Unique `id` of the OAuth 2.0 Client Secret | |
### Return type
[**OAuth2ClientSecret**](OAuth2ClientSecret.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listJwk
> List<OAuth2ClientJsonWebKey> listJwk(appId)
List all the OAuth 2.0 Client JSON Web Keys
Lists all JSON Web Keys for an OAuth 2.0 client app
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
try {
List result = apiInstance.listJwk(appId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#listJwk");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
### Return type
[**List<OAuth2ClientJsonWebKey>**](OAuth2ClientJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listOAuth2ClientSecrets
> List<OAuth2ClientSecret> listOAuth2ClientSecrets(appId)
List all OAuth 2.0 Client Secrets
Lists all client secrets for an OAuth 2.0 client app
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ApplicationSsoCredentialOAuth2ClientAuthApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ApplicationSsoCredentialOAuth2ClientAuthApi apiInstance = new ApplicationSsoCredentialOAuth2ClientAuthApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
try {
List result = apiInstance.listOAuth2ClientSecrets(appId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ApplicationSsoCredentialOAuth2ClientAuthApi#listOAuth2ClientSecrets");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
### Return type
[**List<OAuth2ClientSecret>**](OAuth2ClientSecret.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **401** | Unauthorized | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy