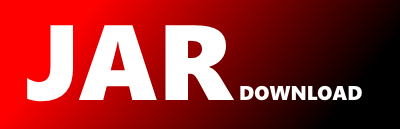
docs.AuthenticatorApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# AuthenticatorApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activateAuthenticator**](AuthenticatorApi.md#activateAuthenticator) | **POST** /api/v1/authenticators/{authenticatorId}/lifecycle/activate | Activate an Authenticator |
| [**activateAuthenticatorMethod**](AuthenticatorApi.md#activateAuthenticatorMethod) | **POST** /api/v1/authenticators/{authenticatorId}/methods/{methodType}/lifecycle/activate | Activate an Authenticator Method |
| [**createAuthenticator**](AuthenticatorApi.md#createAuthenticator) | **POST** /api/v1/authenticators | Create an Authenticator |
| [**deactivateAuthenticator**](AuthenticatorApi.md#deactivateAuthenticator) | **POST** /api/v1/authenticators/{authenticatorId}/lifecycle/deactivate | Deactivate an Authenticator |
| [**deactivateAuthenticatorMethod**](AuthenticatorApi.md#deactivateAuthenticatorMethod) | **POST** /api/v1/authenticators/{authenticatorId}/methods/{methodType}/lifecycle/deactivate | Deactivate an Authenticator Method |
| [**getAuthenticator**](AuthenticatorApi.md#getAuthenticator) | **GET** /api/v1/authenticators/{authenticatorId} | Retrieve an Authenticator |
| [**getAuthenticatorMethod**](AuthenticatorApi.md#getAuthenticatorMethod) | **GET** /api/v1/authenticators/{authenticatorId}/methods/{methodType} | Retrieve an Authenticator Method |
| [**getWellKnownAppAuthenticatorConfiguration**](AuthenticatorApi.md#getWellKnownAppAuthenticatorConfiguration) | **GET** /.well-known/app-authenticator-configuration | Retrieve the Well-Known App Authenticator Configuration |
| [**listAuthenticatorMethods**](AuthenticatorApi.md#listAuthenticatorMethods) | **GET** /api/v1/authenticators/{authenticatorId}/methods | List all Methods of an Authenticator |
| [**listAuthenticators**](AuthenticatorApi.md#listAuthenticators) | **GET** /api/v1/authenticators | List all Authenticators |
| [**replaceAuthenticator**](AuthenticatorApi.md#replaceAuthenticator) | **PUT** /api/v1/authenticators/{authenticatorId} | Replace an Authenticator |
| [**replaceAuthenticatorMethod**](AuthenticatorApi.md#replaceAuthenticatorMethod) | **PUT** /api/v1/authenticators/{authenticatorId}/methods/{methodType} | Replace an Authenticator Method |
## activateAuthenticator
> AuthenticatorBase activateAuthenticator(authenticatorId)
Activate an Authenticator
Activates an authenticator by `authenticatorId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
try {
AuthenticatorBase result = apiInstance.activateAuthenticator(authenticatorId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#activateAuthenticator");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
### Return type
[**AuthenticatorBase**](AuthenticatorBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## activateAuthenticatorMethod
> AuthenticatorMethodBase activateAuthenticatorMethod(authenticatorId, methodType)
Activate an Authenticator Method
Activates a Method for an Authenticator identified by `authenticatorId` and `methodType` > **Note:** <x-lifecycle class=\"ea\"></x-lifecycle> > The AAGUID Group object supports the Early Access (Self-Service) Allow List for FIDO2 (WebAuthn) Authenticators feature. Enable the feature for your org from the **Settings** > **Features** page in the Admin Console. > This feature has several limitations when enrolling a security key: > - Enrollment is currently unsupported on Firefox. > - Enrollment is currently unsupported on Chrome if User Verification is set to DISCOURAGED and a PIN is set on the security key. > - If prompted during enrollment, users must allow Okta to see the make and model of the security key.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
AuthenticatorMethodType methodType = AuthenticatorMethodType.fromValue("cert"); // AuthenticatorMethodType | Type of authenticator method
try {
AuthenticatorMethodBase result = apiInstance.activateAuthenticatorMethod(authenticatorId, methodType);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#activateAuthenticatorMethod");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
| **methodType** | [**AuthenticatorMethodType**](.md)| Type of authenticator method | [enum: cert, duo, email, idp, otp, password, push, security_question, signed_nonce, sms, totp, voice, webauthn] |
### Return type
[**AuthenticatorMethodBase**](AuthenticatorMethodBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## createAuthenticator
> AuthenticatorBase createAuthenticator(authenticator, activate)
Create an Authenticator
Creates an authenticator
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
AuthenticatorBase authenticator = new AuthenticatorBase(); // AuthenticatorBase |
Boolean activate = true; // Boolean | Whether to execute the activation lifecycle operation when Okta creates the authenticator
try {
AuthenticatorBase result = apiInstance.createAuthenticator(authenticator, activate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#createAuthenticator");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticator** | [**AuthenticatorBase**](AuthenticatorBase.md)| | |
| **activate** | **Boolean**| Whether to execute the activation lifecycle operation when Okta creates the authenticator | [optional] [default to true] |
### Return type
[**AuthenticatorBase**](AuthenticatorBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## deactivateAuthenticator
> AuthenticatorBase deactivateAuthenticator(authenticatorId)
Deactivate an Authenticator
Deactivates an authenticator by `authenticatorId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
try {
AuthenticatorBase result = apiInstance.deactivateAuthenticator(authenticatorId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#deactivateAuthenticator");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
### Return type
[**AuthenticatorBase**](AuthenticatorBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deactivateAuthenticatorMethod
> AuthenticatorMethodBase deactivateAuthenticatorMethod(authenticatorId, methodType)
Deactivate an Authenticator Method
Deactivates a Method for an Authenticator identified by `authenticatorId` and `methodType` > **Note:** <x-lifecycle class=\"ea\"></x-lifecycle> > The AAGUID Group object supports the Early Access (Self-Service) Allow List for FIDO2 (WebAuthn) Authenticators feature. Enable the feature for your org from the **Settings** > **Features** page in the Admin Console. > This feature has several limitations when enrolling a security key: > - Enrollment is currently unsupported on Firefox. > - Enrollment is currently unsupported on Chrome if User Verification is set to DISCOURAGED and a PIN is set on the security key. > - If prompted during enrollment, users must allow Okta to see the make and model of the security key.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
AuthenticatorMethodType methodType = AuthenticatorMethodType.fromValue("cert"); // AuthenticatorMethodType | Type of authenticator method
try {
AuthenticatorMethodBase result = apiInstance.deactivateAuthenticatorMethod(authenticatorId, methodType);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#deactivateAuthenticatorMethod");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
| **methodType** | [**AuthenticatorMethodType**](.md)| Type of authenticator method | [enum: cert, duo, email, idp, otp, password, push, security_question, signed_nonce, sms, totp, voice, webauthn] |
### Return type
[**AuthenticatorMethodBase**](AuthenticatorMethodBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getAuthenticator
> AuthenticatorBase getAuthenticator(authenticatorId)
Retrieve an Authenticator
Retrieves an authenticator from your Okta organization by `authenticatorId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
try {
AuthenticatorBase result = apiInstance.getAuthenticator(authenticatorId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#getAuthenticator");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
### Return type
[**AuthenticatorBase**](AuthenticatorBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getAuthenticatorMethod
> AuthenticatorMethodBase getAuthenticatorMethod(authenticatorId, methodType)
Retrieve an Authenticator Method
Retrieves a Method identified by `methodType` of an Authenticator identified by `authenticatorId` > **Note:** <x-lifecycle class=\"ea\"></x-lifecycle> > The AAGUID Group object supports the Early Access (Self-Service) Allow List for FIDO2 (WebAuthn) Authenticators feature. Enable the feature for your org from the **Settings** > **Features** page in the Admin Console. > This feature has several limitations when enrolling a security key: > - Enrollment is currently unsupported on Firefox. > - Enrollment is currently unsupported on Chrome if User Verification is set to DISCOURAGED and a PIN is set on the security key. > - If prompted during enrollment, users must allow Okta to see the make and model of the security key.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
AuthenticatorMethodType methodType = AuthenticatorMethodType.fromValue("cert"); // AuthenticatorMethodType | Type of authenticator method
try {
AuthenticatorMethodBase result = apiInstance.getAuthenticatorMethod(authenticatorId, methodType);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#getAuthenticatorMethod");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
| **methodType** | [**AuthenticatorMethodType**](.md)| Type of authenticator method | [enum: cert, duo, email, idp, otp, password, push, security_question, signed_nonce, sms, totp, voice, webauthn] |
### Return type
[**AuthenticatorMethodBase**](AuthenticatorMethodBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getWellKnownAppAuthenticatorConfiguration
> List<WellKnownAppAuthenticatorConfiguration> getWellKnownAppAuthenticatorConfiguration(oauthClientId)
Retrieve the Well-Known App Authenticator Configuration
Retrieves the well-known app authenticator configuration. Includes an app authenticator's settings, supported methods, and other details.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String oauthClientId = "oauthClientId_example"; // String | Filters app authenticator configurations by `oauthClientId`
try {
List result = apiInstance.getWellKnownAppAuthenticatorConfiguration(oauthClientId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#getWellKnownAppAuthenticatorConfiguration");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **oauthClientId** | **String**| Filters app authenticator configurations by `oauthClientId` | |
### Return type
[**List<WellKnownAppAuthenticatorConfiguration>**](WellKnownAppAuthenticatorConfiguration.md)
### Authorization
No authorization required
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **429** | Too Many Requests | - |
## listAuthenticatorMethods
> List<AuthenticatorMethodBase> listAuthenticatorMethods(authenticatorId)
List all Methods of an Authenticator
Lists all Methods of an Authenticator identified by `authenticatorId` > **Note:** <x-lifecycle class=\"ea\"></x-lifecycle> > The AAGUID Group object supports the Early Access (Self-Service) Allow List for FIDO2 (WebAuthn) Authenticators feature. Enable the feature for your org from the **Settings** > **Features** page in the Admin Console. > This feature has several limitations when enrolling a security key: > - Enrollment is currently unsupported on Firefox. > - Enrollment is currently unsupported on Chrome if User Verification is set to DISCOURAGED and a PIN is set on the security key. > - If prompted during enrollment, users must allow Okta to see the make and model of the security key.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
try {
List result = apiInstance.listAuthenticatorMethods(authenticatorId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#listAuthenticatorMethods");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
### Return type
[**List<AuthenticatorMethodBase>**](AuthenticatorMethodBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listAuthenticators
> List<AuthenticatorBase> listAuthenticators()
List all Authenticators
Lists all authenticators
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
try {
List result = apiInstance.listAuthenticators();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#listAuthenticators");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**List<AuthenticatorBase>**](AuthenticatorBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## replaceAuthenticator
> AuthenticatorBase replaceAuthenticator(authenticatorId, authenticator)
Replace an Authenticator
Replaces the properties for an Authenticator identified by `authenticatorId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
AuthenticatorBase authenticator = new AuthenticatorBase(); // AuthenticatorBase |
try {
AuthenticatorBase result = apiInstance.replaceAuthenticator(authenticatorId, authenticator);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#replaceAuthenticator");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
| **authenticator** | [**AuthenticatorBase**](AuthenticatorBase.md)| | |
### Return type
[**AuthenticatorBase**](AuthenticatorBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replaceAuthenticatorMethod
> AuthenticatorMethodBase replaceAuthenticatorMethod(authenticatorId, methodType, authenticatorMethodBase)
Replace an Authenticator Method
Replaces a Method of `methodType` for an Authenticator identified by `authenticatorId` > **Note:** <x-lifecycle class=\"ea\"></x-lifecycle> > The AAGUID Group object supports the Early Access (Self-Service) Allow List for FIDO2 (WebAuthn) Authenticators feature. Enable the feature for your org from the **Settings** > **Features** page in the Admin Console. > This feature has several limitations when enrolling a security key: > - Enrollment is currently unsupported on Firefox. > - Enrollment is currently unsupported on Chrome if User Verification is set to DISCOURAGED and a PIN is set on the security key. > - If prompted during enrollment, users must allow Okta to see the make and model of the security key.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthenticatorApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthenticatorApi apiInstance = new AuthenticatorApi(defaultClient);
String authenticatorId = "aut1nd8PQhGcQtSxB0g4"; // String | `id` of the Authenticator
AuthenticatorMethodType methodType = AuthenticatorMethodType.fromValue("cert"); // AuthenticatorMethodType | Type of authenticator method
AuthenticatorMethodBase authenticatorMethodBase = new AuthenticatorMethodBase(); // AuthenticatorMethodBase |
try {
AuthenticatorMethodBase result = apiInstance.replaceAuthenticatorMethod(authenticatorId, methodType, authenticatorMethodBase);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthenticatorApi#replaceAuthenticatorMethod");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authenticatorId** | **String**| `id` of the Authenticator | |
| **methodType** | [**AuthenticatorMethodType**](.md)| Type of authenticator method | [enum: cert, duo, email, idp, otp, password, push, security_question, signed_nonce, sms, totp, voice, webauthn] |
| **authenticatorMethodBase** | [**AuthenticatorMethodBase**](AuthenticatorMethodBase.md)| | [optional] |
### Return type
[**AuthenticatorMethodBase**](AuthenticatorMethodBase.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy