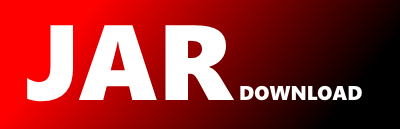
docs.AuthorizationServerKeysApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
# AuthorizationServerKeysApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**getAuthorizationServerKey**](AuthorizationServerKeysApi.md#getAuthorizationServerKey) | **GET** /api/v1/authorizationServers/{authServerId}/credentials/keys/{keyId} | Retrieve an Authorization Server Key |
| [**listAuthorizationServerKeys**](AuthorizationServerKeysApi.md#listAuthorizationServerKeys) | **GET** /api/v1/authorizationServers/{authServerId}/credentials/keys | List all Credential Keys |
| [**rotateAuthorizationServerKeys**](AuthorizationServerKeysApi.md#rotateAuthorizationServerKeys) | **POST** /api/v1/authorizationServers/{authServerId}/credentials/lifecycle/keyRotate | Rotate all Credential Keys |
## getAuthorizationServerKey
> AuthorizationServerJsonWebKey getAuthorizationServerKey(authServerId, keyId)
Retrieve an Authorization Server Key
Retrieves an Authorization Server Key specified by the `keyId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerKeysApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerKeysApi apiInstance = new AuthorizationServerKeysApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
String keyId = "P7jXpG-LG2ObNgY9C0Mn2uf4InCQTmRZMDCZoVNxdrk"; // String | `id` of the certificate key
try {
AuthorizationServerJsonWebKey result = apiInstance.getAuthorizationServerKey(authServerId, keyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerKeysApi#getAuthorizationServerKey");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **keyId** | **String**| `id` of the certificate key | |
### Return type
[**AuthorizationServerJsonWebKey**](AuthorizationServerJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listAuthorizationServerKeys
> List<AuthorizationServerJsonWebKey> listAuthorizationServerKeys(authServerId)
List all Credential Keys
Lists all of the current, future, and expired Keys used by the Custom Authorization Server
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerKeysApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerKeysApi apiInstance = new AuthorizationServerKeysApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
try {
List result = apiInstance.listAuthorizationServerKeys(authServerId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerKeysApi#listAuthorizationServerKeys");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
### Return type
[**List<AuthorizationServerJsonWebKey>**](AuthorizationServerJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## rotateAuthorizationServerKeys
> List<AuthorizationServerJsonWebKey> rotateAuthorizationServerKeys(authServerId, use)
Rotate all Credential Keys
Rotates the current Keys for a Custom Authorization Server. If you rotate Keys, the `ACTIVE` Key becomes the `EXPIRED` Key, the `NEXT` Key becomes the `ACTIVE` Key, and the Custom Authorization Server immediately begins using the new active Key to sign tokens. > **Note:** Okta rotates your Keys automatically in `AUTO` mode. You can rotate Keys yourself in either mode. If Keys are rotated manually, you should invalidate any intermediate cache and fetch the Keys again using the Keys endpoint.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerKeysApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerKeysApi apiInstance = new AuthorizationServerKeysApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
JwkUse use = new JwkUse(); // JwkUse |
try {
List result = apiInstance.rotateAuthorizationServerKeys(authServerId, use);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerKeysApi#rotateAuthorizationServerKeys");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **use** | [**JwkUse**](JwkUse.md)| | |
### Return type
[**List<AuthorizationServerJsonWebKey>**](AuthorizationServerJsonWebKey.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy