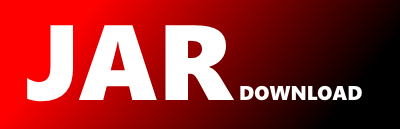
docs.AuthorizationServerPoliciesApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
# AuthorizationServerPoliciesApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activateAuthorizationServerPolicy**](AuthorizationServerPoliciesApi.md#activateAuthorizationServerPolicy) | **POST** /api/v1/authorizationServers/{authServerId}/policies/{policyId}/lifecycle/activate | Activate a Policy |
| [**createAuthorizationServerPolicy**](AuthorizationServerPoliciesApi.md#createAuthorizationServerPolicy) | **POST** /api/v1/authorizationServers/{authServerId}/policies | Create a Policy |
| [**deactivateAuthorizationServerPolicy**](AuthorizationServerPoliciesApi.md#deactivateAuthorizationServerPolicy) | **POST** /api/v1/authorizationServers/{authServerId}/policies/{policyId}/lifecycle/deactivate | Deactivate a Policy |
| [**deleteAuthorizationServerPolicy**](AuthorizationServerPoliciesApi.md#deleteAuthorizationServerPolicy) | **DELETE** /api/v1/authorizationServers/{authServerId}/policies/{policyId} | Delete a Policy |
| [**getAuthorizationServerPolicy**](AuthorizationServerPoliciesApi.md#getAuthorizationServerPolicy) | **GET** /api/v1/authorizationServers/{authServerId}/policies/{policyId} | Retrieve a Policy |
| [**listAuthorizationServerPolicies**](AuthorizationServerPoliciesApi.md#listAuthorizationServerPolicies) | **GET** /api/v1/authorizationServers/{authServerId}/policies | List all Policies |
| [**replaceAuthorizationServerPolicy**](AuthorizationServerPoliciesApi.md#replaceAuthorizationServerPolicy) | **PUT** /api/v1/authorizationServers/{authServerId}/policies/{policyId} | Replace a Policy |
## activateAuthorizationServerPolicy
> activateAuthorizationServerPolicy(authServerId, policyId)
Activate a Policy
Activates an authorization server policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerPoliciesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerPoliciesApi apiInstance = new AuthorizationServerPoliciesApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
apiInstance.activateAuthorizationServerPolicy(authServerId, policyId);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerPoliciesApi#activateAuthorizationServerPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **policyId** | **String**| `id` of the Policy | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## createAuthorizationServerPolicy
> AuthorizationServerPolicy createAuthorizationServerPolicy(authServerId, policy)
Create a Policy
Creates a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerPoliciesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerPoliciesApi apiInstance = new AuthorizationServerPoliciesApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
AuthorizationServerPolicy policy = new AuthorizationServerPolicy(); // AuthorizationServerPolicy |
try {
AuthorizationServerPolicy result = apiInstance.createAuthorizationServerPolicy(authServerId, policy);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerPoliciesApi#createAuthorizationServerPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **policy** | [**AuthorizationServerPolicy**](AuthorizationServerPolicy.md)| | |
### Return type
[**AuthorizationServerPolicy**](AuthorizationServerPolicy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deactivateAuthorizationServerPolicy
> deactivateAuthorizationServerPolicy(authServerId, policyId)
Deactivate a Policy
Deactivates an authorization server policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerPoliciesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerPoliciesApi apiInstance = new AuthorizationServerPoliciesApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
apiInstance.deactivateAuthorizationServerPolicy(authServerId, policyId);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerPoliciesApi#deactivateAuthorizationServerPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **policyId** | **String**| `id` of the Policy | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteAuthorizationServerPolicy
> deleteAuthorizationServerPolicy(authServerId, policyId)
Delete a Policy
Deletes a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerPoliciesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerPoliciesApi apiInstance = new AuthorizationServerPoliciesApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
apiInstance.deleteAuthorizationServerPolicy(authServerId, policyId);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerPoliciesApi#deleteAuthorizationServerPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **policyId** | **String**| `id` of the Policy | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getAuthorizationServerPolicy
> AuthorizationServerPolicy getAuthorizationServerPolicy(authServerId, policyId)
Retrieve a Policy
Retrieves a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerPoliciesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerPoliciesApi apiInstance = new AuthorizationServerPoliciesApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
AuthorizationServerPolicy result = apiInstance.getAuthorizationServerPolicy(authServerId, policyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerPoliciesApi#getAuthorizationServerPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **policyId** | **String**| `id` of the Policy | |
### Return type
[**AuthorizationServerPolicy**](AuthorizationServerPolicy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listAuthorizationServerPolicies
> List<AuthorizationServerPolicy> listAuthorizationServerPolicies(authServerId)
List all Policies
Lists all policies
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerPoliciesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerPoliciesApi apiInstance = new AuthorizationServerPoliciesApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
try {
List result = apiInstance.listAuthorizationServerPolicies(authServerId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerPoliciesApi#listAuthorizationServerPolicies");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
### Return type
[**List<AuthorizationServerPolicy>**](AuthorizationServerPolicy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replaceAuthorizationServerPolicy
> AuthorizationServerPolicy replaceAuthorizationServerPolicy(authServerId, policyId, policy)
Replace a Policy
Replaces a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.AuthorizationServerPoliciesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
AuthorizationServerPoliciesApi apiInstance = new AuthorizationServerPoliciesApi(defaultClient);
String authServerId = "GeGRTEr7f3yu2n7grw22"; // String | `id` of the Authorization Server
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
AuthorizationServerPolicy policy = new AuthorizationServerPolicy(); // AuthorizationServerPolicy |
try {
AuthorizationServerPolicy result = apiInstance.replaceAuthorizationServerPolicy(authServerId, policyId, policy);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling AuthorizationServerPoliciesApi#replaceAuthorizationServerPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **authServerId** | **String**| `id` of the Authorization Server | |
| **policyId** | **String**| `id` of the Policy | |
| **policy** | [**AuthorizationServerPolicy**](AuthorizationServerPolicy.md)| | |
### Return type
[**AuthorizationServerPolicy**](AuthorizationServerPolicy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy