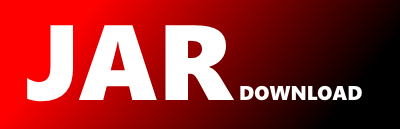
docs.BrandsApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# BrandsApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**createBrand**](BrandsApi.md#createBrand) | **POST** /api/v1/brands | Create a Brand |
| [**deleteBrand**](BrandsApi.md#deleteBrand) | **DELETE** /api/v1/brands/{brandId} | Delete a brand |
| [**getBrand**](BrandsApi.md#getBrand) | **GET** /api/v1/brands/{brandId} | Retrieve a Brand |
| [**listBrandDomains**](BrandsApi.md#listBrandDomains) | **GET** /api/v1/brands/{brandId}/domains | List all Domains associated with a Brand |
| [**listBrands**](BrandsApi.md#listBrands) | **GET** /api/v1/brands | List all Brands |
| [**replaceBrand**](BrandsApi.md#replaceBrand) | **PUT** /api/v1/brands/{brandId} | Replace a Brand |
## createBrand
> Brand createBrand(createBrandRequest)
Create a Brand
Creates a new brand in your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.BrandsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
BrandsApi apiInstance = new BrandsApi(defaultClient);
CreateBrandRequest createBrandRequest = new CreateBrandRequest(); // CreateBrandRequest |
try {
Brand result = apiInstance.createBrand(createBrandRequest);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling BrandsApi#createBrand");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **createBrandRequest** | [**CreateBrandRequest**](CreateBrandRequest.md)| | [optional] |
### Return type
[**Brand**](Brand.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Successfully created the brand | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **409** | Could not create the new brand because same name already exist. | - |
| **429** | Too Many Requests | - |
## deleteBrand
> deleteBrand(brandId)
Delete a brand
Deletes a brand by `brandId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.BrandsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
BrandsApi apiInstance = new BrandsApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
apiInstance.deleteBrand(brandId);
} catch (ApiException e) {
System.err.println("Exception when calling BrandsApi#deleteBrand");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | Successfully deleted the brand. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **409** | Conflict | - |
| **429** | Too Many Requests | - |
## getBrand
> BrandWithEmbedded getBrand(brandId, expand)
Retrieve a Brand
Retrieves a brand by `brandId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.BrandsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
BrandsApi apiInstance = new BrandsApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
List expand = Arrays.asList(); // List | Specifies additional metadata to be included in the response
try {
BrandWithEmbedded result = apiInstance.getBrand(brandId, expand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling BrandsApi#getBrand");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **expand** | [**List<String>**](String.md)| Specifies additional metadata to be included in the response | [optional] [enum: themes, domains, emailDomain] |
### Return type
[**BrandWithEmbedded**](BrandWithEmbedded.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the brand | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listBrandDomains
> List<DomainResponse> listBrandDomains(brandId)
List all Domains associated with a Brand
Lists all domains associated with a brand by `brandId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.BrandsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
BrandsApi apiInstance = new BrandsApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
List result = apiInstance.listBrandDomains(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling BrandsApi#listBrandDomains");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**List<DomainResponse>**](DomainResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully returned the list of domains for the brand | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listBrands
> List<BrandWithEmbedded> listBrands(expand, after, limit, q)
List all Brands
Lists all the brands in your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.BrandsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
BrandsApi apiInstance = new BrandsApi(defaultClient);
List expand = Arrays.asList(); // List | Specifies additional metadata to be included in the response
String after = "after_example"; // String | The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination).
Integer limit = 20; // Integer | A limit on the number of objects to return
String q = "q_example"; // String | Searches the records for matching value
try {
List result = apiInstance.listBrands(expand, after, limit, q);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling BrandsApi#listBrands");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **expand** | [**List<String>**](String.md)| Specifies additional metadata to be included in the response | [optional] [enum: themes, domains, emailDomain] |
| **after** | **String**| The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
| **limit** | **Integer**| A limit on the number of objects to return | [optional] [default to 20] |
| **q** | **String**| Searches the records for matching value | [optional] |
### Return type
[**List<BrandWithEmbedded>**](BrandWithEmbedded.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully returned the list of brands | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## replaceBrand
> Brand replaceBrand(brandId, brand)
Replace a Brand
Replaces a brand by `brandId` Passing an invalid `brandId` returns a `404 Not Found` status code with the error code `E0000007`. Not providing `agreeToCustomPrivacyPolicy` with `customPrivacyPolicyUrl` returns a `400 Bad Request` status code with the error code `E0000001`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.BrandsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
BrandsApi apiInstance = new BrandsApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
BrandRequest brand = new BrandRequest(); // BrandRequest |
try {
Brand result = apiInstance.replaceBrand(brandId, brand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling BrandsApi#replaceBrand");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **brand** | [**BrandRequest**](BrandRequest.md)| | |
### Return type
[**Brand**](Brand.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully replaced the brand | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy